How to Remove the Last Character from a String in JavaScript
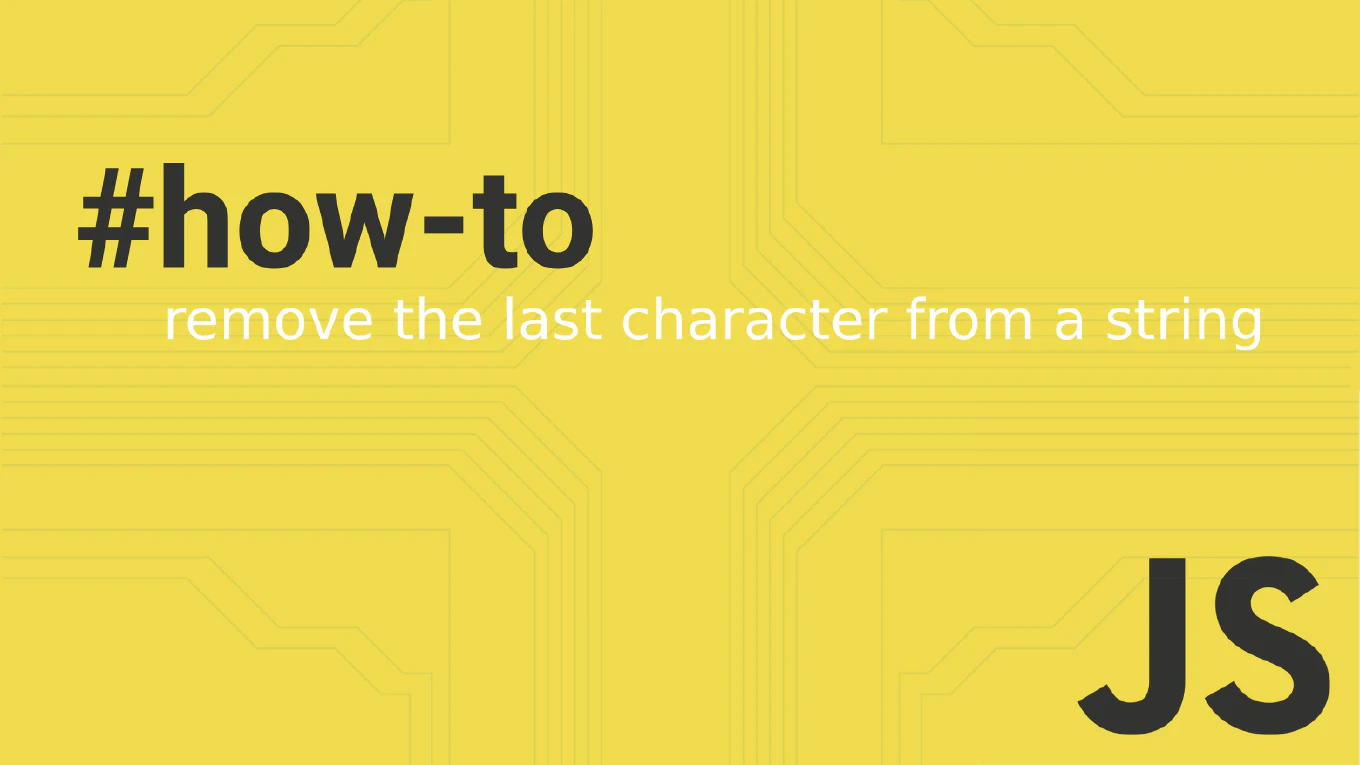
Removing the last character from a string in JavaScript is a common task, whether you’re dealing with user input or formatting strings. JavaScript provides several methods to achieve this, including slice()
, substring()
, and replace()
. This article will cover these methods in detail, ensuring you can effectively remove the last character from a string.
Speed up your responsive apps and websites with fully-featured, ready-to-use open-source admin panel templates—free to use and built for efficiency.
Using the slice()
Method
The slice()
method is straightforward to remove the last character from a string. This method extracts a section of a string and returns it as a new string without modifying the original string.
const str = 'Hello World'
const newStr = str.slice(0, -1)
console.log(newStr) // Output: 'Hello Worl'
Here, slice(0, -1)
removes the last character because 0 indicates the start index and -1
indicates the last index (final character).
Using the substring()
Method
The substring()
method is another way to remove the last character from a string. Unlike slice()
, the substring()
method does not support negative index. Instead, you specify the length of the string minus one to exclude the last character.
const str = 'Hello World'
const newStr = str.substring(0, str.length - 1)
console.log(newStr) // Output: 'Hello Worl'
In this example, substring(0, str.length - 1)
removes the last character by using the length of the string minus one as the end index.
Using the replace()
Method
The replace()
method can also remove the last character by using a regular expression. This method is useful if you need to conditionally remove the last character based on its value.
const str = 'Hello World'
const newStr = str.replace(/.$/, '')
console.log(newStr) // Output: 'Hello Worl'
The regular expression /.
matches any character, and $
indicates the end of the string, effectively removing the last character.
Removing the Last Character Conditionally
If you need to remove the last character only if it meets certain conditions (e.g., it’s a comma or whitespace), you can extend the replace()
method with specific patterns.
const str = 'Hello World,'
const newStr = str.replace(/,$/, '')
console.log(newStr) // Output: 'Hello World'
In this example, replace(/,$/, '')
removes the last character only if it’s a comma.
Using substr()
Method
Although less common, the substr()
method can also remove the last character. This method is considered deprecated but is still found in legacy code.
const str = 'Hello World'
const newStr = str.substr(0, str.length - 1)
console.log(newStr) // Output: 'Hello Worl'
Benchmark Results
Benchmarking different methods to remove the last character from a string shows varying performance levels. Below are the benchmark results:
substring()
(15,426,000 operations per second) 🏆 - 100%slice()
(15,407,415 operations per second) - 99.88%substr()
(14,796,091 operations per second) - 95.92%replace()
(7,968,516 operations per second) - 51.66%
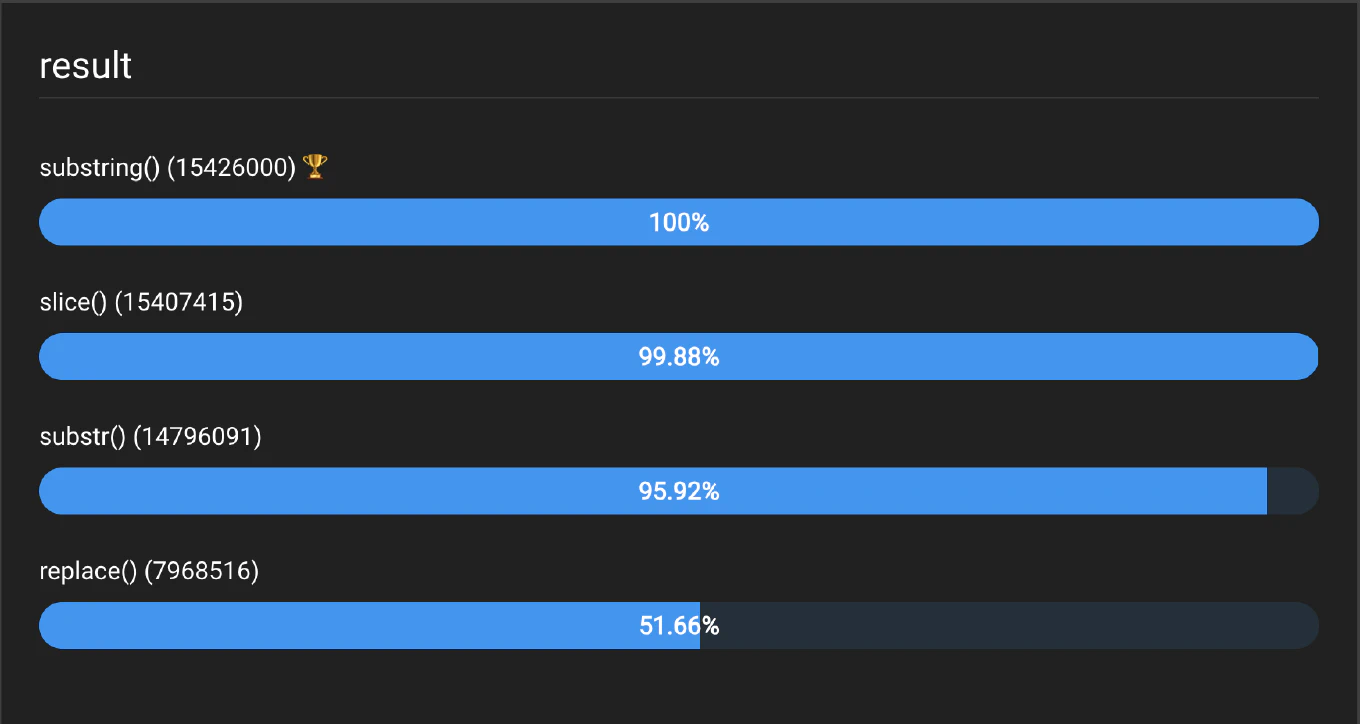
Conclusion
JavaScript offers multiple ways to remove the last character from a string. The slice()
method is generally preferred due to its simplicity and support for negative indexing. The substring()
and replace()
methods provide alternative approaches that can be useful in different scenarios. Understanding these methods allows you to choose the most appropriate one for your specific use case.
By mastering these methods, you’ll be well-equipped to handle string manipulations in your JavaScript projects effectively. Whether you need a modified string or want to ensure the integrity of the original string, these techniques will help you achieve the desired outcome efficiently.