How to Get Unique Values from a JavaScript Array
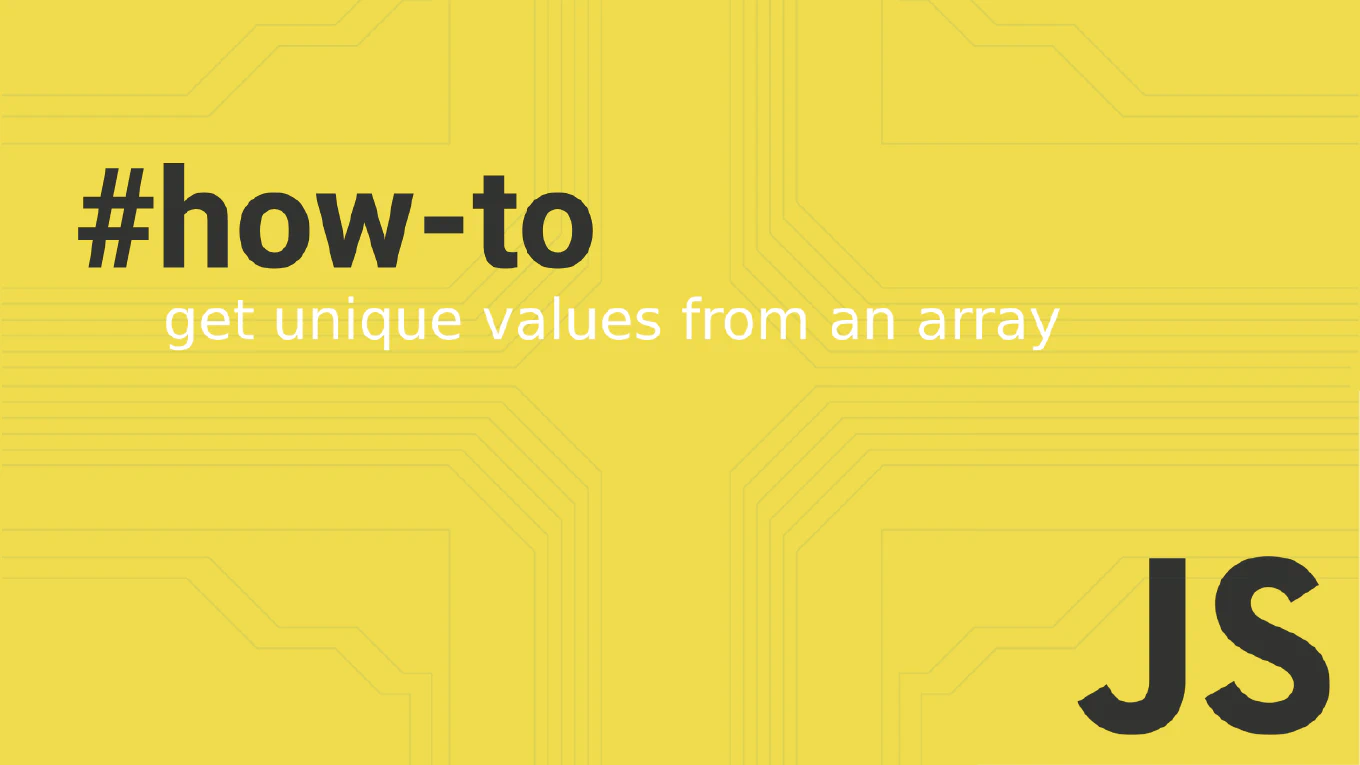
In JavaScript, handling arrays and ensuring their uniqueness is a common task. Here are several methods to achieve unique values from an array, ensuring you remove duplicate values effectively.
Using Set
and the Spread Operator
The most efficient way to get unique values from an array is by using the Set
object along with the spread operator. This method is concise and leverages ES6 features:
const array = [1, 2, 2, 3, 4, 4, 5]
const uniqueArray = [...new Set(array)]
console.log(uniqueArray) // Output: [1, 2, 3, 4, 5]
The Set
object automatically removes duplicate values, and the spread operator converts it back to an array.
Using filter
and indexOf
Another method involves using the filter
function along with indexOf
to ensure each value is only included once:
const array = [1, 2, 2, 3, 4, 4, 5]
const uniqueArray = array.filter((value, index, self) => self.indexOf(value) === index)
console.log(uniqueArray) // Output: [1, 2, 3, 4, 5]
In this approach, indexOf
checks if the current value’s index matches its first occurrence in the array.
Using a Helper Array
A more manual method creates a new array from the original array using a helper array to keep track of unique values:
const array = [1, 2, 2, 3, 4, 4, 5]
const uniqueArray = []
array.forEach(item => {
if (!uniqueArray.includes(item)) {
uniqueArray.push(item)
}
})
console.log(uniqueArray) // Output: [1, 2, 3, 4, 5]
Here, the includes
method checks if the item is already in the uniqueArray
before adding it.
Handling Arrays of Objects
When dealing with an array of objects, uniqueness can be based on a specific property. For example, to get unique objects by the age
property:
const array = [
{ name: 'Alice', age: 25 },
{ name: 'Bob', age: 25 },
{ name: 'Charlie', age: 30 }
]
const uniqueArray = array.filter((value, index, self) =>
index === self.findIndex((t) => (
t.age === value.age
))
)
console.log(uniqueArray) // Output: [{ name: 'Alice', age: 25 }, { name: 'Charlie', age: 30 }]
This method uses findIndex
to ensure only the first occurrence of each age is included.
Benchmarking Different Methods
Performance is crucial when dealing with large arrays. Below is a benchmark comparison of different methods for obtaining unique values from an array.
Benchmark Results
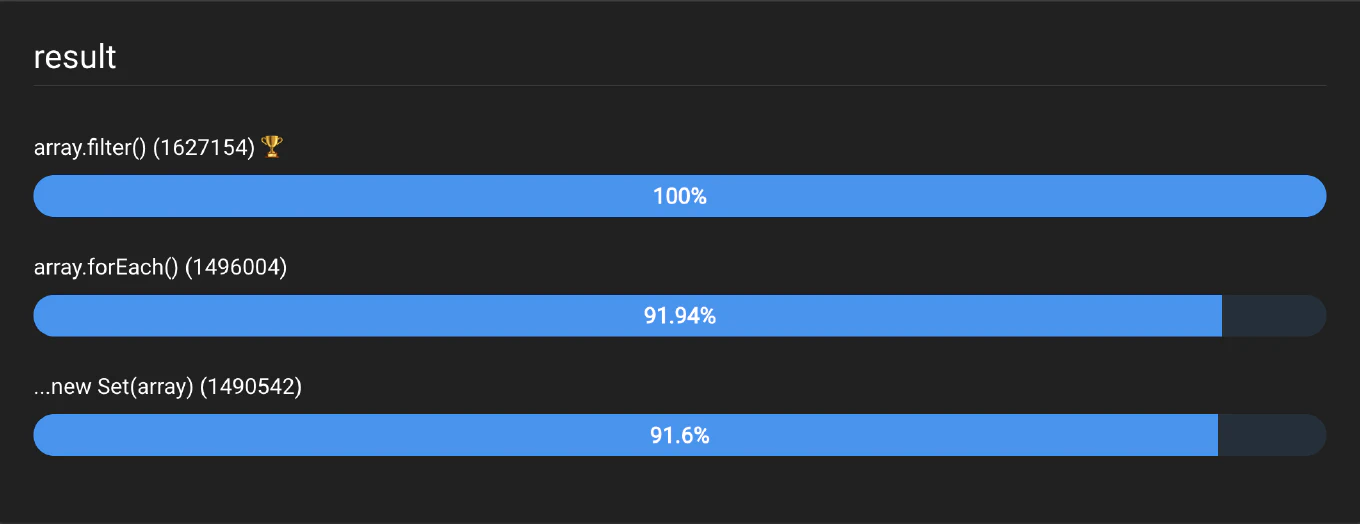
According to the benchmark from jsben.ch:
array.filter()
method is the fastest.array.forEach()
and...new Set(array)
are nearly as fast but slightly slower.
Conclusion
Each method has its use cases. Using Set
is the most straightforward for primitive values. For more complex scenarios, such as arrays of objects, filtering with findIndex
or using a helper array can be more appropriate.
These techniques will help you efficiently remove duplicates and ensure the resulting array contains only unique values from your arrays in JavaScript.