The Wacky World of JavaScript: Unraveling the Oddities
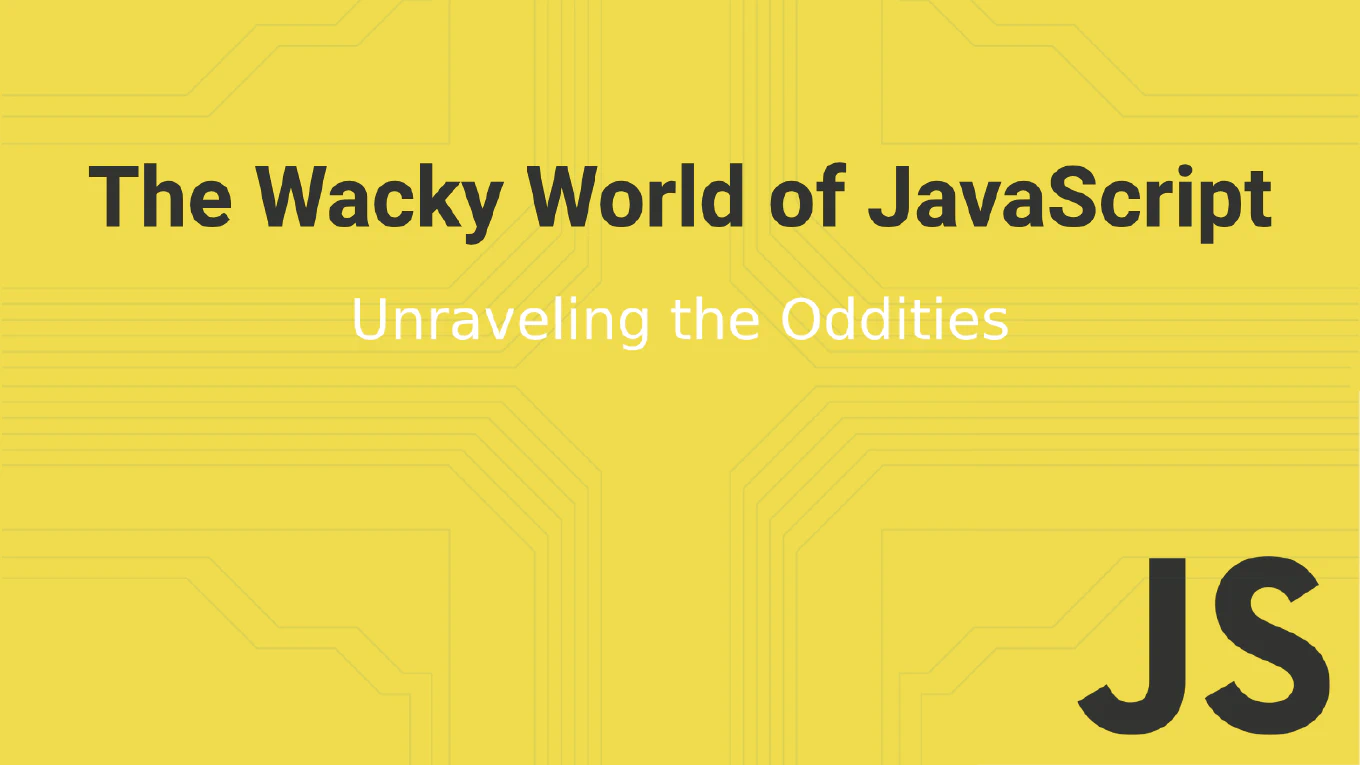
JavaScript, the ubiquitous language of the web, is known for its flexibility and, at times, its perplexing behavior. Let’s dive into some of the more peculiar aspects of JavaScript that can leave both novice and experienced developers scratching their heads.
NaN is a Number?
typeof NaN // "number"
Despite its name, NaN
(Not-a-Number) is considered a type of number in JavaScript. This can lead to some confusing situations, but it’s important to remember that typeof NaN
returns "number"
.
Big Numbers Get Rounded
9999999999999999 // 10000000000000000
JavaScript uses double-precision floating-point format numbers, which can cause large integers to be rounded. Here, 9999999999999999
gets rounded to 10000000000000000
.
Floating-Point Arithmetic
0.5 + 0.1 == 0.6 // true
0.1 + 0.2 == 0.3 // false
Due to floating-point precision, 0.1 + 0.2
does not exactly equal 0.3
. Instead, it results in 0.30000000000000004
, making the comparison to 0.3
false.
Math Functions
Math.max() // -Infinity
Math.min() // Infinity
Calling Math.max()
without arguments returns -Infinity
, while Math.min()
returns Infinity
. This is a quirky yet predictable behavior when no parameters are provided.
Adding Arrays and Objects
[] + [] // ""
[] + {} // "[object Object]"
{ } + [] // 0
- Adding two empty arrays results in an empty string due to type coercion.
- Adding an empty array to an empty object gives
"[object Object]"
. { } + []
is interpreted as a block followed by an array, resulting in0
.
True Equals One
true == 1 // true
true === 1 // false
true
is loosely equal to1
due to type coercion.- However,
true
is not strictly equal to1
because they are of different types.
Length of a Weird String
(![] + [] + ![]).length // 10
Here’s a breakdown:
![]
isfalse
, which coerces to the string"false"
.- Concatenating
false
with an empty array results in"false"
. - Adding another
false
gives"falsefalse"
. - The length of
"falsefalse"
is10
.
Mixing Numbers and Strings
9 + "1" // "91"
91 - "1" // 90
- Adding a number and a string results in string concatenation (
"91"
). - Subtracting a string from a number coerces the string to a number, allowing the arithmetic operation (
90
).
Empty Array Equals Zero
[] == 0 // true
An empty array is loosely equal to 0
due to type coercion.
Adding and Subtracting Booleans
true + true + true === 3 // true
true - true // 0
true
is coerced to1
, so1 + 1 + 1
equals3
.- Subtracting
true
fromtrue
results in0
.
Conclusion
JavaScript’s quirks can be both amusing and baffling. Understanding these oddities is crucial for debugging and writing more predictable code. So, the next time you encounter a strange JavaScript behavior, remember that there’s often a logical (if not always intuitive) explanation behind it.
Happy coding!