How to replace all occurrences of a string in JavaScript?
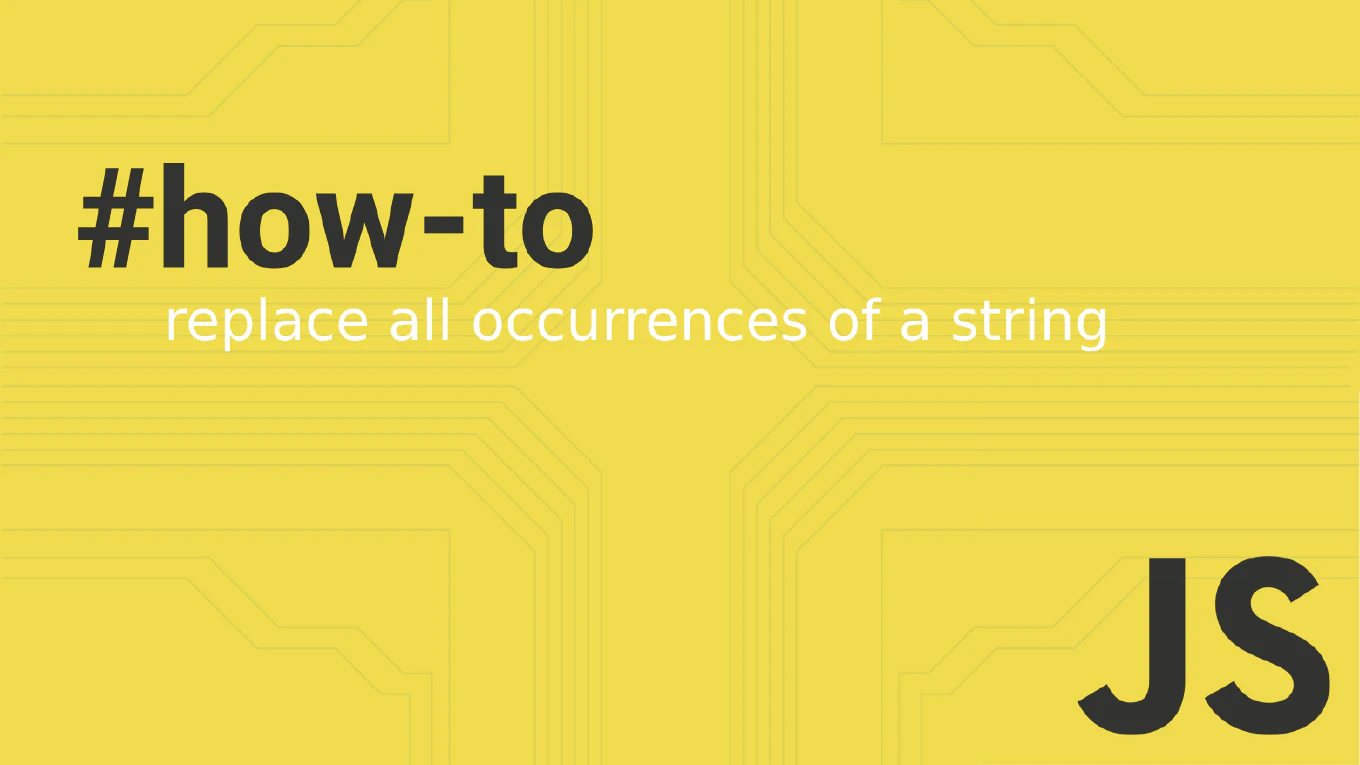
When working with JavaScript, you often must replace all occurrences of a specific substring within a string. This is a common task in various scenarios, such as formatting user input, modifying text content, or processing data. However, the native replace
method in JavaScript only replaces the first occurrence of the pattern by default. This article will guide you through different techniques to replace all occurrences of a string in JavaScript effectively.
Speed up your responsive apps and websites with fully-featured, ready-to-use open-source admin panel templates—free to use and built for efficiency.
Understanding the replace
Method
The replace
method in JavaScript is used to replace a pattern in a string with a new substring. However, by default, this method searches for the pattern and replaces only the first occurrence of it:
const str = 'hello world, hello universe'
const newStr = str.replace('hello', 'hi')
console.log(newStr) // 'hi world, hello universe'
In the example above, only the first occurrence of ‘hello’ is replaced, leaving the second occurrence unchanged. If you want to replace all occurrences of ‘hello’ with ‘hi’, you’ll need to use other methods.
Using a Regular Expression with the g
Flag
To ensure all occurrences of a substring are replaced, utilize a regular expression with the global flag (g). This approach ensures that every match of the search pattern is replaced:
Example: Using replace
with a Global Regular Expression
const str = 'hello world, hello universe'
const newStr = str.replace(/hello/g, 'hi')
console.log(newStr) // 'hi world, hi universe'
In this example, the global regular expression /hello/g
matches all instances of ‘hello’, ensuring that every occurrence is replaced with ‘hi’. This is one of the most common ways to replace multiple occurrences of a substring in JavaScript.
Handling Special Characters in Regular Expressions
When using a regular expression, special characters like .
or *
have special meanings. If your search string contains these characters, you’ll need to escape them using a backslash (\
):
const str = 'a.b.c.d'
const newStr = str.replace(/\./g, '-')
console.log(newStr) // 'a-b-c-d'
Here, the dot (.
) is escaped to treat it as a literal character rather than its default behavior of matching any character.
Replacing All Occurrences Without Using a Regular Expression
If you prefer not to use regular expressions, there are other methods to achieve the same result. One such approach is using split()
and join()
.
Example: Using split
and join
to Replace All Occurrences
const str = 'hello world, hello universe'
const newStr = str.split('hello').join('hi')
console.log(newStr) // 'hi world, hi universe'
In this approach:
- The
split
method breaks the original string into an array based on the search pattern. - The
join
method then combines the array elements back into a new string with the replacement string.
This method returns a new string with all instances of the search pattern replaced.
Using the replaceAll
Method
With ECMAScript 2021 (ES12), JavaScript introduced the replaceAll
method, which simplifies the process of replacing all occurrences of a substring:
const str = 'hello world, hello universe'
const newStr = str.replaceAll('hello', 'hi')
console.log(newStr) // 'hi world, hi universe'
The replaceAll
method searches for all instances of the search pattern and replaces them with the new substring, making it a straightforward solution. However, it’s important to note that replaceAll
is not supported in older browsers.
Performance Considerations
When dealing with large strings or performance-critical code, consider the following:
- Regular expressions are powerful but may introduce overhead.
split
andjoin
are simple and effective for straightforward replacements.replaceAll
is the most user-friendly but requires compatibility with modern JavaScript environments.
Conclusion
Replacing all occurrences of a string in JavaScript can be accomplished using several methods:
- Regular expressions with the global flag (
g
) provide flexibility and power. split
andjoin
offer a non-regex alternative.replaceAll
is the most modern and intuitive solution, but it requires support from major browsers.
By understanding these techniques, you can choose the best approach for your specific needs, ensuring your string manipulation tasks in JavaScript are both efficient and effective.