How to fix “SyntaxError: Cannot use import statement outside a module”?
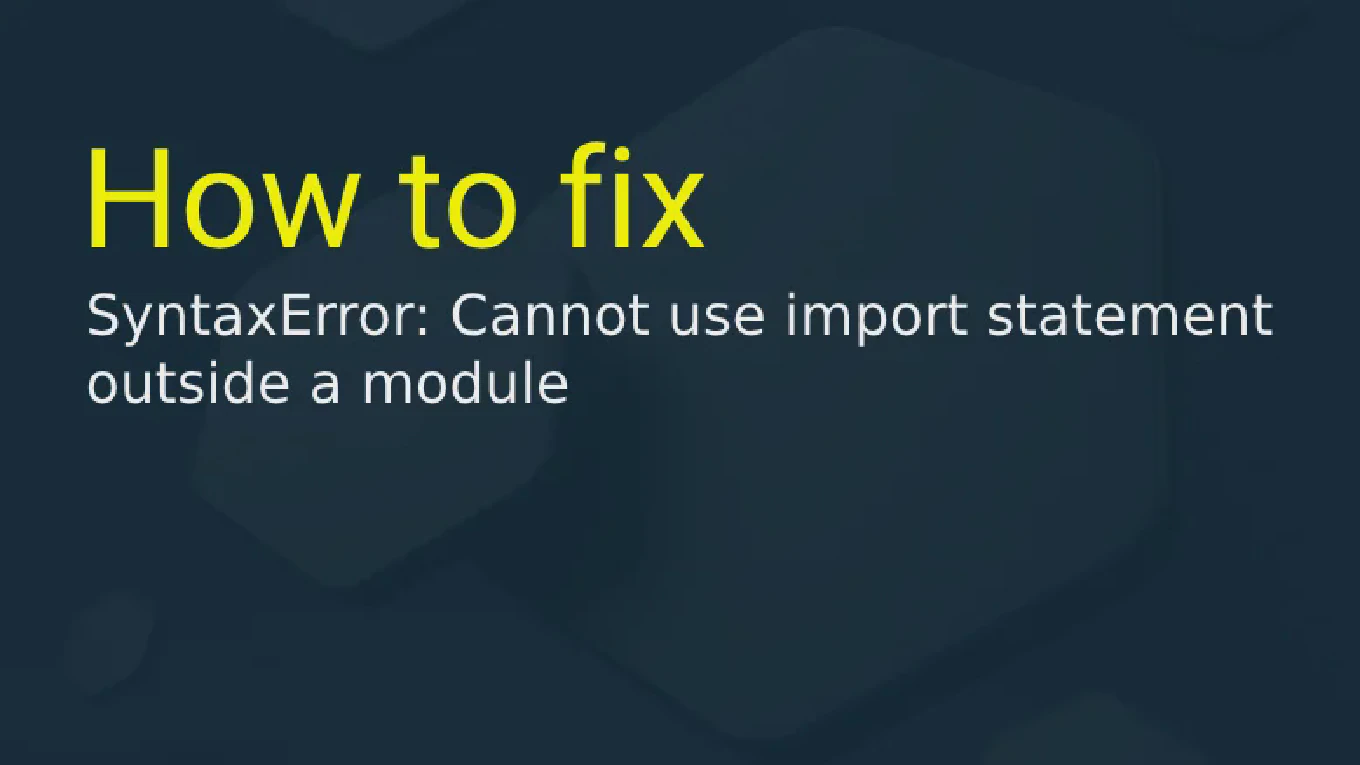
In your development journey, encountering errors is a norm, but some can leave you scratching your head. One such instance is when you’re met with “Cannot use import statement outside a module” in JavaScript. This error can be a hurdle, but with the right guidance, you can overcome it easily. In this article, we’ll explore the why and how of fixing this common issue, equipping you with the tools to continue coding without interruptions.
Speed up your responsive apps and websites with fully-featured, ready-to-use open-source admin panel templates—free to use and built for efficiency.
Understanding the Error Message
First things first, let’s demystify the error message. The JavaScript ES6 version introduced import/export syntax that allows developers to modularize their code for maintainability and reusability. However, when JavaScript encounters an import statement in a script that it doesn’t recognize as a module, it triggers this error.
Can I Use Import Statement Outside a Module?
The short answer is no. Import statements are part of the module system and are typically used within modules. If you try to use them in a regular script, JavaScript engines will not understand it and thus, throw an error.
So, How Do You Correct This?
Let’s break it down into actionable steps.
Step 1: Ensure Module Support in Your Environment
Before using import statements, make sure that your environment supports ES6 modules.
To ensure ES6 module support in Node.js, use a version with the --experimental-modules
flag. The flag was first introduced in Node.js 8.5.0. In Node.js v13.2.0, the flag was replaced with a new implementation, and ES modules were supported without any flags. Upgrade to Node.js v13.2.0 or higher to use ES modules without flags.
The latest version of Node.js fully supports ES modules as they are currently specified and allows for interoperability between them and its original module format, CommonJS.
For browsers, check if they support module scripts. Most modern browsers do, but it’s always good to verify. Most modern browsers, such as Chrome, Firefox, Edge, and Safari, have support for ES modules, eliminating the need for browser polyfills, bundlers, or transpilers.
Using the React or Vue JavaScript-based frontend libraries eliminates the need for them, as they already support the ES imports and exports fields by default. However, older browsers may not support ES syntax, which is why these tools are necessary for cross-platform compatibility.
One common reason for errors in an older browser is when a page’s HTML files are lacking the type="module"
attribute. The error happens because JavaScript running on the web does not have built-in support for ES module syntax. When attempting a cross-origin resource-sharing, one may encounter an error with loading an ES module from a different domain.
Step 2: Designate Your Script as a Module
If using HTML, ensure your <script>
tags have the type "module"
like so:
...
<script type="module" src="yourfile.js"></script>
...
For Node.js, use the .mjs
file extension or add "type": "module"
in your package.json
to indicate that you’re using ES6 modules.
{
"type": "module"
}
Step 3: Correct Syntax and File Paths
Syntax and correct file paths are critical. Always check that your import statements are precise and the paths to the modules are correct.
import { someFunction } from './someModule.js';
Step 4: Configure Build Tools
Make sure that your bundler or transpiler is set up to handle ES6 modules. This may involve installing the necessary plugins or presets and configuring them correctly in your project configuration files.
For webpack, you can use the @babel/preset-env
preset along with the @babel/plugin-transform-modules-commonjs
plugin to handle ES6 modules. Install these dependencies and add them to your Babel configuration file (usually .babelrc
or babel.config.js
). Here’s an example configuration:
{
"presets": [
"@babel/preset-env"
],
"plugins": [
"@babel/plugin-transform-modules-commonjs"
]
}
For Babel, you can specify the modules
option in the @babel/preset-env
preset to handle modules. Set it to "auto"
or "commonjs"
depending on your needs. Here’s an example configuration:
{
"presets": [
["@babel/preset-env", {
"modules": "auto"
}]
]
}
With these configurations, your bundler or transpiler should be able to properly handle import statements and convert them into a format that is compatible with your environment.
Step 5: Use the Script in an HTTP Server Environment
When working in a local environment, browsers might fail to import modules due to CORS policies. Use an HTTP server instead of opening your HTML files directly with a browser.
Advanced Topics: Using Import in TypeScript and Beyond
Now let’s delve deeper with some advanced scenarios you may encounter.
How to Use Import Statement in TypeScript?
TypeScript embraces ES6 modules and supports the import statement natively. The types ensure safe and consistent importing across your codebase. However, remember to transpile your TypeScript to JavaScript, targeting the module system of your environment during the build process. This ensures compatibility.
{
"compilerOptions": {
"target": "esnext",
"module": "commonjs",
"esModuleInterop": true,
// ... your other options
}
}
How to Import Require Module in JavaScript?
To mix ES6 imports with CommonJS’s require()
method, a common pattern for Node.js applications, you can use dynamic imports:
async function loadModule() {
let module = await import('module-name');
}
Dynamic imports can offer a bridge between the two systems, allowing greater flexibility within your codebase.
Summary
The “Cannot use import statement outside a module” error can occur for various reasons, depending on whether you’re in a browser-side or server-side JavaScript environment. Factors such as incorrect syntax, improper configurations, and unsupported file extensions are among the most frequent causes of this error.
Most modern browsers have support for ES modules, but it is important to ensure compatibility with older browsers. Bundlers like Webpack enable you to compile all source code and their dependencies into a single output that can be understood by older browsers.
To indicate that the module is an ES module, be sure to include the type="module"
attribute in the HTML file. Furthermore, although it is commonly done to use the .js extension for CommonJS, you also have the choice to use the .mjs extension to enable importing ES modules.
Let’s summarize the key points from our journey:
- Import statements can only be used within modules.
- Ensure your environment supports modules, and scripts are correctly designated.
- Always check the syntax and paths in your import statements.
- Configure bundlers and transpilers if used.
- In local development, use an HTTP server to avoid CORS errors.
- Seek compatibility between different module systems with transpiling or dynamic importing when necessary.
Now that you understand how to address the “Cannot use import statement outside a module” error, it’s time to implement these solutions in your projects. By doing so, you’ll enjoy a cleaner, organized, and more efficient codebase.