How to check if a key exists in JavaScript object?
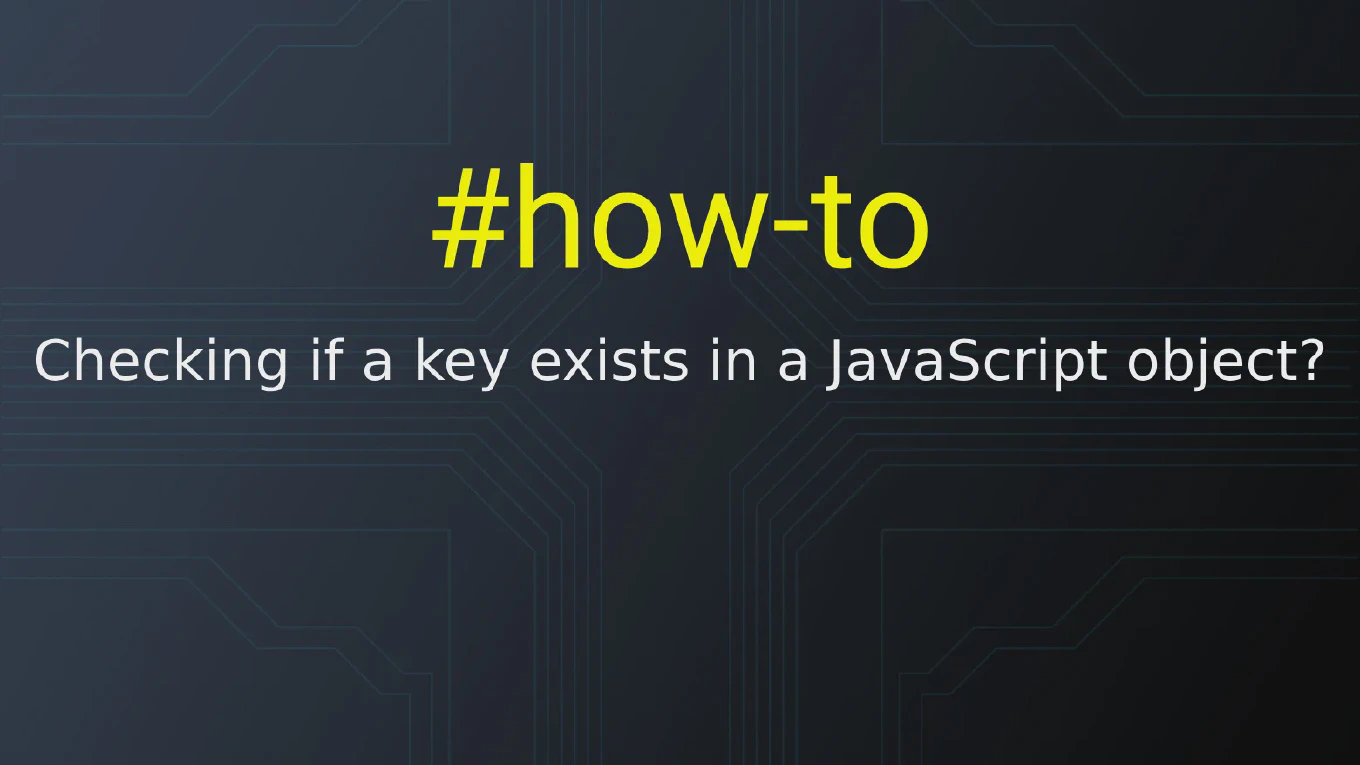
In the intricate world of JavaScript, a common task for developers is determining whether a specific key exists within an object. It’s an elementary, yet crucial skill that can significantly affect the flow and reliability of your code. In this comprehensive guide, we’ll unearth the various methods to perform this check, unravel their nuances, and understand how to apply them, even in complex nested structures. By the end of this article, you’ll be equipped with the expertise to write robust and error-free JavaScript applications.
The in
Operator: A Preliminary Check
One of the most straightforward methods to check for a key’s existence in a JavaScript object is by utilizing the in
operator. This operator works seamlessly, returning a boolean — true
if the key is found and false
otherwise. Let’s consider an illustrative example:
const person = {
name: 'John',
surname: 'Doe',
age: 23,
email: '[email protected]'
};
console.log('name' in person); // Returns true
console.log('address' in person); // Returns false
Here, we have a person
object with four keys: name
, surname
, age
, and email
. To verify the presence of the name key, we simply place the in
operator before the object and the key in question. The response is immediate and transparent: true
indicates the key exists; if not, we get false
. It’s efficient and effective for quick checks.
Harnessing hasOwnProperty
Move a notch up, and you’ll encounter the hasOwnProperty
method. This powerful method is a part of every JavaScript object’s prototype and serves a specific purpose: to check whether the key is a direct, own property of the object, as opposed to being inherited through the prototype chain.
const person = {
name: 'John',
surname: 'Doe',
age: 23,
email: '[email protected]'
};
console.log(person.hasOwnProperty('name')); // Returns true
console.log(person.hasOwnProperty('address')); // Returns false
Utilizing hasOwnProperty
, we make a direct call on the object, passing the key as an argument. It ferrets out whether the key is an innate property of the object itself — a true testament to its presence.
A Concise Alternative: Comparison with undefined
In certain scenarios, where brevity is key, you may opt for a more succinct approach — directly comparing the key’s value to undefined
. This particular method is not only brief but remarkably expressive.
const person = {
name: 'John',
surname: 'Doe',
age: 23,
email: '[email protected]'
};
console.log(person.name !== undefined); // Returns true
console.log(person.address !== undefined); // Returns false
Above, we’ve straightforwardly checked if the value of the name
key diverges from undefined
. If it does, it’s a resounding yes — the key is part of the object.
Performance
For performance comparison between the methods that are in, hasOwnProperty
and key is undefined
, see this benchmark:
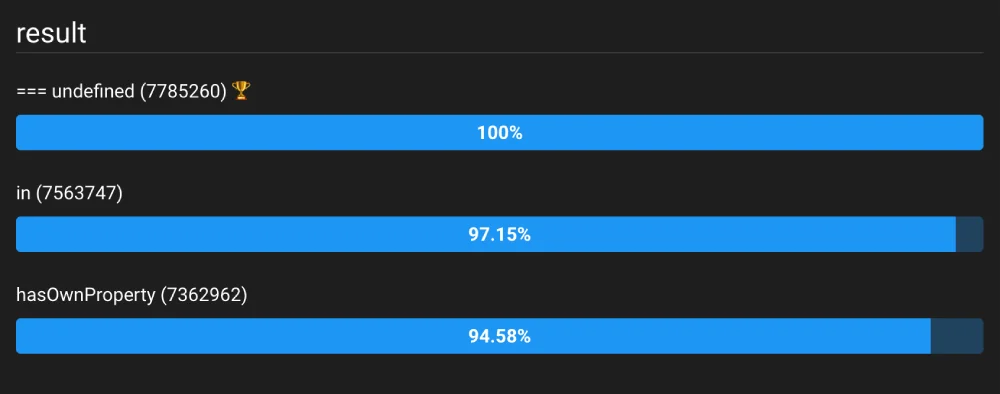
Navigating Nested Objects
The complication arises when objects aren’t mere flat structures but nested ones. Checking keys in such cases requires a mix of techniques employing the in
operator, hasOwnProperty
method.
const person = {
name: 'John',
surname: 'Doe',
age: 23,
email: '[email protected]',
address: {
country: 'Poland',
street: 'Krzywa 12',
city: 'Katowice'
}
};
console.log('address' in person && 'country' in person.address); // Returns true
console.log(person.hasOwnProperty('address') && person.address.hasOwnProperty('country')); // Returns true
console.log(person.address.country !== undefined); // Returns true
In this dense jungle of nested objects, we first validate the existence of the address
key within the person object. Post confirmation, we delve deeper to check for the country
key within the address
object. Whether you choose the in
operator, the hasOwnProperty
method or optional chaining, the end goal is to ensure that each step of the property chain is solidly established.
Summary
In this definitive guide, we’ve walked through the multiple pathways to verify if keys exist in both flat and nested JavaScript objects. Here are the key takeaways:
- Use the
in
operator for a swift, surface-level check. - Deploy the
hasOwnProperty
method for a more precise confirmation, ensuring the key is not inherited. - Opt for direct comparison with
undefined
when the shortest syntax is paramount. - For nested objects, combine these techniques with additional logical checks or optional chaining.
Remember, choosing the most apt method hinges on your specific use case and how your object is structured. Effective utilization of these techniques will lead to improved code stability and a better grasp of JavaScript’s capabilities. Now, with this knowledge at your disposal, embrace the art of detection and may your coding endeavors be ever-fructifying.
Happy coding!
Are you looking to further bolster your JavaScript acumen? Here are some queries you might find intriguing:
- How to check that object has keys in JS?
- How to check if key exists in map object JavaScript?
- How to check if a key is present in JSON object in JavaScript?
- How to check if a key exists in an array JavaScript?
We will cover this topics in future posts.