How to declare the optional function parameters in JavaScript?
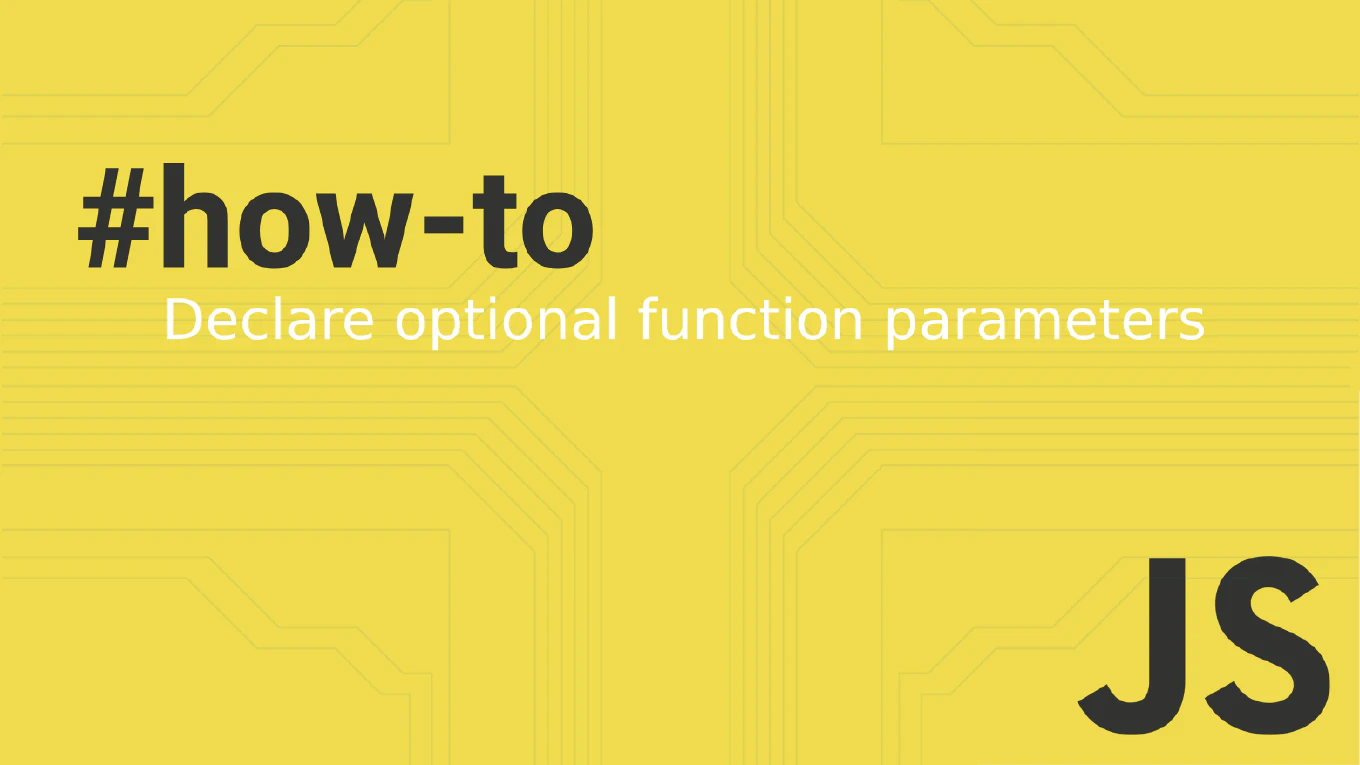
JavaScript is a versatile language that offers various methods to enhance the flexibility and adaptability of your code, especially when dealing with function parameters. Optional parameters in functions make your functions more flexible and easier to work with, allowing you to specify arguments that may or may not be passed when the function is called. In this article, we will delve into how you can declare optional function parameters in JavaScript, making your journey into web development smoother and more efficient.
Speed up your responsive apps and websites with fully-featured, ready-to-use open-source admin panel templates—free to use and built for efficiency.
Understanding Optional Parameters
Optional parameters allow functions to be called with fewer arguments than defined in the function’s declaration. This feature can significantly simplify your code, making it more readable and maintainable. Let’s explore the various methods to achieve this in JavaScript.
Using Default Parameter Values
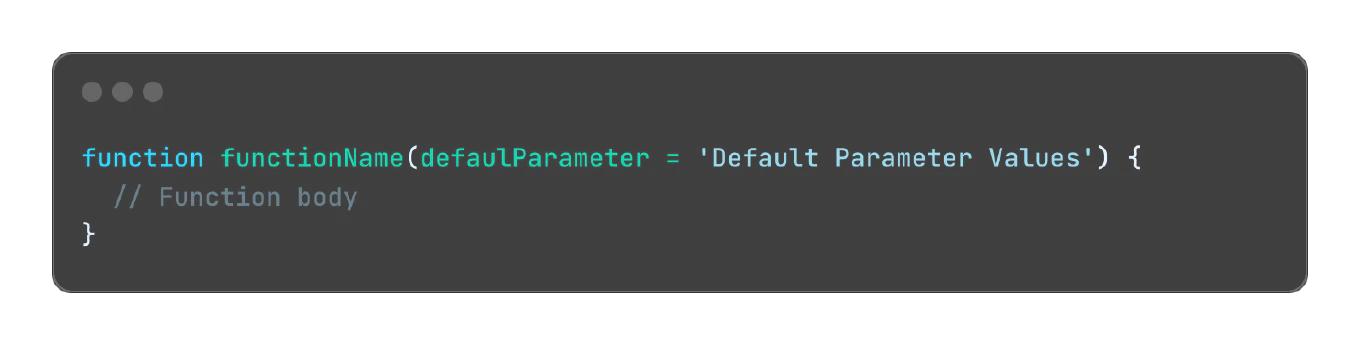
One of the most straightforward methods to declare optional parameters is assigning default values directly in the function signature. This feature was introduced in ES6 (ECMAScript 2015) and provides a clean and concise way to handle undefined parameters.
function welcome(username = 'New User') {
console.log(`Welcome, ${username}!`)
}
welcome() // Output: Welcome, New User!
welcome('Alice') // Output: Welcome, Alice!
This method ensures that if the username
parameter isn’t provided, it defaults to 'New User'
.
Leveraging the Logical OR Operator (||)
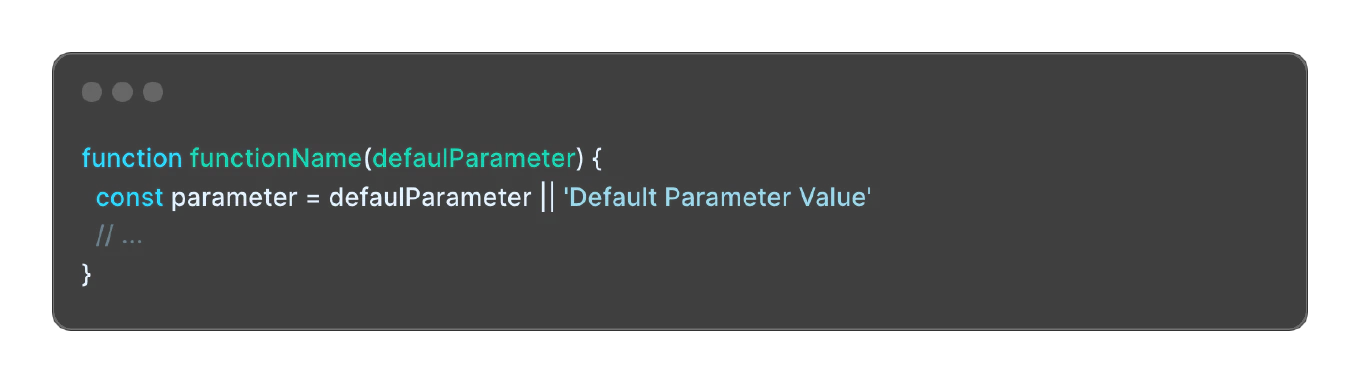
Before ES6, a typical pattern to assign default values to parameters involved using the logical OR operator within the function body.
function welcome(username) {
const name = username || 'New User'
console.log(`Welcome, ${name}!`)
}
welcome() // Output: Welcome, New User!
welcome('Alice') // Output: Welcome, Alice!
This technique checks if username
is truthy; if not, it defaults to 'New User'
. However, this approach can lead to unintended results with falsy values like 0
, null
, or ''
(empty string).
Using the arguments.length Property
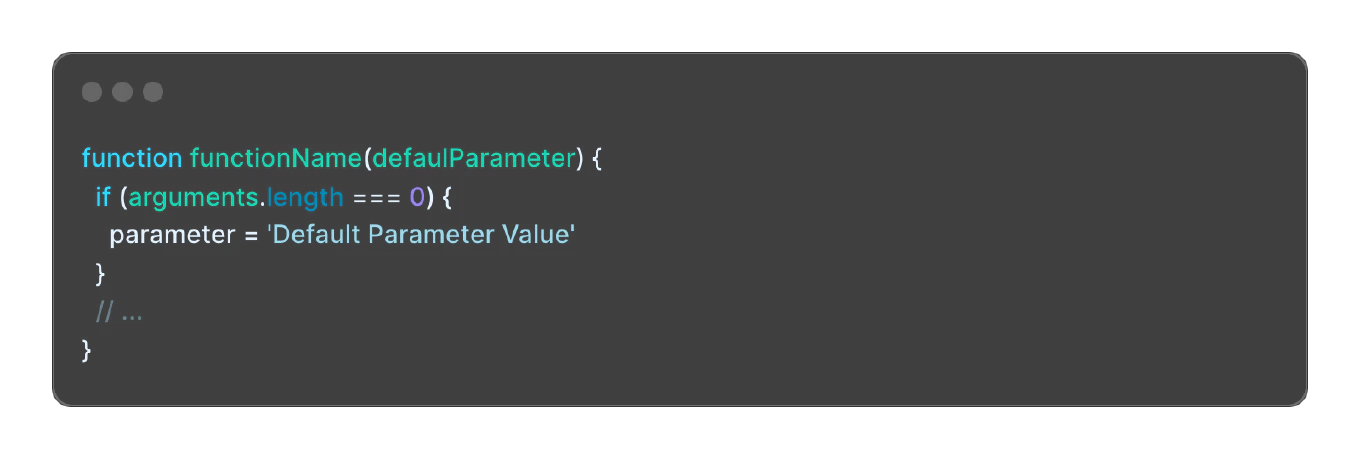
Another way to handle optional parameters is by checking the arguments.length
property, which returns the number of arguments passed to the function.
function welcome(username) {
if (arguments.length === 0) {
username = 'New User'
}
console.log(`Welcome, ${username}!`)
}
welcome() // Output: Welcome, New User!
welcome('Alice') // Output: Welcome, Alice!
This method is more verbose and less preferred compared to default parameters.
Destructured Parameter with Default Value Assignment
With ES6, you can also use destructuring assignment in function parameters to specify default values, offering a more powerful and flexible way to deal with optional parameters, especially when dealing with objects.
function welcome({ username = 'New User' } = {}) {
console.log(`Hello, ${username}!`)
}
welcome() // Output: Welcome, New User!
welcome({username:'Alice'}) // Output: Welcome, Alice!
This approach is beneficial when your function needs to accept multiple parameters encapsulated within an object.
Passing undefined vs. Other Falsy Values
It’s important to note that passing undefined
to a function with default parameters will trigger the default value, whereas other falsy values (null
, 0
, false
, NaN
, ''
) will not. This distinction is crucial for understanding how default values work compared to other methods like the logical OR operator.
function substract(a, b = 1) {
console.log(a - b)
}
substract(5) // Output: 4
substract(5, 0) // Output: 5
substract(5, false) // Output: 5
substract(5, NaN) // Output: NaN
substract(5, '') // Output: 5
substract(5, 3) // Output: 2
Antipatterns in Declaring Optional Parameters
Overusing arguments Object without Default Parameters
Before ES6 introduced default parameter values, a common approach was to rely heavily on the arguments
object to manually check for and assign default values to parameters. This method can lead to verbose and hard-to-read code.
// Antipattern: Excessive use of arguments object
function addUserDetails(name, age) {
name = arguments[0] || 'Unknown Name'
age = arguments[1] || 'Unknown Age'
// This becomes unwieldy with more parameters
}
Why to avoid: This approach clutters the function with manual checks and makes it difficult to immediately understand the function’s parameters and their default values.
Misusing the Logical OR Operator for All Types of Default Values
Using the logical OR operator (||
) to set default values works well for strings and numbers but can lead to unexpected results with boolean parameters or other falsy values that are valid inputs.
// Antipattern: Misusing logical OR for boolean parameters
function setNotificationSettings(emailAlerts, pushNotifications) {
emailAlerts = emailAlerts || true // Incorrectly overrides false to true
pushNotifications = pushNotifications || false
}
Why to avoid: This misuse fails to distinguish between a false
value passed intentionally and the absence of a value, leading to incorrect assignment and potentially buggy behavior.
Not Using Default Parameters for ES6 and Beyond
Ignoring the default parameter syntax available in ES6 and continuing to use older patterns for default values is a missed opportunity for cleaner, more readable code.
// Antipattern: Not utilizing default parameters in ES6
function logMessage(message) {
message = typeof message !== 'undefined' ? message : 'Default message'
console.log(message)
}
Why to avoid: This not only makes the code more verbose than necessary but also overlooks the improved readability and functionality provided by ES6 features.
Relying on Undefined to Trigger Default Parameters
Explicitly passing undefined
to trigger a default parameter can be confusing and lead to code that’s harder to understand, especially for those new to JavaScript.
// Antipattern: Explicitly passing undefined
function displayBanner(text = 'Welcome!') {
console.log(text)
}
displayBanner(undefined) // Intentionally passing undefined
Why to avoid: While technically correct, it’s clearer and more intuitive to omit the argument altogether if you wish to use the default value.
Final Thoughts
Declaring optional parameters in JavaScript functions enhances their flexibility and usability. Whether you’re using ES6 features like default parameter values and destructuring assignments or older patterns like the logical OR operator and the arguments.length
property, understanding these techniques is vital for writing effective and efficient JavaScript code.
By mastering optional parameters, you’ll be able to create more versatile and robust functions, improving the overall quality of your web applications. Remember, the choice of method depends on your specific needs and the JavaScript version you’re working with, so choose the one that best fits your scenario.
Happy coding!