How to compare dates with JavaScript
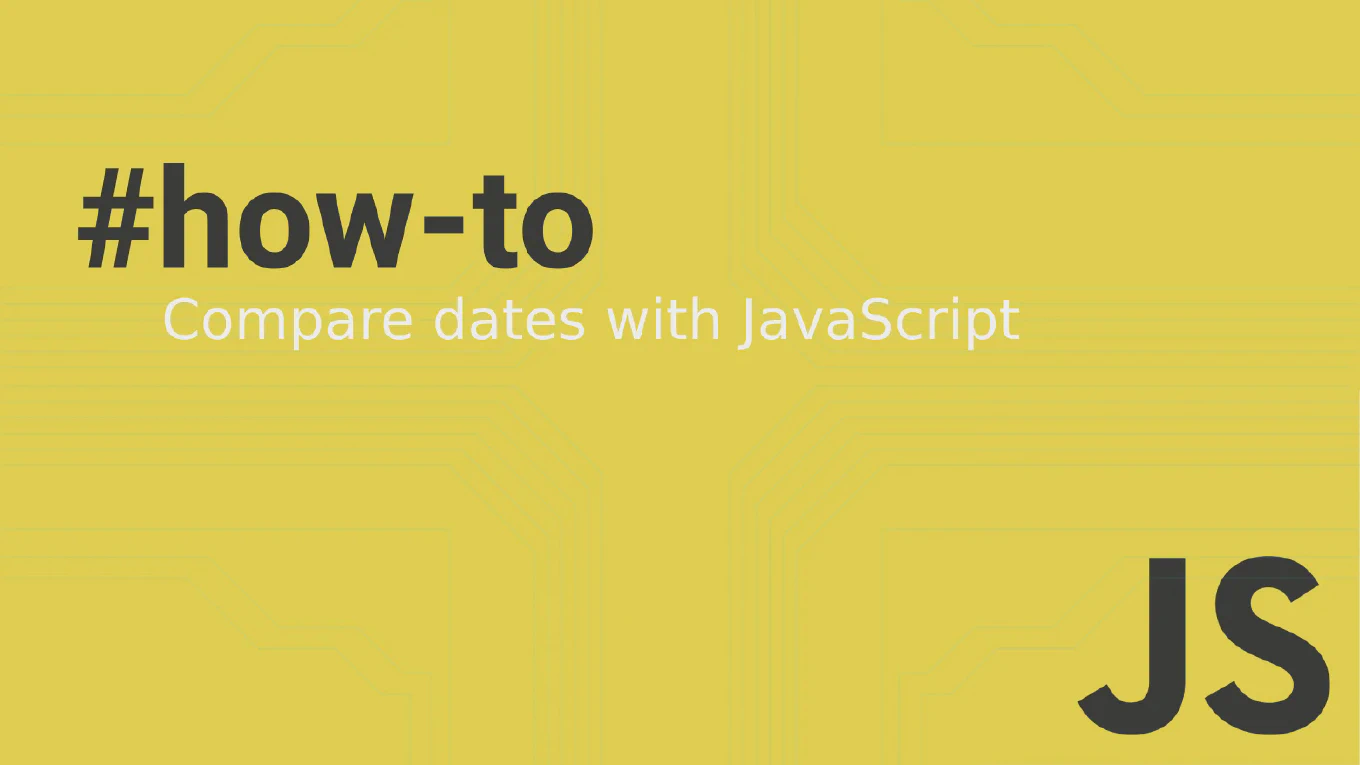
As the digital world intertwines more deeply with our lives, understanding the intricacies of web development becomes increasingly relevant. JavaScript stands at the forefront of this realm, with its ability to enrich user experiences. Among its many capabilities, the power to compare dates in JavaScript is a particularly useful skill that enables developers to deal with schedules, events, and time-based data more effectively.
Why Compare Dates in JavaScript?
The need for date comparison in JavaScript is ubiquitous across web applications. From scheduling systems, to calendar widgets, to validation of expiration dates on forms, the applications are endless. But why is it crucial to have a good grasp of date comparison? The answer lies in the details—accurate date manipulation ensures the seamless functioning of features that depend on temporal data. It’s the difference between a smooth user experience and a frustrating one.
Understanding The Date Object in JavaScript
Before delving into the nitty-gritty of date comparison, it’s essential to understand the Date object—a built-in object in JavaScript that stores date and time. It provides methods for date and time manipulation, including adding, subtracting, and comparing dates.
Here’s how you can initialize a date object:
const today = new Date();
const specificDate = new Date(2024, 0, 1); // January 1, 2024
Note that JavaScript months are zero-indexed, meaning January is 0, February is 1, and so on up to December being 11.
Strategies to Compare Dates in JavaScript
Using Comparison Operators
JavaScript’s comparison operators (>
, <
, ==
, ===
, !=
, !==
) can be used to compare two Date objects directly.
const date1 = new Date(2024, 0, 1);
const date2 = new Date(2024, 0, 15);
if (date1 < date2) {
console.log('date1 is earlier than date2');
}
Time Method Comparison
Another way to compare dates is by converting them to their time value—a number representing milliseconds since January 1, 1970, UTC.
const date1 = new Date('2024-01-01').getTime();
const date2 = new Date('2024-01-15').getTime();
if (date1 < date2) {
console.log('date1 is earlier than date2');
}
toISOString Method
For comparing across different time zones, converting dates to a standardized format like ISO string can level the playing field:
const date1 = new Date('2024-01-01T00:00:00Z').toISOString();
const date2 = new Date('2024-01-15T00:00:00Z').toISOString();
if (date1 < date2) {
console.log('date1 is earlier than date2');
}
Edge Cases and Pitfalls
While comparing dates seems straightforward, several edge cases and pitfalls can cause bugs and erroneous behavior:
- Timezone Differences: Always be aware of the time zone when creating date objects.
- Leap Years: Special cases like February 29 should be handled explicitly to avoid miscalculations.
- Daylight Saving Time: This can affect the time part of Date objects and lead to incorrect comparisons if not accounted for.
Best Practices for Comparing Dates
To ensure robust and error-free date comparison in JavaScript, adopt these best practices:
- Use Libraries When Necessary: Utilizing libraries like Moment.js or Date-fns can simplify date manipulations and comparisons by handling edge cases and timezones effectively.
- Thorough Testing: Implement unit tests covering different scenarios like leap years and time boundaries.
- UTC Over Local Time: Whenever possible, use Coordinated Universal Time (UTC) to avoid inconsistencies related to local time settings.
Wrapping Up and Going Forward
Mastering date comparison in JavaScript equips developers with the tools necessary to create dynamic and responsive web applications. Understanding the nuances of the Date object and employing best practices will not only spare users from confusion and frustration but also contribute to a polished end product.
When you’ve absorbed the contents of this guide, put your skills into practice. As with any programming venture, the real learning comes from doing. And when you’ve implemented a feature that hinges on accurately comparing dates, take a moment to thank those who’ve paved the way with open-source resources like freeCodeCamp—a testament to the generous spirit of the coding community.
In the dynamic landscape of web development, keep your skills current with continuing education and stay in the loop about new libraries and standards that could change the way you handle dates and time.
Dive into date comparisons with confidence, and remember that every line of code adds up to a greater understanding of the vast JavaScript ecosystem!