React Loading Button Component
Loading Button
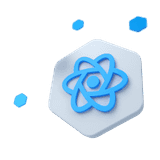
React loading buttons are interactive elements that provide visual feedback to users, indicating that an action is being processed. These buttons typically display a loading spinner or animation.
Other Frameworks
CoreUI components are available as native Angular, Bootstrap (Vanilla JS), and Vue components. To learn more please visit the following pages.
Example#
Create basic React Loading Buttons with different styles: primary, outline, and ghost. These buttons show a loading state when clicked.
<CLoadingButton color="primary" timeout={2000}>Submit</CLoadingButton><CLoadingButton color="primary" variant="outline" timeout={2000}>Submit</CLoadingButton><CLoadingButton color="primary" variant="ghost" timeout={2000}>Submit</CLoadingButton>
Spinners#
Border (Default)#
The default option. Use loading buttons with a border spinner to indicate loading status.
<CLoadingButton color="info" timeout={2000}>Submit</CLoadingButton><CLoadingButton color="success" variant="outline" timeout={2000}>Submit</CLoadingButton><CLoadingButton color="warning" variant="ghost" timeout={2000}>Submit</CLoadingButton>
Grow#
Switch to a grow spinner for React loading buttons by adding spinnerType="grow"
.
<CLoadingButton color="info" spinnerType="grow" timeout={2000}>Submit</CLoadingButton><CLoadingButton color="success" spinnerType="grow" variant="outline" timeout={2000}>Submit</CLoadingButton><CLoadingButton color="warning" spinnerType="grow" variant="ghost" timeout={2000}>Submit</CLoadingButton>
API#
Check out the documentation below for a comprehensive guide to all the props you can use with the components mentioned here.