How to create a single page application using React.js
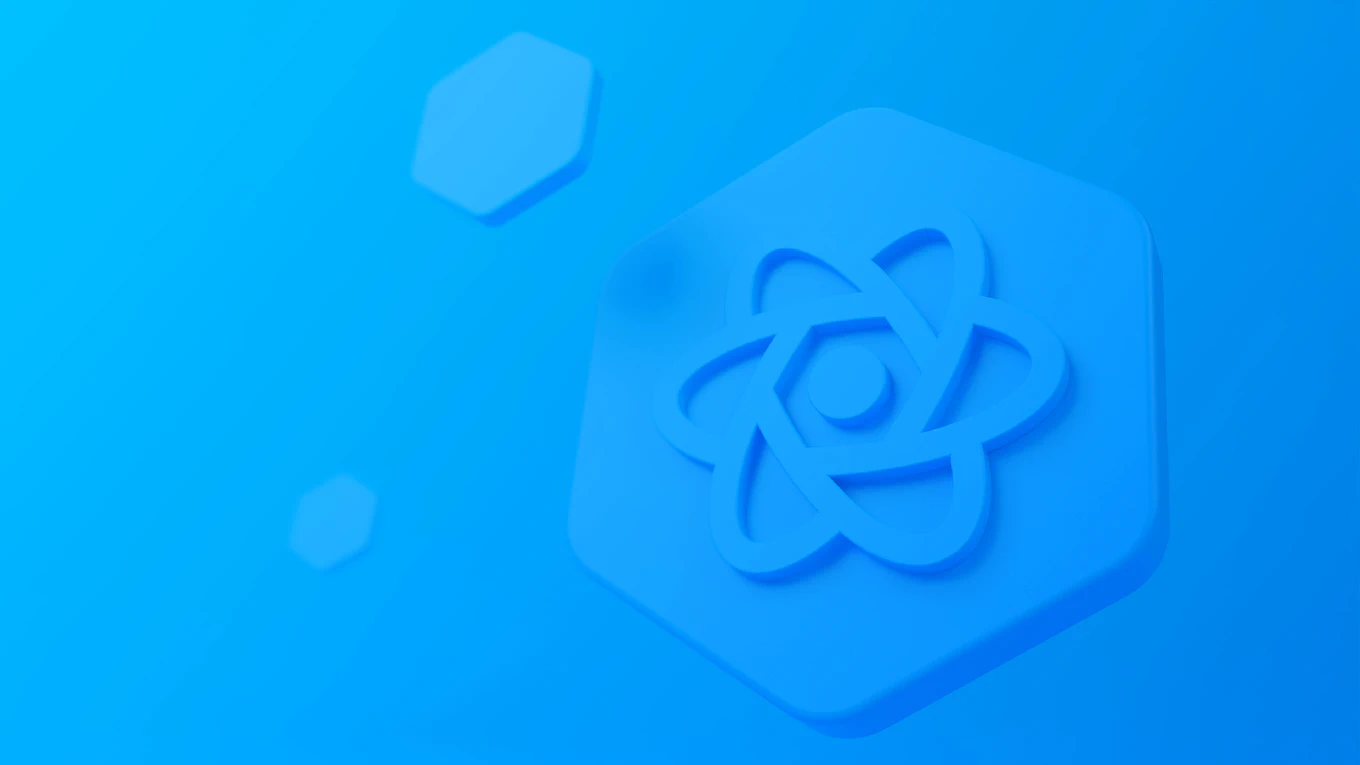
In this tutorial, you will learn how to create a single page application using ReactJS. You will learn how to create a simple ReactJS application that renders a simple message box.
If you are looking to build a web application in a few hours, I have some good news.
This article will show you how to quickly create a single-page application using ReactJS
I know how frustrating it is to find a single-page application that works.
I am a fan of building applications, but I would not say I like that feeling of having to re-write things each time I need to update a minor part of my web app.
What Is A Single Page Application?
A Single Page Application (SPA) is a web application that is designed to be displayed as a single, static page.
This approach makes the application more user-friendly and easier to navigate, as users can see the entire application at once.
With a traditional web application, users are redirected to a new page every time they make a change to their data, which can be frustrating if they want to edit information in multiple places.
In contrast, SPA-based applications are completely focused on one thing at a time, allowing users to work through each step in a consistent manner.
Single Page Application vs Multi Page Application
Lets compare these two kinds of application;
Single Page Application
UI/UX: Single Page Applications (SPA) are a great way to create a fantastic user experience on mobile devices.
They consist of a single web page that loads all content using JavaScript. They request the markup and data independently and render pages straight to the browser.
Reloading: Single-page applications are web pages that only require a single HTML page to load.
They are generally used to perform actions that would take too long to load via traditional web pages, such as downloading files or rendering images.
A single-page application comprises HTML and JavaScript, usually including a server-side language (such as PHP) that processes data and returns the results.
Multiple Page Applications
UI/UX: Multi-page applications are helpful because they allow users to access more information in one place.
However, the user experience can be limited because the user must load multiple pages and navigate between them. This cannot be very clear to the user.
Reloading: The multi-page application (MPA) design pattern is a common approach to building web applications. It provides an easy way for users to navigate multiple pages without re-submit their data each time.
The example of MPA is our React Admin Dashboard Template
Advantages of SPAs
A single page application has several advantages over traditional web applications. Here are some of the most notable:
Simplicity: SPAs are easier to develop and maintain than traditional web applications. Developers only need to build a single HTML file for the SPA. No server-side changes are necessary.
Reusability: You can reuse the same JavaScript, CSS, and HTML code for multiple pages, and there are tools that allow developers to package these components into reusable modules.
Security: SPAs make it easier to secure web pages, because they can be protected by a firewall or require login credentials.
Creating Single Page Application With React
You have to follow the steps below for building a react single page application;
- Use your desired location to create the react app with the command below;
npx create-react-app app-name
App-name directory to be built in the following default files:
app-name
├── README.md
├── node_modules
├── package.json
├── .gitignore
├── public
│ ├── favicon.ico
│ ├── index.html
│ ├── logo192.png
│ ├── logo512.png
│ ├── manifest.json
│ └── robots.txt
└── src
├── App.css
├── App.js
├── App.test.js
├── index.css
├── index.js
├── logo.svg
├── serviceWorker.js
└── setupTests.js
- Execute the following command to install react-router-dom:
npm install react-router-dom
- Wrapping The App Component
React Router has 2 types of routers: BrowserRouter and HashRouter. We’re using BrowserRouter in this example because it makes the URL’s look like example.com/about rather than example.com/#/about. React Router uses a hash (hash) at the end of the URL to determine what page to load.
You must include the code below in your src.index.js:
import React from "react"
import { render } from "react-dom"
import { BrowserRouter } from "react-router-dom"
import App from "./App"
render(
<BrowserRouter>
<App />
</BrowserRouter>,
document.querySelector("#root")
)
- The following code should be used to create a file named src/pages/HomePage.js:
import React from "react";
export default function HomePage() {
return (
<>
<h1>Hey from HomePage</h1>
<p>This is your awesome HomePage subtitle</p>
</>
);
}
- The following code should be used to create a file named src/pages/UserPage.js.
import React from "react";
import { useParams } from "react-router-dom";
export default function UserPage() {
let { id } = useParams();
return (
<>
<h1>Hello there user {id}</h1>
<p>This is your awesome User Profile page</p>
</>
);
}
- Route and switch group all routes together to make sure that they take precedence over each other.
The following routes should be there in your App.js file:
import React from "react"
import { Route, Switch } from "react-router-dom"
// We will create these two pages in a moment
import HomePage from "./pages/HomePage"
import UserPage from "./pages/UserPage"
export default function App() {
return (
<Switch>
<Route exact path="/" component={HomePage} />
<Route path="/:id" component={UserPage} />
</Switch>
)
}
- You can link to a page inside the SPA.
import React from "react"
import { Link } from "react-router-dom"
export default function HomePage() {
return (
<div className="container">
<h1>Home </h1>
<p>
<Link to="/your desired link">Your desired link.</Link>
</p>
</div>
)
}
You are now ready to run the code and see your development at https://localhost:3000.
Frequently Asked Questions
Is ReactJS a single page application?
ReactJS can create a single page application. ReactJS is a JavaScript library for building user interfaces.
What are some examples of single-page applications?
Some examples of single-page applications are: -Gmail -Facebook -Twitter -LinkedIn'
Is React better or angular?
Both React and Angular have their own strengths and weaknesses. Ultimately, the best answer for which framework to choose depends on the specific needs of your project.
Why is React good for single page applications?
React is great for single page applications because it allows developers to create applications that are mobile first and responsive. React allows developers to create applications that are fast and easy to update, which is great for mobile applications.
Conclusion
The best way to learn something is by doing it. So, if you want to learn how to create a single page application using ReactJS, you can follow this tutorial and build a fully functional, real-world application.