How to limit items in a .map loop in JavaScript
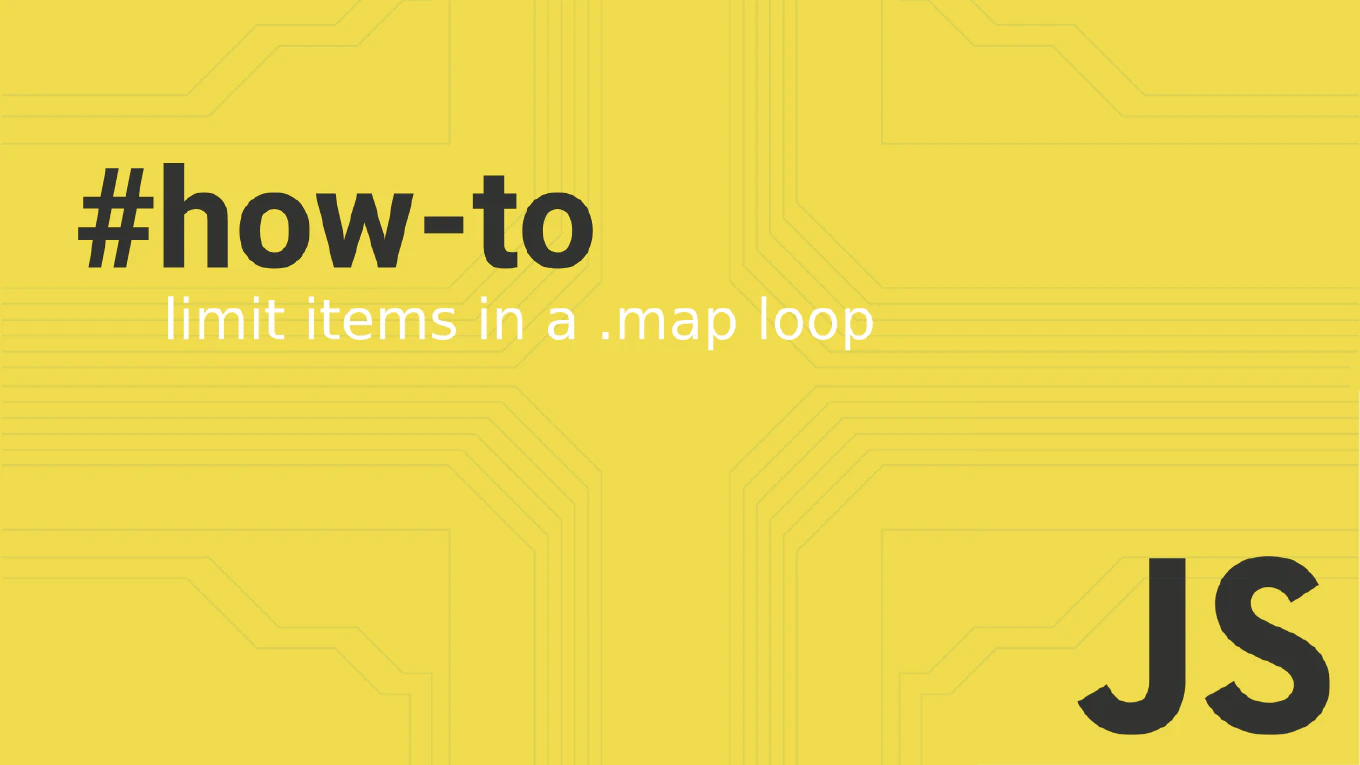
When working with arrays in JavaScript, the .map method is a powerful tool for transforming elements. However, there are scenarios where you need to limit the number of items processed by .map. This can be particularly useful when handling large datasets or when you only need a subset of data for performance reasons. Here, we’ll explore different methods to limit items in a .map loop.
Speed up your responsive apps and websites with fully-featured, ready-to-use open-source admin panel templates—free to use and built for efficiency.
Using .slice
Before .map
The most straightforward way to limit the number of items in a .map
loop is by using the .slice
method. The .slice
method returns a shallow copy of a portion of an array into a new array object selected from start
to end
(end not included).
const data = [1, 2, 3, 4, 5, 6, 7, 8, 9, 10]
const limitedData = data.slice(0, 5).map(item => item * 2)
console.log(limitedData) // Output: [2, 4, 6, 8, 10]
In this example, .slice(0, 5)
extracts the first five elements from the array before applying the .map
method to double each value.
Using .filter
with .map
Another approach is to use the .filter
method to control the number of items passed to .map
. This method can be combined with a condition based on the index.
const data = [1, 2, 3, 4, 5, 6, 7, 8, 9, 10]
const limitedData = data.filter((item, index) => index < 5).map(item => item * 2)
console.log(limitedData) // Output: [2, 4, 6, 8, 10]
Here, .filter((item, index) => index < 5)
ensures only the first five elements are passed to .map
.
Using Index in the .map
Callback
You can also include the index directly within the .map
callback to conditionally process elements.
const data = [1, 2, 3, 4, 5, 6, 7, 8, 9, 10]
const limitedData = data.map((item, index) => {
if (index < 5) {
return item * 2
}
}).filter(Boolean)
console.log(limitedData) // Output: [2, 4, 6, 8, 10]
In this method, the .map
callback checks if the index is less than 5, returning the transformed item only for these indices. The .filter(Boolean)
step removes any undefined
values resulting from indices 5 and above.
Combining .slice
and .map
in React
When working with React, limiting items in a .map
loop can be efficiently managed using .slice
.
const MyComponent = ({ data }) => {
return (
<div>
{data.slice(0, 5).map(item => (
<div key={item.id}>{item.name}</div>
))}
</div>
)
}
In this React component, data.slice(0, 5)
ensures only the first five items are rendered by the .map
method, enhancing performance by limiting unnecessary DOM elements.
Conclusion
Limiting items in a .map
loop can be achieved through various methods like .slice
, .filter
, or conditions within the .map
callback. Each method has its use cases, and choosing the right one depends on your specific scenario. By understanding these techniques, you can optimize your JavaScript code for better performance and readability.