How to Convert a Map to an Array in JavaScript
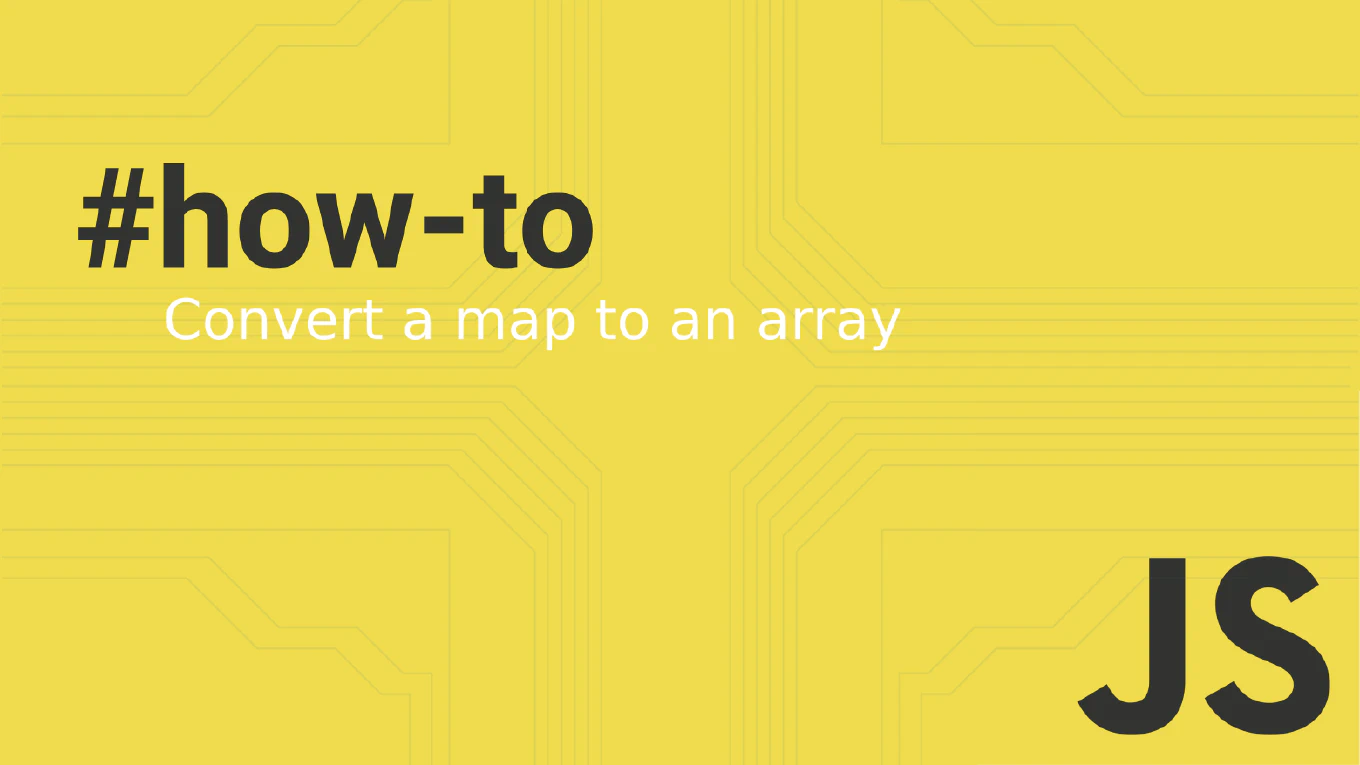
In JavaScript, the Map object is a collection of key-value pairs where each key is unique. Converting a Map to an array can be essential for data manipulation and iteration. This article explores various methods to convert a Map to an array, discussing the syntax and usage of each approach.
Using Array.from()
The Array.from()
method is a straightforward way to convert a Map to an array. It creates a new array from an iterable object, such as a Map.
const map = new Map([['key1', 'value1'], ['key2', 'value2']])
const array = Array.from(map)
console.log(array) // Output: [['key1', 'value1'], ['key2', 'value2']]
This method creates a new array containing the key-value pairs of the map as sub-arrays.
Using Spread Operator
The spread syntax (...
) can be used to expand the entries of a Map into an array.
const map = new Map([['key1', 'value1'], ['key2', 'value2']])
const array = [...map]
console.log(array) // Output: [['key1', 'value1'], ['key2', 'value2']]
This approach provides a concise way to convert a map into an array.
Converting Map Keys to an Array
To convert only the keys of a Map to an array, you can use the keys()
method combined with Array.from()
or the spread operator.
const map = new Map([['key1', 'value1'], ['key2', 'value2']])
const keysArray = Array.from(map.keys())
console.log(keysArray) // Output: ['key1', 'key2']
Using this method, you extract the keys into a new array.
Converting Map Values to an Array
Similarly, to convert only the values of a Map to an array, use the values()
method.
const map = new Map([['key1', 'value1'], ['key2', 'value2']])
const valuesArray = Array.from(map.values())
console.log(valuesArray) // Output: ['value1', 'value2']
This method helps extract the values from the map into a new array.
Converting Map to an Array of Objects
To convert a Map to an array of objects, you can use the Array.from() method with a mapping function.
const map = new Map([['key1', 'value1'], ['key2', 'value2']])
const arrayOfObjects = Array.from(map, ([key, value]) => ({ key, value }))
console.log(arrayOfObjects) // Output: [{ key: 'key1', value: 'value1' }, { key: 'key2', value: 'value2' }]
This method transforms the key-value pairs into objects.
Using Object.fromEntries()
If you need to convert a Map to an object first and then to an array of objects, Object.fromEntries()
is useful.
const map = new Map([['key1', 'value1'], ['key2', 'value2']])
const obj = Object.fromEntries(map)
const arrayOfObjects = Object.entries(obj).map(([key, value]) => ({ key, value }))
console.log(arrayOfObjects) // Output: [{ key: 'key1', value: 'value1' }, { key: 'key2', 'value': 'value2' }]
This method is beneficial when working with objects derived from a map.
Using a Loop
You can manually iterate over the Map and push each entry into an array.
const map = new Map([['key1', 'value1'], ['key2', 'value2']])
const array = []
map.forEach((value, key) => {
array.push([key, value])
})
console.log(array) // Output: [['key1', 'value1'], ['key2', 'value2']]
This method offers a more manual approach to achieve the same result.
Real-Life Solutions
In real-life scenarios, converting a Map to an array is often required when you need to manipulate or iterate over the data in a format that arrays can handle more easily. Here are some common situations:
-
Data Transformation: When processing data from APIs, it’s common to receive data as a Map. Converting this to an array of objects can simplify data manipulation and transformation tasks. We sometimes use those techniques when need to convert API data and use them in our Smart Tables and Multi Select components
-
Rendering Lists: Front-end frameworks like React often require arrays to render lists of components. Converting a Map to an array allows you to use
map()
,filter()
, and other array methods for rendering. -
Data Export: When exporting data to formats like CSV or JSON, having the data in an array format can be more convenient.
-
Performing Aggregations: Aggregation operations, such as summing values or finding averages, are often easier to perform on arrays using methods like
reduce()
.
Summary
Converting a Map to an array in JavaScript can be done using various methods such as Array.from(), the spread operator, and manual iteration. Each method offers flexibility depending on whether you need the keys, values, or both. Understanding these techniques enhances your ability to manipulate Map objects effectively.
By understanding and using these methods, you can efficiently handle and manipulate Map objects in your JavaScript code, ensuring that you can convert a map into an array in various ways suitable for different scenarios.