How to change opacity on hover in CSS
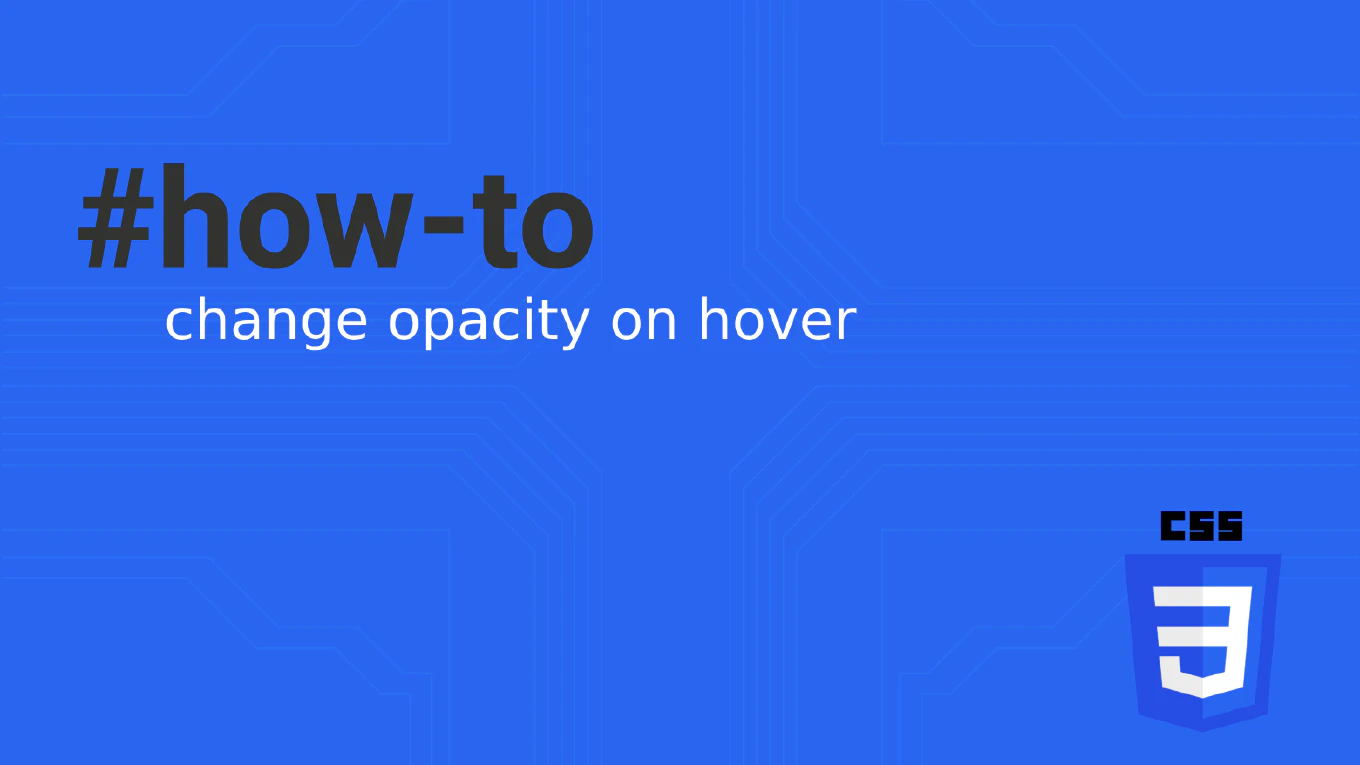
Changing the opacity of images on hover is a common effect used in web design to create dynamic and interactive user interfaces. This article will walk you through the process of using the opacity property in CSS to achieve this effect.
Speed up your responsive apps and websites with fully-featured, ready-to-use open-source admin panel templates—free to use and built for efficiency.
Understanding the Opacity Property
The opacity
property in CSS controls the transparency level of an element, making it fully opaque (opacity: 1
) or fully transparent (opacity: 0
). When applied to an image or any element, the opacity
property affects not just the background color but also the content inside the element, making everything within the element more transparent.
Syntax Example
.image-class {
opacity: 0.5;
}
This code will make any element with the class .image-class
50% transparent.
Implementing Image Opacity on Hover
To change the image opacity when a user hovers over it, you can use the hover
pseudo class in CSS. This pseudo class allows you to apply a different style when the user interacts with the element by placing their mouse over it.
Basic Example
Here’s a simple example of how to change an image’s opacity on hover:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<style>
.image-class {
opacity: 1;
transition: opacity 0.3s;
}
.image-class:hover {
opacity: 0.5;
}
</style>
</head>
<body>
<img src="example.jpg" alt="example image" class="image-class">
</body>
</html>
In this example, the image starts fully opaque (opacity: 1
). When the user hovers over the image, its opacity decreases to 50% (opacity: 0.5
), thanks to the transition
property, creating a smooth fade effect.
Image Hover with Child Elements
When working with more complex layouts, such as an image container with child elements, you might want to apply the hover effect only to the image while keeping the child elements unaffected. Here’s how you can do it:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<style>
.image-container {
position: relative;
}
.image-class {
opacity: 1;
transition: opacity 0.3s;
}
.image-container:hover .image-class {
opacity: 0.3;
}
.child-elements {
position: absolute;
top: 0;
left: 0;
color: white;
}
</style>
</head>
<body>
<div class="image-container">
<img src="example.jpg" alt="example image" class="image-class">
<div class="child-elements">Overlay Text</div>
</div>
</body>
</html>
In this setup, the image-class
will have its opacity adjusted when the image-container
is hovered over, while the child-elements
remain fully visible and unaffected by the opacity change.
Advanced Techniques: Using RGBA and HSLA for Background Opacity
Sometimes, you may want to change the background opacity without affecting the text or other elements inside. This can be achieved using rgba
or hsla
color values, which include an alpha parameter to set the background’s transparency.
Example Using RGBA
.image-container {
background-color: rgba(0, 0, 255, 0.5); /* Blue background with 50% opacity */
}
.image-container:hover {
background-color: rgba(0, 0, 255, 0.3); /* Background becomes more transparent */
}
This example changes the background color’s opacity on hover while leaving the content inside fully opaque.
Enhancing the Hover Effect with Transitions
To make the hover effect more visually appealing, consider using the transition
property to create a fade effect. The transition
property allows you to define the timing and easing function of the change, giving your website a more polished look.
Example with Transition
.image-class {
opacity: 1;
transition: opacity 0.5s ease-in-out;
}
.image-class:hover {
opacity: 0.2;
}
This code creates a smooth transition effect when changing the image’s opacity, enhancing the user experience.
Conclusion
Using the opacity property with the hover pseudo class is a powerful way to create interactive and visually appealing effects on your website. Whether you’re changing the opacity of an image or adjusting the background opacity using rgba color values, CSS provides a simple yet effective means to enhance your web design.
Incorporate these techniques into your projects to create responsive and engaging user interfaces that capture your audience’s attention.