How to loop through a 2D array in JavaScript
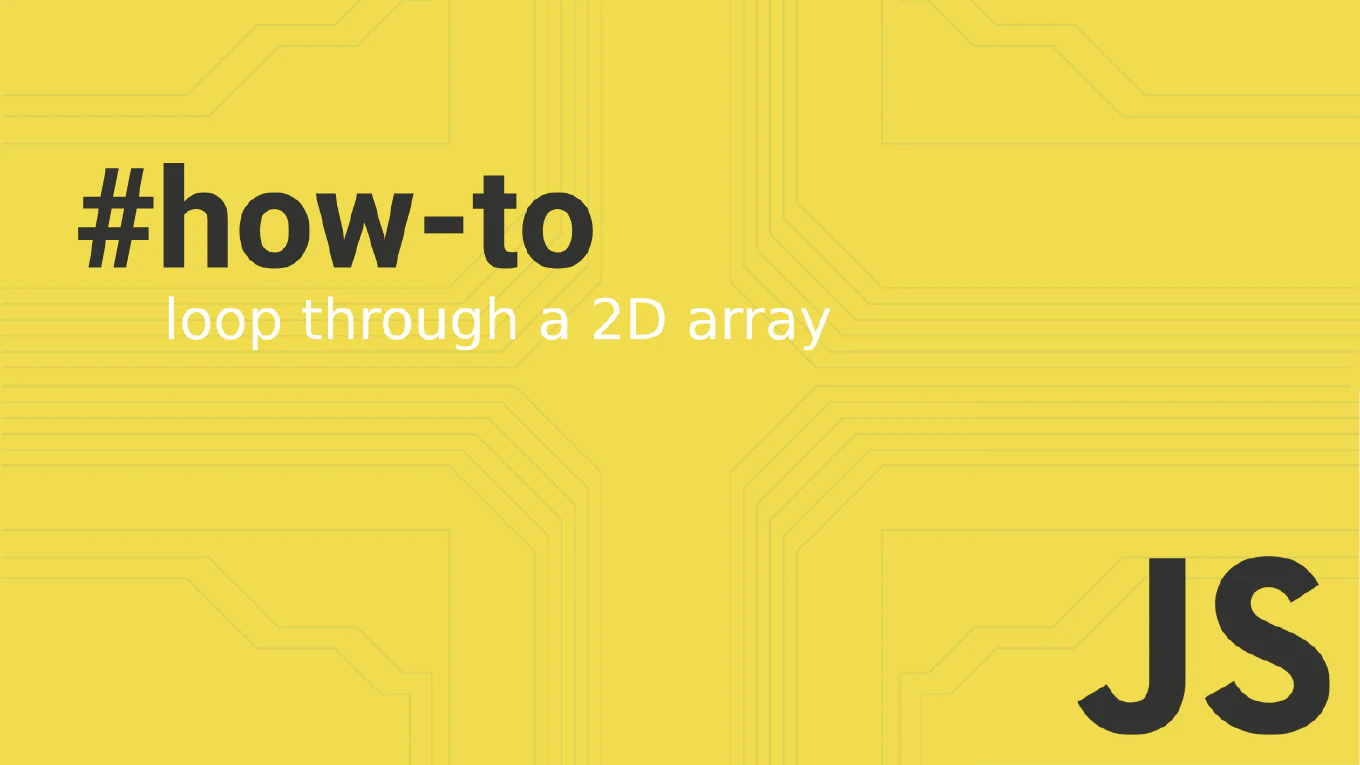
In JavaScript, a two-dimensional (2D) array is an array of arrays. It’s often used to store data in a tabular form. Traversing these arrays efficiently is crucial for many software development tasks. This article covers various methods to loop through a 2D array in JavaScript, offering examples to demonstrate each technique.
Basic 2D Array Structure
When dealing with a two-dimensional array, understanding the concept of outer array element and inner array elements is crucial. The outer array elements are the main arrays that hold other arrays as their elements. The inner array elements are the elements within each of these nested arrays. Iterating through a 2D array involves handling both outer array elements and inner array elements effectively.
To create a two-dimensional array in JavaScript, use the array literal notation:
const array = [
[1, 2, 3],
[4, 5, 6],
[7, 8, 9]
]
Using Nested For Loops
The most straightforward way to iterate through a 2D array is using nested for
loops:
const array = [
[1, 2, 3],
[4, 5, 6],
[7, 8, 9]
]
for (let i = 0; i < array.length; i++) {
for (let j = 0; j < array[i].length; j++) {
console.log(array[i][j])
}
}
Here, the outer loop traverses the rows of the multidimensional array, while the inner loop traverses the elements within each row.
Using For…Of Loops
The for...of
loop simplifies iterating over arrays in JavaScript:
for (const row of array) {
for (const element of row) {
console.log(element)
}
}
This method provides a cleaner syntax and avoids using array indices directly.
Using ForEach Method
The forEach
method can also be used to iterate through a 2D array:
array.forEach(row => {
row.forEach(element => {
console.log(element)
})
})
This method takes a callback function that executes for each element in the array, providing an elegant way to handle nested arrays.
Using Map Method
The map
method is typically used for transforming arrays, but it can also iterate through a multi dimensional array:
array.map(row => {
row.map(element => {
console.log(element)
})
})
Like forEach
, map
applies a function to each element in the array, making it versatile for both iteration and transformation.
Accessing Elements at Specific Indices
To access elements at a specified index in a 2D array:
const element = array[1][2] // Accesses the element in the 2nd row and 3rd column
console.log(element) // Outputs 6
Adding and Removing Elements
To add elements to a 2D array:
array[0].push(10) // Adds 10 to the end of the first row
console.log(array[0]) // Outputs [1, 2, 3, 10]
To remove elements:
array[1].pop() // Removes the last element from the second row
console.log(array[1]) // Outputs [4, 5]
Practical Example
Here’s a practical example to sum all array elements in a 2D array:
let sum = 0
array.forEach(row => {
row.forEach(element => {
sum += element
})
})
console.log(sum) // Outputs 45
Conclusion
Traversing a two-dimensional array in JavaScript can be done using various methods such as nested loops, for...of
, forEach
, and map
. Understanding these techniques is essential for any frontend developer working with complex data structures.
By following these examples and experimenting with your 2D arrays, you’ll master the art of iterating through multidimensional arrays in JavaScript. Happy coding!