Understanding the difference between `for...in` and `for...of` statements in JavaScript
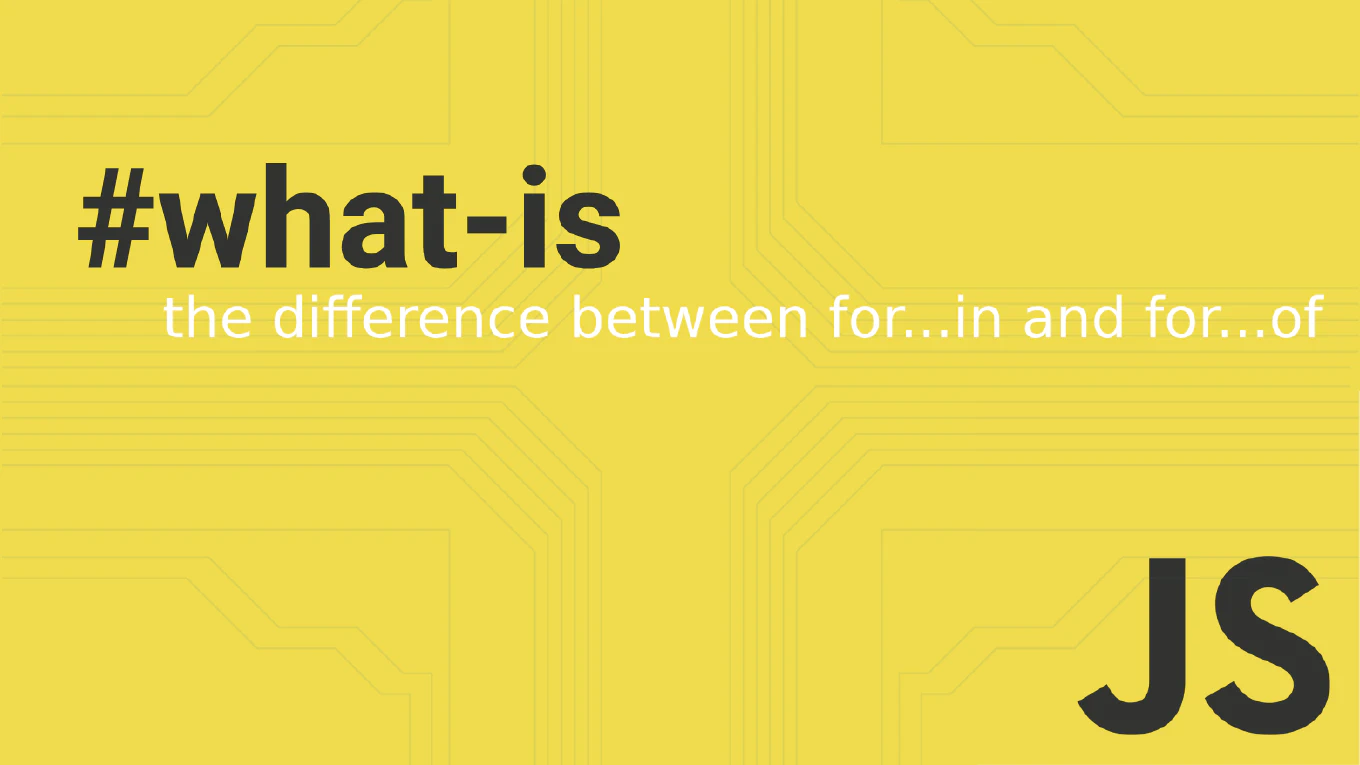
As JavaScript continues to evolve, understanding the nuances of its looping mechanisms becomes essential for developers aiming to write efficient and bug-free code. Two commonly used looping statements, for...in
and for...of
, often lead to confusion due to their overlapping use cases. Grasping the difference between these loops is crucial for iterating over objects and iterable objects effectively, ensuring optimal performance and maintainability in your codebase.
Speed up your responsive apps and websites with fully-featured, ready-to-use open-source admin panel templates—free to use and built for efficiency.
Introduction
In the realm of JavaScript, looping constructs are fundamental for traversing data structures such as arrays and objects. However, choosing the wrong loop can result in unexpected behavior, especially when dealing with object properties and iterable objects. This article delves into the differences between the for...in
and for...of
statements, providing experienced frontend developers with the insights needed to make informed decisions in their coding practices.
Key Concepts and Terminology
Before diving into the differences, it’s essential to understand the core concepts:
- Objects and Properties: Objects in JavaScript are collections of key-value pairs, where keys are strings (or symbols) representing property names.
- Enumerable Properties: These are properties of an object that can be iterated over, typically those defined directly on the object rather than inherited through the prototype chain.
- Iterable Objects: Objects that implement the iterable protocol, allowing them to be iterated over using constructs like
for...of
. Common iterable objects include arrays, strings, and Maps. - Data Structures: Structures like arrays and objects that store collections of data, each with unique methods and properties for data manipulation.
for...in
Loop
The for...in
loop is designed to iterate over the property keys of an object. It’s particularly useful when you need to access all enumerable properties, including those inherited through the prototype chain.
Best Practices:
- Use
for...in
when iterating over object properties. - Always check for
hasOwnProperty
to ensure you’re accessing the object’s own properties, avoiding inherited ones.
Example:
const user = {
name: 'Alice',
age: 30,
profession: 'Developer'
}
for (const key in user) {
if (user.hasOwnProperty(key)) {
console.log(key, user[key])
}
}
Potential Pitfalls:
- Iterating over prototype properties can lead to unexpected behavior if
hasOwnProperty
checks are omitted. - Not suitable for iterating over array elements, as it iterates over property keys, not values.
for...of
Loop
Introduced in ES6, the for...of
loop is tailored for iterating over iterable objects, providing direct access to their values. This loop is ideal for arrays, strings, Maps, Sets, and other built-in iterables.
Best Practices:
- Use
for...of
when you need to iterate over the values of an iterable object. - It’s more readable and concise when dealing with array elements compared to traditional
for
loops.
Example:
const fruits = ['apple', 'banana', 'cherry']
for (const fruit of fruits) {
console.log(fruit)
}
Potential Pitfalls:
- Cannot be used to iterate over non-iterable objects like plain objects.
- Does not provide access to the index or key directly; for that, consider using
entries()
or other array methods.
When to Use Each Loop
-
Use
for...in
when:- Iterating over the keys of an object.
- Accessing all enumerable properties, including those from the prototype chain (with appropriate checks).
-
Use
for...of
when:- Iterating over the values of an iterable object.
- Working with arrays, strings, or other iterable data structures where the value is the primary focus.
Case Study: Choosing the Right Loop
Consider a scenario where you’re handling user data stored in an object versus a list of user names in an array.
Using for...in
for Object Properties:
const userData = {
id: 1,
name: 'Bob',
role: 'Admin'
}
for (const key in userData) {
if (userData.hasOwnProperty(key)) {
console.log(`${key}: ${userData[key]}`)
}
}
Using for...of
for Array Elements:
const userNames = ['Alice', 'Bob', 'Charlie']
for (const name of userNames) {
console.log(name)
}
In this case, using for...in
for the userData
object ensures that all property keys are accessed correctly, while for...of
efficiently iterates over each name in the userNames
array.
Conclusion
Understanding the distinction between for...in
and for...of
statements is vital for writing clear and efficient JavaScript code. While for...in
excels at iterating over object properties, for...of
is the go-to choice for iterable objects like arrays. By selecting the appropriate loop, developers can enhance code readability, prevent bugs related to unexpected property iterations, and leverage JavaScript’s powerful data handling capabilities.
Key Takeaways
for...in
iterates over an object’s property keys, including inherited enumerable properties.for...of
iterates over the values of iterable objects like arrays and strings.- Always use
hasOwnProperty
withfor...in
to avoid unexpected inherited properties. - Choose the loop type based on whether you need keys or values from your data structure.
Recommended Resources
- CoreUI Blog – Comprehensive guides and best practices for frontend development.
- MDN Web Docs on
for...in
– Official documentation and examples. - JavaScript.info on Iteration – In-depth tutorials on loops and iterable objects.