How to check if an array is empty in JavaScript?
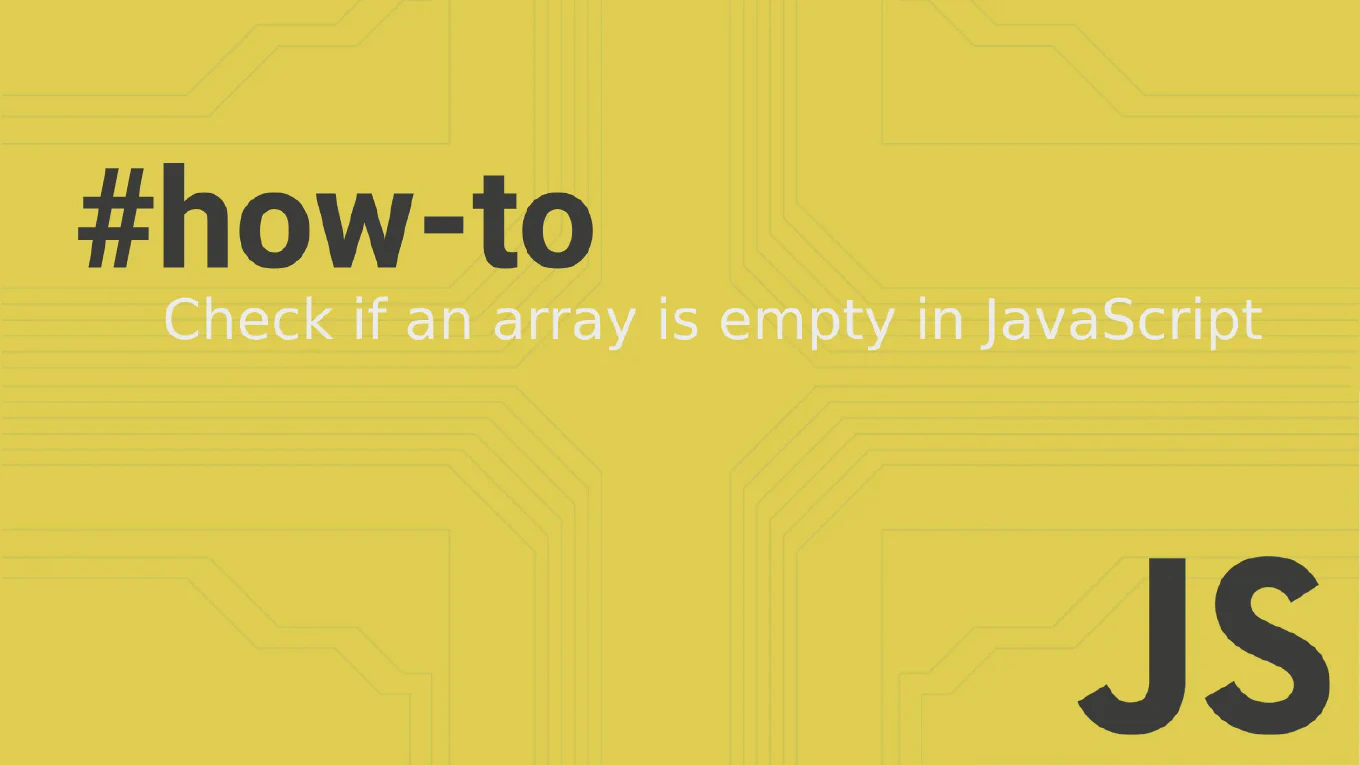
In the ever-evolving world of web development, JavaScript stands as a cornerstone, powering dynamic and interactive elements across countless websites and applications. Among its many tasks, one seemingly straightforward yet pivotal operation is determining whether an array is empty. This operation is crucial in various scenarios, such as enabling or disabling buttons based on user input or ensuring data integrity before processing. In this article, part of the “how to” series, we delve into the intricacies of checking for empty arrays in JavaScript. This guide promises to enlighten those new to the programming world and provide valuable insights for seasoned developers.
Method 1: The Length Property
The most straightforward way to check if an array is empty is by using the length
property. If the length is 0
, the array is empty.
const array = [];
if (array.length === 0) {
console.log('The array is empty.')
} else {
console.log('The array is not empty.')
}
Method 2: Using Array.isArray() with Length
While Array.isArray()
alone does not check for emptiness, combining it with a check for length ensures that not only is the variable an array, but it’s also empty. This is a more robust check when you’re not certain of the variable’s type.
const array = [];
if (Array.isArray(array) && array.length === 0) {
console.log('The array is empty.')
} else {
console.log('The array is not empty or not an array.')
}
Method 3: Using isEmpty()
Function to Check if an Array is Empty
Consider the following code snippet as an introduction:
function isEmpty(array) {
return Array.isArray(array) && array.length === 0
}
This function, isEmpty
, concisely encapsulates the logic needed to determine if an array is empty. Let’s dissect its workings step by step:
-
Array Validation: The function utilizes
Array.isArray()
to ascertain if the provided input (array
) is indeed an array. This is crucial because other types (like objects or strings) might also possess alength
property, which could lead to misleading outcomes if not properly checked. -
Length Check: The function examines the
length
property once confirmed as an array. An empty array will have a length of 0, thus fulfilling our criteria for being “empty.”
Example in Action
To illustrate the function’s practicality, let’s apply it to various arrays:
console.log(isEmpty([])) // Output: true
console.log(isEmpty([1, 2, 3])) // Output: false
console.log(isEmpty('')) // Output: false
console.log(isEmpty({})) // Output: false
The isEmpty
function is a clean and effective way to verify that a given input is an empty array, making it a handy tool in various programming contexts where such a check is necessary.
The Importance of Handling Empty Arrays
Empty arrays might seem trivial at first glance. However, their proper handling can significantly impact web application functionality and user experience. Imagine a scenario where a form submission button remains disabled because the script incorrectly assumes that the input field (captured as an array) contains data. Minor oversights can frustrate users and detract from the application’s overall quality.
Understanding the Array.isArray()
Method
At the heart of our exploration is the Array.isArray() method, a reliable way to confirm whether a given variable is an array. This method effectively bypasses potential confusion with null, undefined, and non-array variables, ensuring our scripts are robust and error-free.
Why Not Just Use the length
Property?
An astute question arises: why not rely solely on the length
property to determine if an array is empty? The answer lies in JavaScript’s flexibility. Since various data types could technically have a length
property, we must first ensure we deal with an array to avoid false positives or negatives.
The length
Property on Strings
const string = 'Hello World'
console.log(string.length) // Output: 11
console.log(Array.isArray(string)) // Output: false
When used on a string, the length
property returns the number of characters in the string. In this example, 'Hello World'
contains 11 characters, so string.length
outputs 11
. This straightforward use case shows the direct application of the length
property on a string, which is exactly what you typically need for strings.
Why This Matters
In discussions about the utility of the length
property, the distinction between strings and arrays (and other array-like objects) is significant because:
-
Different Types, Same Property: Both strings and arrays have a
length
property, but the semantics around them might lead to different expectations and checks in your code. For example, while you might use length to find out how many characters a string has, for arrays, you might be interested in the number of elements, which could include undefined values or holes in sparse arrays. -
Understanding Your Data: Knowing whether you’re dealing with a string, an array, or another array-like object (e.g.,
NodeList
,arguments
object) affects how you might want to use thelength
property. For instance, if you’re manipulating collections of elements (like DOM elements in aNodeList
), knowing that you’re not working with a true array can influence how you iterate over those elements or convert them into a true array for further operations. -
Beyond Length: The example subtly hints at a broader principle in JavaScript programming: understanding the type and structure of your data is as important as knowing the properties and methods available to you. While length is a universally useful property, how and why you use it can vary greatly depending on the context—ranging from simple size checks to more complex logic that involves type-checking, filtering, and mapping over data structures.
How don’t check if an array is empty in JavaScript?
JavaScript offers a wide range of methods to check if an array is empty, ranging from straightforward to the more convoluted or “stupid” ways that are more about showcasing creativity or the quirks of the language rather than practicality. Here are some less conventional (not advisable) ways to check if an array is empty. Please don’t use them in your projects.
-
The Array.length Property with a Logical NOT Operator. A concise way to check for an empty array is by using the logical NOT (
!
) operator twice before the array’slength
property This converts the length to a boolean where0
(empty array) becomestrue
.const isEmpty = array => !!array.length === false
-
Using JSON.stringify: Convert the array to a JSON string and compare it with the string representation of an empty array.
const isEmpty = array => JSON.stringify(array) === '[]'
-
Using a combination of map and reduce: Try to sum the lengths of elements after mapping, which makes no sense for checking emptiness but works if you just want to do it roundaboutly.
const isEmpty = array => array.map(item => 1).reduce((a, b) => a + b, 0) === 0
-
Employing Array.prototype.join: Convert the array to a string using a delimiter and check if the resulting string is empty.
const isEmpty = array => array.join(',') === ''
-
Using Array.prototype.filter: Filter the array based on a condition that’s always false and check the length.
const isEmpty = array => array.filter(() => false).length === 0
-
Serialize to JSON and back: Convert the array to JSON and then parse it to an array, checking if the length is zero.
const isEmpty = array => JSON.parse(JSON.stringify(array)).length === 0
-
Checking the array’s toString method: This is an indirect way to see if the array is empty.
const isEmpty = array => array.toString() === ''
-
Exploiting the spread operator with Math.min or Math.max: Since
Math.min()
/Math.max()
returns Infinity/-Infinity when called with no arguments, this method exploits that fact in a nonsensical way to check for an empty array.const isEmpty = array => Math.min(...array) === Infinity
-
Using a custom reducer to “calculate” emptiness: This is an overly complicated way to see if an array has elements.
const isEmpty = array => array.reduce((acc, value) => acc + 1, 0) === 0
-
Detecting array emptiness by trying to access an undefined property: This uses the fact that accessing an index that doesn’t exist in an array returns
undefined
.const isEmpty = array => array[0] === undefined && array.length === 0
-
Invoke a function with array spread and check arguments length: Use a function call with spread syntax to check if any arguments were passed.
const isEmpty = array => ((...args) => args.length === 0)(...array)
Conclusion and Takeaways
Mastering array manipulation, including accurately identifying empty arrays, is a foundational skill in JavaScript programming. The Array.isArray()
method, paired with a check on the length
property, offers a reliable and efficient solution to this common task. As we’ve seen, such seemingly minor details can significantly affect web applications’ functionality and user experience.
For new and experienced developers, understanding these nuances is critical to crafting robust, error-free code. Remember, the journey through JavaScript is continuous, with each tutorial and guide enhancing your skills and knowledge.
Remember, every line of code is a step toward mastery. Happy coding!