How to get the last element in an array in JavaScript
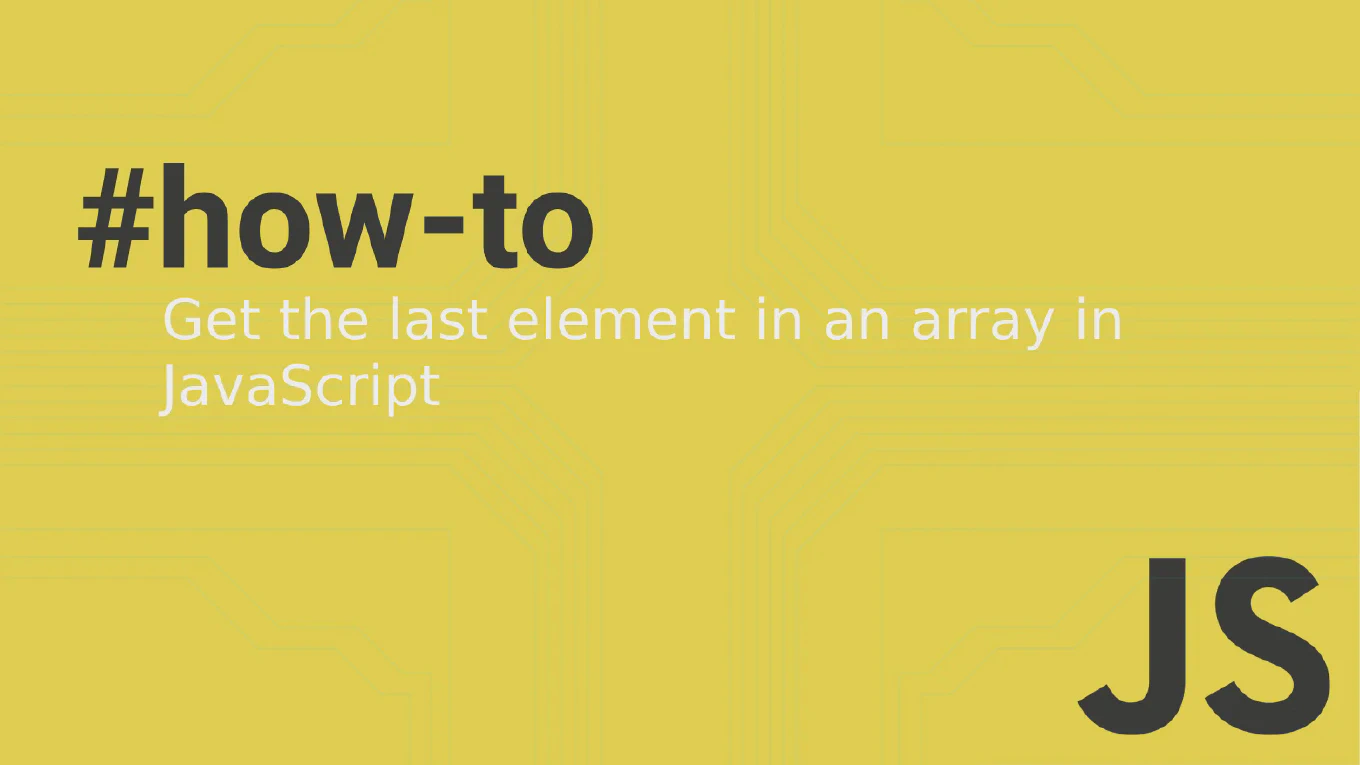
JavaScript arrays are fundamental structures in any developer’s arsenal, designed to store multiple values in a single, easy-to-manage variable. But no matter how proficient you are in JavaScript, there are always new tricks to learn and old ones to be reminded of. One such handy trick is retrieving the last element of an array—an operation that might seem straightforward but comes with nuances worth understanding. In this deep dive into JavaScript arrays, we’ll explore multiple ways to get to that elusive last item and offer insights into which method suits your coding needs best.
Speed up your responsive apps and websites with fully-featured, ready-to-use open-source admin panel templates—free to use and built for efficiency.
What’s an Array and Why the Last Element Matters?
Before we delve into the methods of accessing the last element of an array, it’s crucial to grasp the concept of what an array is. In JavaScript, an array is a container object that holds a predefined number of values of a single type. These values can be anything—from numbers and strings to more complex objects and even other arrays.
Accessing the last element is important for various reasons—perhaps you’re dealing with a data structure where the most recently added information is most pertinent, or your logic requires manipulation of the final entry. Whatever the reason, JavaScript provides several ways to pinpoint and retrieve the last item in an array.
Extracting the Last Element: Various Methods Explored
By Leveraging the Array Length Property
The length property of an array reveals the number of elements it contains. Since JavaScript arrays are zero-indexed—meaning, the index of the first element is 0—the index of the last element will always be one less than the length of the array. Here’s the simplest way to get the last item:
const array = [1, 2, 3, 4, 5, 6, 7, 8, 9]
const lastElement = array[array.length - 1]
console.log(lastElement) // Output: 9
Utilizing the slice()
Method for Non-Destructive Access
The slice()
method serves up a new array containing elements from a specified range. If you pass a negative index to it, slice()
counts backward from the end, making it a clean way to access the last element without altering the original array:
const array = [1, 2, 3, 4, 5, 6, 7, 8, 9]
const lastElement = array.slice(-1)[0]
console.log(lastElement) // Output: 9
The pop()
Method: Quick but Destructive
Using pop()
might be the quickest way to grab the last element, but it comes with a significant trade-off: it changes the array’s size by removing the last element. If you need the last element and don’t mind altering the original array, pop()
is your method:
const array = [1, 2, 3, 4, 5, 6, 7, 8, 9]
const lastElement = array.pop()
console.log(lastElement) // Output: 9
Benchmarking: Which Method Wins the Race?
Performance might be critical based on the application you’re working on. Let’s pit these three methods against each other:
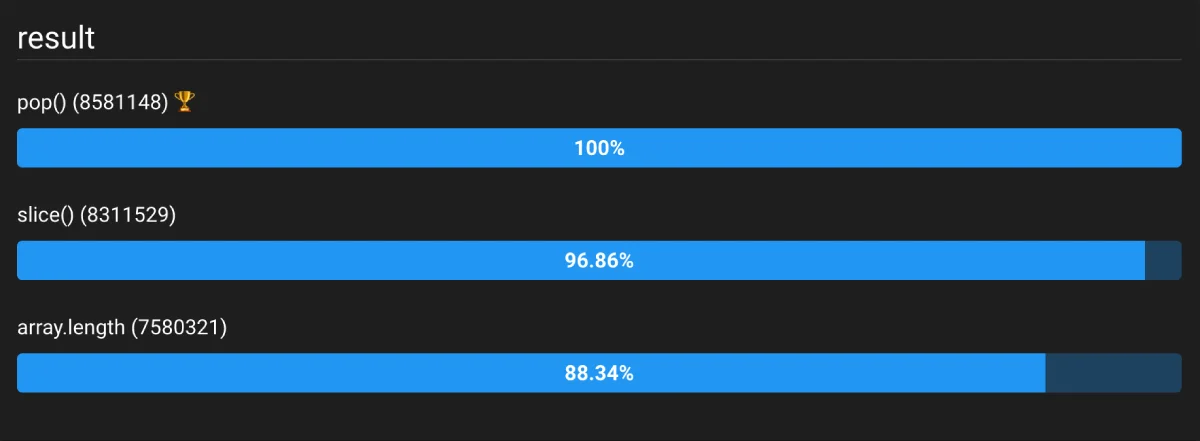
While the pop()
method manifests as the fastest in most cases, it’s important to consider its side effects on the array. If preserving the array is a priority, the slice()
method becomes your go-to, offering a middle ground in terms of performance and non-destructiveness.
Conclusion: The Last Element Uncovered
Retrieving the last element of a JavaScript array is an operation of deceptive simplicity. By understanding the intricacies of the length
property, the slice()
method, and the pop()
function, developers can choose the most suitable approach depending on whether speed or non-mutability is their primary concern.
Making informed choices about these methods can optimize the performance, readability, and maintainability of your code. Whether you’re a seasoned JavaScript developer or sharpening your coding skills, knowing these array manipulation techniques is indispensable.
Learning how to efficiently manage and access array elements ensures you can handle data smoothly, allowing you to focus on the more complex logic and functionality of your applications. Remember, the smallest details in your code can sometimes make the most significant impact.
Now, equipped with this knowledge, your next step could be to delve into more advanced array operations or perhaps explore JavaScript’s other features. Whichever path you take, keep experimenting, keep learning, and let your JavaScript journey continue.
FAQ
What is an array in JavaScript?
An array in JavaScript is a container object that can hold multiple values at once, making it easier to manage related data.
Why is accessing the last element of an array important?
It’s often crucial for operations that need the most recent addition or for specific manipulations on the last entry of the array.
How can I retrieve the last element of an array using the length property?
const array = [1, 2, 3, 4, 5]
const lastElement = array[array.length - 1]
console.log(lastElement) // Output: 5
Is there a non-destructive way to access the last element of an array?
Yes, the slice() method provides a non-destructive way by returning a new array containing the last element:
const lastElement = array.slice(-1)[0]
console.log(lastElement) // Output: 5
Can I use the pop() method to get the last element?
Yes, but pop() removes the element from the array, altering its size:
const lastElement = array.pop()
console.log(lastElement) // Output: 5
Which method is the fastest for accessing the last element?
pop()
is typically fastest but modifies the array. slice()
is a non-destructive alternative, with direct access via the length property being the simplest method.
How do I choose the right method for my needs?
Choose based on whether you need a non-destructive method (slice()
), fast access with modification (pop()
), or simple read-only access (using the length property).
How to get the index of the last element in an array in JavaScript?
The index of the last element can be found using array.length - 1
, as array indexing starts at 0.
How to get the last element of an array in JavaScript?
The last element of the array can be accessed using array[array.length - 1]
for a direct approach, array.slice(-1)[0]
for a non-destructive method, or array.pop()
if altering the array is acceptable.
How to get the second last element of an array in JavaScript?
To access the second last element, you can use array[array.length - 2]
:
const secondLastElement = array[array.length - 2]
console.log(secondLastElement)
How to get the last element of an array in JavaScript without removing it?
For non-destructive access without using pop()
, use either slice()
or direct indexing:
-
Using
slice()
:const lastElement = array.slice(-1)[0] console.log(lastElement)
-
Using direct indexing:
const lastElement = array[array.length - 1] console.log(lastElement)
How to get the last element of an array in JavaScript without using length?
This can be achieved by using the slice()
method to avoid direct reliance on the array’s length:
const lastElement = array.slice(-1)[0]
console.log(lastElement)
How to pop the last element of an array in JavaScript?
To remove and return the last element, pop()
is used:
const lastElement = array.pop()
console.log(lastElement)