How to round a number to two decimal places in JavaScript
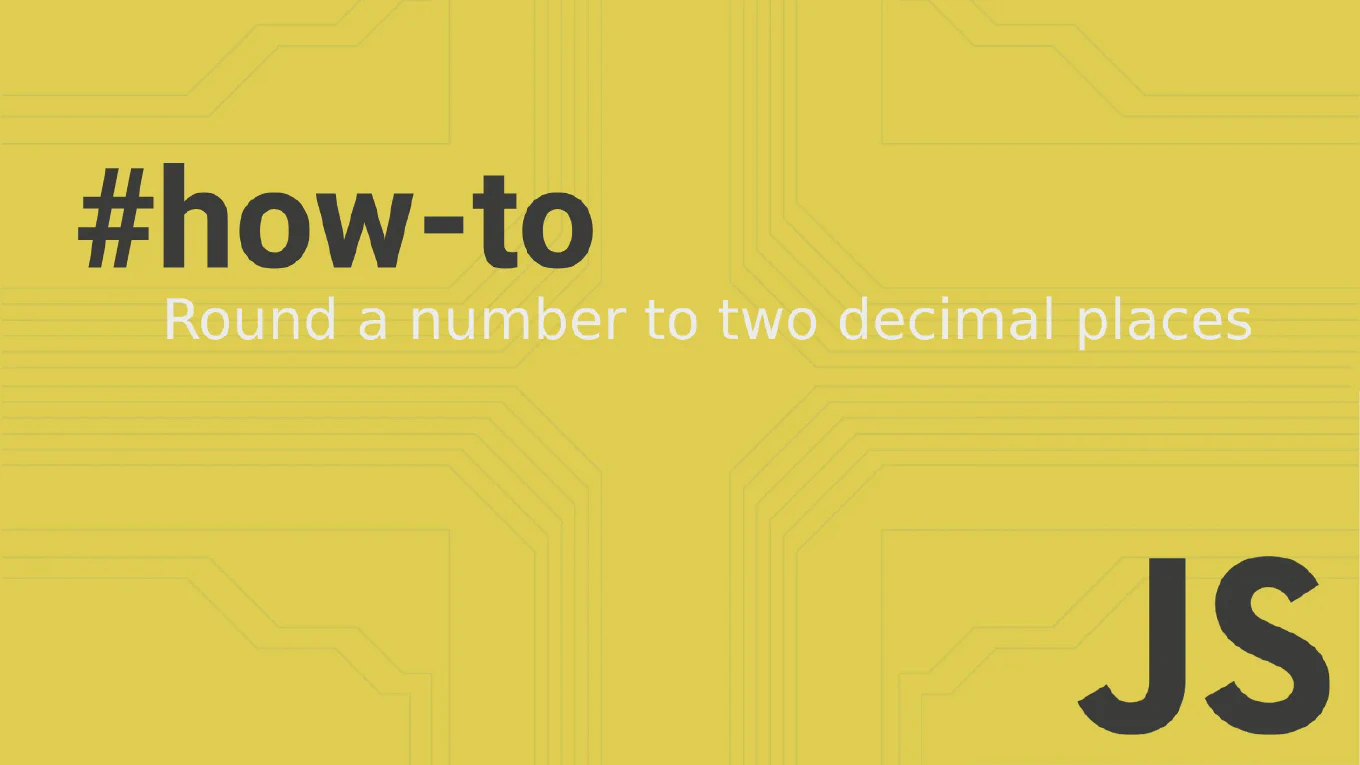
In the ever-evolving landscape of web development, mastering JavaScript nuances can significantly enhance your applications’ functionality and user experience. A joint yet critical operation in numerous computing scenarios is to round numbers to a specific number of decimal places. Among these, rounding to two decimal places is particularly relevant across financial calculations, statistical reporting, and data presentation for its balance between precision and readability. This comprehensive guide will walk you through the intricacies of rounding numbers to two decimal places in JavaScript, ensuring you confidently apply this operation.
The Importance of Precision in Number Handling
JavaScript, the programming language of the Web, is an integral part of software development, enabling dynamic content to run across millions of websites. When it comes to dealing with numbers, especially when they involve decimals, precision is paramount. Whether it’s calculating financial transactions, scientific measurements, or statistical data analysis, the need to round to two decimal places arises frequently.
But why exactly two decimal places? Mainly, it aligns with the most common monetary format used globally, thus simplifying financial calculations. Moreover, in many statistical reports, a two-decimal precision strikes the right balance between accuracy and comprehensibility.
Rounding Numbers in JavaScript: An Overview
Rounding numbers in JavaScript might seem straightforward at first glance, but it encompasses several methods, each with its unique applications. At its core, JavaScript provides built-in functions like Math.round()
, Math.ceil()
, and Math.floor()
, yet these functions round numbers to the nearest whole number. A more nuanced approach is required to achieve rounding to two decimal places.
How to Round a Number to 2 Decimal Places in JavaScript?
The quest for rounding numbers to two decimal places in JavaScript is a common challenge that prompts many developers to seek efficient and reliable solutions. Let’s delve into the specific techniques that enable precise rounding.
1. Using toFixed()
Method
The toFixed()
method is a simple way to round numbers to a specified number of decimal places, returning the result as a string.
const number = 4.687
const rounded = number.toFixed(2)
console.log(rounded) // Outputs: 4.69
Note: Since toFixed()
returns a string, converting it to a number with parseFloat()
is necessary for further numerical operations.
let roundedNumber = parseFloat(number.toFixed(2))
2. Multiplication and Division Trick
Another method to round to two decimal places involves multiplying the number by 100 (shifting the decimal point two places to the right), using the Math.round()
function, and then dividing back by 100.
const number = 4.687
const rounded = Math.round(number * 100) / 100
console.log(rounded) // Outputs: 4.69
This method ensures the result is a number, maintaining the essence of mathematical operations.
3. Dynamic Precision with Math.round()
Combining Math.pow()
with Math.round()
offers a dynamic solution for those seeking a more flexible approach that can adapt to rounding to various decimal places.
function roundTo(num, precision) {
const factor = Math.pow(10, precision)
return Math.round(num * factor) / factor
}
const rounded = roundTo(4.687, 2)
console.log(rounded) // Outputs: 4.69
4. Leveraging Number.EPSILON
for Precision
To address floating-point arithmetic issues, Number.EPSILON
can be a game-changer, ensuring rounding operations are more accurate.
const number = 3.009
const rounded = Math.round((number + Number.EPSILON) * 100) / 100
console.log(rounded) // Outputs: 3.01
5. Custom Rounding Function
A custom function can be versatile for a purely numeric solution without built-in method limitations.
function roundTo(number) {
return +(Math.round(number + "e+2") + "e-2")
}
const rounded = roundTo(3.009)
console.log(rounded); // Outputs: 3.01
6. Rounding with Intl.NumberFormat
For locale-sensitive rounding, Intl.NumberFormat
offers a sophisticated approach to formatting numbers according to local standards.
const number = 4.687
const formatter = new Intl.NumberFormat('en-US', {
style: 'decimal',
maximumFractionDigits: 2,
minimumFractionDigits: 2,
})
const rounded = formatter.format(number)
console.log(rounded) // Outputs: 4.69
This method rounds the number and formats it according to the specified locale, making it invaluable for international applications.
Frequently Asked Questions
-
How do you round to 2 decimal places in general?
The key to rounding to 2 decimal places is to manipulate the number such that the function applies rounding at the correct decimal position, as illustrated through the methods above.
-
How do you round numbers in JavaScript without built-in methods?
If you prefer not to use
toFixed()
due to its string output, using the multiplication and division method or custom functions withMath.round()
provides a purely numeric solution.
Key Takeaways & Next Steps
Mastering the rounding of numbers to two decimal places in JavaScript empowers developers to handle numerical data with greater precision and flexibility. Whether your application requires financial calculations, data presentation, or any scenario demanding precise numerical manipulation, the methods outlined in this guide offer robust solutions to achieve desired outcomes.
You can tackle various rounding challenges in your JavaScript projects by understanding toFixed(), the multiplication and division trick, and the dynamic precision rounding technique.
Beyond rounding numbers, consider exploring JavaScript’s extensive mathematical functions to enhance your applications’ numerical handling capabilities. Remember, in web development, every bit of precision counts towards creating seamless, functional, and user-friendly experiences.
Leverage this knowledge to refine your programming skills and contribute to developing high-quality software that meets users’ needs with precision and accuracy.