React Calendar Component
Calendar
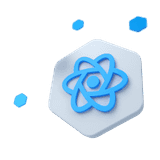
The React Calendar Component is a versatile, customizable tool for creating responsive calendars in React, supporting day, month, and year selection, and global locales.
Other Frameworks
CoreUI components are available as native Angular, Bootstrap (Vanilla JS), and Vue components. To learn more please visit the following pages.
Example#
Explore the React Calendar component's basic usage through sample code snippets demonstrating its core functionality.
Days#
Select specific days using the React Calendar component. The example below shows basic usage.
Weeks#
Set the selectionType
to week
to enable selection of entire week. You can also add showWeekNumber
to show week numbers.
CalendarWeeksExample
Months#
Set the selectionType
property to month
to enable selection of entire months.
Years#
Set the selectionType
property to year
to enable years range selection.
Multiple calendar panels#
Display multiple calendar panels side by side by setting the calendars
property. This can be useful for selecting ranges or comparing dates across different months.
Range selection#
Enable range selection to allow users to pick a start and end date. This example shows how to configure the React Calendar component to handle date ranges.
Disabled dates#
The React Calendar component includes functionality to disable specific dates, such as weekends or holidays, using the disabledDates
prop. It accepts:
- A single
Date
or an array ofDate
objects. - A function or an array of functions that take a
Date
object as an argument and return a boolean indicating whether the date should be disabled. - A mixed array of
Date
objects and functions.
To disable certain dates, you can provide them in an array. For date ranges, use nested arrays, where each inner array indicates a start date and an end date for that range:
Disabling weekends#
You can disable weekends by passing a function to the disabledDates
prop. Here's how to do it:
In the example above:
disableWeekends
is a function that checks if a date falls on a Saturday (6
) or a Sunday (0
).- The
disabledDates
prop is set to thedisableWeekends
function, which ensures that all weekends are disabled in the calendar.
This prop takes an array and applies custom logic to determine which dates should be disabled.
Combining functions and specific dates#
You can also combine specific dates and functions in the disabledDates
array. For instance:
In this example:
disableWeekends
disables weekends as before.specificDates
is an array of specific dates to disable.- The
disabledDates
prop combines both, allowing you to disable weekends and specific dates simultaneously.
Locale#
The CoreUI React Calendar allows users to display dates in non-English locales, making it suitable for international applications.
Auto#
By default, the Calendar component uses the browser's default locale. However, you can easily configure it to use a different locale supported by the JavaScript Internationalization API. This feature helps create inclusive and accessible applications for a diverse audience.
Chinese#
Here is an example of the React Calendar component with Chinese locale settings.
Japanese#
Below is an example of the Calendar component with Japanese locale settings.
Korean#
Here is an example of the Calendar component with Korean locale settings.
Right to left support#
RTL support is built-in and can be explicitly controlled through the $enable-rtl
variables in scss.
Hebrew#
Example of the Calendar component with RTL support, using the Hebrew locale.
Persian#
Example of the React Calendar component with Persian locale settings.
API#
Check out the documentation below for a comprehensive guide to all the props you can use with the components mentioned here.