React Date Range Picker Component
Date Range Picker
Limited-time offer for the first 100 customers in 2025. Use code 2025SKY25 at checkout.
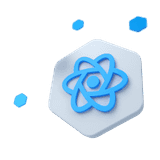
Other Frameworks
CoreUI components are available as native Angular, Bootstrap (Vanilla JS), and Vue components. To learn more please visit the following pages.
Example#
Explore basic usage of the Date Range Picker through sample code snippets that demonstrate its core functionality.
Days#
Select date ranges by day with examples including basic usage, as well as variants featuring time pickers and footers for enhanced functionality.
With timepicker#
Our React DateRangePicker component not only supports date selection but also includes a time picker feature for selecting a specific time of day. Enable the React TimePicker by passing a timepicker
prop to the <CDatePicker/>
component.
With footer#
Here's an example with an additional footer. The footer is useful for displaying extra information or actions related to the selected date, such as "Today" or "Clear" buttons. The footer component is fully customizable and can be styled to match the rest of the application.
Weeks#
To enable week selection, set the selectionType
property to week
and pass the showWeekNumber
prop to display week numbers.
Months#
Set the selectionType
property to month
to enable months range selection.
Years#
Set the selectionType
property to year
to enable years range selection.
Sizing#
Set heights using size
property like size="lg"
and size="sm"
.
Disabled#
Add the disabled
boolean attribute on an input to give it a grayed out appearance and remove pointer events.
Readonly#
Add the inputReadOnly
boolean attribute to prevent modification of the input's value.
Disabled dates#
Our React Date Range Picker component features the ability to disable specific dates, such as weekends or holidays, by using the disabledDate
prop. This prop takes an array and uses custom logic to determine which dates should be disabled.
Disabling weekends#
You can disable weekends by passing a function to the disabledDates
prop. Here's how to do it:
Custom ranges#
In order to configure custom date ranges in the Date Range component, you must use the ranges
prop to define a set of predefined ranges. These ranges can include predefined options such as "Today", "Yesterday", "Last 7 Days", etc.
Locale#
CoreUI's React Date Range Picker enables the display of dates and times in non-English locales, making it essential for international users and multilingual support.
Auto#
By default, the DateRangePicker component uses the default browser locale, but it can be easily configured to use a different locale supported by the JavaScript Internationalization API. To set the locale, you can pass the desired language code as a prop to the DateRangePicker component. This feature enables the creation of more inclusive and accessible applications that cater to a diverse audience.
Chinese#
Here is an example of a simple React Date Range Picker with Chinese localization.
Japanese#
Below is an example of a basic React Date Range Picker with Japanese locales.
Korean#
Here's a simple example of a Date Range Picker with Korean locales.
Right to left support#
RTL support is built-in and can be explicitly controlled through the $enable-rtl
variables in scss.
Hebrew#
Persian#
Custom formats#
Heads up! As of v5.0.0, the format
property is removed in <CDateRangePicker>
. Instead, utilize the inputDateFormat
to format dates into custom strings and inputDateParse
to parse custom strings into Date objects.
The provided code demonstrates how to use the inputDateFormat
and inputDateParse
properties. In this example, the format
and parse
functions from date-fns
are employed to tailor the date presentation and interpretation.
import { format, parse } from 'date-fns'import { es } from 'date-fns/locale'
The inputDateFormat
property formats the date into a custom string, while the inputDateParse
property parses a custom string into a Date object. The code showcases the date range in different formats based on locale, such as 'MMMM dd, yyyy' and 'yyyy MMMM dd', and accommodates different locales, like 'en-US' and 'es-ES'.
With timepicker#
If you need to display custom date and time formats, use the timepicker
property along with inputDateParse
and inputDateFormat
. This example shows how you can customize both the date and time formats, allowing users to select a range with time specified, such as 'MMM dd, yyyy h:mm:ss a'
.
Weeks#
If you want to show weeks in a custom format, set the selectionType
to 'week'
. This example demonstrates how to use inputDateParse
and inputDateFormat
to present and parse week-based date strings, like 'wo yyyy'
. Including the showWeekNumber
property allows you to display week numbers, which can be formatted according to your needs.
Months#
If your application requires month selection in a specific format, use the selectionType
set to 'month'
. This example shows how to customize the month display using inputDateFormat
and how to parse month strings with inputDateParse
, allowing for formats such as 'MMM yyyy'
.
Years#
If you need to format years in a custom way, use the selectionType
set to 'year'
. This example provides guidance on using inputDateFormat
and inputDateParse
to work with custom year formats like 'yy'
.
API#
Check out the documentation below for a comprehensive guide to all the props you can use with the components mentioned here.