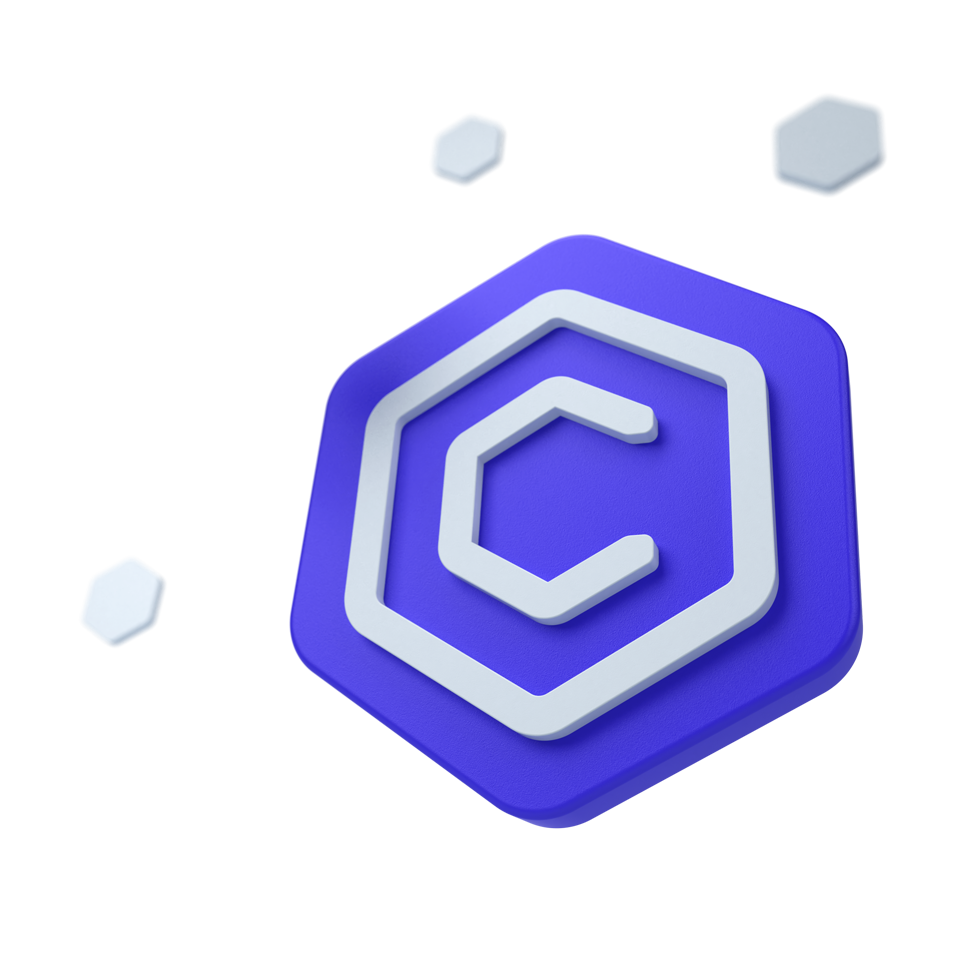
Bootstrap 5 Calendar
Calendar
The Bootstrap Calendar Component is a versatile, customizable tool for creating responsive calendars in Bootstrap, supporting day, month, and year selection, and global locales.
🤖 Looking for the LLM-optimized version? View llm.md
Other frameworks
CoreUI components are available as native Angular, React, and Vue components. To learn more please visit the following pages.
Example
Explore the Bootstrap 5 Calendar component’s basic usage through sample code snippets demonstrating its core functionality.
Days
Select specific days using the Bootstrap Calendar component. The example below shows basic usage.
<div class="d-flex justify-content-center">
<div
class="border rounded"
data-coreui-locale="en-US"
data-coreui-start-date="2024/02/13"
data-coreui-toggle="calendar"
></div>
</div>
Weeks
Set the data-coreui-selection-type
to week
to enable selection of entire week. You can also use data-coreui-show-week-number="true"
to show week numbers.
<div class="d-flex justify-content-center">
<div
class="border rounded"
data-coreui-locale="en-US"
data-coreui-selection-type="week"
data-coreui-show-week-number="true"
data-coreui-start-date="2024W15"
data-coreui-toggle="calendar"
></div>
</div>
Months
Set the data-coreui-selection-type
to month
to enable selection of entire months.
<div class="d-flex justify-content-center">
<div
class="border rounded"
data-coreui-locale="en-US"
data-coreui-selection-type="month"
data-coreui-start-date="2024-2"
data-coreui-toggle="calendar"
></div>
</div>
Years
Set the data-coreui-selection-type
to year
to enable years range selection.
<div class="d-flex justify-content-center">
<div
class="border rounded"
data-coreui-locale="en-US"
data-coreui-selection-type="year"
data-coreui-start-date="2024"
data-coreui-toggle="calendar"
></div>
</div>
Multiple calendar panels
Display multiple calendar panels side by side by setting the data-coreui-calendars
attribute. This can be useful for selecting ranges or comparing dates across different months.
<div class="d-flex justify-content-center">
<div
class="border rounded"
data-coreui-calendars="2"
data-coreui-locale="en-US"
data-coreui-toggle="calendar"
></div>
</div>
Range selection
Enable range selection by adding data-coreui-range="true"
to allow users to pick a start and end date. This example demonstrates how to configure the Bootstrap 5 Calendar component to handle date ranges.
<div class="d-flex justify-content-center">
<div
class="border rounded"
data-coreui-locale="en-US"
data-coreui-calendars="2"
data-coreui-range="true"
data-coreui-start-date="2022/08/23"
data-coreui-end-date="2022/09/08"
data-coreui-toggle="calendar"
></div>
</div>
Disabled dates
The Bootstrap Calendar component includes functionality to disable specific dates, such as weekends or holidays, using the disabledDates
option. It accepts:
- A single
Date
or an array ofDate
objects. - A function or an array of functions that take a
Date
object as an argument and return a boolean indicating whether the date should be disabled. - A mixed array of
Date
objects and functions.
To disable certain dates, you can provide them in an array. For date ranges, use nested arrays, where each inner array indicates a start date and an end date for that range:
<div class="d-flex justify-content-center">
<div id="myCalendarDisabledDates" class="border rounded"></div>
</div>
const myCalendarDisabledDates = document.getElementById('myCalendarDisabledDates')
if (myCalendarDisabledDates) {
const optionsCalendarDisabledDates = {
calendarDate: new Date(2022, 2, 1),
calendars: 2,
disabledDates: [
[new Date(2022, 2, 4), new Date(2022, 2, 7)],
new Date(2022, 2, 16),
new Date(2022, 3, 16),
[new Date(2022, 4, 2), new Date(2022, 4, 8)]
],
locale: 'en-US',
maxDate: new Date(2022, 5, 0),
minDate: new Date(2022, 1, 1)
}
new coreui.Calendar(myCalendarDisabledDates, optionsCalendarDisabledDates)
}
Disabling weekends
To disable weekends, provide a function for the disabledDates
option. Here’s the method:
<div class="d-flex justify-content-center">
<div id="myCalendarDisabledDates2" class="border rounded"></div>
</div>
const myCalendarDisabledDates2 = document.getElementById('myCalendarDisabledDates2')
if (myCalendarDisabledDates2) {
const disableWeekends = date => {
const day = date.getDay()
return day === 0 || day === 6
}
const optionsCalendarDisabledDates2 = {
calendars: 2,
disabledDates: disableWeekends,
locale: 'en-US'
}
new coreui.Calendar(myCalendarDisabledDates2, optionsCalendarDisabledDates2)
}
In the example above:
disableWeekends
is a function that checks if a date falls on a Saturday (6
) or a Sunday (0
).- The
disabledDates
option utilizes thedisableWeekends
function to disable all weekends in the calendar.
Combining functions and specific dates
You can also combine specific dates and functions in the disabledDates
array. For instance:
<div class="d-flex justify-content-center">
<div id="myCalendarDisabledDates3" class="border rounded"></div>
</div>
const myCalendarDisabledDates3 = document.getElementById('myCalendarDisabledDates3')
if (myCalendarDisabledDates3) {
const disableWeekends = date => {
const day = date.getDay()
return day === 0 || day === 6
}
const specificDates = [
new Date(2024, 10, 25),
new Date(2024, 11, 4),
new Date(2024, 11, 12)
]
const optionsCalendarDisabledDates3 = {
calendarDate: new Date(2024, 10, 1),
calendars: 2,
disabledDates: [disableWeekends, ...specificDates],
locale: 'en-US'
}
new coreui.Calendar(myCalendarDisabledDates3, optionsCalendarDisabledDates3)
}
In this example:
disableWeekends
disables weekends as before.specificDates
is an array of specific dates to disable.- The
disabledDates
option combines both, allowing you to disable weekends and specific dates simultaneously.
Non-english locale
The CoreUI Bootstrap Calendar allows users to display dates in non-English locales, making it suitable for international applications.
Auto
By default, the Calendar component uses the browser’s default locale. However, you can easily configure it to use a different locale supported by the JavaScript Internationalization API. This feature helps create inclusive and accessible applications for a diverse audience.
<div class="d-flex justify-content-center">
<div class="border rounded" data-coreui-toggle="calendar"></div>
</div>
Chinese
Here is an example of the Bootstrap Calendar component with Chinese locale settings.
<div class="d-flex justify-content-center">
<div class="border rounded" data-coreui-locale="zh-CN" data-coreui-toggle="calendar"></div>
</div>
Japanese
Below is an example of the Calendar component with Japanese locale settings.
<div class="d-flex justify-content-center">
<div class="border rounded" data-coreui-locale="ja" data-coreui-toggle="calendar"></div>
</div>
Korean
Here is an example of the Calendar component with Korean locale settings.
<div class="d-flex justify-content-center" dir="rtl">
<div class="border rounded" data-coreui-locale="ko" data-coreui-toggle="calendar"></div>
</div>
Right to left support
RTL support is built-in and can be explicitly controlled through the $enable-rtl
variables in scss.
Hebrew
Example of the Calendar component with RTL support, using the Hebrew locale.
<div class="d-flex justify-content-center" dir="rtl">
<div class="border rounded" data-coreui-locale="he-IL" data-coreui-toggle="calendar"></div>
</div>
Persian
Example of the Bootstrap Calendar component with Persian locale settings.
<div class="d-flex justify-content-center">
<div class="border rounded" data-coreui-locale="fa-IR" data-coreui-toggle="calendar"></div>
</div>
Usage
Heads up! In our documentation, all examples show standard CoreUI implementation. If you are using a Bootstrap-compatible version of CoreUI, remember to use the following changes:
- In the constructor, please use bootstrap instead of coreui. For example,
new bootstrap.Alert(...)
instead ofnew coreui.Alert(...)
- In events, please use bs instead of coreui, for example
close.bs.alert
instead ofclose.coreui.alert
- In data attributes, please use bs instead of coreui. For example,
data-bs-toggle="..."
instead ofdata-coreui-toggle="..."
Via data attributes
Add data-coreui-toggle="calendar"
to a div
element.
<div data-coreui-toggle="calendar"></div>
Via JavaScript
Call the time picker via JavaScript:
<div class="calendar"></div>
const calendarElementList = Array.prototype.slice.call(document.querySelectorAll('.calendar'))
const calendarList = calendarElementList.map(calendarEl => {
return new coreui.Calendar(calendarEl)
})
Options
Options can be passed using data attributes or JavaScript. To do this, append an option name to data-coreui-
, such as data-coreui-animation="{value}"
. Remember to convert the case of the option name from “camelCase” to “kebab-case” when using data attributes. For instance, you should write data-coreui-custom-class="beautifier"
rather than data-coreui-customClass="beautifier"
.
Starting with CoreUI 4.2.0, all components support an experimental reserved data attribute named data-coreui-config
, which can contain simple component configurations as a JSON string. If an element has attributes data-coreui-config='{"delay":50, "title":689}'
and data-coreui-title="Custom Title"
, then the final value for title
will be Custom Title
, as the standard data attributes will take precedence over values specified in data-coreui-config
. Moreover, existing data attributes can also hold JSON values like data-coreui-delay='{"show":50, "hide":250}'
.
Name | Type | Default | Description |
---|---|---|---|
ariaNavNextMonthLabel |
string | 'Next month' |
A string that provides an accessible label for the button that navigates to the next month in the calendar. This label is read by screen readers to describe the action associated with the button. |
ariaNavNextYearLabel |
string | 'Next year' |
A string that provides an accessible label for the button that navigates to the next year in the calendar. This label is intended for screen readers to help users understand the button’s functionality. |
ariaNavPrevMonthLabel |
string | 'Previous month' |
A string that provides an accessible label for the button that navigates to the previous month in the calendar. Screen readers will use this label to explain the purpose of the button. |
ariaNavPrevYearLabel |
string | 'Previous year' |
A string that provides an accessible label for the button that navigates to the previous year in the calendar. This label helps screen reader users understand the button’s function. |
calendarDate |
date, number, string, null | null |
Default date of the component. |
calendars |
number | 2 |
The number of calendars that render on desktop devices. |
disabledDates |
array, function, null | null |
Specify the list of dates that cannot be selected. |
endDate |
date, number, string, null | null |
Initial selected to date (range). |
firstDayOfWeek |
number | 1 |
Sets the day of start week.
|
locale |
string | 'default' |
Sets the default locale for components. If not set, it is inherited from the navigator.language. |
maxDate |
date, number, string, null | null |
Max selectable date. |
minDate |
date, number, string, null | null |
Min selectable date. |
range |
boolean | false |
Allow range selection |
selectAdjacementDays |
boolean | false |
Set whether days in adjacent months shown before or after the current month are selectable. This only applies if the showAdjacementDays option is set to true. |
selectionType |
'day' , 'week' , 'month' , 'year' |
day |
Specify the type of date selection as day, week, month, or year. |
showAdjacementDays |
boolean | true |
Set whether to display dates in adjacent months (non-selectable) at the start and end of the current month. |
showWeekNumber |
boolean | false |
Set whether to display week numbers in the calendar. |
startDate |
date, number, string, null | null |
Initial selected date. |
weekdayFormat |
number, ’long’, ’narrow’, ‘short’ | 2 |
Set length or format of day name. |
weekNumbersLabel |
string | null |
Label displayed over week numbers in the calendar. |
Methods
Method | Description |
---|---|
update |
Updates the configuration of the calendar. |
dispose |
Destroys a component. (Removes stored data on the DOM element) |
getInstance |
Static method which allows you to get the calendar instance associated to a DOM element, you can use it like this: coreui.Calendar.getInstance(element) |
getOrCreateInstance |
Static method which returns a calendar instance associated to a DOM element or create a new one in case it wasn’t initialized. You can use it like this: coreui.Calendar.getOrCreateInstance(element) |
Events
Method | Description |
---|---|
calendarDateChange.coreui.calendar |
Callback fired when the calendar date changed. |
calendarMouseleave.coreui.calendar |
Callback fired when the cursor leave the calendar. |
cellHover.coreui.calendar |
Callback fired when the user hovers over the calendar cell. |
endDateChange.coreui.calendar |
Callback fired when the end date changed. |
selectEndChange.coreui.calendar |
Callback fired when the selection type changed. |
startDateChange.coreui.calendar |
Callback fired when the start date changed. |
const myCalendar = document.getElementById('myCalendar')
myCalendar.addEventListener('endDateChange.coreui.calendar', date => {
// do something...
})
Customizing
CSS variables
Calendar use local CSS variables on .calendar
for enhanced real-time customization. Values for the CSS variables are set via Sass, so Sass customization is still supported, too.
--cui-calendar-table-margin: #{$calendar-table-margin};
--cui-calendar-table-cell-size: #{$calendar-table-cell-size};
--cui-calendar-nav-padding: #{$calendar-nav-padding};
--cui-calendar-nav-border-color: #{$calendar-nav-border-color};
--cui-calendar-nav-border: #{$calendar-nav-border-width} solid var(--cui-calendar-nav-border-color);
--cui-calendar-nav-date-color: #{$calendar-nav-date-color};
--cui-calendar-nav-date-hover-color: #{$calendar-nav-date-hover-color};
--cui-calendar-nav-icon-width: #{$calendar-nav-icon-width};
--cui-calendar-nav-icon-height: #{$calendar-nav-icon-height};
--cui-calendar-nav-icon-double-next: #{escape-svg($calendar-nav-icon-double-next)};
--cui-calendar-nav-icon-double-prev: #{escape-svg($calendar-nav-icon-double-prev)};
--cui-calendar-nav-icon-next: #{escape-svg($calendar-nav-icon-next)};
--cui-calendar-nav-icon-prev: #{escape-svg($calendar-nav-icon-prev)};
--cui-calendar-nav-icon-color: #{$calendar-nav-icon-color};
--cui-calendar-nav-icon-hover-color: #{$calendar-nav-icon-hover-color};
--cui-calendar-cell-header-inner-color: #{$calendar-cell-header-inner-color};
--cui-calendar-cell-week-number-color: #{$calendar-cell-week-number-color};
--cui-calendar-cell-hover-color: #{$calendar-cell-hover-color};
--cui-calendar-cell-hover-bg: #{$calendar-cell-hover-bg};
--cui-calendar-cell-focus-box-shadow: #{$calendar-cell-focus-box-shadow};
--cui-calendar-cell-disabled-color: #{$calendar-cell-disabled-color};
--cui-calendar-cell-selected-color: #{$calendar-cell-selected-color};
--cui-calendar-cell-selected-bg: #{$calendar-cell-selected-bg};
--cui-calendar-cell-range-bg: #{$calendar-cell-range-bg};
--cui-calendar-cell-range-hover-bg: #{$calendar-cell-range-hover-bg};
--cui-calendar-cell-range-hover-border-color: #{$calendar-cell-range-hover-border-color};
--cui-calendar-cell-today-color: #{$calendar-cell-today-color};
--cui-calendar-cell-week-number-color: #{$calendar-cell-week-number-color};
SASS variables
$calendar-table-margin: .5rem;
$calendar-table-cell-size: 2.75rem;
$calendar-nav-padding: .5rem;
$calendar-nav-border-width: 1px;
$calendar-nav-border-color: var(--#{$prefix}border-color);
$calendar-nav-date-color: var(--#{$prefix}body-color);
$calendar-nav-date-hover-color: var(--#{$prefix}primary);
$calendar-nav-icon-width: 1rem;
$calendar-nav-icon-height: 1rem;
$calendar-nav-icon-color: var(--#{$prefix}tertiary-color);
$calendar-nav-icon-hover-color: var(--#{$prefix}body-color);
$calendar-nav-icon-double-next: url("data:image/svg+xml,<svg xmlns='http://www.w3.org/2000/svg' viewBox='0 0 512 512' role='img'><polygon fill='#000' points='95.314 447.313 72.686 424.687 245.373 252 72.686 79.313 95.314 56.687 290.627 252 95.314 447.313'></polygon><polygon fill='#000' points='255.314 447.313 232.686 424.687 405.373 252 232.686 79.313 255.314 56.687 450.627 252 255.314 447.313'></polygon></svg>");
$calendar-nav-icon-double-prev: url("data:image/svg+xml,<svg xmlns='http://www.w3.org/2000/svg' viewBox='0 0 512 512' role='img'><polygon fill='#000' points='416.686 447.313 221.373 252 416.686 56.687 439.314 79.313 266.627 252 439.314 424.687 416.686 447.313'></polygon><polygon fill='#000' points='256.686 447.313 61.373 252 256.686 56.687 279.314 79.313 106.627 252 279.314 424.687 256.686 447.313'></polygon></svg>");
$calendar-nav-icon-next: url("data:image/svg+xml,<svg xmlns='http://www.w3.org/2000/svg' viewBox='0 0 512 512' role='img'><polygon fill='#000' points='179.313 451.313 156.687 428.687 329.372 256 156.687 83.313 179.313 60.687 374.627 256 179.313 451.313'></polygon></svg>");
$calendar-nav-icon-prev: url("data:image/svg+xml,<svg xmlns='http://www.w3.org/2000/svg' viewBox='0 0 512 512' role='img'><polygon fill='#000' points='324.687 451.313 129.373 256 324.687 60.687 347.313 83.313 174.628 256 347.313 428.687 324.687 451.313'></polygon></svg>");
$calendar-cell-header-inner-color: var(--#{$prefix}secondary-color);
$calendar-cell-week-number-color: var(--#{$prefix}secondary-color);
$calendar-cell-hover-color: var(--#{$prefix}body-color);
$calendar-cell-hover-bg: var(--#{$prefix}tertiary-bg);
$calendar-cell-disabled-color: var(--#{$prefix}tertiary-color);
$calendar-cell-focus-box-shadow: $focus-ring-box-shadow;
$calendar-cell-selected-color: $white;
$calendar-cell-selected-bg: var(--#{$prefix}primary);
$calendar-cell-range-bg: rgba(var(--#{$prefix}primary-rgb), .125);
$calendar-cell-range-hover-bg: rgba(var(--#{$prefix}primary-rgb), .25);
$calendar-cell-range-hover-border-color: var(--#{$prefix}primary);
$calendar-cell-today-color: var(--#{$prefix}danger);
$calendar-cell-week-number-color: var(--#{$prefix}tertiary-color);