How to capitalize the first letter in JavaScript?
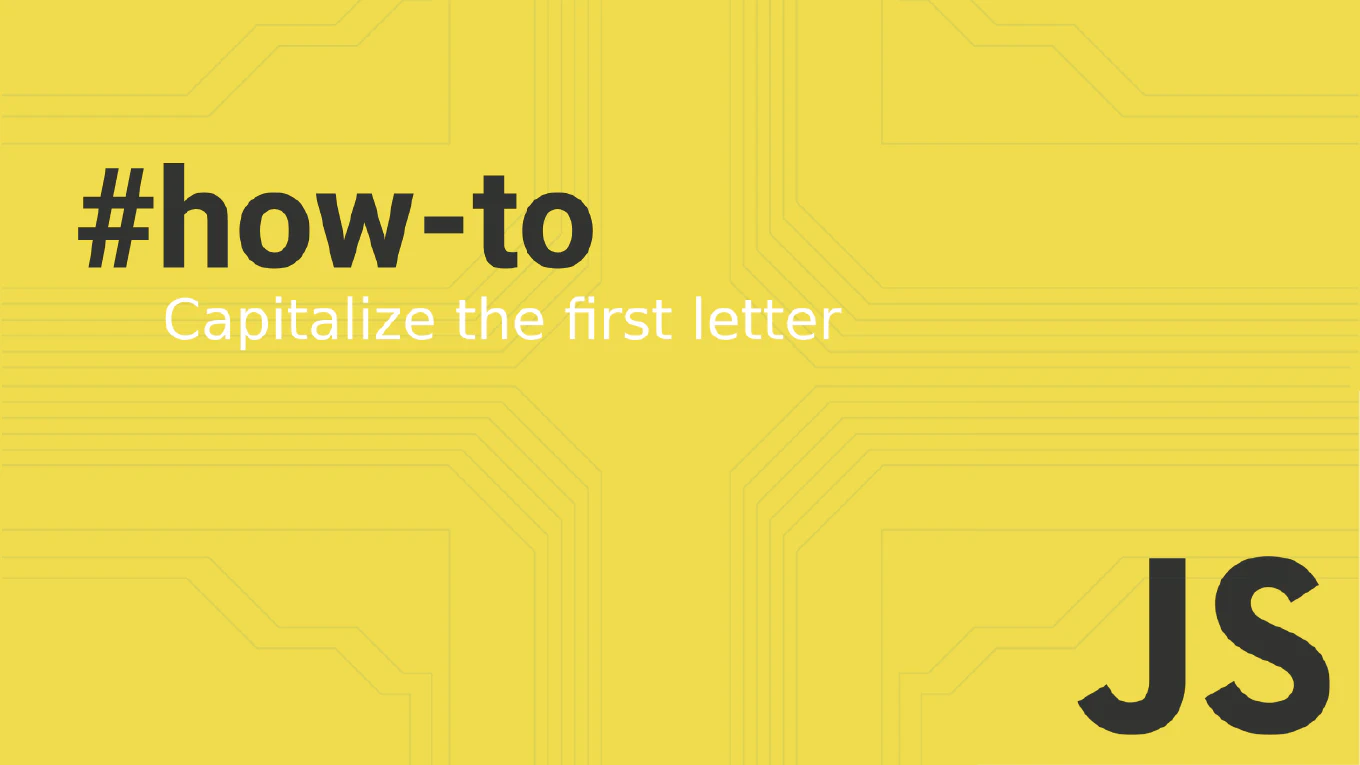
In the vast landscape of web development, manipulating strings is akin to a daily ritual. Among the myriad of manipulations, capitalizing the first letter of a string stands out for its simplicity and necessity. This seemingly straightforward task can be achieved through various JavaScript techniques, each with its unique flair. But why stop at JavaScript? In the world of web styling, CSS also offers a sleek solution. This blog post explores five methods to capitalize the first letter of strings in JavaScript and dips into the CSS realm for a styling-based approach.
Method 1: Using charAt(), toUpperCase(), and slice()
This method is a testament to the power of simplicity. It uses basic string manipulation methods to achieve our goal.
function capitalizeFirstLetter(string) {
return string.charAt(0).toUpperCase() + string.slice(1)
}
// Example usage
const example1 = 'javascript'
console.log(capitalizeFirstLetter(example1)) // Output: Javascript
Explanation: This function takes a string as an argument and capitalizes its first letter. It does this by using charAt(0)
to access the first character of the string and toUpperCase()
to convert this character to uppercase. The slice(1)
method is then used to extract the rest of the string starting from the second character. These two parts—the capitalized first character and the rest of the string—are concatenated together to form the capitalized string.
Method 2: Regex and replace()
Leverage the power of regular expressions to capitalize the first letter succinctly.
function capitalizeFirstLetter(string) {
return string.replace(/^./, string[0].toUpperCase())
}
// Example usage
const example2 = 'javascript'
console.log(capitalizeFirstLetter(example2)) // Output: Javascript
Explanation: This function also capitalizes the first letter of the input string, but it uses a regular expression (/^./
) combined with the replace()
method. The regular expression matches the first character of the string, and replace()
replaces this character with its uppercase version. This method is efficient for directly targeting and transforming the first character without splitting the string.
Method 3: split(), map(), and join()
Dive into array methods to transform your string, showcasing JavaScript’s functional programming capabilities.
function capitalizeFirstLetter(string) {
return string.split('').map((char, index) =>
index === 0 ? char.toUpperCase() : char).join('')
}
// Example usage
const example3 = 'javascript'
console.log(capitalizeFirstLetter(example3)) // Output: Javascript
Explanation: In this approach, the input string is first split into an array of individual characters using split('')
. The map()
function is then used to iterate over this array, applying a condition that transforms only the first character (at index 0) to uppercase. All other characters remain unchanged. Finally, join('')
merges the characters back into a single string. This method effectively demonstrates the use of array methods for string manipulation.
Method 4: ES6 Spread Syntax and join()
Embrace the modern JavaScript syntax for a more concise and readable solution.
function capitalizeFirstLetter(string) {
return [...string][0].toUpperCase() + [...string].slice(1).join('')
}
// Example usage
const example4 = 'javascript'
console.log(capitalizeFirstLetter(example4)) // Output: Javascript
Explanation: This method utilizes ES6 spread syntax to spread the string into an array of its characters. It then capitalizes the first character of the array (which corresponds to the first character of the string) and concatenates this with the rest of the characters, which are merged back into a string using join('')
. This method highlights the flexibility of ES6 features for concise and effective string manipulation.
Method 5: CSS for Styling Text
Sometimes, the solution lies outside the script. CSS’s text-transform
property can visually capitalize the first letter of text within a paragraph, offering a purely stylistic approach.
p::first-letter {
text-transform: capitalize
}
Explanation: This CSS rule targets the first letter of every <p>
element and applies capitalization, affecting only the rendering of the text, not its underlying content.
FAQ
Why capitalize the first letter of a string?
Capitalizing the first letter is often used for formatting purposes, making text aesthetically pleasing and easier to read, especially in titles and headings.
How can I capitalize the first letter of each word in JavaScript?
To capitalize the first letter of each word, you can split the string into words, capitalize the first letter of each, and then join them back together. Here’s how you can do it:
function capitalizeWords(string) {
return string.split(' ').map(word =>
word.charAt(0).toUpperCase() + word.slice(1)
).join(' ')
}
// Example usage
const sentence = 'capitalize each word in this sentence'
console.log(capitalizeWords(sentence)); // Output: Capitalize Each Word In This Sentence
This code splits the input string into an array of words, uses map() to capitalize the first letter of each word, and then joins the array back into a single string with spaces.
Does JavaScript have a built-in method for capitalizing the first letter?
No, JavaScript does not include a built-in method specifically for this purpose, but as shown, several straightforward approaches can achieve it.
Is the CSS method applicable for dynamic content?
The CSS method is a great solution for static pages or content that does not require manipulation after rendering. For dynamic content, JavaScript methods provide more flexibility.
Can I use these methods to capitalize letters after special characters or in a sentence?
Yes, for capitalizing letters following special characters or spaces (like after a period), you might adjust the regex in Method 2 or modify the split() argument in Method 3 to target these specific cases.
Conclusion
This comprehensive guide has walked you through multiple pathways to capitalize the first letter of strings in JavaScript, along with a CSS styling trick. Each method offers unique insights into string manipulation and text styling, ensuring your text is presented exactly as you desire.