What are the three dots `...` in JavaScript do?
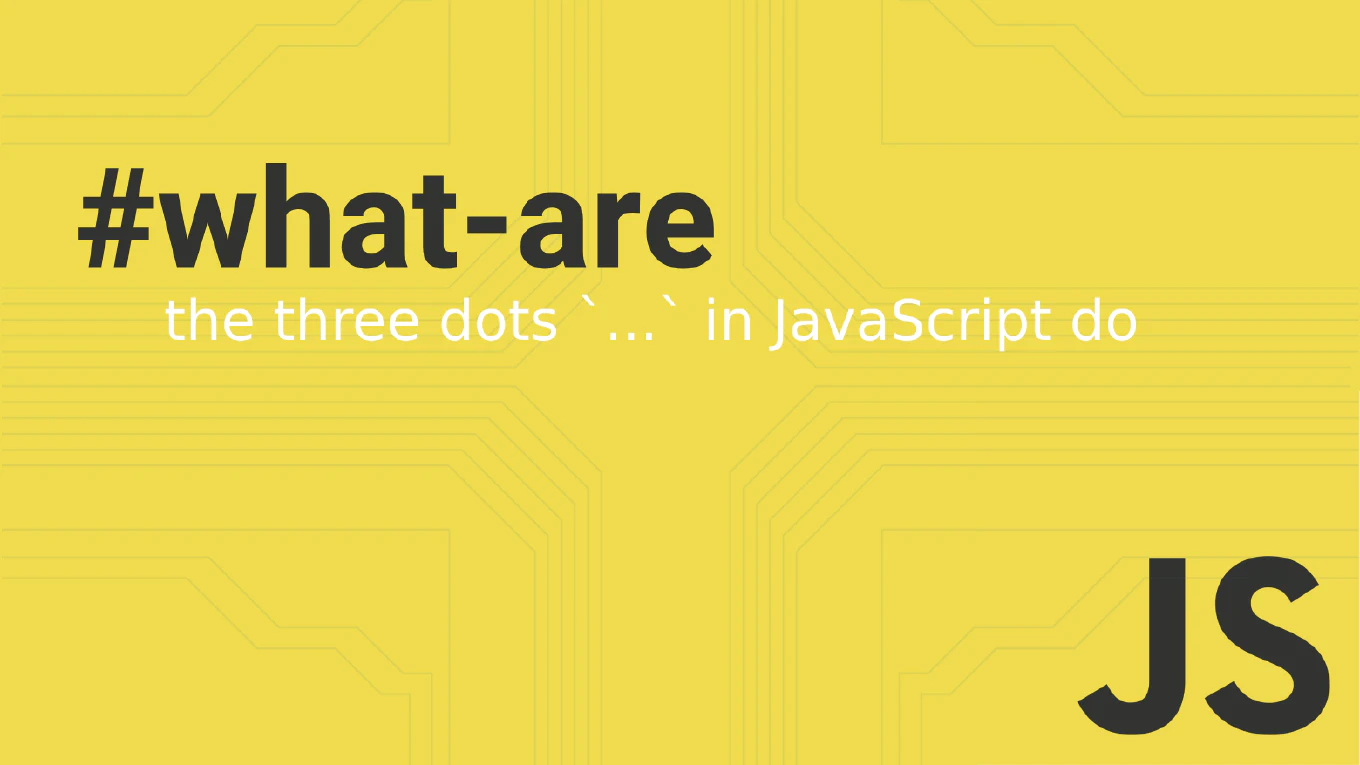
In JavaScript, the three dots operator (...
) is a versatile feature introduced in ES6. Known as the spread operator and rest parameter, these three dots have transformed how developers handle arrays, objects, and function parameters. Understanding this syntax is crucial for any software developer looking to write clean, efficient, and maintainable code. In this article, we’ll explore the various ways you can use the spread operator, spread syntax, and rest operator in your JavaScript code.
Speed up your responsive apps and websites with fully-featured, ready-to-use open-source admin panel templates—free to use and built for efficiency.
The Spread Operator or Spread Syntax in JavaScript
The spread operator allows you to expand elements of an array or object into individual elements. This is especially useful for tasks like merging objects, copying arrays, or passing a variable number of arguments to a function. Mastering the spread operator syntax can simplify your code and make it more readable.
Expanding an Array
const numbers = [1, 2, 3]
const moreNumbers = [...numbers, 4, 5, 6]
console.log(moreNumbers) // Output: [1, 2, 3, 4, 5, 6]
In this example, the spread operator ...numbers
expands the numbers
array into its individual elements, which are then combined with 4, 5, 6
to form a new array.
Copying an Object
const person = { name: 'John', age: 30 }
const newPerson = { ...person, age: 35 }
console.log(newPerson) // Output: { name: 'John', age: 35 }
Here, the spread syntax is used to create a shallow copy of the person
object. The original object remains unchanged, while the age
property in the new object is updated.
The Rest Parameter and Rest Operator
The rest parameter works in the opposite way to the spread operator. It allows you to gather multiple elements into a single array or object, which is particularly useful in functions where you don’t know the exact number of arguments in advance.
Using Rest Parameters in Functions
function sum(...args) {
return args.reduce((total, current) => total + current, 0)
}
console.log(sum(1, 2, 3, 4)) // Output: 10
In this example, the rest operator collects all arguments passed to the sum
function into an array called args
. The reduce
method then sums all the values in the array.
Practical Applications of the Spread Operator
The spread syntax have many practical applications, especially when working with arrays and objects. Some of the most common uses include:
- Merging Objects: Combine properties from multiple objects into one object.
- Expanding Arrays: Use the spread operator to expand arrays into individual elements.
- Function Calls: Pass an array as individual arguments to a function using the spread operator.
Merging Objects
const obj1 = { a: 1, b: 2 }
const obj2 = { c: 3, d: 4 }
const mergedObj = { ...obj1, ...obj2 }
console.log(mergedObj) // Output: { a: 1, b: 2, c: 3, d: 4 }
In this example, obj1
and obj2
are combined into a final object using the spread operator.
Merging Arrays
The spread operator is also incredibly useful for merging arrays. Instead of using traditional methods like concat
, you can merge arrays cleanly and efficiently with the spread operator.
const arr1 = [1, 2, 3]
const arr2 = [4, 5, 6]
const mergedArr = [...arr1, ...arr2]
console.log(mergedArr) // Output: [1, 2, 3, 4, 5, 6]
Here, arr1
and arr2
are merged into one array, mergedArr
, using the spread operator. This method is not only concise but also easy to read and maintain.
Using the Spread Operator in Function Calls
function myFunc(x, y, z) {
console.log(x, y, z)
}
const args = [0, 1, 2]
myFunc(...args) // Output: 0 1 2
Here, the spread operator expands the args
array into individual arguments, making it easier to handle function parameters dynamically.
Conclusion
Understanding the three dots operator—whether as a spread operator or a rest operator—is essential for any software developer working with JavaScript. It provides a more intuitive way to handle arrays, objects, and function parameters. Whether you’re merging arrays, creating shallow copies of objects, or managing variable numbers of arguments, the spread and rest operators are indispensable tools in modern JavaScript development.
By incorporating these concepts into your code, you can write more flexible and efficient programs that are easier to maintain and understand.