React Range Slider Component
Range Slider
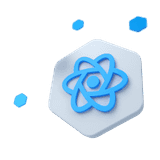
Enhance your forms with our customizable React Range Slider component for advanced range selection.
Other Frameworks
CoreUI components are available as native Angular, Bootstrap (Vanilla JS), and Vue components. To learn more please visit the following pages.
Overview#
The React Range Slider component allows users to select a value or range of values within a predefined range. Unlike the standard <input type="range">
, the Range Slider offers enhanced customization options, including multiple handles, labels, tooltips, and vertical orientation. It ensures consistent styling across browsers and provides a rich set of features for advanced use cases.
Features#
- Multiple Handles: Select single or multiple values within the range.
- Custom Labels: Display labels at specific points on the slider.
- Tooltips: Show dynamic tooltips displaying current values.
- Vertical Orientation: Rotate the slider for vertical layouts.
- Clickable Labels: Enable users to click on labels to set slider values.
- Disabled State: Disable the slider to prevent user interaction.
Basic React Range Slider#
Create a simple range slider with default settings.
Multiple handles#
Enable multiple handles to allow the selection of a range or/and multiple values.
Vertical Range Slider#
Rotate the slider to a vertical orientation.
Disabled#
Disable the slider to prevent user interaction.
Min and max#
React Range Slider component has implicit values for min
and max
—0
and 100
, respectively. You may specify new values for those using the min
and max
attributes.
Steps#
Range Slider inputs automatically "snap" to whole numbers. To modify this behavior, set a step
value. In the example below, we increase the number of steps by specifying step="0.25"
.
Distance#
Sets the minimum distance between multiple slider handles by setting distance
and ensures that the handles do not overlap or get too close.
Labels#
Add labels to specific points on the slider for better context. If you provide an array of strings, as in the example below, then labels will be spaced at equal distances from the beginning to the end of the slider.
Labels customization#
Labels can be configured as an array of strings or objects. When using objects, you can specify additional properties like value
, label
, className
, and style
.
Clickable labels#
By default, users can click on labels to set the slider to specific values. You can disable this feature by setting clickableLabels
to false
.
Tooltips#
By default, tooltips display the current value of each handle. You can disable tooltips by setting tooltips
to false
Tooltips formatting#
Customize the content of tooltips using the tooltipsFormat
option. This can be a function that formats the tooltip text based on the current value.
Track#
The track
property allows you to customize how the slider's track is displayed. By default, the track
property is set to 'fill'
enabling dynamic filling of the track based on the slider's current value(s). This means the filled portion of the track will adjust automatically as the slider handle(s) move, offering a responsive visual representation of the selected range.
Disable filling#
If you set track
to false
, the slider's track will not display any fill. Only the default track background will be visible, which can be useful for minimalist designs or when you use more then two handles.
Accessibility#
The React.js Range Slider component is built with accessibility in mind. Each slider handle includes the following ARIA attributes:
role="slider"
aria-valuemin
: Minimum valuearia-valuemax
: Maximum valuearia-valuenow
: Current valuearia-orientation
: horizontal or vertical
Additionally, ensure that labels and tooltips are clear and descriptive to provide the best experience for all users.
API#
Check out the documentation below for a comprehensive guide to all the props you can use with the components mentioned here.