How to check if an element is visible in JavaScript
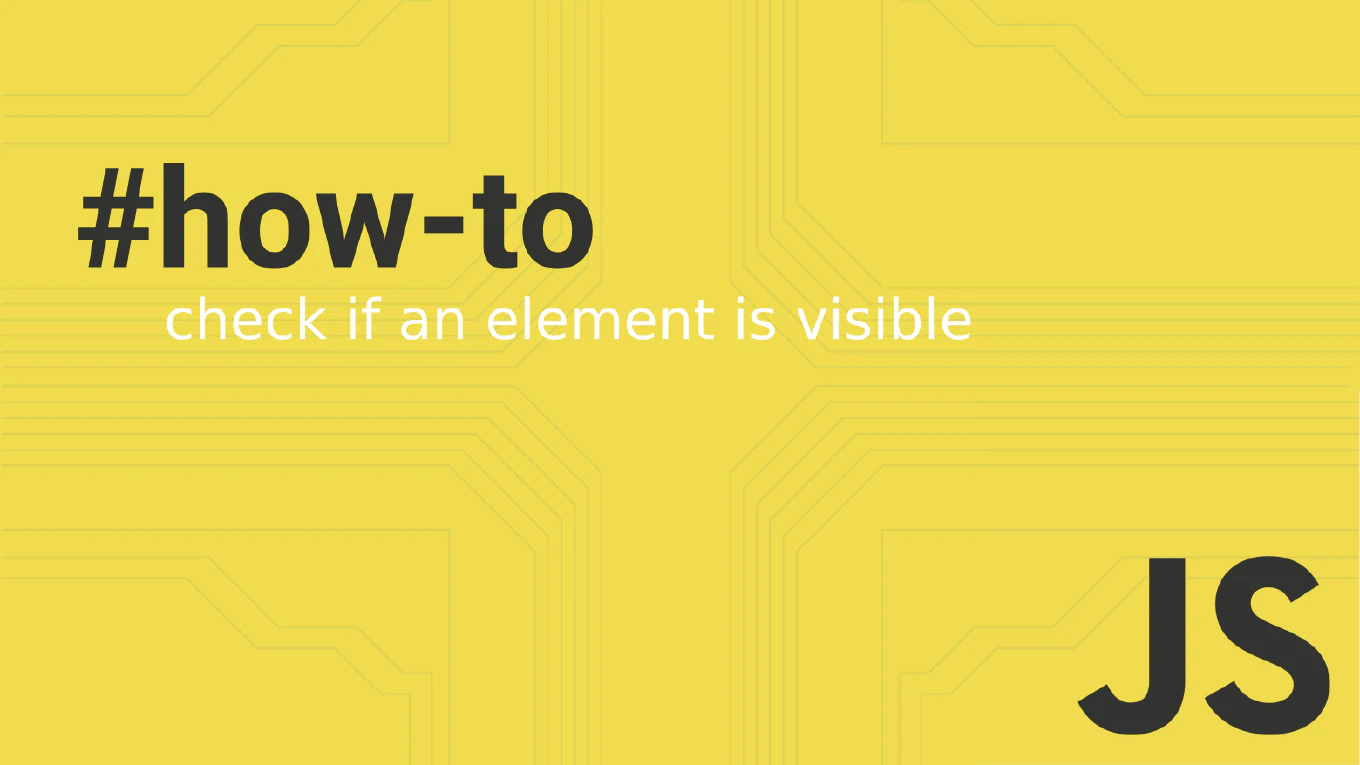
In JavaScript, determining if a DOM element is visible is crucial for tasks like lazy-loading images, triggering animations, and enhancing accessibility. This article covers two main contexts for checking visibility: general visibility (whether the element is rendered and visible based on CSS properties) and viewport visibility (whether the element is visible within the user’s current view). We’ll also delve into the checkVisibility()
method, including its options and limitations, and its browser support. Additionally, we’ll discuss how these techniques are applied in real-world components, such as carousels in popular UI frameworks.
Speed up your responsive apps and websites with fully-featured, ready-to-use open-source admin panel templates—free to use and built for efficiency.
General Visibility of an Element
General visibility refers to whether an element is rendered on the page and visible based on its CSS properties. This includes properties like display
, visibility
, and opacity
.
Using the checkVisibility()
Method
The checkVisibility()
method is a powerful API feature introduced in modern browsers that simplifies the process of determining if an element is visible. It evaluates whether an element is part of the document layout, isn’t hidden by any ancestor elements, and considers CSS properties like visibility
and opacity
.
const isVisible = element.checkVisibility()
Important Note
The checkVisibility()
method became widely available across the latest devices and browser versions starting in March 2024. However, this visibility function might not work in older devices or browsers. If your project needs to support older environments, it’s crucial to implement fallback methods to ensure compatibility.
Understanding Optional Options in checkVisibility()
The checkVisibility()
method includes an optional parameter that allows for more granular control over what is considered “visible.” This can be particularly useful in scenarios where elements might be technically visible but partially obscured or affected by specific CSS properties.
Available Options
-
checkOpacity
: This option allows you to determine if an element’s visibility is affected by itsopacity
. If set totrue
, the function will returnfalse
for elements withopacity: 0
.const isVisible = element.checkVisibility({ checkOpacity: true })
-
checkVisibilityCSS
: This option checks whether thevisibility
CSS property is set tohidden
. If set totrue
, the function will returnfalse
if the element or any ancestor element hasvisibility: hidden
.const isVisible = element.checkVisibility({ checkVisibilityCSS: true })
-
checkDisplayNone
: This option checks if thedisplay
property is set tonone
. If set totrue
, the function will returnfalse
for elements withdisplay: none
.const isVisible = element.checkVisibility({ checkDisplayNone: true })
-
checkContentVisibility
: This option checks thecontent-visibility
property, which can affect whether content within an element is rendered or not. If the content is not rendered,checkVisibility()
will returnfalse
.const isVisible = element.checkVisibility({ checkContentVisibility: true })
These options provide fine-tuned control, allowing developers to determine visibility under various conditions. For example, if you want to ensure that an element is considered visible only if it has both opacity
greater than 0 and is not set to visibility: hidden
, you can pass these options to the checkVisibility()
method.
const isVisible = element.checkVisibility({
checkOpacity: true,
checkVisibilityCSS: true
})
Checking General Visibility with CSS Properties
Before the checkVisibility()
method, developers often checked specific CSS properties to determine if an element was visible:
display: none
: The element is removed from the layout and is not visible.visibility: hidden
: The element is hidden but still occupies space in the layout.opacity: 0
: The element is fully transparent, making it invisible but still interactive.
Here’s how you can manually check these properties:
const isGenerallyVisible = (element) => {
const style = window.getComputedStyle(element)
return style.display !== 'none' && style.visibility !== 'hidden' && style.opacity !== '0'
}
This visibility function checks if an element is visible on the page according to these CSS properties.
Visibility Within the Viewport
Visibility within the viewport refers to whether an element is visible within the user’s current screen area, which is essential for tasks like lazy-loading content or triggering animations.
Using getBoundingClientRect()
A common method to check if an element is visible within the viewport is by using getBoundingClientRect()
. This method returns a DOMRect
object that provides information about the element’s dimensions and position relative to the viewport.
const isVisibleInViewport = (element) => {
const rect = element.getBoundingClientRect()
return (
rect.top >= 0 &&
rect.left >= 0 &&
rect.bottom <= (window.innerHeight || document.documentElement.clientHeight) &&
rect.right <= (window.innerWidth || document.documentElement.clientWidth)
)
}
This function returns true
if the entire element is within the viewport’s dimensions, ensuring that the element is visible on the screen.
Using the Intersection Observer API
For more complex scenarios, the Intersection Observer API allows you to observe changes in the visibility of an element relative to an ancestor element or the top-level document’s viewport.
const observer = new IntersectionObserver((entries) => {
entries.forEach(entry => {
if (entry.isIntersecting) {
console.log('Element is in viewport')
} else {
console.log('Element is not in viewport')
}
})
})
observer.observe(document.querySelector('#myElement'))
This API is particularly useful for dynamic content loading, such as infinite scrolling or lazy-loading images.
Real-World Example: Carousels
In practical web development, these visibility techniques are often used in UI components, such as carousels, to determine if they are within the viewport. For instance, in the React Carousel Component, Vue Carousel Component, and Angular Carousel Component, visibility checks are used to start animations only when the carousel is visible within the viewport. This ensures that resources are not wasted on off-screen elements and improves the user experience by triggering animations at the right time.
Combining Methods for Comprehensive Visibility Checks
In some cases, it’s important to combine general visibility checks with viewport visibility checks to ensure an element is fully visible to the user:
const isElementFullyVisible = (element) => {
return isGenerallyVisible(element) && isVisibleInViewport(element) && element.checkVisibility()
}
This combined approach ensures that the element is visible within the viewport and fully visible on the page, considering all relevant factors.
Conclusion
Understanding and correctly implementing visibility checks is essential for modern web development. The checkVisibility()
method offers a powerful, integrated solution for general visibility checks, but developers should be aware that it may not be supported on older browsers or devices. Traditional methods like getBoundingClientRect()
and the Intersection Observer API remain valuable for handling viewport visibility.
By mastering these techniques, you can create more responsive and user-friendly web applications, ensuring that your content is visible when and where it matters most. Additionally, implementing these visibility checks in components like carousels ensures optimal performance and user experience by starting animations only when the content is within view.