How to Remove Elements from a JavaScript Array
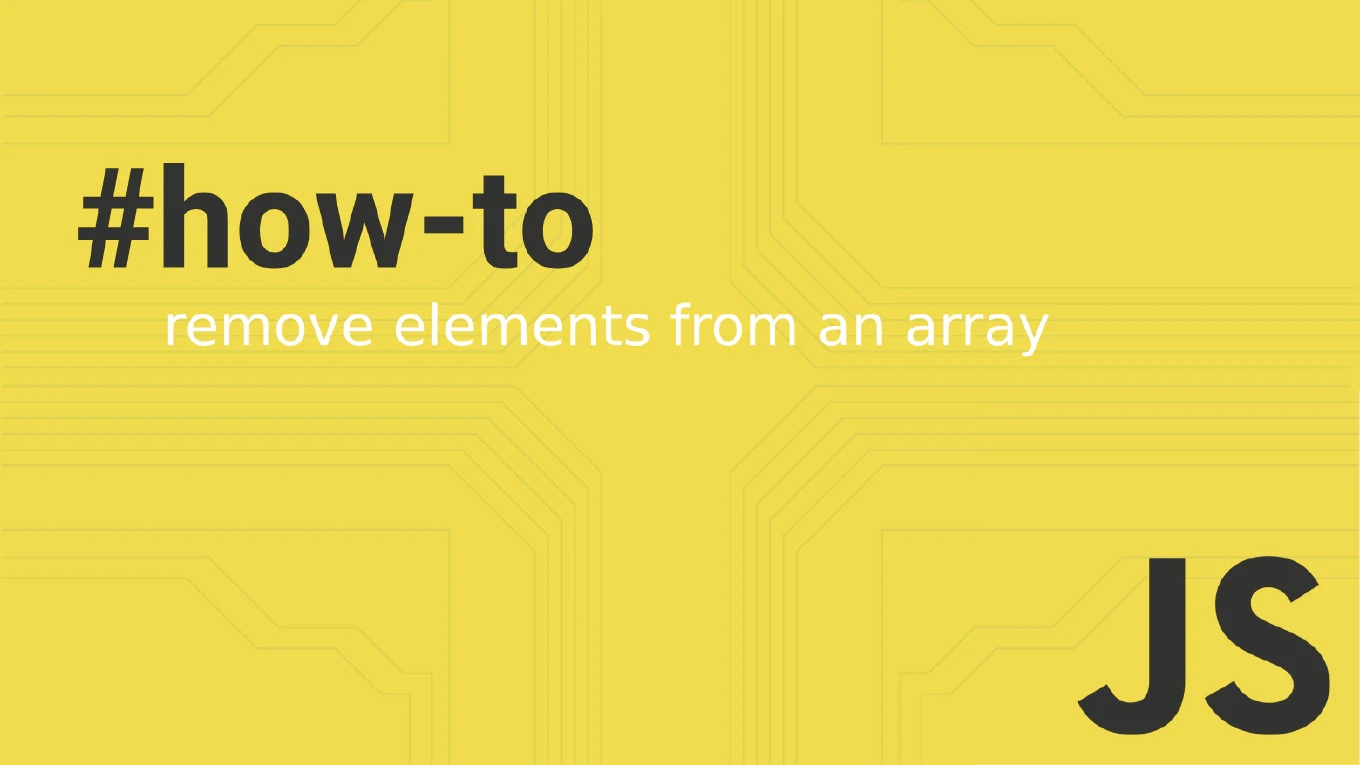
Removing elements from an array in JavaScript is a fundamental skill every developer should master. Whether you need to remove the first element, remove the last element, or filter out unwanted elements based on a specified value, understanding multiple techniques to remove array elements ensures your code remains clean and efficient. In this post, we will explore a few methods to remove element from array in javascript, explain how they work on the original array or when they create a new array, and show you the potential pitfalls.
Speed up your responsive apps and websites with fully-featured, ready-to-use open-source admin panel templates—free to use and built for efficiency.
Key Concepts and Different Methods
1. The splice
Method
One of the most popular ways to remove elements from an array in JavaScript is the splice
method. This method modifies the original array directly. It takes at least two arguments (the start index and the number of elements to remove). You can also insert new elements with the same method, making it highly versatile.
// simple example
const fruits = ['apple', 'banana', 'cherry', 'date']
// Remove 1 element of the array starting at index 1
const removedElements = fruits.splice(1, 1)
console.log(removedElements) // ['banana']
console.log(fruits) // ['apple', 'cherry', 'date']
splice
method usage count: It’s often the go-to method to remove array elements in place.- It can return the removed elements, which is helpful if you need to keep track of those items.
- The
splice
method can also add new elements, making it a multi-purpose approach.
2. The pop
Method
The pop
method removes the last element from a given array. This method removes a single item from the end of the array and returns that removed element. Because the pop
method modifies the original array, you should note that the array length reduced by one.
const arr = ['red', 'green', 'blue']
const removedElement = arr.pop() // pop method removes the last element
console.log(removedElement) // 'blue'
console.log(arr) // ['red', 'green']
- This is the quickest method to remove the last element if all the elements beyond your target are already out of scope.
- The return value is the removed element.
3. The shift
Method
When you need to remove the first element, consider the shift
method. The shift
method removes the first array element, shifting all the elements console output if you log the array afterwards. Because this method removes from the front, every subsequent element is re-indexed, and the length of the array is updated accordingly.
const colors = ['purple', 'orange', 'pink']
const firstArrayElement = colors.shift() // shift method removes the first element
console.log(firstArrayElement) // 'purple'
console.log(colors) // ['orange', 'pink']
- Use
shift method
carefully, especially when you rely on the current array index, since re-indexing can complicate your logic.
4. The filter
Method
If you want to create a new array instead of mutating the original array, the filter
method is a powerful choice. This method creates a new array containing all the elements that match a condition, so it’s a great way to exclude a particular element or a set of unwanted elements from your input array.
const numbers = [1, 2, 3, 4, 5]
const filtered = numbers.filter(num => num !== 3) // remove an element with specified value
console.log(filtered) // [1, 2, 4, 5]
console.log(numbers) // [1, 2, 3, 4, 5] (original array is unchanged)
- The
filter
method allows you to preserve existing elements that meet your condition. - Because it creates a new array, your original array is left intact.
5. The delete
operator
Some developers use the delete
operator to delete element directly from an array. However, this approach leaves an empty slot in the array, rather than collapsing its indices. You may end up with an empty array index that can cause confusion when checking array length or iterating with a while loop.
const items = ['keyboard', 'mouse', 'monitor']
delete items[1]
console.log(items) // ['keyboard', empty, 'monitor']
- The
delete
operator does not affect the length property of the array. - This is rarely the best method to remove array elements because it can complicate subsequent elements and hamper clarity in your code.
Best Practices and Case Study
In many real-world applications dealing with JavaScript objects and arrays, you might need to remove array elements based on user input or a dynamically generated condition. By using methods like splice
or filter
, you can handle different methods of removal gracefully. For instance, if you must remove an element of the array with a specified value, consider filter
. If you just want to remove the first element or remove the last element quickly, reach for shift
or pop
.
A practical scenario is filtering items in an online store’s shopping cart. If the user selects a particular element to delete, the app could rely on splice
method (for immediate mutation) or filter
method (to create a new array and maintain the old state). Always keep track of the array length and verify the new length after each operation to avoid off-by-one errors, especially when indexing remaining elements.
Additionally, libraries often provide convenient abstractions for removing elements from an array in JavaScript. You can also rely on the array prototype and array splice functionality behind the scenes for custom remove method implementations. Be mindful of negative index usage in some scenarios—though standard array methods like splice
interpret negative indices differently than Python or Ruby.
Summary
Removing elements from an array in JavaScript can be tackled in various ways. Some methods, like pop
and shift
, target just one element. Others, like splice
and filter
, offer more fine-grained control, from removing elements in place to creating a brand-new collection. By exploring the return value and effects on the length of the array, you can pick the right method to remove for your use case. Remember to avoid the delete
operator in most situations, and keep an eye on the remaining elements after any modification.
Next Steps
- Experiment with each method to remove array elements on your own projects.
- Explore advanced techniques with the rest operator for merging and removing elements console outputs in unique ways.
With these techniques in hand, you’ll be able to handle any scenario involving removing elements from an array, whether you’re manipulating a large dataset or simply trimming down a small list for a front-end feature.