How to remove a property from an object in Javascript
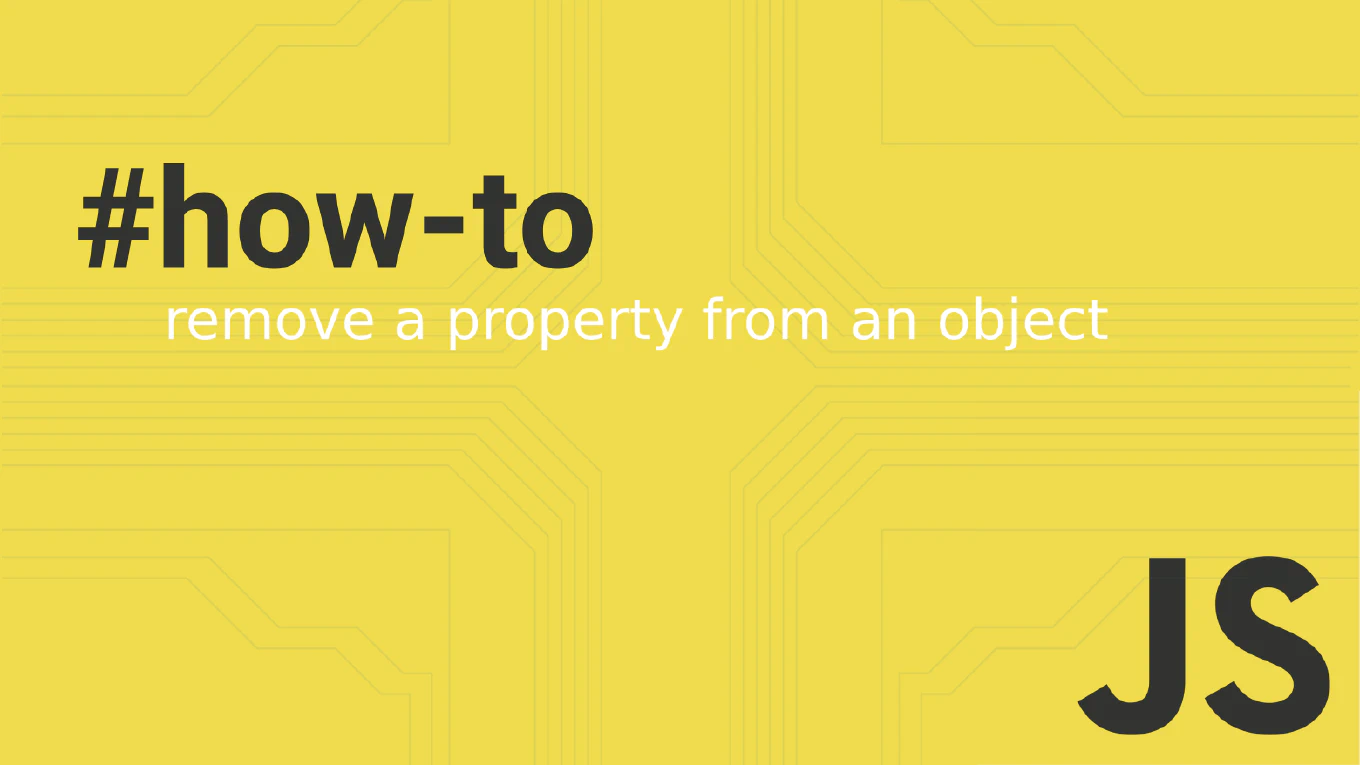
In JavaScript, working with objects is a fundamental part of building applications. Often, you may need to remove properties from an object, whether it’s for cleaning up data or preparing an object for a specific use case. This article explores various techniques for removing properties from a JavaScript object, discussing the advantages and potential pitfalls of each method. We’ll also cover how to remove multiple properties from a single object efficiently.
Speed up your responsive apps and websites with fully-featured, ready-to-use open-source admin panel templates—free to use and built for efficiency.
Methods for Removing Properties from a JavaScript Object
1. Using the delete
Operator
The most direct way to remove a property from a JavaScript object is by using the delete
operator. This operator is straightforward and easy to use, but it comes with certain caveats that developers need to be aware of.
const person = {
name: 'John Doe',
age: 30,
profession: 'Developer'
}
delete person.age
console.log(person) // Output: { name: 'John Doe', profession: 'Developer' }
How the delete
Operator Works
The delete
operator removes a specified property from a JavaScript object. After deletion, the property is no longer accessible within the original object. However, the delete
operator does not affect the object’s prototype chain, so if a property exists in the prototype, it can still be accessed.
Potential Pitfalls of delete
While the delete
operator is easy to use, it has a few downsides:
- Performance Issues: The
delete
operator can cause performance degradation, especially in performance-critical applications. JavaScript engines optimize objects for speed, but when a property is deleted, it can lead to de-optimization. - Mutating Objects: The
delete
operator mutates the original object by removing the specified property. This can lead to unintended side effects, especially when working with shared objects in a global scope.
2. Setting the Property to undefined
or null
Another approach to removing properties from a JavaScript object is to set the property’s value to undefined
or null
. This doesn’t physically remove the property from the object but indicates that the property has no value.
const person = {
name: 'John Doe',
age: 30,
profession: 'Developer'
}
person.age = undefined
console.log(person) // Output: { name: 'John Doe', age: undefined, profession: 'Developer' }
Differences Between undefined
and null
undefined
: Represents a variable or property that hasn’t been assigned a value.null
: Represents the intentional absence of any object value.
This method is useful when you need to retain the structure of the original object but want to indicate that a property has no value.
Potential Pitfalls
Setting a property to undefined
or null
means the property still exists in the object. This can lead to confusion if other parts of your code expect the property to be completely absent.
3. Using Object Destructuring and Rest Syntax
ES6 introduced a more elegant and functional approach to removing properties from a JavaScript object using object destructuring combined with the rest syntax.
const person = {
name: 'John Doe',
age: 30,
profession: 'Developer'
}
const { age, ...newPerson } = person
console.log(newPerson) // Output: { name: 'John Doe', profession: 'Developer' }
Advantages of Object Destructuring
- Immutability: This method doesn’t mutate the original object but creates a new object with the remaining properties.
- Readability: The syntax is concise and clear, making it easier to understand at a glance.
- Non-Destructive: By creating a shallow copy of the object, this method preserves the original object.
Potential Pitfalls
- Memory Usage: Since this method creates a new object, it could lead to increased memory usage, especially when dealing with large objects.
4. Using Reflect.deleteProperty
JavaScript also provides the Reflect.deleteProperty
method for developers who prefer a more formal and potentially safer way to delete object properties.
const employee = {
name: 'Jane Smith',
age: 25,
position: 'Designer'
}
Reflect.deleteProperty(employee, 'age')
console.log(employee) // Output: { name: 'Jane Smith', position: 'Designer' }
Why Use Reflect.deleteProperty
The Reflect.deleteProperty
method is part of the Reflect API and provides a more consistent and reliable way to handle property removal. It behaves similarly to the delete
operator but with fewer quirks.
Potential Pitfalls
As with the delete
operator, this method still comes with potential performance costs and should be used judiciously in performance-critical code.
5. How to Remove Multiple Properties from a JavaScript Object
In some cases, you may need to remove multiple properties from a JavaScript object simultaneously. One efficient way to achieve this is by using object destructuring with the rest syntax.
const person = {
name: 'John Doe',
age: 30,
profession: 'Developer',
country: 'USA'
}
const { age, country, ...newPerson } = person
console.log(newPerson) // Output: { name: 'John Doe', profession: 'Developer' }
This approach allows you to easily remove multiple properties while keeping the remaining properties intact in a new object. This method is especially useful when working with larger objects or when the properties to be removed are not adjacent.
Another way to remove multiple properties is by using a loop with the delete
operator:
const person = {
name: 'John Doe',
age: 30,
profession: 'Developer',
country: 'USA'
}
const keysToRemove = ['age', 'country']
keysToRemove.forEach(key => delete person[key])
console.log(person) // Output: { name: 'John Doe', profession: 'Developer' }
This method directly mutates the original object, so it’s important to use it with caution.
Conclusion
Removing properties from JavaScript objects is a common task with multiple approaches. The delete
operator is the most direct method but can lead to performance issues and mutations of the original object. Setting properties to undefined
or null
is useful when you want to retain the object’s structure. Object destructuring with rest syntax offers a modern, immutable way to remove properties, while Reflect.deleteProperty
provides a more formalized method.
When you need to remove multiple properties, object destructuring with rest syntax or looping through the properties with the delete
operator can be effective solutions. Each method has its place, and the best choice depends on your specific use case. Understanding the advantages and pitfalls of each approach allows you to make informed decisions, leading to more efficient and maintainable JavaScript code.