How to get element ID in JavaScript
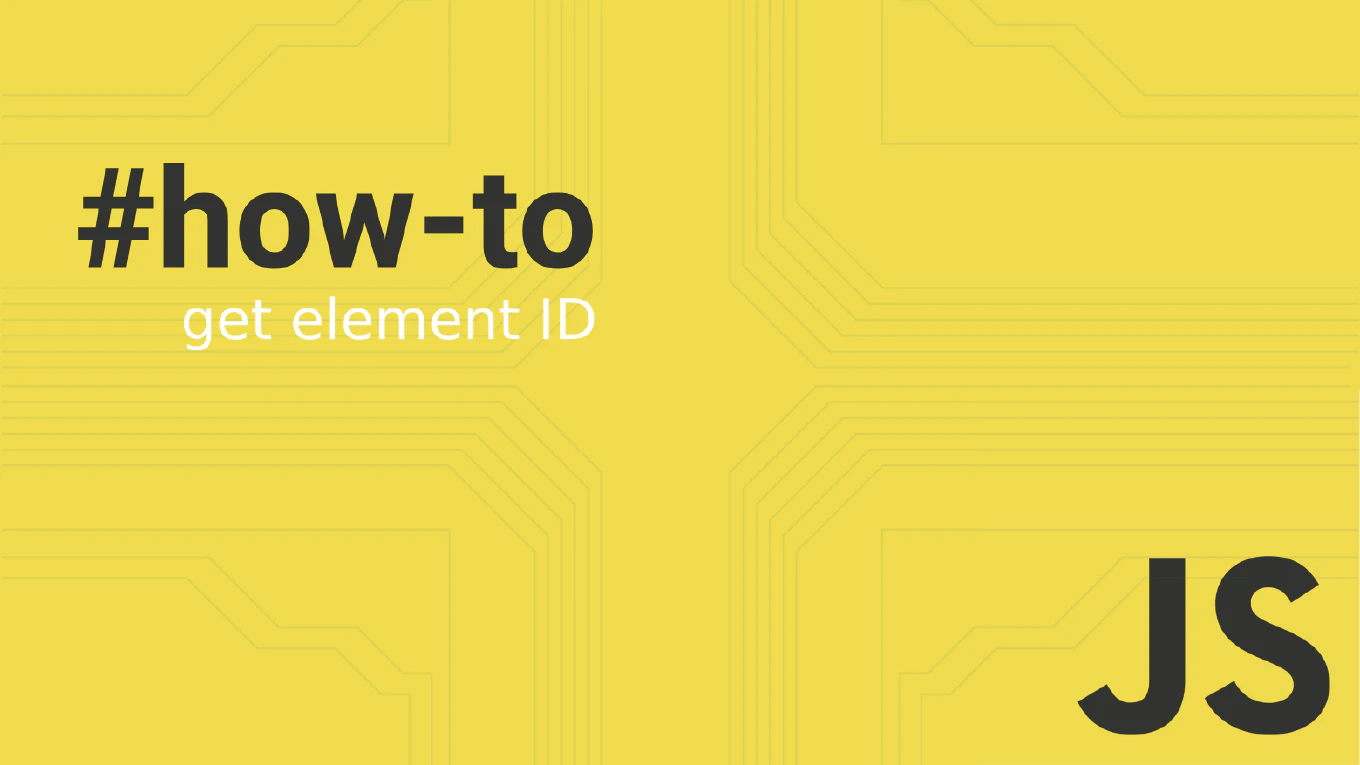
When working with JavaScript, accessing and manipulating HTML elements is a common task. One frequently asked question is, “How can I get an element’s ID value with JavaScript?” This guide will explore various methods to retrieve the ID of an HTML element using JavaScript.
Understanding the id
Attribute
The id
attribute in HTML is a unique identifier assigned to elements. This identifier is case sensitive and must be unique within a document, allowing JavaScript to access specific elements easily.
Using the id
Property
One of the simplest ways to get an element’s ID is by using the .id
property of the DOM element. Here’s an example:
const myElement = document.getElementById('exampleElement')
console.log(myElement.id)
In the above code, document.getElementById
retrieves the element with the specified ID, and the .id
property accesses the ID attribute of the element.
Looping Through Elements
If you need to retrieve the IDs of multiple elements, you can loop through all the elements of a specific type, such as input fields:
const inputs = document.getElementsByTagName('input')
for (let i = 0; i < inputs.length; i++) {
console.log(inputs[i].id)
}
This code retrieves all input elements and alerts their IDs.
Using getAttribute
Method
Another method to get an element’s ID is by using the getAttribute
method:
const element = document.querySelector('.exampleClass')
const idValue = element.getAttribute('id')
console.log(`ID: ${idValue}`)
This method is useful if you prefer to work directly with attributes.
Event Listeners and ID Retrieval
You can also retrieve an element’s ID within an event listener. This is particularly useful for dynamically generated content:
function showId(event) {
const id = event.target.id
console.log(`Button ID: ${id}`)
}
const button = document.getElementById('myButton')
button.addEventListener('click', showId)
Here, the event listener passes the event object to the showId
function, which retrieves and logs the ID of the clicked button.
Using jQuery for ID Retrieval
If you are using jQuery, retrieving an element’s ID becomes even simpler:
$('.exampleClass').each(function() {
const id = $(this).attr('id')
console.log(id)
})
This jQuery code snippet loops through elements with the class exampleClass
and alerts their IDs.
Handling Edge Cases
Be cautious when accessing the id
property, as it can return unexpected values if an element has a child with the name ‘id’.
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Get Element ID Example</title>
</head>
<body>
<form id="myExampleForm">
<input type="hidden" id="id" name="id" />
</form>
<script>
const form = document.getElementById('myExampleForm');
console.warn(form.id)
</script>
</body>
</html>
Ensure that the ID is a string to avoid such issues:
const extractId = (element) => {
const { id } = element
if (typeof id === 'string') {
return id
}
return null
}
This function checks if the ID is a string before returning it.
Practical Example
Here’s a complete example that ties everything together:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Get Element ID Example</title>
</head>
<body>
<button id="exampleButton">Click Me</button>
<script>
const button = document.getElementById('exampleButton')
button.addEventListener('click', function() {
const id = button.id
console.log(`Button ID: ${id}`)
})
</script>
</body>
</html>
In this example, clicking the button alerts its ID.
Conclusion
Getting an element’s ID in JavaScript is straightforward, with multiple methods available depending on your needs. Whether using the .id
property, getAttribute
method, or event listeners, JavaScript provides flexible options for accessing and manipulating HTML elements.
By understanding these techniques, you can efficiently retrieve and utilize element IDs in your JavaScript code, enhancing the interactivity and functionality of your web applications.