How to sleep in Javascript
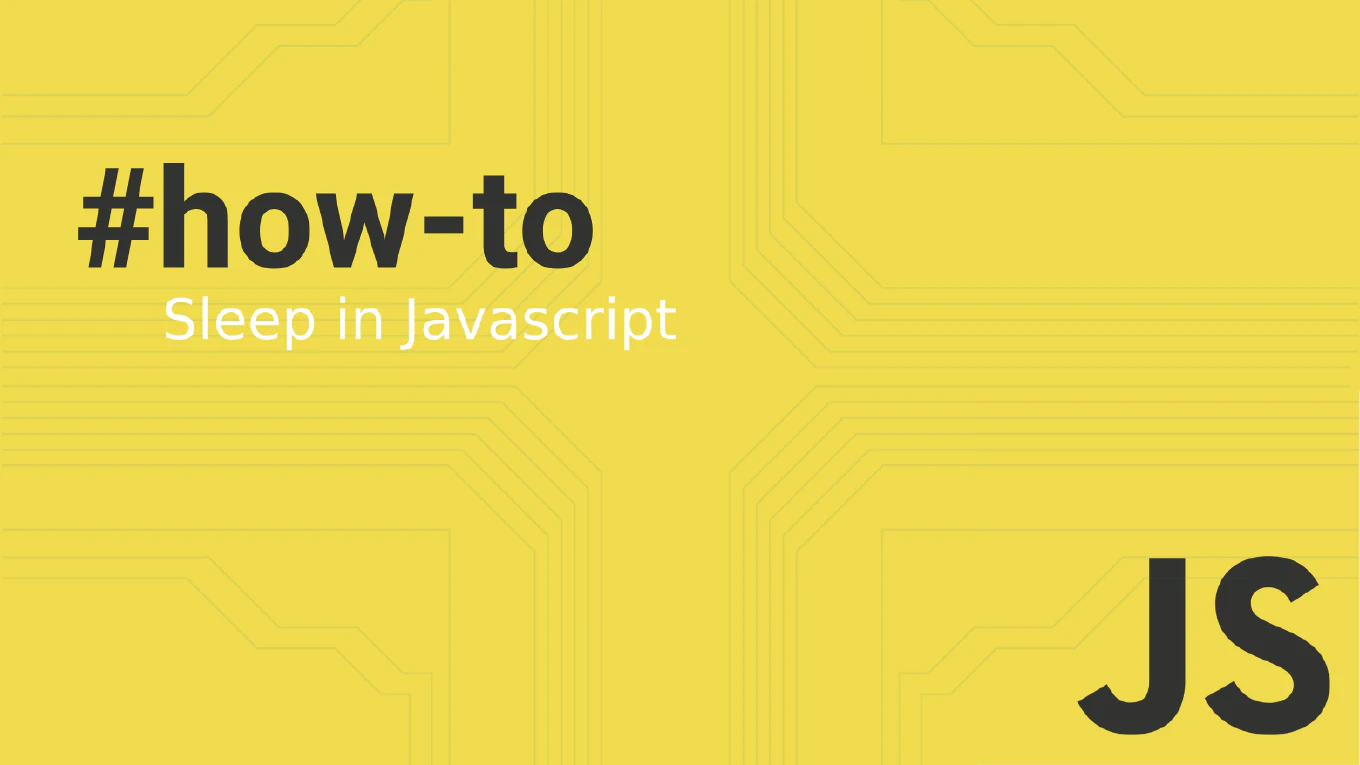
In the realm of programming, sometimes there’s a need to pause the execution of code for a fixed amount of time. While many programming languages come with a built-in sleep function to handle this, JavaScript requires a slightly different approach. Let’s explore the concept of the JavaScript sleep function, including how to implement it using setTimeout
, promises, and async/await
syntax.
Speed up your responsive apps and websites with fully-featured, ready-to-use open-source admin panel templates—free to use and built for efficiency.
Introducing Delays with setTimeout
JavaScript’s setTimeout
function is the cornerstone of introducing delays. It schedules code to be executed after a specified delay, but it does not pause the rest of the code from running. Here’s a basic example:
console.log('Hello')
setTimeout(() => { console.log('World!') }, 2000)
This code will immediately log “Hello” and then “World!” after a 2-second delay. However, if you have code following setTimeout
, it will execute without waiting for the timeout to complete, potentially leading to unexpected behavior.
The JavaScript Version of sleep()
Unlike languages like Java or Python that have a time.sleep method directly available, JavaScript uses the setTimeout
function to delay execution. However, setTimeout
is an asynchronous function, meaning the rest of your code continues to execute without waiting for the timeout to complete. This is where the concept of creating a JavaScript sleep function comes in handy.
Function sleep: Creating a Pause
To simulate a sleep function in JavaScript, we can create a function called sleep
that returns a Promise
resolved with setTimeout
. Here’s how you can define it:
function sleep(ms) {
return new Promise(resolve => setTimeout(resolve, ms))
}
This function takes a time period in milliseconds as an argument and uses setTimeout
to delay the resolution of the Promise
. This method allows us to pause execution for a specified amount of time.
Async Function: Utilizing the sleep Function
To effectively use the sleep
function, we employ async
and await
. Here’s an example:
async function delayedGreeting() {
console.log('Hello')
await sleep(2000) // Pause for 2 seconds
console.log('World!')
}
In this code snippet, await sleep(2000);
pauses the execution of our async function
for two seconds. The await
operator is used to wait for the sleep
promise to settle, making the function execution asynchronous.
One-liner Sleep: Quick Inline Delays
For scenarios where a quick, one-time delay is needed, a one-liner version of the sleep function proves invaluable:
await new Promise(resolve => setTimeout(resolve, 1000))
This line pauses the execution of an async function for 1 second and is particularly useful for inline delays without the need for a defined function.
Understanding Asynchronous Code
JavaScript is non-blocking, meaning it doesn’t stop executing code when it hits an asynchronous function like setTimeout
. Instead, it uses callback functions to execute code after a certain event or time delay. The sleep
method we defined uses this asynchronous model to create a delay without blocking the execution of other code.
An anonymous function or an anonymous callback function can be passed to setTimeout
as shown in the sleep
function example. This flexibility allows JavaScript to handle asynchronous operations effectively, without pausing the entire script.
Await Sleep: Pausing Current Async Function
It’s crucial to note that await
only pauses the current async function where it’s used. This means the rest of your JavaScript code outside the async function continues executing. This selective pausing helps manage delays in specific parts of your code without affecting the overall responsiveness of your application.
Best Practices and Considerations
- Increasing Timeouts: Sometimes, you might want to execute a series of actions with increasing delays. This can be achieved by carefully managing the timeout values in subsequent
setTimeout
calls. - Avoid Blocking: Using
async/await
with thesleep
function helps avoid blocking your JavaScript code, maintaining the non-blocking nature of web applications. - Error Handling: When creating promises, always consider potential errors. In the case of the
sleep
function, errors are less of a concern as we’re only dealing with time delays, but it’s a good practice in general.
Conclusion
Creating a JavaScript version of the sleep
function using setTimeout
, promises, and async/await
offers a powerful way to introduce delays in your code. This method respects JavaScript’s asynchronous nature, allowing you to write more readable and efficient code. Whether you’re managing API calls, animations, or simply need to pause execution, understanding how to implement and use a sleep function in JavaScript can significantly enhance your coding toolkit.
Frequently Asked Questions
1. Why doesn’t JavaScript have a built-in sleep function?
JavaScript is designed to be non-blocking to keep the user interface interactive. A built-in sleep()
function would halt the execution of the script, potentially freezing the web page. This is why JavaScript opts for asynchronous functions like setTimeout()
to manage delays without blocking the code execution.
2. Can I use the sleep function in a loop?
Yes, you can use the sleep
function within a loop, especially if you’re working with async/await
. For instance, to print numbers 1 to 5 with a delay, you could do something like this:
async function delayedNumbers() {
for (let i = 1; i <= 5; i++) {
await sleep(1000) // Wait for 1 second
console.log(i)
}
}
This makes sure that there’s a 1-second pause before each number is printed.
3. Is it okay to use sleep in JavaScript for timing animations?
While you can use the sleep
function for animations, it’s generally better to use built-in web APIs designed for animations, like requestAnimationFrame
. This is because requestAnimationFrame
is optimized for browser animations and will pause when the user navigates to a different browser tab, making it more efficient than sleep
.
4. How does the sleep function work with Promises?
The sleep
function works by returning a Promise
that resolves after a certain delay, set with setTimeout()
. The await
keyword is used to pause the execution until the promise resolves, effectively creating a delay. This doesn’t block the entire program but only pauses the async function in which await
is called.
5. Can sleep be used in synchronous functions?
No, the sleep
function created with Promises and setTimeout
cannot be used directly in synchronous functions because it relies on await
, which is only valid in async
functions. If you try to use await
in a non-async function, you’ll encounter a syntax error.
6. Does using sleep affect the performance of my web application?
Using sleep
appropriately, especially within async functions, should not negatively impact your application’s performance significantly. However, excessive or unnecessary use of sleep
, especially with long delays, can lead to poor user experiences, as it might seem like your application is unresponsive or slow.
7. Are there alternatives to using sleep for delaying actions in JavaScript?
Yes, there are several alternatives depending on your use case:
- For animations, consider using
requestAnimationFrame
or CSS animations. - For delaying actions until a certain condition is met, you might use
setInterval
to check the condition regularly. - Libraries like RxJS offer more sophisticated ways to handle asynchronous operations and delays.
Remember, understanding your specific needs and the tools available will help you choose the best approach for introducing delays in your JavaScript code.