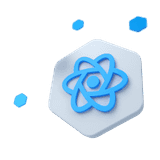
React Date Picker Component
Date Picker
The React Date Picker Component is an essential tool for any React-based application that requires date selection functionality.
Other frameworks
CoreUI components are available as native Angular, Bootstrap (Vanilla JS), and Vue components. To learn more please visit the following pages.
About#
This component, provided by CoreUI for React.js, offers a user-friendly interface and various customization options to ensure an excellent user experience.
With its responsive design and support for all modern browsers, the React Date Picker Component can be easily integrated into any application. The component comes with an array of styling options, including color, size, and shape, that can be easily adjusted to fit the application's design.
The React Date Picker Component is also fully customizable, allowing developers to tailor the component to their specific needs. With its set of APIs, developers can control the default value, visibility, and date range of the component.
One of the standout features of the React Date Picker Component is its accessibility. CoreUI has ensured that the component is fully compliant with the Web Content Accessibility Guidelines (WCAG), making it accessible to all users. The component supports keyboard navigation and screen readers, ensuring that users with disabilities can also benefit from its functionality.
Example#
Below is an example of a basic React DatePicker.
Days#
Basic examples demonstrating how to pick dates using the React Date Picker Component.
<div className="row"> <div className="col-sm-6 col-lg-5 mb-3 mb-sm-0"> <CDatePicker label="Date Picker" locale="en-US" /> </div> <div className="col-sm-6 col-lg-5"> <CDatePicker date="2022/2/16" label="Date Picker" locale="en-US" /> </div></div>
With timepicker#
In addition to supporting date selection, our React DatePicker component also includes a TimePicker feature that allows users to select a specific time of day. React TimePicker can be enabled by passing a timepicker
prop to the <CDatePicker/>
component
<div className="row"> <div className="col-sm-6 col-lg-5 mb-3 mb-sm-0"> <CDatePicker locale="en-US" timepicker /> </div> <div className="col-sm-6 col-lg-5"> <CDatePicker date="2023/03/15 02:22:13 PM" locale="en-US" timepicker /> </div> </div>
With footer#
Here is an example with the additional footer. The footer can be useful for displaying additional information or actions related to the selected date, such as "Today" or "Clear" buttons. The footer component is fully customizable and can be styled to match the rest of the application.
<div className="row"> <div className="col-sm-6 col-lg-5 mb-3 mb-sm-0"> <CDatePicker footer locale="en-US" /> </div> <div className="col-sm-6 col-lg-5"> <CDatePicker date="2022/2/16" footer locale="en-US" /> </div></div>
Weeks#
Illustration of week selection using the React Date Picker, including week number display.
<div className="row"> <div className="col-sm-6 col-lg-5 mb-3 mb-sm-0"> <CDatePicker label="Week Picker" locale="en-US" selectionType="week" showWeekNumber /> </div> <div className="col-sm-6 col-lg-5"> <CDatePicker date="2025W07" label="Week Picker" locale="en-US" selectionType="week" showWeekNumber /> </div></div>
Months#
Selecting whole months with the React Date Picker Component.
<div className="row"> <div className="col-sm-6 col-lg-5 mb-3 mb-sm-0"> <CDatePicker label="Month Picker" locale="en-US" selectionType="month" /> </div> <div className="col-sm-6 col-lg-5"> <CDatePicker label="Month Picker" locale="en-US" selectionType="month" date="2022-08" /> </div></div>
Years#
Picking years using the React Date Picker.
<div className="row"> <div className="col-sm-6 col-lg-5 mb-3 mb-sm-0"> <CDatePicker label="Year Picker" locale="en-US" selectionType="year" /> </div> <div className="col-sm-6 col-lg-5"> <CDatePicker label="Year Picker" locale="en-US" selectionType="year" date="2022" /> </div></div>
Sizing#
Set heights using size
property like size="lg"
and size="sm"
.
<CDatePicker locale="en-US" size="lg" /><CDatePicker locale="en-US" size="sm" />
Disabled#
Add the disabled
boolean attribute on the React Date Picker to give it a grayed out appearance and remove pointer events.
<CDatePicker disabled locale="en-US" />
Readonly#
Add the inputReadOnly
boolean attribute to prevent modification of the input's value.
<CDatePicker inputReadOnly locale="en-US" />
Disabled dates#
CoreUI React DatePicker component includes a feature that allows you to disable certain dates, such as weekends or holidays. This can be accomplished by passing an array to disabledDate
prop to the component, which determines which dates should be disabled based on custom logic.
const calendarDate = new Date(2022, 2, 1)const disabledDates = [ [new Date(2022, 2, 4), new Date(2022, 2, 7)], // range of dates that cannot be selected new Date(2022, 2, 16), // single date that cannot be selected new Date(2022, 3, 16), // single date that cannot be selected [new Date(2022, 4, 2), new Date(2022, 4, 8)], // range of dates that cannot be selected]const maxDate = new Date(2022, 5, 0)const minDate = new Date(2022, 1, 1)return ( <div className="row"> <div className="col-lg-4"> <CDatePicker calendarDate={calendarDate} disabledDates={disabledDates} locale="en-US" maxDate={maxDate} minDate={minDate} /> </div> </div>)
Disabling weekends#
You can disable weekends by passing a function to the disabledDates
prop. Here's how to do it:
const disableWeekends = (date) => { const day = date.getDay() return day === 0 || day === 6 // Disable Sundays (0) and Saturdays (6)}
return ( <div className="row"> <div className="col-lg-5"> <CDatePicker disabledDates={disableWeekends} locale="en-US" /> </div> </div>)
Non-english locale#
CoreUI React Date Picker allows users to display dates and times in a non-English locale. This is useful for applications that have international users or need to support multiple languages.
Auto#
By default, the DatePicker component uses the default browser locale, but it can be easily configured to use a different locale supported by the JavaScript Internationalization API. To set the locale, you can simply pass the desired language code as a prop to the DatePicker component. This feature enables to create more inclusive and accessible applications that cater to a diverse audience.
<CDatePicker />
Chinese#
Below is an example of a basic React Date Picker with Chinese locales.
<CDatePicker placeholder="入住日期" locale="zh-CN" />
Japanese#
Below is an example of a basic React Date Picker with Japanese locales.
<CDatePicker placeholder="日付を選択" locale="ja" />
Korean#
Below is an example of a basic React Date Picker with Korean locales.
<CDatePicker placeholder="날짜 선택" locale="ko" />
Right to left support#
RTL support is built-in and can be explicitly controlled through the $enable-rtl
variables in scss.
Hebrew#
<CDatePicker placeholder="בחר תאריך" locale="he-IL" />
Persian#
<CDatePicker placeholder="تاریخ شروع" locale="fa-IR" />
Custom formats#
Heads up! As of v5.0.0, the format
property is removed in <CDatePicker>
. Instead, utilize the inputDateFormat
to format dates into custom strings and inputDateParse
to parse custom strings into Date objects.
The provided code demonstrates how to use the inputDateFormat
and inputDateParse
properties. In this example, the format
and parse
functions from date-fns
are employed to tailor the date presentation and interpretation.
import { format, parse } from 'date-fns'import { es } from 'date-fns/locale'
The inputDateFormat
property formats the date into a custom string, while the inputDateParse
property parses a custom string into a Date object. The code showcases the date range in different formats based on locale, such as 'MMMM dd, yyyy' and 'yyyy MMMM dd', and accommodates different locales, like 'en-US' and 'es-ES'.
<div className="row"> <div className="col-lg-4"> <CDatePicker date="2022/08/17" label="Date picker" locale="en-US" inputDateParse={(date) => parse(date, 'MMMM dd, yyyy', new Date())} inputDateFormat={(date) => format(new Date(date), 'MMMM dd, yyyy')} /> </div> <div className="col-lg-4"> <CDatePicker date="2022/08/17" label="Selector de fechas" locale="es-ES" placeholder="Seleccionar fecha" inputDateParse={(date) => parse(date, 'yyyy MMMM dd', new Date(), { locale: es })} inputDateFormat={(date) => format(new Date(date), 'yyyy MMMM dd', { locale: es })} /> </div></div>
With timepicker#
If you need to display custom date and time formats, use the timepicker
property along with inputDateParse
and inputDateFormat
. This allows users to select a date with a specified time format, such as 'MMM dd, yyyy h:mm:ss a'
.
<div className="row"> <div className="col-lg-5"> <CDatePicker date="2022/08/03 02:34:17 AM" label="Date picker" locale="en-US" timepicker inputDateParse={(date) => parse(date, 'MMM dd, yyyy h:mm:ss a', new Date())} inputDateFormat={(date) => format(new Date(date), 'MMM dd, yyyy h:mm:ss a')} /> </div> <div className="col-lg-5"> <CDatePicker date="2022/08/03 02:34:17 AM" label="Selector de fechas" locale="es-ES" timepicker inputDateParse={(date) => parse(date, 'yyyy MMM dd h:mm:ss a', new Date(), { locale: es })} inputDateFormat={(date) => format(new Date(date), 'yyyy MMM dd h:mm:ss a', { locale: es })} /> </div></div>
Weeks#
If you want to show weeks in a custom format, set the selectionType
to 'week'
. This configuration allows you to use inputDateParse
and inputDateFormat
to present and parse week-based date strings, like 'wo yyyy'
. Additionally, the showWeekNumber
property can be used to display week numbers in your preferred format.
<div className="row"> <div className="col-lg-6"> <CDatePicker date="2022W10" label="Date picker" locale="en-US" inputDateParse={(date) => parse(date, 'wo YYYY', new Date(), { useAdditionalWeekYearTokens: true, })} inputDateFormat={(date) => format(new Date(date), 'wo yyyy')} selectionType="week" showWeekNumber /> </div> <div className="col-lg-6"> <CDatePicker date="2022W10" label="Selector de fechas" locale="es-ES" inputDateParse={(date) => parse(date, 'wo YYYY', new Date(), { locale: es, useAdditionalWeekYearTokens: true, })} inputDateFormat={(date) => format(new Date(date), 'wo yyyy', { locale: es })} selectionType="week" showWeekNumber /> </div></div>
Months#
If your application requires month selection in a specific format, set the selectionType
property to 'month'
. Using inputDateFormat
and inputDateParse
, you can customize the display of months, such as using the format 'MMM yyyy'
.
<div className="row"> <div className="col-lg-6"> <CDatePicker date="2022-08" label="Date range" locale="en-US" inputDateParse={(date) => parse(date, 'MMM yyyy', new Date())} inputDateFormat={(date) => format(new Date(date), 'MMM yyyy')} selectionType="month" /> </div> <div className="col-lg-6"> <CDatePicker date="2022-08" label="Selector de fechas" locale="es-ES" inputDateParse={(date) => parse(date, 'MMM yyyy', new Date(), { locale: es })} inputDateFormat={(date) => format(new Date(date), 'MMM yyyy', { locale: es })} selectionType="month" /> </div></div>
Years#
If you need to work with custom year formats, set the selectionType
to 'year'
. This enables you to use inputDateFormat
and inputDateParse
to format years in a manner that suits your application's requirements, like 'yy'
.
<div className="row"> <div className="col-lg-6"> <CDatePicker date="2022" label="Date range" locale="en-US" inputDateParse={(date) => parse(date, 'yy', new Date())} inputDateFormat={(date) => format(new Date(date), 'yy')} selectionType="year" /> </div> <div className="col-lg-6"> <CDatePicker date="2022" label="Selector de fechas" locale="es-ES" inputDateParse={(date) => parse(date, 'yy', new Date(), { locale: es })} inputDateFormat={(date) => format(new Date(date), 'yy', { locale: es })} selectionType="year" /> </div></div>
Customizing#
CSS variables#
React date pickers use local CSS variables on .date-picker
and .calendar
for enhanced real-time customization. Values for the CSS variables are set via Sass, so Sass customization is still supported, too.
--cui-date-picker-zindex: #{$date-picker-zindex};--cui-date-picker-font-family: #{$date-picker-font-family};--cui-date-picker-font-size: #{$date-picker-font-size};--cui-date-picker-font-weight: #{$date-picker-font-weight};--cui-date-picker-line-height: #{$date-picker-line-height};--cui-date-picker-color: #{$date-picker-color};--cui-date-picker-bg: #{$date-picker-bg};--cui-date-picker-box-shadow: #{$date-picker-box-shadow};--cui-date-picker-border-width: #{$date-picker-border-width};--cui-date-picker-border-color: #{$date-picker-border-color};--cui-date-picker-border-radius: #{$date-picker-border-radius};--cui-date-picker-disabled-color: #{$date-picker-disabled-color};--cui-date-picker-disabled-bg: #{$date-picker-disabled-bg};--cui-date-picker-disabled-border-color: #{$date-picker-disabled-border-color};--cui-date-picker-focus-color: #{$date-picker-focus-color};--cui-date-picker-focus-bg: #{$date-picker-focus-bg};--cui-date-picker-focus-border-color: #{$date-picker-focus-border-color};--cui-date-picker-focus-box-shadow: #{$date-picker-focus-box-shadow};--cui-date-picker-placeholder-color: #{$date-picker-placeholder-color};--cui-date-picker-padding-y: #{$date-picker-padding-y};--cui-date-picker-padding-x: #{$date-picker-padding-x};--cui-date-picker-gap: #{$date-picker-gap};--cui-date-picker-indicator-width: #{$date-picker-indicator-width};--cui-date-picker-indicator-icon: #{escape-svg($date-picker-indicator-icon)};--cui-date-picker-indicator-icon-color: #{$date-picker-indicator-icon-color};--cui-date-picker-indicator-icon-size: #{$date-picker-indicator-icon-size};--cui-date-picker-cleaner-width: #{$date-picker-cleaner-width};--cui-date-picker-cleaner-icon: #{escape-svg($date-picker-cleaner-icon)};--cui-date-picker-cleaner-icon-color: #{$date-picker-cleaner-icon-color};--cui-date-picker-cleaner-icon-hover-color: #{$date-picker-cleaner-icon-hover-color};--cui-date-picker-cleaner-icon-size: #{$date-picker-cleaner-icon-size};--cui-date-picker-separator-width: #{$date-picker-separator-width};--cui-date-picker-separator-icon: #{escape-svg($date-picker-separator-icon)};--cui-date-picker-separator-icon-rtl: #{escape-svg($date-picker-separator-icon-rtl)};--cui-date-picker-separator-icon-size: #{$date-picker-separator-icon-size};--cui-date-picker-dropdown-bg: #{$date-picker-dropdown-bg};--cui-date-picker-dropdown-border-width: #{$date-picker-dropdown-border-width};--cui-date-picker-dropdown-border-color: #{$date-picker-dropdown-border-color};--cui-date-picker-dropdown-border-radius: #{$date-picker-dropdown-border-radius};--cui-date-picker-dropdown-box-shadow: #{$date-picker-dropdown-box-shadow};--cui-date-picker-ranges-width: #{$date-picker-ranges-width};--cui-date-picker-ranges-padding: #{$date-picker-ranges-padding};--cui-date-picker-ranges-border-width: #{$date-picker-ranges-border-width};--cui-date-picker-ranges-border-color: #{$date-picker-ranges-border-color};--cui-date-picker-timepicker-width: #{$date-picker-timepicker-width};--cui-date-picker-timepicker-border-color: #{$date-picker-timepicker-border-color};--cui-date-picker-timepicker-border-top: #{$date-picker-timepicker-border-width} solid var(--cui-date-picker-timepicker-border-color);--cui-date-picker-footer-padding: #{$date-picker-footer-padding};--cui-date-picker-footer-border-width: #{$date-picker-footer-border-width};--cui-date-picker-footer-border-color: #{$date-picker-footer-border-color};
--cui-calendar-table-margin: #{$calendar-table-margin};--cui-calendar-table-cell-size: #{$calendar-table-cell-size};--cui-calendar-nav-padding: #{$calendar-nav-padding};--cui-calendar-nav-border-color: #{$calendar-nav-border-color};--cui-calendar-nav-border: #{$calendar-nav-border-width} solid var(--cui-calendar-nav-border-color);--cui-calendar-nav-date-color: #{$calendar-nav-date-color};--cui-calendar-nav-date-hover-color: #{$calendar-nav-date-hover-color};--cui-calendar-nav-icon-width: #{$calendar-nav-icon-width};--cui-calendar-nav-icon-height: #{$calendar-nav-icon-height};--cui-calendar-nav-icon-double-next: #{escape-svg($calendar-nav-icon-double-next)};--cui-calendar-nav-icon-double-prev: #{escape-svg($calendar-nav-icon-double-prev)};--cui-calendar-nav-icon-next: #{escape-svg($calendar-nav-icon-next)};--cui-calendar-nav-icon-prev: #{escape-svg($calendar-nav-icon-prev)};--cui-calendar-nav-icon-color: #{$calendar-nav-icon-color};--cui-calendar-nav-icon-hover-color: #{$calendar-nav-icon-hover-color};--cui-calendar-cell-header-inner-color: #{$calendar-cell-header-inner-color};--cui-calendar-cell-week-number-color: #{$calendar-cell-week-number-color};--cui-calendar-cell-hover-color: #{$calendar-cell-hover-color};--cui-calendar-cell-hover-bg: #{$calendar-cell-hover-bg};--cui-calendar-cell-focus-box-shadow: #{$calendar-cell-focus-box-shadow};--cui-calendar-cell-disabled-color: #{$calendar-cell-disabled-color};--cui-calendar-cell-selected-color: #{$calendar-cell-selected-color};--cui-calendar-cell-selected-bg: #{$calendar-cell-selected-bg};--cui-calendar-cell-range-bg: #{$calendar-cell-range-bg};--cui-calendar-cell-range-hover-bg: #{$calendar-cell-range-hover-bg};--cui-calendar-cell-range-hover-border-color: #{$calendar-cell-range-hover-border-color};--cui-calendar-cell-today-color: #{$calendar-cell-today-color};--cui-calendar-cell-week-number-color: #{$calendar-cell-week-number-color};
How to use CSS variables#
const vars = { '--my-css-var': 10, '--my-another-css-var': "red" }return <CDatePicker style={vars}>...</CDatePicker>
SASS variables#
$date-picker-zindex: 1000 !default;$date-picker-font-family: $input-font-family !default;$date-picker-font-size: $input-font-size !default;$date-picker-font-size-sm: $input-font-size-sm !default;$date-picker-font-size-lg: $input-font-size-lg !default;$date-picker-font-weight: $input-font-weight !default;$date-picker-line-height: $input-line-height !default;$date-picker-color: $input-color !default;$date-picker-bg: $input-bg !default;$date-picker-box-shadow: $box-shadow-inset !default;
$date-picker-border-width: $input-border-width !default;$date-picker-border-color: $input-border-color !default;$date-picker-border-radius: $border-radius !default;$date-picker-border-radius-sm: $border-radius-sm !default;$date-picker-border-radius-lg: $border-radius-lg !default;
$date-picker-invalid-border-color: var(--cui-form-invalid-border-color) !default;$date-picker-valid-border-color: var(--cui-form-valid-border-color) !default;
$date-picker-disabled-color: $input-disabled-color !default;$date-picker-disabled-bg: $input-disabled-bg !default;$date-picker-disabled-border-color: $input-disabled-border-color !default;
$date-picker-focus-color: $input-focus-color !default;$date-picker-focus-bg: $input-focus-bg !default;$date-picker-focus-border-color: $input-focus-border-color !default;$date-picker-focus-box-shadow: $input-btn-focus-box-shadow !default;
$date-picker-placeholder-color: var(--cui-secondary-color) !default;
$date-picker-padding-y: $input-padding-y !default;$date-picker-padding-x: $input-padding-x !default;$date-picker-gap: $input-padding-x !default;
$date-picker-padding-y-sm: $input-padding-y-sm !default;$date-picker-padding-x-sm: $input-padding-x-sm !default;$date-picker-gap-sm: $input-padding-x-sm !default;
$date-picker-padding-y-lg: $input-padding-y-lg !default;$date-picker-padding-x-lg: $input-padding-x-lg !default;$date-picker-gap-lg: $input-padding-x-lg !default;
$date-picker-cleaner-width: 1.25rem !default;$date-picker-cleaner-icon-size: 1rem !default;$date-picker-cleaner-icon-color: var(--cui-tertiary-color) !default;$date-picker-cleaner-icon: url("data:image/svg+xml,<svg xmlns='http://www.w3.org/2000/svg' viewBox='0 0 512 512' role='img'><polygon fill='#000' points='306.912 214.461 256 265.373 205.088 214.461 182.461 237.088 233.373 288 182.461 338.912 205.088 361.539 256 310.627 306.912 361.539 329.539 338.912 278.627 288 329.539 237.088 306.912 214.461'></polygon><path fill='#000' d='M472,96H384V40H352V96H160V40H128V96H40a24.028,24.028,0,0,0-24,24V456a24.028,24.028,0,0,0,24,24H472a24.028,24.028,0,0,0,24-24V120A24.028,24.028,0,0,0,472,96Zm-8,352H48V128h80v40h32V128H352v40h32V128h80Z'></path></svg>") !default;$date-picker-cleaner-icon-hover-color: var(--cui-body-color) !default;
$date-picker-cleaner-width-sm: 1rem !default;$date-picker-cleaner-width-lg: 1.5rem !default;$date-picker-cleaner-icon-size-sm: .875rem !default;$date-picker-cleaner-icon-size-lg: 1.25rem !default;
$date-picker-indicator-width: 1.25rem !default;$date-picker-indicator-icon-size: 1rem !default;$date-picker-indicator-icon-color: var(--cui-tertiary-color) !default;$date-picker-indicator-icon: url("data:image/svg+xml,<svg xmlns='http://www.w3.org/2000/svg' viewBox='0 0 512 512' role='img'><path fill='#000' d='M472,96H384V40H352V96H160V40H128V96H40a24.028,24.028,0,0,0-24,24V456a24.028,24.028,0,0,0,24,24H472a24.028,24.028,0,0,0,24-24V120A24.028,24.028,0,0,0,472,96Zm-8,352H48V128h80v40h32V128H352v40h32V128h80Z'></path><rect width='32' height='32' x='112' y='224' fill='#000'></rect><rect width='32' height='32' x='200' y='224' fill='#000'></rect><rect width='32' height='32' x='280' y='224' fill='#000'></rect><rect width='32' height='32' x='368' y='224' fill='#000'></rect><rect width='32' height='32' x='112' y='296' fill='#000'></rect><rect width='32' height='32' x='200' y='296' fill='#000'></rect><rect width='32' height='32' x='280' y='296' fill='#000'></rect><rect width='32' height='32' x='368' y='296' fill='#000'></rect><rect width='32' height='32' x='112' y='368' fill='#000'></rect><rect width='32' height='32' x='200' y='368' fill='#000'></rect><rect width='32' height='32' x='280' y='368' fill='#000'></rect><rect width='32' height='32' x='368' y='368' fill='#000'></rect></svg>") !default;$date-picker-indicator-invalid-icon: $date-picker-indicator-icon !default;$date-picker-indicator-invalid-icon-color: var(--cui-form-invalid-color) !default;$date-picker-indicator-valid-icon: $date-picker-indicator-icon !default;$date-picker-indicator-valid-icon-color: var(--cui-form-valid-color) !default;
$date-picker-indicator-width-sm: 1rem !default;$date-picker-indicator-width-lg: 1.5rem !default;$date-picker-indicator-icon-size-sm: .875rem !default;$date-picker-indicator-icon-size-lg: 1.25rem !default;
$date-picker-separator-width: 1.25rem !default;$date-picker-separator-icon-size: 1rem !default;$date-picker-separator-icon-color: var(--cui-tertiary-color) !default;$date-picker-separator-icon: url("data:image/svg+xml,<svg xmlns='http://www.w3.org/2000/svg' viewBox='0 0 512 512' role='img'><polygon fill='#000' points='359.873 121.377 337.246 144.004 433.243 240.001 16 240.001 16 240.002 16 272.001 16 272.002 433.24 272.002 337.246 367.996 359.873 390.623 494.498 256 359.873 121.377'></polygon></svg>") !default;$date-picker-separator-icon-rtl: url("data:image/svg+xml,<svg xmlns='http://www.w3.org/2000/svg' viewBox='0 0 512 512' role='img'><polygon fill='#000' points='497.333 239.999 80.092 239.999 176.087 144.004 153.46 121.377 18.837 256 153.46 390.623 176.087 367.996 80.09 271.999 497.333 271.999 497.333 239.999'></polygon></svg>") !default;
$date-picker-separator-width-sm: 1rem !default;$date-picker-separator-width-lg: 1.55rem !default;$date-picker-separator-icon-size-sm: .875rem !default;$date-picker-separator-icon-size-lg: 1.25rem !default;
$date-picker-dropdown-bg: var(--cui-body-bg) !default;$date-picker-dropdown-border-color: var(--cui-border-color) !default;$date-picker-dropdown-border-width: var(--cui-border-width) !default;$date-picker-dropdown-border-radius: var(--cui-border-radius) !default;$date-picker-dropdown-box-shadow: var(--cui-box-shadow) !default;
$date-picker-ranges-width: 10rem !default;$date-picker-ranges-padding: $spacer * .5 !default;$date-picker-ranges-border-width: 1px !default;$date-picker-ranges-border-color: var(--cui-border-color) !default;
$date-picker-timepicker-width: (7 * $calendar-table-cell-size) + (2 * $calendar-table-margin) !default;$date-picker-timepicker-border-width: 1px !default;$date-picker-timepicker-border-color: var(--cui-border-color) !default;
$date-picker-footer-padding: .5rem !default;$date-picker-footer-border-width: 1px !default;$date-picker-footer-border-color: var(--cui-border-color) !default;
$calendar-table-margin: .5rem !default;$calendar-table-cell-size: 2.75rem !default;
$calendar-nav-padding: .5rem !default;$calendar-nav-border-width: 1px !default;$calendar-nav-border-color: var(--cui-border-color) !default;$calendar-nav-date-color: var(--cui-body-color) !default;$calendar-nav-date-hover-color: var(--cui-primary) !default;$calendar-nav-icon-width: 1rem !default;$calendar-nav-icon-height: 1rem !default;$calendar-nav-icon-color: var(--cui-tertiary-color) !default;$calendar-nav-icon-hover-color: var(--cui-body-color) !default;
$calendar-nav-icon-double-next: url("data:image/svg+xml,<svg xmlns='http://www.w3.org/2000/svg' viewBox='0 0 512 512' role='img'><polygon fill='#000' points='95.314 447.313 72.686 424.687 245.373 252 72.686 79.313 95.314 56.687 290.627 252 95.314 447.313'></polygon><polygon fill='#000' points='255.314 447.313 232.686 424.687 405.373 252 232.686 79.313 255.314 56.687 450.627 252 255.314 447.313'></polygon></svg>") !default;$calendar-nav-icon-double-prev: url("data:image/svg+xml,<svg xmlns='http://www.w3.org/2000/svg' viewBox='0 0 512 512' role='img'><polygon fill='#000' points='416.686 447.313 221.373 252 416.686 56.687 439.314 79.313 266.627 252 439.314 424.687 416.686 447.313'></polygon><polygon fill='#000' points='256.686 447.313 61.373 252 256.686 56.687 279.314 79.313 106.627 252 279.314 424.687 256.686 447.313'></polygon></svg>") !default;$calendar-nav-icon-next: url("data:image/svg+xml,<svg xmlns='http://www.w3.org/2000/svg' viewBox='0 0 512 512' role='img'><polygon fill='#000' points='179.313 451.313 156.687 428.687 329.372 256 156.687 83.313 179.313 60.687 374.627 256 179.313 451.313'></polygon></svg>") !default;$calendar-nav-icon-prev: url("data:image/svg+xml,<svg xmlns='http://www.w3.org/2000/svg' viewBox='0 0 512 512' role='img'><polygon fill='#000' points='324.687 451.313 129.373 256 324.687 60.687 347.313 83.313 174.628 256 347.313 428.687 324.687 451.313'></polygon></svg>") !default;
$calendar-cell-header-inner-color: var(--cui-secondary-color) !default;$calendar-cell-week-number-color: var(--cui-secondary-color) !default;
$calendar-cell-hover-color: var(--cui-body-color) !default;$calendar-cell-hover-bg: var(--cui-tertiary-bg) !default;$calendar-cell-disabled-color: var(--cui-tertiary-color) !default;
$calendar-cell-focus-box-shadow: $focus-ring-box-shadow !default;
$calendar-cell-selected-color: $white !default;$calendar-cell-selected-bg: var(--cui-primary) !default;
$calendar-cell-range-bg: rgba(var(--cui-primary-rgb), .125) !default;$calendar-cell-range-hover-bg: rgba(var(--cui-primary-rgb), .25) !default;$calendar-cell-range-hover-border-color: var(--cui-primary) !default;
$calendar-cell-today-color: var(--cui-danger) !default;
$calendar-cell-week-number-color: var(--cui-tertiary-color) !default;
API#
CDatePicker#
import { CDatePicker } from '@coreui/react-pro'// orimport CDatePicker from '@coreui/react-pro/src/components/date-picker/CDatePicker'
Property | Description | Type | Default |
---|---|---|---|
ariaNavNextMonthLabel 5.5.0+ | A string that provides an accessible label for the button that navigates to the next month in the calendar. This label is read by screen readers to describe the action associated with the button. | string | - |
ariaNavNextYearLabel 5.5.0+ | A string that provides an accessible label for the button that navigates to the next year in the calendar. This label is intended for screen readers to help users understand the button's functionality. | string | - |
ariaNavPrevMonthLabel 5.5.0+ | A string that provides an accessible label for the button that navigates to the previous month in the calendar. Screen readers will use this label to explain the purpose of the button. | string | - |
ariaNavPrevYearLabel 5.5.0+ | A string that provides an accessible label for the button that navigates to the previous year in the calendar. This label helps screen reader users understand the button's function. | string | - |
calendarDate | Default date of the component | string | Date | - |
cancelButton | Toggle visibility or set the content of cancel button. | ReactNode | 'Cancel' |
cancelButtonColor | Sets the color context of the cancel button to one of CoreUI’s themed colors. | 'primary' | 'secondary' | 'success' | 'danger' | 'warning' | 'info' | 'dark' | 'light' | string | 'primary' |
cancelButtonSize | Size the cancel button small or large. | 'sm' | 'lg' | 'sm' |
cancelButtonVariant | Set the cancel button variant to an outlined button or a ghost button. | 'outline' | 'ghost' | 'ghost' |
className | A string of all className you want applied to the component. | string | - |
cleaner | Toggle visibility or set the content of the cleaner button. | boolean | - |
closeOnSelect 4.8.0+ | If true the dropdown will be immediately closed after submitting the full date. | boolean | - |
confirmButton | Toggle visibility or set the content of confirm button. | ReactNode | 'OK' |
confirmButtonColor | Sets the color context of the confirm button to one of CoreUI’s themed colors. | 'primary' | 'secondary' | 'success' | 'danger' | 'warning' | 'info' | 'dark' | 'light' | string | 'primary' |
confirmButtonSize | Size the confirm button small or large. | 'sm' | 'lg' | 'sm' |
confirmButtonVariant | Set the confirm button variant to an outlined button or a ghost button. | 'outline' | 'ghost' | - |
container | Set container type for the component. | 'dropdown' | 'inline' | - |
date | Initial selected date. | string | Date | - |
dayFormat 4.3.0+ | Set the format of day name. | 'numeric' | '2-digit' | ((date: Date) => string | number) | 'numeric' |
disabled | Toggle the disabled state for the component. | boolean | - |
disabledDates | Specify the list of dates that cannot be selected. | DisabledDate | DisabledDate[] | - |
dropdownClassNames | A string of all className you want applied to the dropdown menu. | string | - |
feedback 4.2.0+ | Provide valuable, actionable feedback. | ReactNode | - |
feedbackInvalid 4.2.0+ | Provide valuable, actionable feedback. | ReactNode | - |
feedbackValid 4.2.0+ | Provide valuable, actionable invalid feedback when using standard HTML form validation which applied two CSS pseudo-classes, :invalid and :valid . | ReactNode | - |
firstDayOfWeek | Sets the day of start week. - 0 - Sunday, - 1 - Monday, - 2 - Tuesday, - 3 - Wednesday, - 4 - Thursday, - 5 - Friday, - 6 - Saturday, | number | 1 |
floatingClassName 4.5.0+ | A string of all className you want applied to the floating label wrapper. | string | - |
footer | Toggle visibility of footer element. | boolean | - |
footerContent | Add custom elements to the footer. | ReactNode | - |
id | The id global attribute defines an identifier (ID) that must be unique in the whole document. [Deprecated since v5.3.0] The name attributes for input element is generated based on this property until you define name prop ex.: - {id}-date | string | - |
indicator | Toggle visibility or set the content of the input indicator. | ReactNode | - |
inputDateFormat 5.0.0+ | Custom function to format the selected date into a string according to a custom format. | (date: Date) => string | - |
inputDateParse 5.0.0+ | Custom function to parse the input value into a valid Date object. | (date: string | Date) => Date | - |
inputOnChangeDelay 5.0.0+ | Defines the delay (in milliseconds) for the input field's onChange event. | number | - |
inputReadOnly | Toggle the readonly state for the component. | boolean | - |
invalid | Set component validation state to invalid. | boolean | - |
label 4.2.0+ | Add a caption for a component. | ReactNode | - |
locale | Sets the default locale for components. If not set, it is inherited from the browser. | string | 'default' |
maxDate | Max selectable date. | string | Date | - |
minDate | Min selectable date. | string | Date | - |
name 5.3.0+ | The name attribute for the input element. | string | - |
navNextDoubleIcon | The custom next double icon. | ReactNode | - |
navNextIcon | The custom next icon. | ReactNode | - |
navPrevDoubleIcon | The custom prev double icon. | ReactNode | - |
navPrevIcon | The custom prev icon. | ReactNode | - |
navYearFirst 4.3.0+ | Reorder year-month navigation, and render year first. | boolean | - |
navigation | Show arrows navigation. | boolean | - |
onDateChange | Callback fired when the date changed. | (date: Date, formatedDate?: string) => void | - |
onDateHover | Callback fired when the user hovers over the calendar cell. | (date: string | Date) => void | - |
onHide | Callback fired when the component requests to be hidden. | () => void | - |
onSelectEndChange | Callback fired when the selection type changed. | (value: boolean) => void | - |
onShow | Callback fired when the component requests to be shown. | () => void | - |
onViewChanged | Callback fired when the view type of calendar changed. | (view: string) => void | - |
placeholder | Specifies short hints that are visible in start date and end date inputs. | string | string[] | Select date |
portal 5.9.0+ | Generates dropdown menu using createPortal. | boolean | - |
rangesButtonsColor | Sets the color context of the cancel button to one of CoreUI’s themed colors. | 'primary' | 'secondary' | 'success' | 'danger' | 'warning' | 'info' | 'dark' | 'light' | string | - |
rangesButtonsSize | Size the ranges button small or large. | 'sm' | 'lg' | - |
rangesButtonsVariant | Set the ranges button variant to an outlined button or a ghost button. | 'outline' | 'ghost' | - |
required 4.10.0+ | When present, it specifies that date must be filled out before submitting the form. | boolean | - |
selectAdjacementDays 4.11.0+ | Set whether days in adjacent months shown before or after the current month are selectable. This only applies if the showAdjacementDays option is set to true. | boolean | - |
selectionType 5.0.0+ | Specify the type of date selection as day, week, month, or year. | 'day' | 'week' | 'month' | 'year' | - |
separator | Default icon or character character that separates two dates. | ReactNode | - |
showAdjacementDays 4.11.0+ | Set whether to display dates in adjacent months (non-selectable) at the start and end of the current month. | boolean | - |
showWeekNumber 5.0.0+ | Set whether to display week numbers in the calendar. | boolean | - |
size | Size the component small or large. | 'sm' | 'lg' | - |
text 4.2.0+ | Add helper text to the component. | ReactNode | - |
timepicker | Provide an additional time selection by adding select boxes to choose times. | boolean | - |
todayButton | Toggle visibility or set the content of today button. | ReactNode | 'Today' |
todayButtonColor | Sets the color context of the today button to one of CoreUI’s themed colors. | 'primary' | 'secondary' | 'success' | 'danger' | 'warning' | 'info' | 'dark' | 'light' | string | 'primary' |
todayButtonSize | Size the today button small or large. | 'sm' | 'lg' | 'sm' |
todayButtonVariant | Set the today button variant to an outlined button or a ghost button. | 'outline' | 'ghost' | - |
toggler | The content of toggler. | ReactNode | - |
tooltipFeedback 4.2.0+ | Display validation feedback in a styled tooltip. | boolean | - |
valid | Set component validation state to valid. | boolean | - |
visible | Toggle the visibility of dropdown menu component. | boolean | - |
weekNumbersLabel 5.0.0+ | Label displayed over week numbers in the calendar. | string | - |
weekdayFormat | Set length or format of day name. | number | 'long' | 'narrow' | 'short' | ((date: Date) => string | number) | 2 |