How to Open All Links in New Tab Using JavaScript
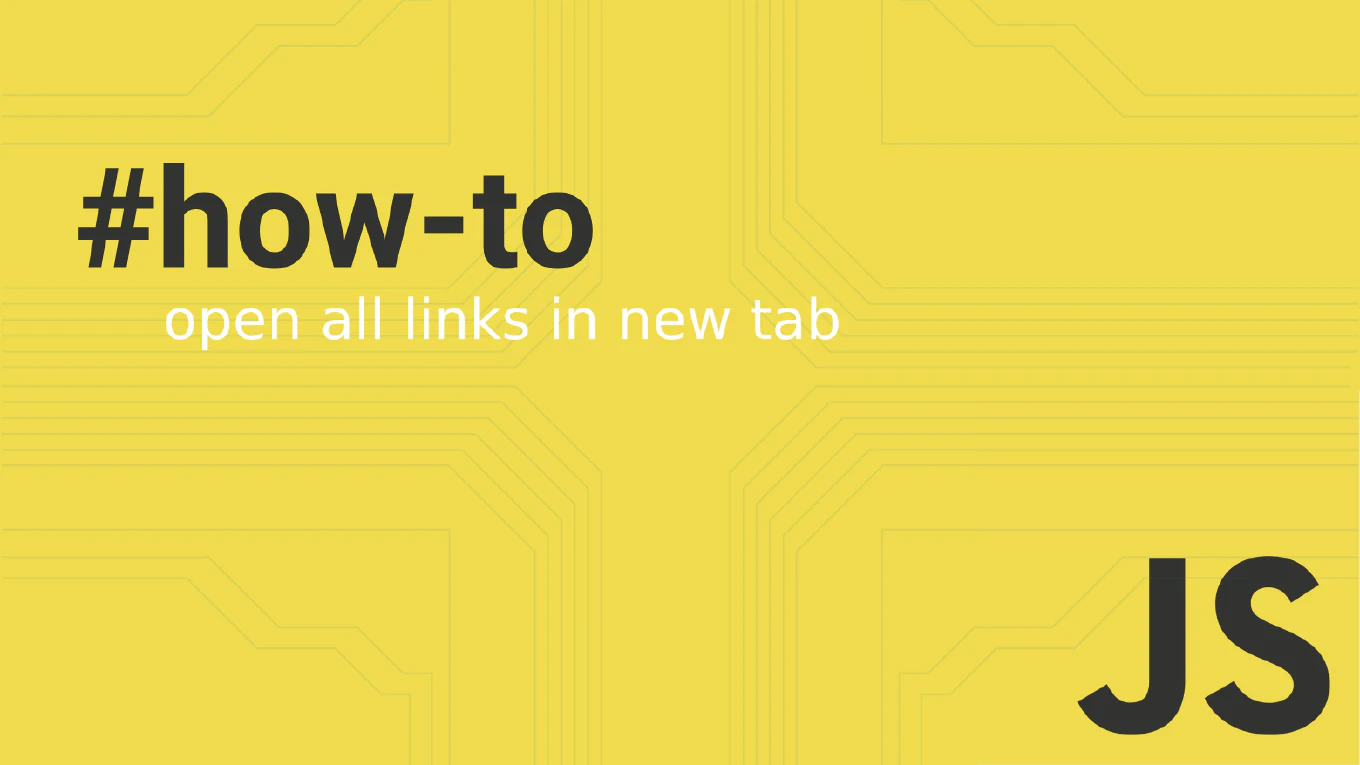
Opening all links in a new tab can enhance user experience, especially when dealing with external links. This comprehensive guide will show you how to open all the links using JavaScript.
Understanding the Basics
Before diving into the JavaScript code, it’s essential to understand the core HTML elements and attributes involved, such as the target
attribute.
HTML Document Structure
<!DOCTYPE html>
<html>
<head>
<title>Open Links in New Tab</title>
</head>
<body>
<a href="https://example.com">Example</a>
<a href="https://anotherexample.com">Another Example</a>
<!-- More links -->
</body>
</html>
Using JavaScript to Target Links
To open all links in a new tab, we need to target the anchor elements (<a>
tags) and modify their target
attribute.
Adding JavaScript Code
<script>
document.addEventListener('DOMContentLoaded', () => {
const links = document.querySelectorAll('a')
links.forEach(link => {
link.setAttribute('target', '_blank')
})
})
</script>
Explanation of the Code
The document.addEventListener('DOMContentLoaded', () => {})
ensures the code runs after the HTML document is fully loaded. The line const links = document.querySelectorAll('a')
selects all the anchor elements in the document. The links.forEach(link => { link.setAttribute('target', '\_blank') })
sets the target
attribute to _blank
for each link, ensuring they open in a new tab.
Using JavaScript for Open External Links Only
If you want to target only external links, you can filter the links based on their href
attribute.
<script>
document.addEventListener('DOMContentLoaded', () => {
const links = document.querySelectorAll('a')
links.forEach(link => {
if (link.href.includes('http')) {
link.setAttribute('target', '_blank')
}
})
})
</script>
Advantages of Opening Links in New Tabs
Opening links in new tabs enhances user experience by keeping the original web page open and is useful for referencing external resources without navigating away from the current page.
Potential Drawbacks
Opening links in new tabs can be annoying for users who prefer to open links in the same tab and may lead to a clutter of open tabs if overused.
Ensuring Compatibility Across Browsers
Most modern browsers support the target="_blank"
attribute. However, testing across different browsers is recommended.
Additional JavaScript Customizations
You can extend the script to include more sophisticated logic, such as opening links based on specific conditions or dynamically generated content.
Example with Dynamic Content
<script>
document.addEventListener('DOMContentLoaded', () => {
const links = document.querySelectorAll('a')
links.forEach(link => {
if (link.href.includes('http')) {
link.setAttribute('target', '_blank')
}
})
})
function addLink(url, text) {
const newLink = document.createElement('a')
newLink.href = url
newLink.textContent = text
newLink.setAttribute('target', '_blank')
document.body.appendChild(newLink)
}
addLink('https://newexample.com', 'New Example')
</script>
Conclusion
Opening links in a new tab using JavaScript is a simple yet powerful technique to improve navigation and user experience on your web page. By understanding and implementing the methods discussed, you can ensure that your external links open seamlessly in new tabs, enhancing the usability of your website.