How to show or hide elements in React? A Step-by-Step Guide.
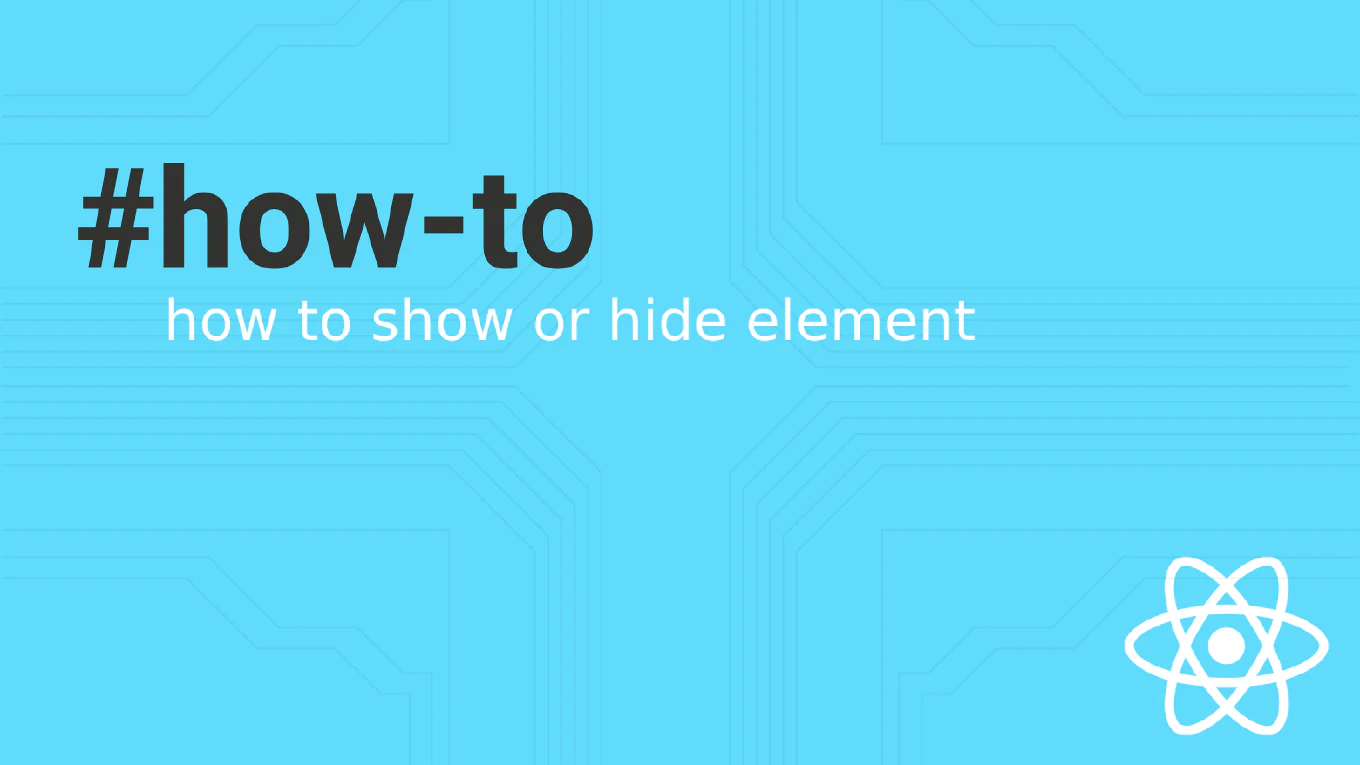
In modern web development, creating dynamic user interfaces is essential. The React library, a popular JavaScript tool, allows developers to build rich user interfaces by efficiently updating and rendering components based on data changes. One common requirement is to show or hide elements in React applications based on user interaction or application state. This article will guide you through different methods to show or hide elements in React using functional components, focusing on React 18+.
Speed up your responsive apps and websites with fully-featured, ready-to-use open-source admin panel templates—free to use and built for efficiency.
Understanding State in Functional Components
To manage the visibility of elements, we’ll use state variables in our functional components. The useState
hook, a built-in hook in React, lets you add state to functional components without converting them into class components. This helps in creating dynamic and responsive interfaces.
import React, { useState } from 'react'
function App() {
const [isVisible, setIsVisible] = useState(true)
return (
<div>
{isVisible && <p>This element is visible.</p>}
<button onClick={() => setIsVisible(!isVisible)}>
Show or Hide
</button>
</div>
)
}
export default App
In the example code above, we import React and useState
at the beginning. The function App
component maintains a state variable isVisible
. The setIsVisible
function toggles the boolean value of isVisible
, effectively showing or hiding the element.
Conditional Rendering Techniques
Using the Ternary Operator
The ternary operator is a concise way to conditionally render elements in React components. It allows you to show or hide elements based on a boolean value in your component state.
function MyComponent() {
const [showElement, setShowElement] = useState(false)
return (
<div>
{showElement ? <p>Element is shown.</p> : <p>Element is hidden.</p>}
<button onClick={() => setShowElement(!showElement)}>
Toggle Element
</button>
</div>
)
}
In this code snippet, the function MyComponent
uses the ternary operator to decide which element to render based on the showElement
state variable.
Returning Null
Another way to hide elements is by conditionally returning null
in the render method of a functional component.
function ChildComponent({ isVisible }) {
if (!isVisible) {
return null
}
return <div>This is a child component.</div>
}
The ChildComponent
will render its content only if isVisible
is true
. Otherwise, it returns null
, and React will not render anything for this component.
Managing Multiple Elements
When dealing with multiple elements or child components, you can manage their visibility using multiple state variables.
function ParentComponent() {
const [showFirst, setShowFirst] = useState(true)
const [showSecond, setShowSecond] = useState(false)
const [showThird, setShowThird] = useState(true)
return (
<div>
{showFirst && <FirstChild />}
{showSecond && <SecondChild />}
{showThird && <ThirdChild />}
{/* Buttons to toggle each child component */}
</div>
)
}
In this example, the ParentComponent
controls the visibility of three child components using three different boolean variables. This approach is useful when you need to show or hide multiple elements independently.
React Show Hide Div on Click
A common scenario is to show or hide a div
when a user clicks a button. This can be achieved using a state variable and an event handler.
import React, { useState } from 'react'
function ToggleDiv() {
const [isVisible, setIsVisible] = useState(false)
function handleClick() {
setIsVisible(!isVisible)
}
return (
<div>
<button onClick={handleClick}>Toggle Div</button>
{isVisible && <div>This div is now visible.</div>}
</div>
)
}
In the function ToggleDiv
, we import React and useState
. The handleClick
function toggles the isVisible
state variable, which controls the rendering of the div
element.
Creating a Custom React Hook for Toggling Visibility
To avoid code repetition, you can create a custom React hook to manage the visibility state. This makes your code reusable and cleaner, especially when multiple components need similar functionality.
Creating the Custom Hook
import { useState } from 'react'
function useToggle(initialValue = false) {
const [isVisible, setIsVisible] = useState(initialValue)
function toggle() {
setIsVisible(prevState => !prevState)
}
return [isVisible, toggle]
}
The useToggle
hook takes an optional initialValue
parameter, which defaults to false
. It returns an array containing the current visibility state (isVisible
) and a function (toggle
) that inverts the state.
Using the Custom Hook
Now that we have our useToggle
hook, let’s use it in a functional component to manage the visibility of an element.
import React from 'react'
import useToggle from './useToggle'
function ToggleComponent() {
const [isVisible, toggleVisibility] = useToggle()
return (
<div>
<button onClick={toggleVisibility}>Toggle Visibility</button>
{isVisible && <div>This component is visible.</div>}
</div>
)
}
export default ToggleComponent
In this ToggleComponent
, we import our custom useToggle
hook. By using useToggle
, we get a clean and reusable solution for toggling visibility. The toggleVisibility
function handles changing the visibility state, making the code much simpler and easier to maintain.
Comparing Functional and Class Components
Before the introduction of hooks, class components were used to manage state and lifecycle methods in React applications. A typical class App extends React.Component
would look like this:
import React from 'react'
class App extends React.Component {
constructor(props) {
super(props)
this.state = {
isVisible: true,
}
this.toggleVisibility = this.toggleVisibility.bind(this)
}
toggleVisibility() {
this.setState((prevState) => ({
isVisible: !prevState.isVisible,
}))
}
render() {
return (
<div>
{this.state.isVisible && <p>This element is visible.</p>}
<button onClick={this.toggleVisibility}>
Show or Hide
</button>
</div>
)
}
}
export default App
In this class App
, we manage the component state using this.state
and update it using this.setState()
. However, with the advent of hooks, functional components can now handle state and lifecycle methods, making them more concise and easier to read.
Using CSS to Hide Elements
You can also hide elements by manipulating the style attribute or applying a CSS class.
function ToggleComponent() {
const [isHidden, setIsHidden] = useState(false)
return (
<div>
<div style={{ display: isHidden ? 'none' : 'block' }}>
This element can be hidden.
</div>
<button onClick={() => setIsHidden(!isHidden)}>
Show or Hide
</button>
</div>
)
}
Here, the display property is used to hide the element in the DOM. This method affects the CSS of the HTML element directly.
Conclusion
Managing the visibility of elements in React applications is straightforward with the use of state variables and conditional rendering. Whether you’re using the ternary operator, returning null
, manipulating the style attribute, or creating a custom hook, React’s functional components provide flexible ways to show or hide elements based on the current state.
By mastering these techniques, you can create dynamic and responsive user interfaces that enhance the user experience in real-world examples.