What is JavaScript Array.pop() Method?
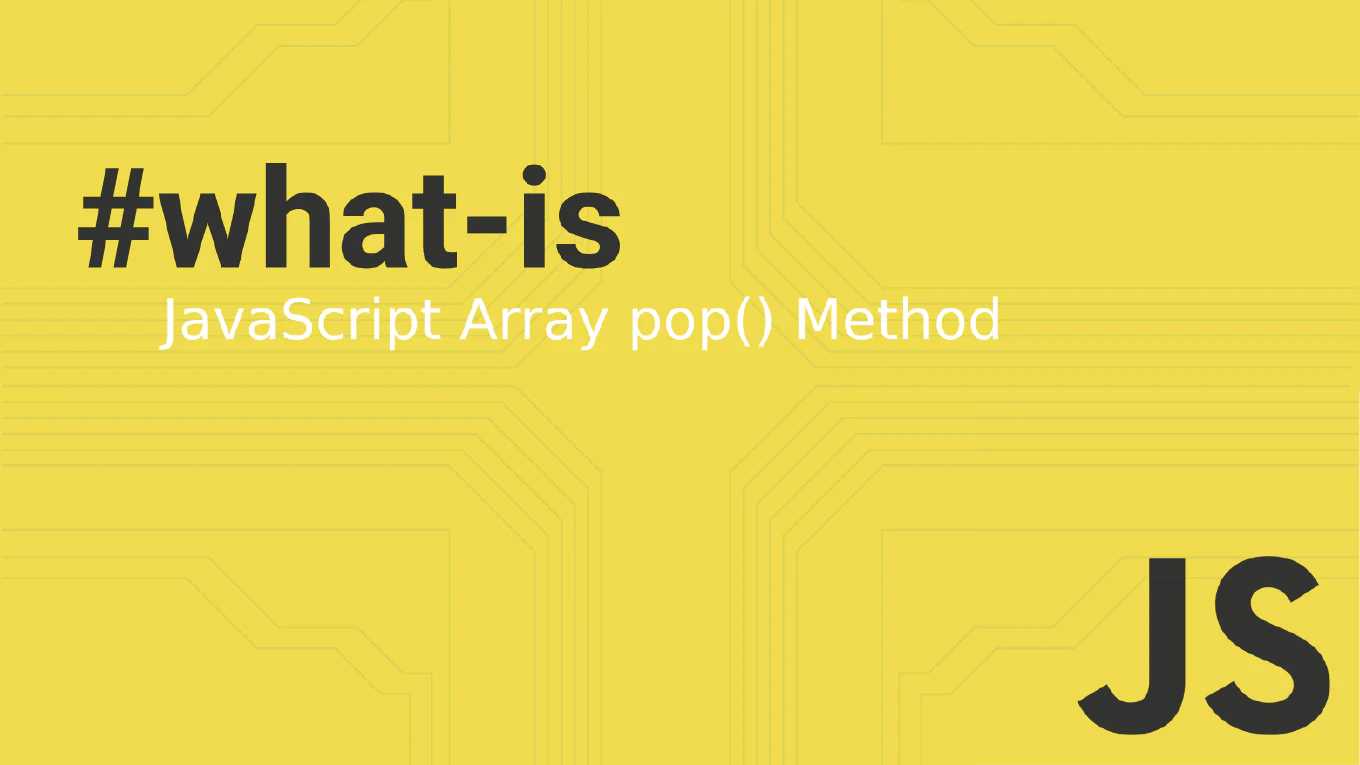
JavaScript arrays are akin to a Swiss Army knife for developers, offering many methods to create and manipulate data efficiently. Among these tools, the pop()
method is a straightforward yet powerful function that beginners should grasp early in their learning journey. This blog post will explain the pop() method, giving you the knowledge to use it effectively in your coding projects.
What is pop() in JavaScript?
Imagine you’re at a party, and guests leave one by one, starting with the last person who arrived. The pop()
method works similarly with JavaScript arrays; it removes the last element from an array and returns it. This action decreases the array’s length by one. If the array is empty, pop()
will return undefined
, indicating no element to remove.
Syntax of pop()
The syntax for pop()
is straightforward since it doesn’t require any parameters:
const arr = ['carrot', 'potato', 'beet']
arr.pop()
In the above code snippet, pop()
is called on arr
, removing the last element, 'beet'
, and reducing the array to ['carrot', 'potato']
.
How pop() Works
Let’s dive deeper with examples to understand how pop()
operates:
Example 1: Removing the Last Element
const vegetables = ['carrot', 'potato', 'beet']
const lastVegetable = vegetables.pop()
console.log(vegetables) // Output: ['carrot', 'potato']
console.log(lastVegetable) // Output: 'beet'
In this example, pop()
removes value 'beet'
from the vegetables
array and stores it in lastVegetable
. The vegetables
array is now shorter by one element.
Example 2: Using pop()
on an Empty Array
const emptyArray = []
const result = emptyArray.pop()
console.log(result) // Output: undefined
When pop()
is called on emptyArray
, it returns undefined
because there is no element to remove.
When to Use pop()
The pop()
method is instrumental in scenarios where you must work with data in a Last-In-First-Out (LIFO) manner. For example, it’s handy for:
- Implementing undo functionality in applications where the most recent action is reverted.
- Manage a stack of items, such as navigation history, in a web application, where the last page visited is the first to be removed from the history stack.
Considerations When Using pop()
While pop()
is a useful method, it’s important to remember that it mutates the original array. If you need to retain the original array, consider using methods that do not alter it, such as slice()
.
Conclusion
The pop()
method is a fundamental part of JavaScript’s array manipulation capabilities, perfect for removing the last element from an array. Its simplicity and utility make it an essential tool in your JavaScript toolbox. As you continue to explore JavaScript arrays, experimenting with pop()
and other array methods will enhance your ability to handle data effectively and elegantly in your projects.
Happy coding, and remember, practice is the key to mastering JavaScript!
Frequently Asked Questions (FAQs)
Does pop()
work on arrays of any type?
Yes, pop()
can be used on arrays containing elements, including strings, numbers, objects, etc. It simply removes the last element from the array, regardless of its type.
Can pop()
remove more than one element at a time?
No, pop()
is designed to remove only the last element of an array. If you need to remove multiple elements from the end, you would need to call pop()
multiple times or use a different method, such as splice()
.
What happens to the original array after using pop()
?
The original array is modified, or mutated, by pop()
. The array’s length is decreased by one, and the last element is removed. If you need to keep the original array intact, consider copying the array before using pop()
.
Is there a method to remove the first element of an array?
Yes, you can use the shift()
method to remove the first element of an array. Like pop()
, shift()
modifies the original array by removing the first element and returns the removed element.
const colors = ['red', 'green', 'blue', 'yellow']
const firstColor = colors.shift()
console.log(colors) // Output: ['green', 'blue', 'yellow']
console.log(firstColor) // Output: 'red'
How can I retrieve the last element of an array without removing it?
To access the last element of an array without modifying the array, you can use the length property to calculate the index of the last element:
const arr = ['carrot', 'potato', 'beet']
const lastElement = arr[arr.length - 1]
console.log(lastElement) // Output: 'beet'
This approach lets you “peek” at the last element without altering the array’s structure.
Are there any performance considerations with using pop()
?
pop()
is generally efficient for array operations because removing an element from the end of an array does not require reindexing the remaining elements. However, for huge arrays or performance-critical applications, it’s always a good practice to benchmark and consider other data structures if necessary.
Can you call pop()
on a string in JavaScript?
No, you cannot directly call pop()
on a string in JavaScript. Strings are not arrays, and pop()
is a method designed specifically for array manipulation. However, you can achieve a similar effect by converting the string to an array, using pop()
on the array, and then converting the array back to a string:
const str = 'Hello, World!'
const arr = str.split('') // Convert string to an array
arr.pop() // Removes the last character from the array
const newStr = arr.join('') // Converts the array back into a string
console.log(newStr) // Output: 'Hello, World'
What is the difference between push()
and pop()
in JavaScript?
The push()
method adds one or more elements to the end of an array and returns the new length of the array, whereas the pop()
method removes the last element from an array and returns that element. Essentially, push()
adds to the end, and pop()
removes from the end of an array.
What is the opposite of JavaScript pop()
?
The opposite of pop()
is the push()
method, which adds an element to the end of an array. While pop()
removes the last element and decreases the array’s length, push()
adds an element and increases the array’s length. For removing and adding elements at the beginning of an array, the shift()
and unshift()
methods are used, respectively.