Understanding the Difference Between NPX and NPM
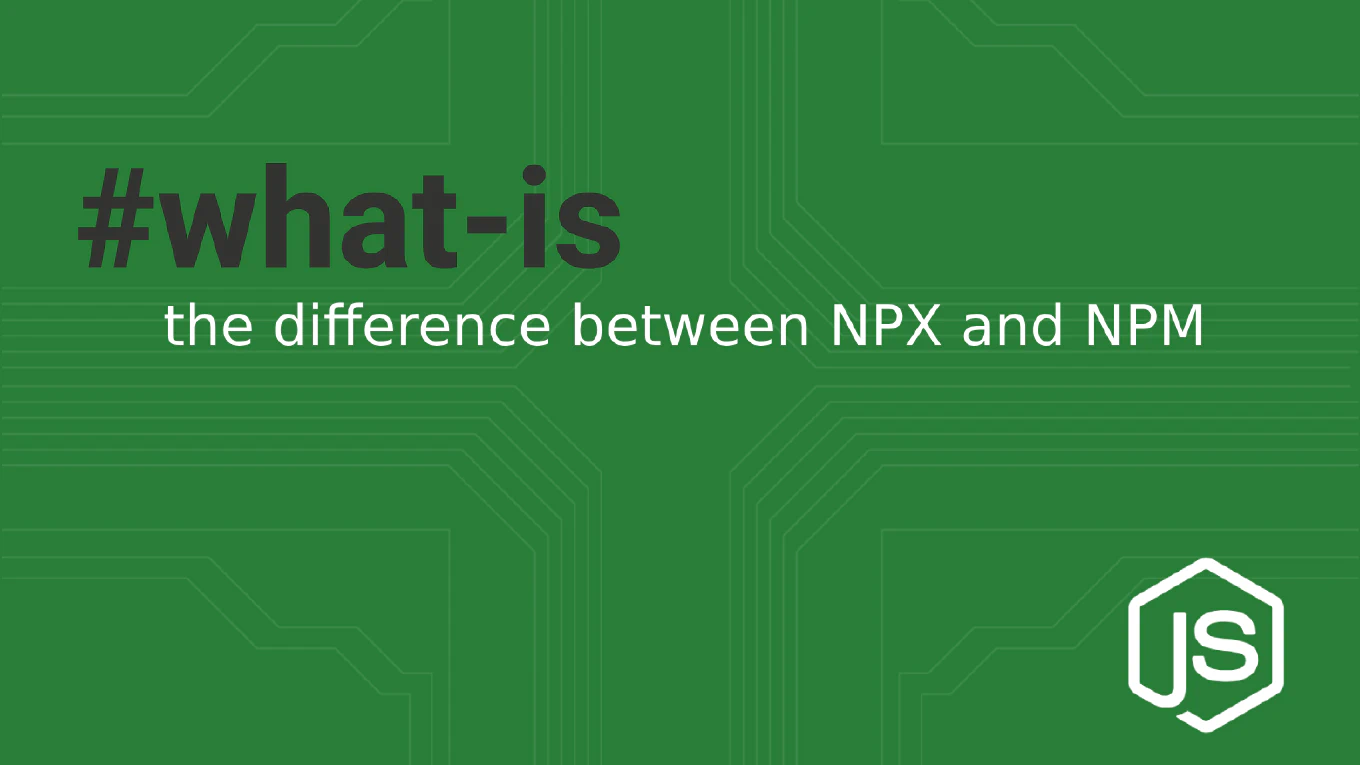
In the ever-evolving landscape of JavaScript development, managing packages efficiently is crucial. Whether you’re a seasoned developer or just starting, understanding the tools at your disposal can significantly impact your workflow. Two such essential tools are npm and npx. While both are integral to the Node Package Manager ecosystem, they serve distinct purposes. This article delves into the difference between npm and npx, elucidating their roles, best practices, and practical applications to enhance your development experience.
Speed up your responsive apps and websites with fully-featured, ready-to-use open-source admin panel templates—free to use and built for efficiency.
Introduction
Package management is a cornerstone of modern JavaScript development. With thousands of packages available in the npm registry, developers rely on tools like npm and npx to install, manage, and execute these packages seamlessly. However, the difference between npm and npx often confuses many. Grasping this distinction is vital for efficient package management, avoiding dependency issues, and optimizing your development workflow. By the end of this article, you’ll have a clear understanding of when and how to use each tool effectively.
Understanding NPX and NPM
What is NPM?
npm, short for Node Package Manager, is the default package manager for Node.js. It facilitates the installation, updating, and management of JavaScript packages and their dependencies. As a package manager, npm simplifies the process of incorporating third-party libraries into your projects, ensuring that all necessary project dependencies are handled efficiently.
Key Features of npm:
- Install packages globally or locally: Use
npm install -g
for global installations ornpm install
for project-specific dependencies. - Manage package versions: Handle different versions of packages to maintain dependency management.
- Access to the npm registry: A vast repository of JavaScript packages available for installation.
What is NPX?
npx is a tool that comes bundled with npm (since version 5.2.0) and serves as an npm package runner. It allows developers to execute Node packages without the need for a global installation. This means you can run JavaScript packages directly from the npm registry without polluting your global package installation space.
Key Features of npx:
- Execute packages without installation: Run packages directly, saving disk space and avoiding package pollution.
- Use specific package versions: Easily execute different versions of the same package without conflicts.
- Simplify command execution: Ideal for running one-off commands or scripts.
Best Practices for Using NPM and NPX
When to Use NPM
-
Managing Project Dependencies: Use
npm install
to add packages to your project, ensuring they’re listed in yourpackage.json
file for consistent dependency management across environments.npm install react
-
Global Package Installation: For tools you use across multiple projects, such as
create-react-app
, install them globally.npm install -g create-react-app
When to Use NPX
-
Running One-Off Commands: Instead of installing a package globally, execute it temporarily using npx.
npx create-react-app my-app
-
Avoiding Version Conflicts: Execute a specific version of a package without affecting global installations.
Best Practices
-
Prefer Local Installations: Whenever possible, install packages locally to maintain project-specific dependencies and avoid conflicts.
npm install lodash
-
Use NPX for Temporary Tasks: Utilize npx for tasks that don’t require persistent installations, keeping your global namespace clean.
-
Regularly Update Packages: Keep your npm packages up-to-date to benefit from the latest features and security patches.
Practical Examples
Using NPM to Install and Manage Packages
# Initialize a new Node.js project
npm init -y
# Install React locally
npm install react react-dom
# Install a development dependency
npm install --save-dev webpack
Using NPX to Execute Packages
# Create a new React app without global installation
npx create-react-app my-new-app
# Run a specific version of a package
npx [email protected] .
Managing Dependencies with NPM
{
"dependencies": {
"react": "^17.0.2",
"lodash": "^4.17.21"
},
"devDependencies": {
"webpack": "^5.38.1"
}
}
Case Study: Streamlining Development Workflow with NPM and NPX
Consider a development team working on multiple React projects. By leveraging npm and npx, the team can maintain consistent dependency management while optimizing their workflow.
- Project Setup: Using
npx create-react-app
ensures each project starts with the latest React setup without the need for global installations. - Dependency Updates: npm manages project-specific dependencies, allowing the team to update packages without affecting other projects.
- Tool Execution: Developers use npx to run tools like webpack or eslint on a per-project basis, avoiding version conflicts and maintaining a clean global environment.
This approach not only enhances productivity but also minimizes potential issues related to package pollution and disk space usage.
Summary
Understanding the difference between npm and npx is essential for effective package management in JavaScript development. While npm excels in managing and installing project dependencies, npx offers a streamlined way to execute packages without permanent installations. By applying best practices such as preferring local installations and utilizing npx for temporary tasks, developers can maintain a clean and efficient development environment.
Further Steps
To deepen your understanding and enhance your development workflow, consider exploring the following resources: