How to convert a string to boolean in JavaScript
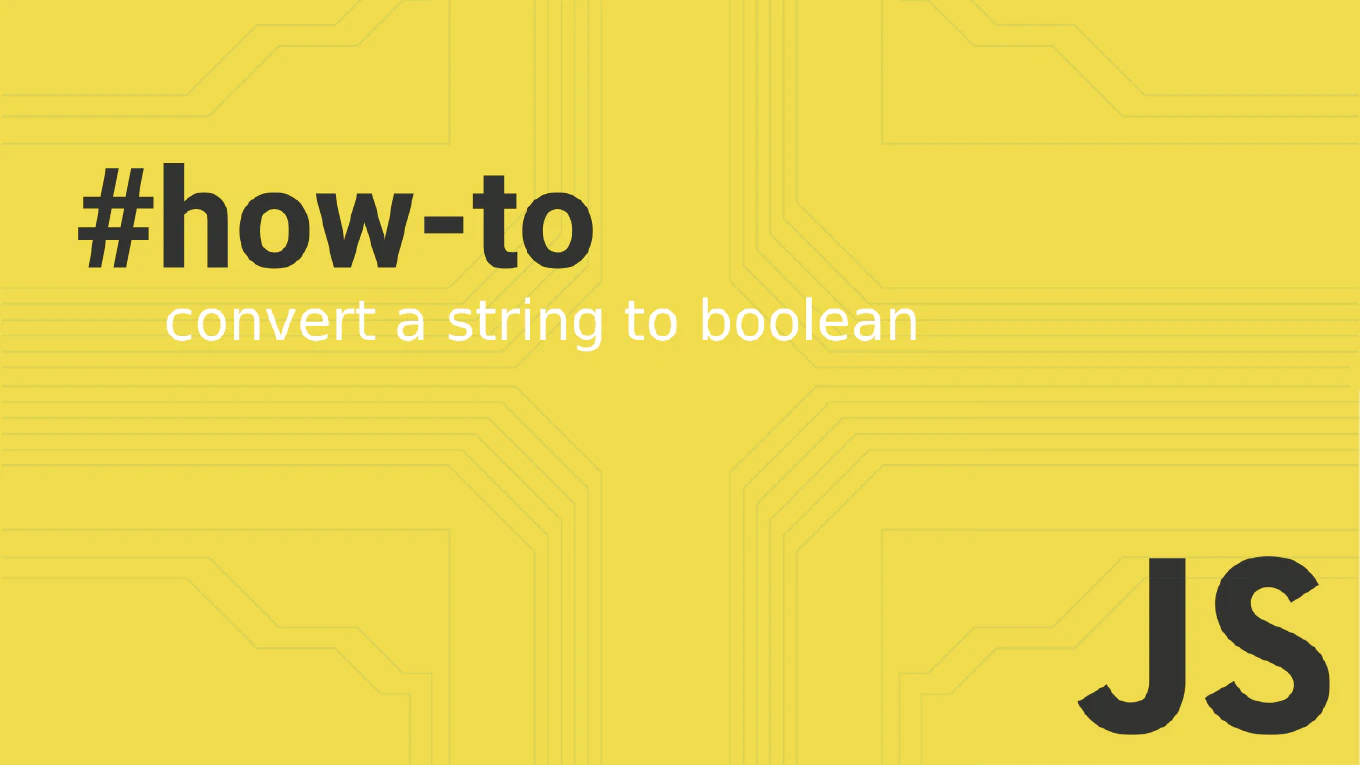
Working with different data types is a common task in JavaScript development. One frequent requirement is to convert a string to a boolean value. This article explores various methods to achieve this conversion, ensuring your code handles truthy values and false conditions accurately.
Understanding Boolean Values in JavaScript
In JavaScript, a boolean represents one of two values: true
or false
. Converting a string value to its corresponding boolean value is essential when processing user input, handling API responses, or evaluating conditions in your application.
Using the Boolean Function
The simplest way to convert a string to a boolean is by using the Boolean
function. This method evaluates the string and returns true for any non-empty string and returns false for an empty string.
const str = 'hello'
const bool = Boolean(str)
console.log(bool) // true
In this example, the string value 'hello'
is converted to true
. If the string were empty (''
), the Boolean
function would return false.
The Double Not Operator
Another common method is using the double not operator (!!
). This operator also converts a string to its boolean equivalent.
const str = ''
const bool = !!str
console.log(bool) // false
Here, the double not operator evaluates the string and returns false because the string is empty.
Caution with Certain Methods
Be cautious when using the Boolean
function or the double not operator for specific needs:
const myBool = Boolean('false') // true
const myBool = !!'false' // true
Any string that isn’t the empty string will evaluate to true
using these methods. While they’re clean and straightforward for boolean conversion, they might not produce the boolean result you’re looking for if the string value is 'false'
. In such cases, these methods will still return true, which might lead to unexpected behavior in your code.
Comparing Strings Directly
Sometimes, you might have specific string values like 'true'
or 'false'
. You can use the strict equality operator (===
) to compare the string and determine its boolean result.
const str = 'true'
const bool = str === 'true'
console.log(bool) // true
This method checks if the string matches 'true'
and returns true if it does. Otherwise, it returns false.
Handling Case Insensitivity
To make the comparison case insensitive, you can convert the string to lowercase using the toLowerCase
method.
const str = 'True'
const bool = str.toLowerCase() === 'true'
console.log(bool) // true
This ensures that inputs like 'True'
, 'TRUE'
, or 'true'
are all correctly evaluated to true
.
Using Regular Expressions
For more complex scenarios, regular expressions can determine if a string represents a true value.
const str = 'yes'
const regex = /^(true|yes|1)$/i
const bool = regex.test(str)
console.log(bool) // true
In this example, the regular expression checks if the string is 'true'
, 'yes'
, or '1'
, ignoring case sensitivity. The test
method evaluates the string and returns true if there’s a match.
Employing the Ternary Operator
The ternary operator offers a concise way to convert a string to a boolean.
const str = 'false'
const bool = str === 'true' ? true : false
console.log(bool) // false
This operator checks the condition and assigns the appropriate boolean value based on the result.
Dealing with Non-Boolean Values
If you need to handle other strings that don’t explicitly represent true
or false
, consider implementing a function that maps string representation of a boolean to its boolean value.
function parseBool(str) {
if (str === undefined || str === null) {
return false
}
return str.toLowerCase() === 'true'
}
const str = undefined
const bool = parseBool(str)
console.log(bool) // false
This function checks if the string is undefined or null and returns false. Otherwise, it performs a case-insensitive comparison to 'true'
.
Handling Errors
When the string does not represent a valid boolean, you might want to throw a new error.
function toBoolean(str) {
if (str.toLowerCase() === 'true') {
return true
} else if (str.toLowerCase() === 'false') {
return false
} else {
throw new Error('Invalid boolean string')
}
}
try {
const str = 'yes'
const bool = toBoolean(str)
console.log(bool)
} catch (error) {
console.log(error.message) // Invalid boolean string
}
This approach ensures your application handles invalid inputs gracefully.
Summary
Converting a string to boolean in JavaScript can be done using various methods like the Boolean
function, double not operator, strict equality operator, regular expressions, and the ternary operator. However, be mindful of the methods you choose, as some can lead to unexpected results with certain string values. Understanding these techniques allows you to handle different scenarios and ensure that your data is accurately converted and stored as a boolean.