How to Redirect to a New URL Using JavaScript Redirect Techniques
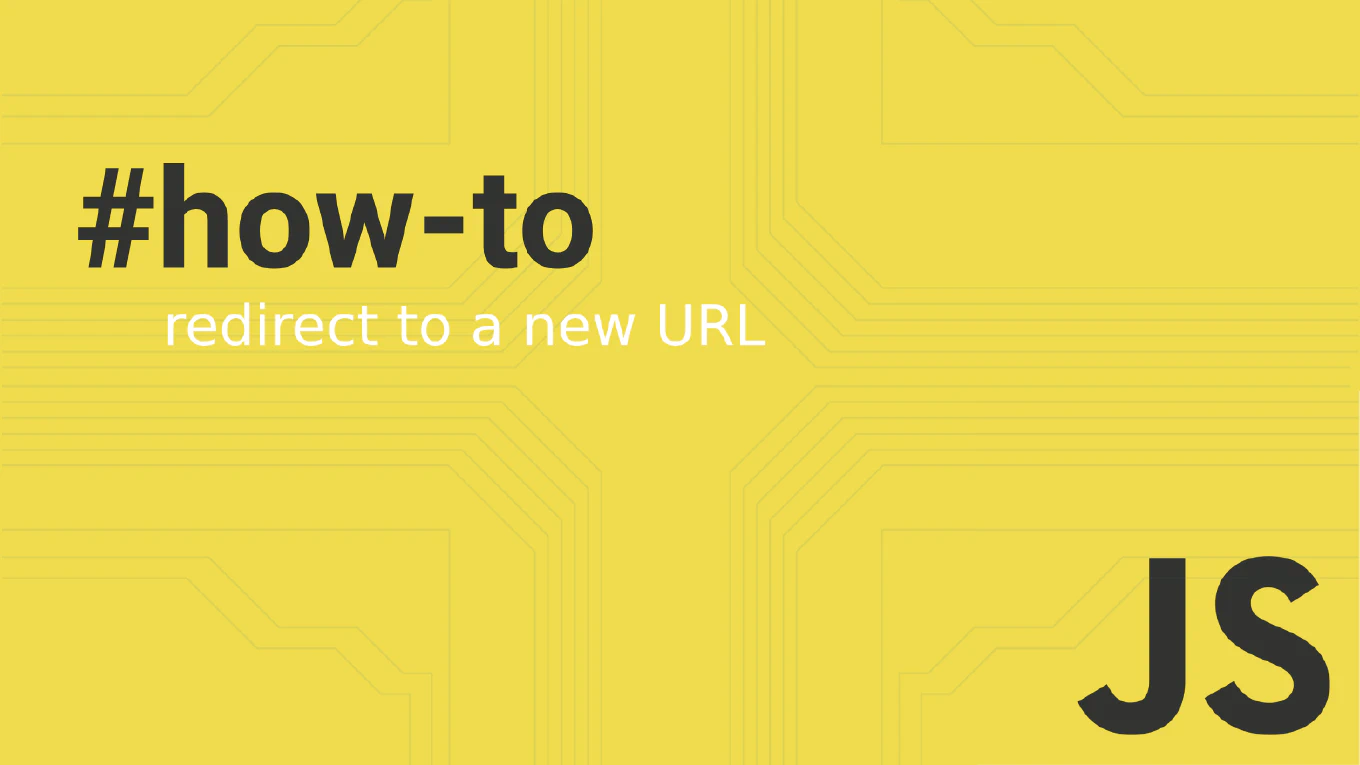
Redirecting users to a new URL using JavaScript is a common and useful practice for web developers. Whether you’re handling successful logins, navigating users after actions, or managing SEO implications, understanding how JavaScript redirects work is crucial. In this guide, you’ll learn the best practices for creating redirects, their implications for SEO performance, and when to choose JavaScript over server-side methods.
Speed up your responsive apps and websites with fully-featured, ready-to-use open-source admin panel templates—free to use and built for efficiency.
What is a JavaScript Redirect?
A JavaScript redirect is a client-side approach that allows you to redirect the user from the current web page to a new URL automatically. Unlike an HTTP redirect, which is executed on the server side and involves specific HTTP status codes (e.g., 301 or 302), JavaScript redirects happen directly within the user’s browser.
Common JavaScript Redirect Methods
The primary way to redirect users with JavaScript involves the window.location
object:
// Basic JS redirect example
const redirectUser = (newUrl) => {
window.location.href = newUrl
}
redirectUser('https://www.example.com')
Here, the href
property of the window.location
object specifies the target URL.
JavaScript vs. Server-Side Redirects
Both JavaScript and server-side redirects have their advantages and drawbacks:
-
Server-side redirects (HTTP Redirect): Recommended for permanent redirects (301) or temporary redirects (302). They are typically faster and better for SEO, as they clearly communicate the new URL to search engines.
-
JavaScript redirects: Useful for dynamic client-side interactions, like redirecting users after form submissions (e.g., successful login to a success page). However, they’re less efficient from an SEO perspective, as search engines might not reliably execute JavaScript, affecting page indexing.
Meta Refresh Redirect
Another client-side redirect method is the HTML meta refresh redirect. It’s implemented directly in the HTML page’s <head>
section:
<meta http-equiv="refresh" content="5;url=https://www.example.com">
This example redirects after 5 seconds, but Google’s SEO guidelines advise against frequent use due to potential confusion for users and search engines.
Comparison: window.location.href
vs. window.location.replace
JavaScript provides two primary methods for URL redirection: window.location.href
and window.location.replace
. Understanding their differences is crucial:
window.location.href
:
- Redirects the user to a specified URL.
- Adds the original page to the browser’s session history.
- Users can use the back button to return to the previous page.
window.location.replace
:
- Redirects the user to a specified URL and replaces the current document in the session history.
- Does not retain the original page in the browser history.
- Users cannot return to the original page using the back button.
Use window.location.replace
when you want to prevent users from navigating back to the previous page, such as after successful login. Use window.location.href
for typical navigation when maintaining browser history is beneficial.
Best Practices for JavaScript Redirects
To ensure your redirects provide a seamless experience without harming your SEO, follow these best practices:
- Avoid redirect loops: Always check your logic carefully to prevent redirect loops that trap users and negatively impact SEO.
- Provide fallback options: Since some older browser versions may fail to execute JavaScript correctly, consider providing alternative navigation.
- Limit user confusion: Clearly indicate if you’re automatically redirecting or give users control through explicit actions like button clicks.
SEO Considerations of JavaScript Redirects
JavaScript redirects can significantly impact SEO performance. Google’s ability to execute JavaScript has improved, but it’s not guaranteed for all scenarios or other search engines. Google advises using server-side redirects when SEO is a priority.
To minimize potential SEO issues:
- Prefer HTTP redirects (301 or 302) for permanent URL changes.
- Use JavaScript redirects primarily for internal user actions or interactions that don’t heavily impact crawling and indexing.
- Ensure internal links point directly to the final URL, minimizing reliance on redirects.
Example: Redirect Users After Successful Login
Below is a common scenario—redirecting users after login to a success page:
// Redirect after successful login
const loginUser = () => {
const loginSuccessful = true
if (loginSuccessful) {
window.location.replace('https://www.example.com/success')
}
}
Using location.replace()
instead of location.href
prevents the previous page from remaining in the browser history, enhancing privacy and navigation.
Key Takeaways
- JavaScript redirects (
window.location.href
,window.location.replace
) are powerful but should be used appropriately. - Server-side redirects are generally better for SEO and should be used for permanent changes.
- Meta refresh redirects are typically discouraged for SEO purposes.
- Ensure redirects are implemented without causing user confusion or negatively impacting your website’s SEO.
By understanding these methods and following best practices, you can effectively create redirections that enhance user experience and support your website’s SEO performance.
Additional Resources
- Google’s guide to JavaScript SEO
- MDN Web Docs: window.location
- CoreUI Documentation for examples of UI interactions
Master JavaScript redirection to create user-friendly websites that also maintain strong SEO.