How to loop through an array in JavaScript
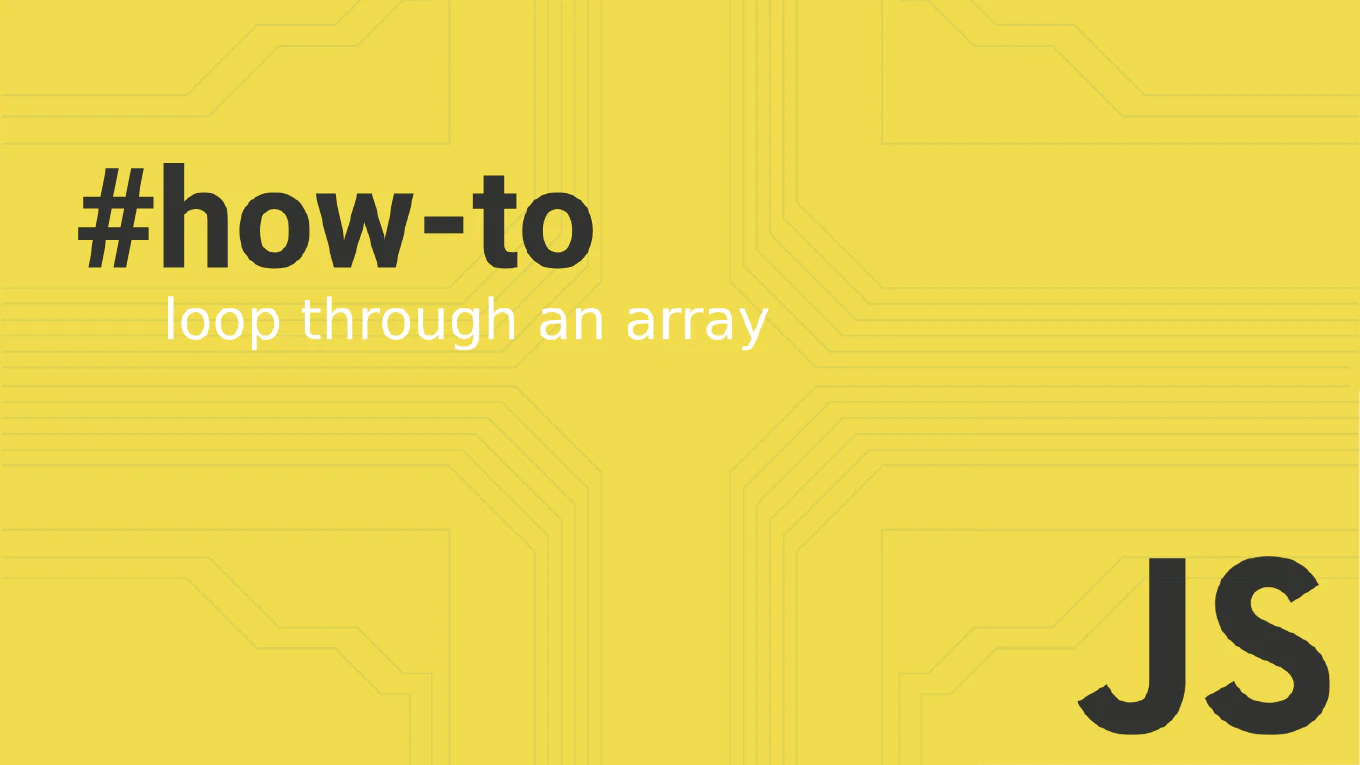
When working with arrays in JavaScript, efficiently looping through array elements is a crucial skill. Here, we’ll explore several methods to iterate over arrays, highlighting their syntax and use cases. Each method allows you to loop through an array, access array elements, and manipulate data effectively.
What is an Array in JavaScript?
An array is a data structure used to store multiple values in a single variable. Each value in an array is called an element, accessible via a numeric index. Arrays in JavaScript are flexible and can store various data types, such as numbers, strings, objects, and even other arrays.
Using while
Loop
The while
loop evaluates a specified condition and executes the loop body as long as the condition is true. This method is useful when the number of iterations is not known beforehand.
const numbers = [1, 2, 3, 4, 5]
let i = 0
while (i < numbers.length) {
console.log(numbers[i])
i++
}
Using for
Loop
The for
loop initializes a variable, checks a condition, and increments the variable in each iteration. It’s a straightforward way to loop through an array and access each element using array indexes.
const numbers = [1, 2, 3, 4, 5]
for (let i = 0; i < numbers.length; i++) {
console.log(numbers[i])
}
Using for…of
Loop
Introduced in ES6, the for…of
loop iterates directly over array elements. This method is cleaner and more readable, especially for arrays and iterable objects.
const numbers = [1, 2, 3, 4, 5]
for (const number of numbers) {
console.log(number)
}
Using forEach
Method
The forEach
method calls a provided function once for each element in the array. It is a higher-order function that simplifies the syntax when working with arrays.
const numbers = [1, 2, 3, 4, 5]
numbers.forEach(number => console.log(number))
Using map
Method
The map
method creates a new array by applying a provided function to each element of the original array. This method is useful for transforming array values and creating new arrays.
const numbers = [1, 2, 3, 4, 5]
const doubled = numbers.map(number => number * 2)
console.log(doubled)
Using reduce
Method
The reduce
method executes a reducer function on each element of the array, resulting in a single output value. This is useful for aggregating data, such as summing up array values.
const numbers = [1, 2, 3, 4, 5]
const sum = numbers.reduce((accumulator, currentValue) => accumulator + currentValue, 0)
console.log(sum)
Using for…in
Loop
The for…in
loop iterates over the enumerable properties of an object, but it can also be used for arrays. However, it’s not recommended for arrays due to potential issues with array indexes and prototype properties.
const numbers = [1, 2, 3, 4, 5]
for (const index in numbers) {
console.log(numbers[index])
}
JavaScript Loop Through Array with Index and Value
You can loop through an array with both the index and value using forEach
. This is helpful when you need both the element and its position.
const numbers = [1, 2, 3, 4, 5]
numbers.forEach((value, index) => {
console.log('Index:', index, 'Value:', value)
})
JavaScript How to Loop Through a 2 Dimensional Array
Looping through a 2D array involves nested loops. Each inner loop iterates over the elements of the sub-array.
const matrix = [
[1, 2, 3],
[4, 5, 6],
[7, 8, 9]
]
for (let i = 0; i < matrix.length; i++) {
for (let j = 0; j < matrix[i].length; j++) {
console.log('Index:', i, j, 'Value:', matrix[i][j])
}
}
Looping Through an Array of Objects
When dealing with an array of objects, you can loop through each object and access its properties. This is common in real-world examples, such as managing lists of users.
const users = [
{ name: 'John', age: 30 },
{ name: 'Jane', age: 25 },
{ name: 'Jim', age: 35 }
]
users.forEach(user => {
console.log('Name:', user.name, 'Age:', user.age)
})
Using map
with an Array of Objects
You can also use the map
method to create a new array based on properties of objects in the original array. This method creates a new array with transformed data.
const userNames = users.map(user => user.name)
console.log(userNames)
Conclusion
JavaScript offers a variety of ways to loop through arrays, each with its own advantages. Understanding these methods allows you to choose the most suitable one for your specific use case. Whether you’re using the classic for
loop, the modern for…of
loop, or array methods like forEach
and map
, iterating over arrays efficiently is a fundamental skill in JavaScript development.