JavaScript printf equivalent
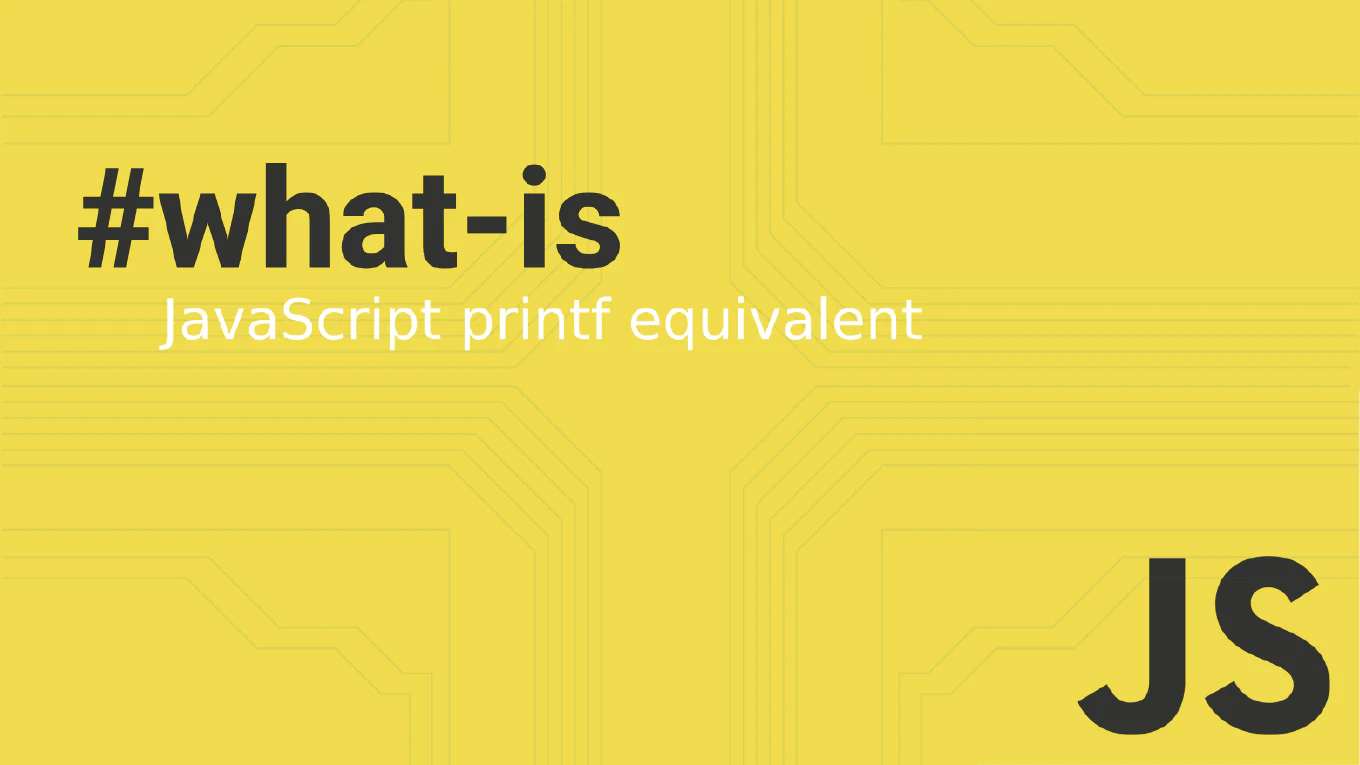
In JavaScript, there’s no built-in printf function, but various methods can achieve the same result for string formatting. Let’s explore how to format strings using template literals and custom functions.
Template literals (Template strings)
Template literal in JavaScript allows embedding variables and expressions directly within strings, offering a powerful alternative to traditional string concatenation.
const name = 'Alice'
const age = 30
console.log(`My name is ${name} and I am ${age} years old`) // My name is Alice and I am 30 years old
Custom format Function
To replicate printf functionality, you can create a custom format method. This method uses the replace function with regular expressions to substitute placeholders with arguments.
String.prototype.format = function(...args) {
return this.replace(/{(\d+)}/g, (match, number) => {
return typeof args[number] !== 'undefined' ? args[number] : match
})
}
const formatString = 'My name is {0} and I am {1} years old'
console.log(formatString.format('Alice', 30)) // My name is Alice and I am 30 years old
Using console.log with Multiple Arguments
A straightforward method is using console.log with multiple arguments for basic string concatenation.
const name = 'Alice'
const age = 30
console.log('My name is', name, 'and I am', age, 'years old') // My name is Alice and I am 30 years old
Practical Examples
Simple String Interpolation
Using template literals for string interpolation:
const product = 'book'
const price = 9.99
console.log(`The price of the ${product} is $${price}`) // The price of the book is $9.99
Custom printf Function
A versatile custom function can be created for varied use cases:
function printf(format, ...args) {
return format.replace(/{(\d+)}/g, (match, number) => {
return typeof args[number] !== 'undefined' ? args[number] : match
})
}
const result = printf('The value of {0} plus {1} is {2}', 3, 4, 3 + 4)
console.log(result) // The value of 3 plus 4 is 7
Handling More Complex Formatting
For more sophisticated formatting needs, such as controlling decimal places or padding, template literals and custom functions can be extended. Here’s an example of formatting a number to two decimal places:
const number = 1234.56789
console.log(`The formatted number is ${number.toFixed(2)}`) // The formatted number is 1234.57
Conclusion
Although JavaScript has no direct printf equivalent, it provides several methods to achieve similar string formatting functionality. Developers can effectively format strings in JavaScript by leveraging template literals, custom format functions, and the versatile console.log. These techniques enhance code readability and maintain flexibility across different formatting scenarios.
You can define custom string formatting functions using the String.prototype.format method in JavaScript. This method returns formatted strings based on placeholders within the string. Regular expressions and the replace method are essential for achieving this functionality. By understanding and implementing these methods, you can effectively replicate the printf function in JavaScript.