Javascript Random - How to Generate a Random Number in JavaScript?
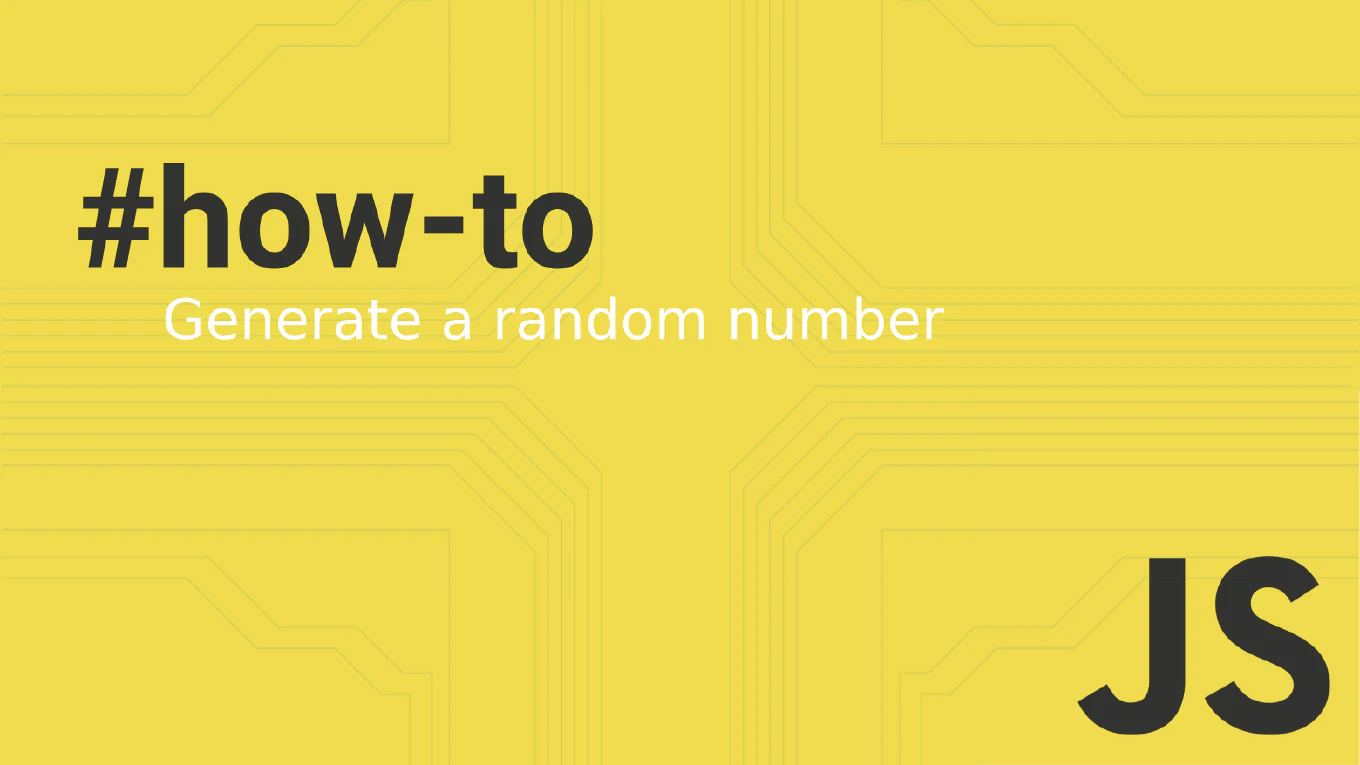
JavaScript offers various ways to generate random numbers, which are essential in many programming scenarios like gaming, simulations, and anytime you need some unpredictability in your output. In this article, we aim to introduce beginners to the simplest methods of generating random numbers in JavaScript using the Math.random()
function.
What is Math.random()
?
Math.random()
is a powerful tool in JavaScript that generates a pseudo-random number—a number that seems random but is actually generated through a deterministic process. It returns a floating-point, or decimal, number between 0 (inclusive) and 1 (exclusive). This means you can get a number as small as 0 but never exactly 1.
Basic Usage of Math.random()
Here’s the most straightforward example to generate a random number:
const randomNumber = Math.random()
console.log(randomNumber)
Each time you run this code, console.log
will display a new random number between 0 and 1.
Generating a Random Number Within a Specific Range
Often, you’ll need a random number between two specific values rather than floating point number between 0 and 1. You can achieve this by adjusting the output of Math.random()
.
Decimal Numbers Within a Range
To generate a random decimal number between two values, you can use this function:
const getRandomNumber = (min, max) => {
return Math.random() * (max - min) + min
}
// Random number between 5 and 10
const randomNumber = getRandomNumber(5, 10)
console.log(randomNumber)
// Random number between 0 and 100
const randomNumber2 = getRandomNumber(0, 100)
console.log(randomNumber2)
This function multiplies the difference between max
and min
by the result of Math.random()
, then adds the min
value. This scales the random number to your desired range.
Random Integer Numbers Within a Range
Generating a whole number (integer) within a range involves a similar approach but includes steps to round off the decimal:
const getRandomInteger = (min, max) => {
min = Math.ceil(min)
max = Math.floor(max)
return Math.floor(Math.random() * (max - min)) + min
}
// Random integer between 5 and 9
const randomInteger = getRandomInteger(5, 10)
console.log(randomInteger)
// Random integer between 0 and 99
const randomInteger2 = getRandomInteger(0, 100)
console.log(randomInteger2)
Here, Math.ceil()
ensures the minimum value is rounded up to the nearest whole number, and Math.floor()
does the opposite for the maximum, ensuring the range is strictly between the specified min (inclusive) and max (exclusive).
Inclusive Random Integers
If you need to include both the minimum and maximum numbers in your range of possible outcomes, adjust the function like so:
const getRandomIntegerInclusive = (min, max) => {
min = Math.ceil(min)
max = Math.floor(max)
return Math.floor(Math.random() * (max - min + 1)) + min
}
// Random integer between 5 and 10, inclusive
const randomInteger3 = getRandomIntegerInclusive(5, 10)
console.log(randomInteger3)
// Random integer between 0 and 100, inclusive
const randomInteger4 = getRandomIntegerInclusive(0, 100)
console.log(randomInteger4)
By adding 1 to (max - min)
, we ensure that the maximum value is also a possible result.
Conclusion
Generating random numbers in JavaScript is a fundamental skill that can be quickly learned and applied in various scenarios. Whether you’re developing games, simulations, or other applications requiring random data, the Math.random()
function is an indispensable tool in your JavaScript toolkit.
Remember, while JavaScript’s random numbers are sufficient for many applications, they are not suitable for cryptographic purposes due to their predictable nature. For such needs, look into cryptographically secure methods provided by modern browsers.
Happy coding!
FAQ
What is Math.random()
used for in JavaScript?
Math.random()
is javascript function used to generate a pseudo-random number between 0 (inclusive) and 1 (exclusive). This function is commonly used in scenarios like gaming, simulations, creating random animations, or anytime randomness is needed in a JavaScript program.
Why does Math.random()
not include 1 in its output?
Math.random()
is designed to return a number from 0 up to but not including 1 to ensure a uniform distribution of numbers over the range 0 to less than 1. This design choice helps in scaling the output to any range without worrying about including the upper bound.
Can Math.random()
generate negative numbers?
No, Math.random()
by itself only generates numbers in the range of 0 to less than 1. If you need a random negative number, you can adjust the output by multiplying by -1 or subtracting from zero.
Is it possible to generate a random string using Math.random()
?
Yes, while Math.random()
directly returns a random number, you can transform this number into a string in various ways. For example, you could convert the number to a string in base 36 to get a random alphanumeric output, which can be useful for creating unique IDs or keys.
Are the random numbers generated by Math.random()
secure for cryptographic use?
No, the numbers generated by Math.random()
are not suitable for cryptographic purposes because they do not provide sufficient randomness required for security features. For cryptographic purposes, you should use the Web Crypto API’s crypto.getRandomValues()
method, which is designed to produce cryptographically secure pseudo-random numbers.
How can I generate a random number within a specific range in JavaScript?
To generate a random number within a range [min, max]
, use the formula: Math.random() * (max - min) + min
. If you need the range to be inclusive of both ends [min, max]
, adjust the formula to: Math.floor(Math.random() * (max - min + 1)) + min
.