How to Center a Button in CSS
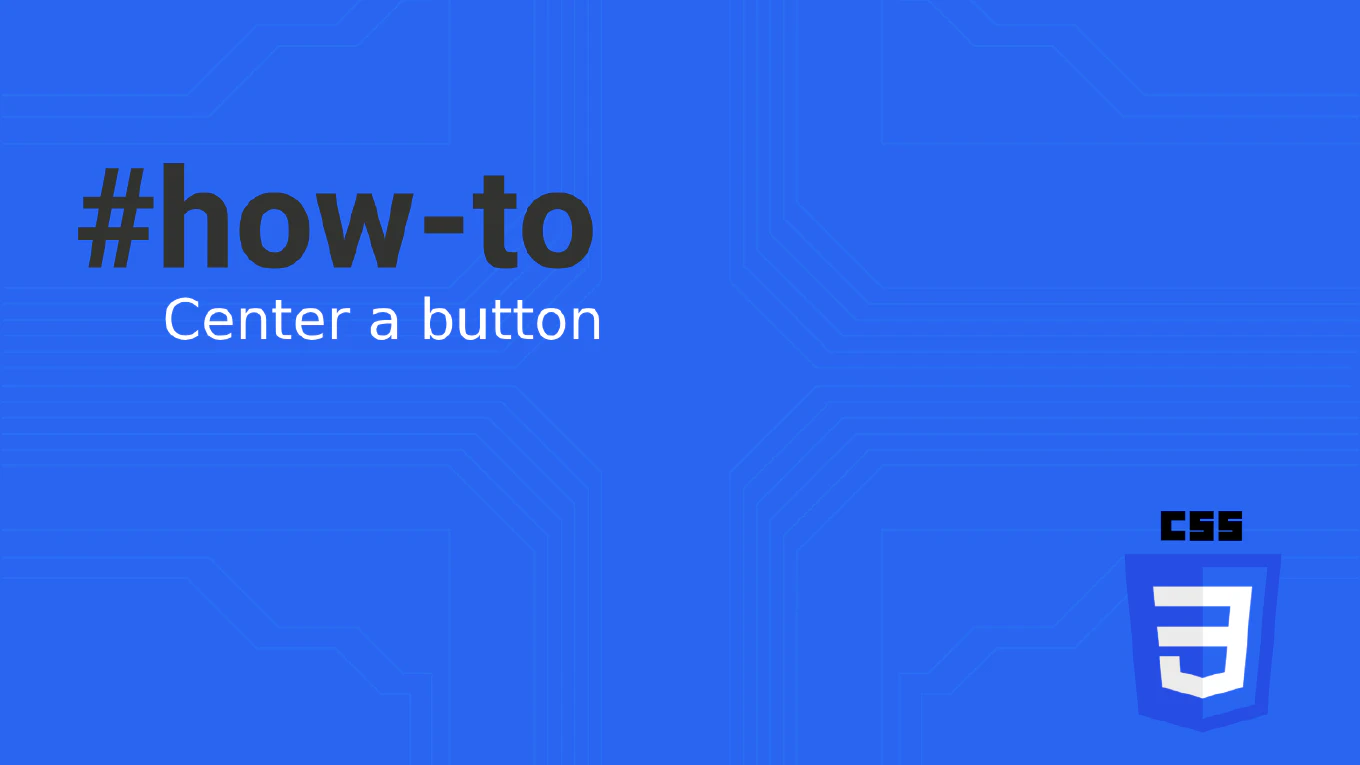
Centering a button in CSS can be achieved using several methods. Here are some simple techniques to help you center a button horizontally and vertically on your web page. The following examples will guide you through the use of CSS centering techniques.
Using CSS Flexbox
CSS Flexbox is a powerful CSS property that makes centering elements easy. To center a button inside a parent container, use the following CSS:
.container {
display: flex; /* Enables CSS flexbox */
justify-content: center; /* Centers horizontally */
align-items: center; /* Centers vertically */
height: 100vh; /* Full height to demonstrate vertical centering */
}
button {
padding: 10px 20px;
}
HTML:
<div class="container">
<button>Centered Button</button>
</div>
CSS Flexbox is especially useful because it adjusts the layout dynamically, making it an excellent choice for responsive designs.
Using CSS Grid
CSS Grid is another layout method that simplifies centering elements within a div element.
.container {
display: grid; /* Enables CSS Grid */
place-items: center; /* Centers both horizontally and vertically */
height: 100vh; /* Full height to demonstrate vertical centering */
}
button {
padding: 10px 20px;
}
HTML:
<div class="container">
<button>Centered Button</button>
</div>
CSS Grid is highly versatile, allowing you to create complex layouts with simple CSS properties.
Using Text Align and Line Height
For simpler layouts, you can use the text-align property and line-height.
.container {
text-align: center; /* Centers horizontally */
height: 100vh; /* Full height to demonstrate vertical centering */
line-height: 100vh; /* Same as height to center vertically */
}
button {
vertical-align: middle; /* Aligns button vertically within the line-height */
padding: 10px 20px;
}
HTML:
<div class="container">
<button>Centered Button</button>
</div>
This method is straightforward but less flexible for complex layouts.
Using Absolute Positioning
Absolute positioning can be useful for more complex layouts.
.container {
position: relative;
height: 100vh; /* Full height to demonstrate vertical centering */
}
button {
position: absolute;
top: 50%; /* Moves button to the middle vertically */
left: 50%; /* Moves button to the middle horizontally */
transform: translate(-50%, -50%); /* Centers the button */
padding: 10px 20px;
}
HTML:
<div class="container">
<button>Centered Button</button>
</div>
To center a button element vertically and horizontally, absolute positioning can be effectively used with properties like top, left, and transform. This method ensures the button is perfectly centered within its parent container.
Absolute positioning gives precise control over the button’s placement but requires a well-defined parent element.
Using Margin Auto
Another simple method to center a block-level element, like a button, is by setting the display property to block and using margin: auto to utilize the display property for centering.
button {
display: block; /* Makes the button a block element */
margin: 0 auto; /* Centers horizontally */
}
HTML:
<div class="container">
<button>Centered Button</button>
</div>
This method is straightforward and works well for horizontal centering.
Conclusion
Centering a button in CSS can be done in various ways, depending on your layout needs. Flexbox and Grid are modern and powerful methods, while text-align, line-height, absolute positioning, and margin auto can be useful for simpler or more specific scenarios. Try out these methods and see which works best for your project!
For more detailed tutorials, visit our CSS Blog. Happy coding!
Additional Tips
- Flexbox: Great for dynamic layouts that need to adjust based on screen size.
- CSS Grid: Ideal for complex layouts where precise control over rows and columns is needed.
- Text Align and Line Height: This method is simple for centering inline elements within a block-level container.
- Absolute Positioning: Best for fixed layouts where the button’s position relative to its container is critical.
- Margin Auto: Easy for centering block-level elements horizontally.
Practice Makes Perfect
Experiment with these methods in different scenarios to understand their strengths and limitations. With practice, you’ll be able to choose the right method for any layout challenge.