React Smart Table Component (DataTable)
Smart Table (DataTable)
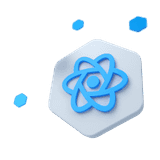
A React Smart Table provides a full set of features for displaying and manipulating tabular data. It allows you to easily create dynamic and interactive tables with features such as sorting, filtering, pagination, and searching. React Smart Table Component (DataTables) makes it easy to work with large datasets, and it is widely used in a variety of applications, including web-based applications, e-commerce sites, and more.
Other Frameworks
CoreUI components are available as native Angular, Bootstrap (Vanilla JS), and Vue components. To learn more please visit the following pages.
Features#
- Filter items by one or all columns
- Sort items by column
- Integrated with CPagination component by default
- Customize style of specific rows, columns and cells
- Customize display of columns
- Load with initial filters and sorter state
- Loading state visualization
- Default header labels generation based on column names
Usage#
Here is an example of how to create a basic DataTable:
Name | Registered | Role | Status | |||
---|---|---|---|---|---|---|
No items found | ||||||
Name | Registered | Role | Status |
Column names#
By default, React Table component will generate the header labels for each column based on the column's data source.
No items found |
Column groups#
The React Smart Table component allows users to group related columns under a common header. This can be useful when displaying data that has multiple categories or when comparing different sets of data. When the Smart Table is rendered, the header group will be displayed as a single header cell that spans the width of the columns included in the group. The group header cells feature can help to make the Smart Table component more organized and easier to read by grouping related data together and making it more visually distinct from other columns.
Group 1 | |||
---|---|---|---|
Subgroup 1 | Subgroup 2 | ||
Subgroup 1A | Subgroup 1B | Subgroup 2A | |
Name | Registered | Role | Status |
No items found | |||
Name | Registered | Role | Status |
Table with headers spanning multiple rows or columns#
In the example below, the table consists of two individual columns and one column group spanning three columns. It has six rows. Two headers that span multiple rows.
Poster availability | ||||
---|---|---|---|---|
Poster name | Color | Sizes available | ||
No items found |
Example source: https://www.w3.org/WAI/tutorials/tables/irregular/
Column sorter#
The column sorter feature enables sorting React.js table data by column values. You can enable sorting by adding columnSorter
to <CSmartTable>
. If you want to disable sorting for a specific column, add sorter: false
to the desired column.
Name | Registered | Role | |
---|---|---|---|
No items found |
Sort multiple columns#
Enables sorting by multiple columns simultaneously by adding columnSorter={{ multiple: true }}
The sorting order will be maintained across multiple columns based on the order of user interaction. This means you can sort by multiple columns, and each column will keep its order.
<CSmartTable columns={[...]} columnSorter={{ multiple: true }} items={[...]}/>
Resetable sorter state#
When columnSorter={{ resetable: true }}
is set, clicking the sorter toggles through three states: ascending, descending, and unsorted. After the third click, it resets the sorting, returning the table to its initial state.
<CSmartTable columns={[...]} columnSorter={{ resetable: true }} items={[...]}/>
Custom sorter#
By default, sorting is done automatically by the component and works as expected when the values in the column are of a single data type: either string (case-insensitive) or number.
In case you want to sort non-string and non-number data, define a custom sorting function for the column. You can use this option to control how the values are compared and sorted. The function receives two arguments (a
, b
) corresponding to two row values and must return:
- a negative value if
a
should appear beforeb
, - zero if
a
andb
are equal, - a positive value if
a
should appear afterb
.
<CSmartTable columns={[ ... { key: 'registered', sorter: (date1, date2) => { const a = new Date(date1.registered) const b = new Date(date2.registered) return a > b ? 1 : b > a ? -1 : 0 } }, ... ]} items={[...]}/>
Column filter#
The column filter feature allows filtering React.js table data based on column values. To enable filtering, add columnFilter
to the <CSmartTable>
component. If you want to disable filtering for a specific column, use filter: false
in the column's configuration.
Name | Registered | Role | |
---|---|---|---|
No items found |
Custom filters#
CoreUI React Smart Table (React DataTables) provides the ability to use custom filtering functions. Custom filters offer advanced filtering capabilities. You can define and integrate custom filtering logic by adding a filter
function to the desired column, enabling more complex data filtering strategies.
Date Range Picker#
Here is an example of how you might use the <CDateRangePicker />
component to apply custom filters to a React DataTable component:
Name | Registered |
---|---|
No items found |
Multi Select#
Here is an example of how you might use the <CMultiSelect />
component to apply custom filters to a React DataTable:
Name | Role |
---|---|
No items found |
Data sources#
You can use Fetch API to load data from different sources and then pass them to <CSmartTable>
External Data (10.000+ records)#
One of the key features of React Smart Table (React DataTables) is the ability to load data from an external source, such as an API or a server-side script. This can be useful if you have a large amount of data that you don't want to load all at once. To load external data into a React Smart Table (React DataTables), you can use the Fetch API to the data source.
Here is an example of how you might use React Smart Table with external data:
First Name | Last Name | Email | Country | IP |
---|---|---|---|---|
No items found | ||||
First Name | Last Name | Email | Country | IP |
JSON (10.000+ records)#
The Fetch API can be used to load JSON data by making a GET request to the endpoint where the data is located.
In this example, the fetch()
method is used to make a GET request to the endpoint https://apitest.coreui.io/fake_data/users.json
, which returns a response.
The response.json()
method is then used to parse the response as a JSON object.
The resulting JSON object is stored in the users
and loaded by <CSmartTable />
.
First Name | Last Name | Email | Country | IP |
---|---|---|---|---|
No items found | ||||
First Name | Last Name | Email | Country | IP |
API#
Check out the documentation below for a comprehensive guide to all the props you can use with the components mentioned here.