How to concatenate a strings in JavaScript?
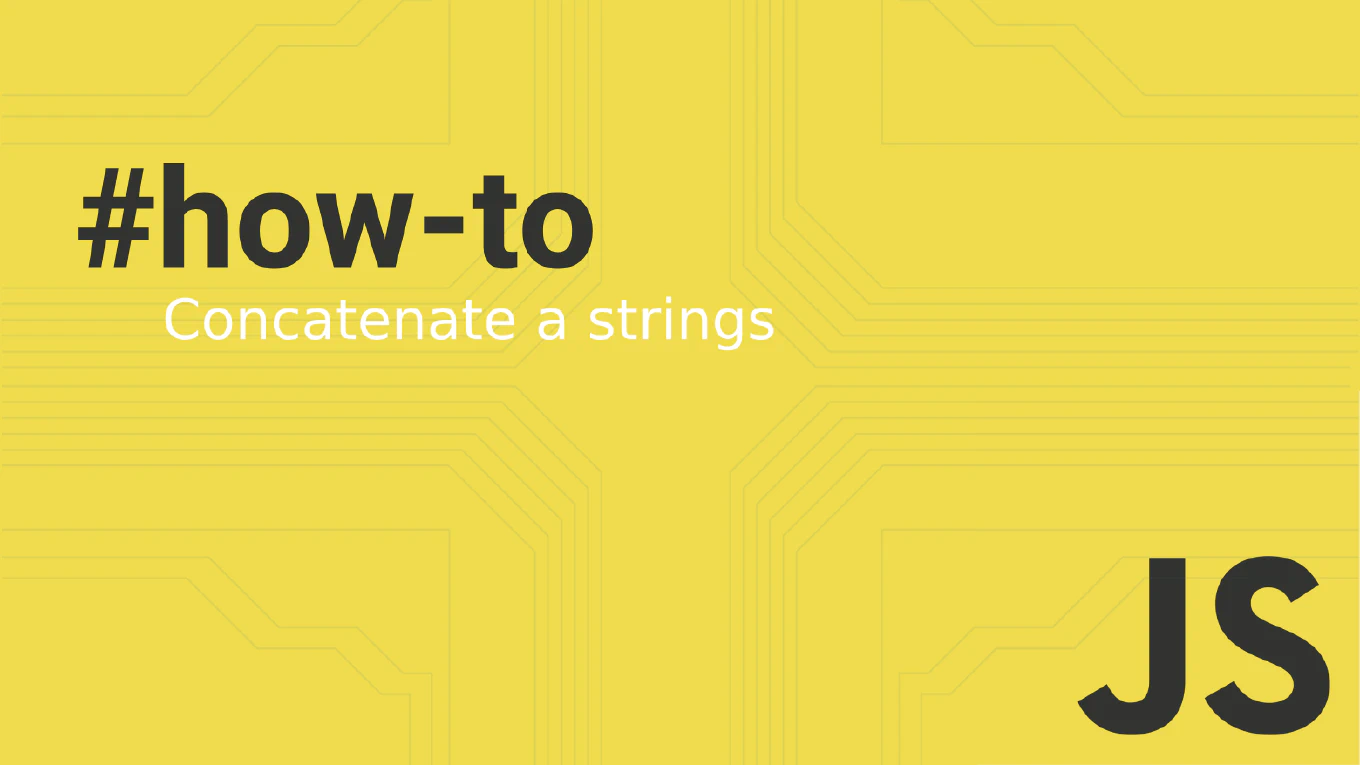
String manipulation, particularly string concatenation, is a cornerstone of JavaScript programming. This guide delves deep into the various techniques for concatenating strings, offering insights and examples to elevate your JavaScript coding skills. Whether you’re a novice or an experienced developer, understanding how to combine strings efficiently will significantly enhance your web development projects.
What is String Concatenation?
String concatenation is the process of joining two or more strings to form a new string. It’s a fundamental concept that finds application in creating dynamic content, processing user inputs, and much more within web development.
Core Methods for Concatenating Strings
The + Operator: A Basic Approach
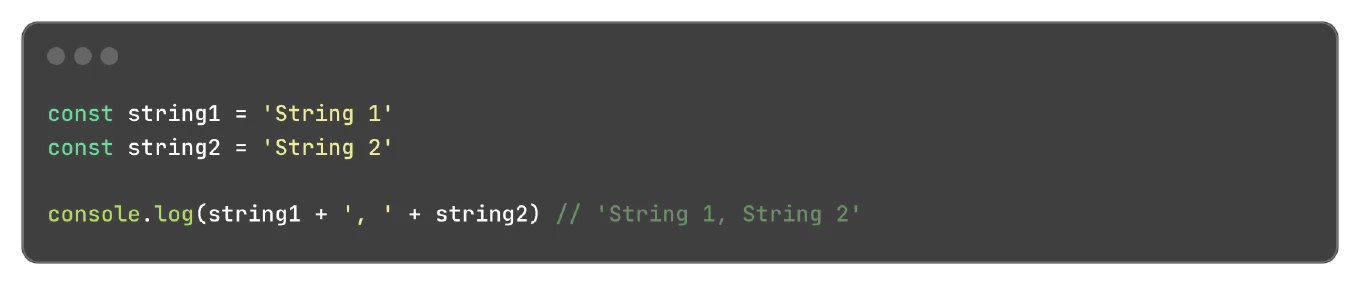
The simplest way to concatenate strings is by using the + operator. This concat method is straightforward and widely used for combining two strings or adding variables to strings.
const greeting = 'Hello'
const name = 'Alice'
console.log(greeting + ', ' + name + '!') // 'Hello, Alice!'
Template Literals: Enhancing Readability

Template literals offer a more advanced and readable approach to string concatenation. Enclosed by back ticks (`), they allow you to embed expressions and variables directly within the string literal.
const firstName = 'Alice'
console.log(`Hello, ${firstName}!`) // 'Hello, Alice!''
The Array.join() Method: For List Concatenation

When it comes to joining array items into a single string, the join method is incredibly effective. It uses a specified separator to combine strings from an array.
const words = ['Hello', 'world']
console.log(words.join(' ')) // 'Hello world'
The String.concat() Method: Merging Multiple Strings
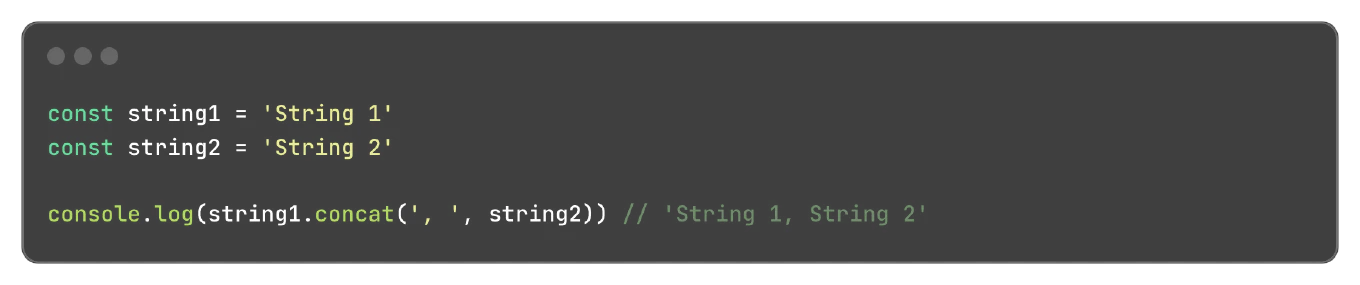
The concat method is a built-in JavaScript function that merges one or more strings into an existing string. Despite being less popular than the + operator and template literals, it serves as a powerful tool for creating new strings.
const string1 = 'Hello'
const string2 = 'world'
console.log(string1.concat(' ', string2)) // 'Hello world'
When to Use Each Method
- Simple concatenations: Opt for the + operator.
- Embedding variables or expressions: Template literals are your best bet for readability and ease of use.
- Combining array elements: The join method excels here, especially with custom separators.
- Appending multiple values: Consider concat for its ability to handle multiple string values at once.
Best Practices and Tips
- Avoid missing spaces: A common issue with the + operator. Template literals inherently solve this by clearly defining string parameters.
- Escape characters: Template literals eliminate the need to escape single or double quotes, making your javascript code cleaner.
- Browser compatibility: While template literals are supported by modern browsers, ensure to transpile your code for compatibility with Internet Explorer.
Advanced Concatenation Techniques
Working with Special Characters
Handling special characters and escaping double quotes can be tricky with traditional concatenation methods. Template literals provide a straightforward solution, as special characters and quotes can be included without escaping.
Concatenating Different Data Types
JavaScript performs type conversion automatically when concatenating strings with other data types. For clarity and predictability, explicitly convert values to strings when necessary.
FAQs on String Concatenation
Q: Can I concatenate strings with non-string variables?
A: Yes, JavaScript automatically converts variables of different data types to strings during concatenation. However, using template literals or explicit type conversion is recommended for clarity.
Q: What method should I use for concatenating strings from an array?
A: The array join method is ideal for this scenario, as it allows you to specify a separator and combines strings from an array efficiently.
Q: Are there performance differences between these methods?
A: While there might be minor performance differences, they are negligible for most use cases. Focus on readability and the specific requirements of your javascript code.
Conclusion
Whether you’re crafting a simple greeting message or generating complex dynamic content, mastering string concatenation techniques in JavaScript is essential. By choosing the right method and adhering to best practices, you can ensure your code is both efficient and readable. Remember, the best approach depends on the specific needs of your project and your personal coding style. Happy coding!
By understanding these concatenation techniques and applying them judiciously in your JavaScript projects, you’re well on your way to becoming a more effective and versatile developer.