How to convert a string to boolean in JavaScript
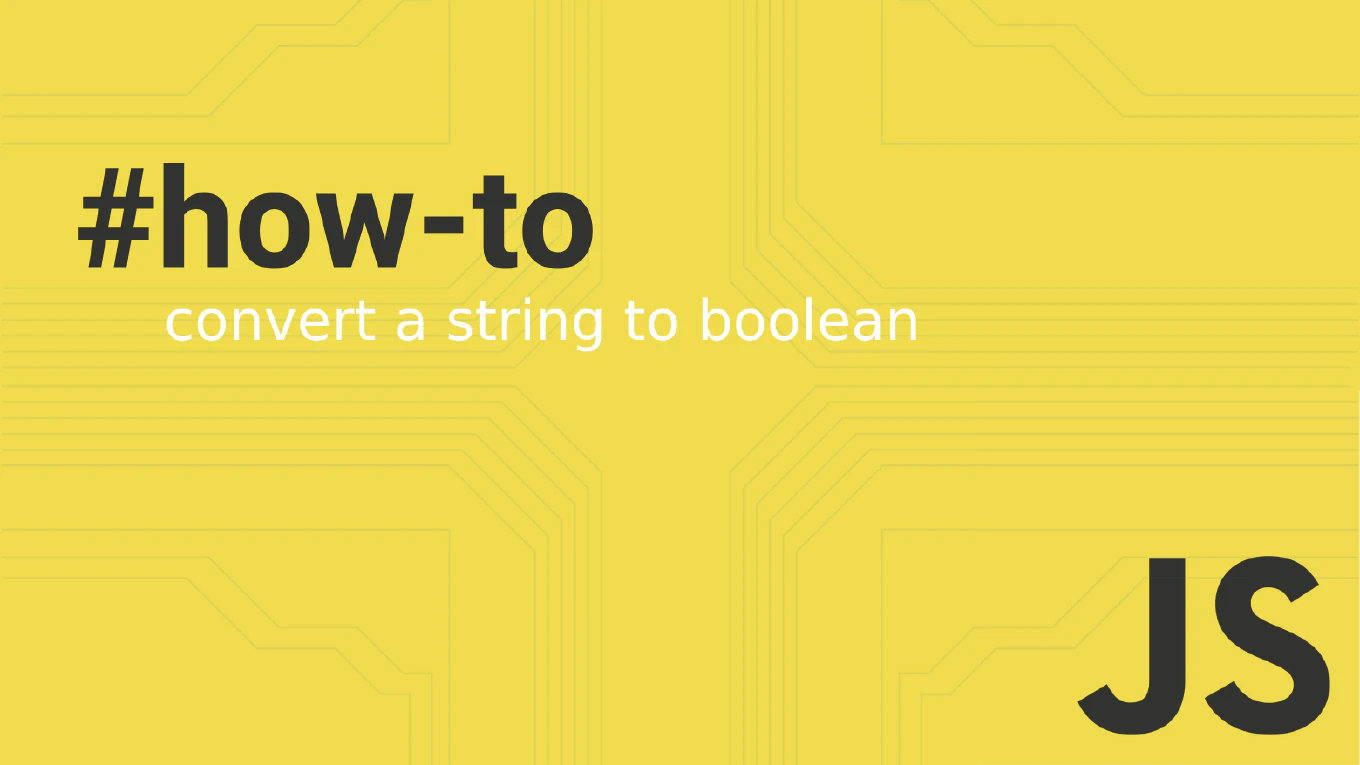
Working with different data types is a common task in JavaScript development. One frequent requirement is to convert a string to a boolean value. This article explores various methods to achieve this conversion, ensuring your code handles truthy values and false conditions accurately.
How to show or hide elements in React? A Step-by-Step Guide.
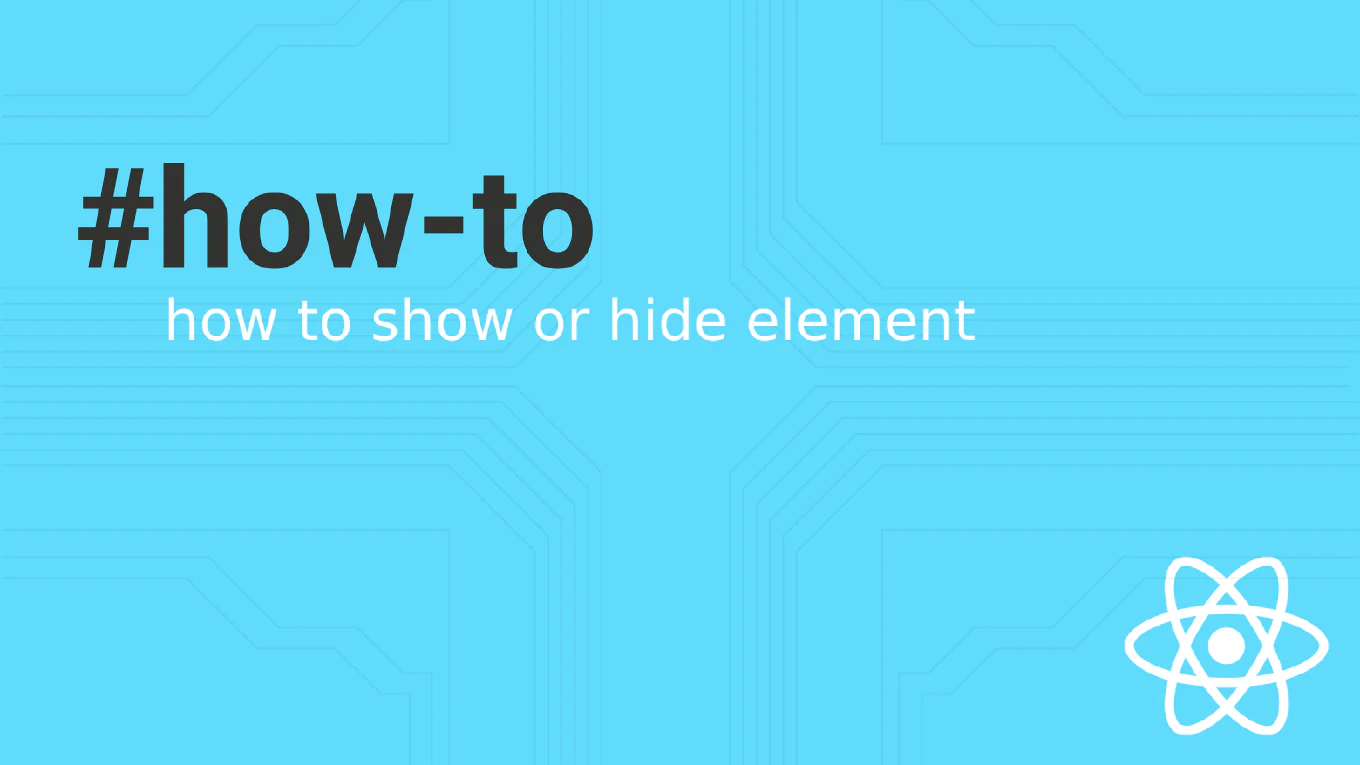
In modern web development, creating dynamic user interfaces is essential. The React library, a popular JavaScript tool, allows developers to build rich user interfaces by efficiently updating and rendering components based on data changes. One common requirement is to show or hide elements in React applications based on user interaction or application state. This article will guide you through different methods to show or hide elements in React using functional components, focusing on React 18+.
How to set focus on an input field after rendering in React
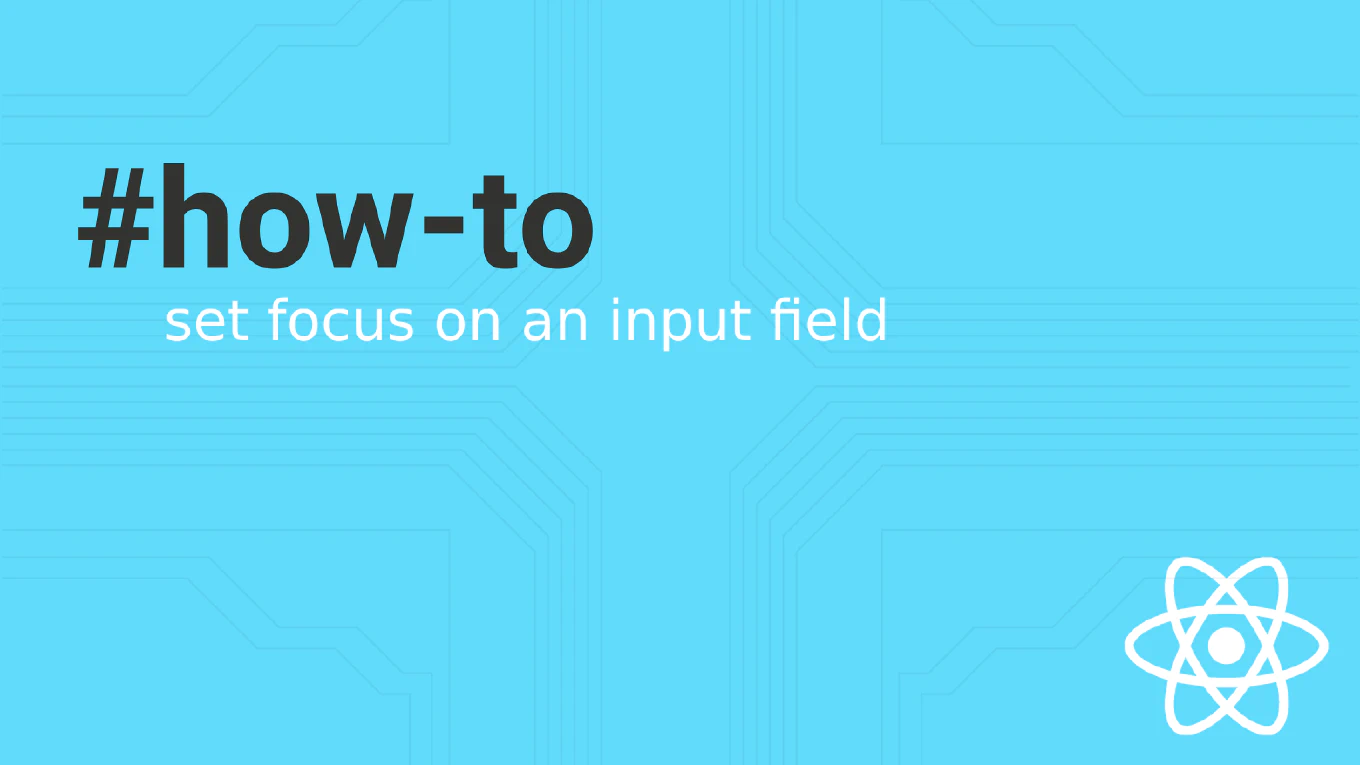
In modern React applications, managing focus on input elements is crucial for enhancing user experience. This practical guide will explore how to set focus on an input field after rendering in functional components using React 18+. We’ll delve into using the autoFocus
attribute, the useRef hook, and the useEffect
hook to achieve this.
Passing props to child components in React function components
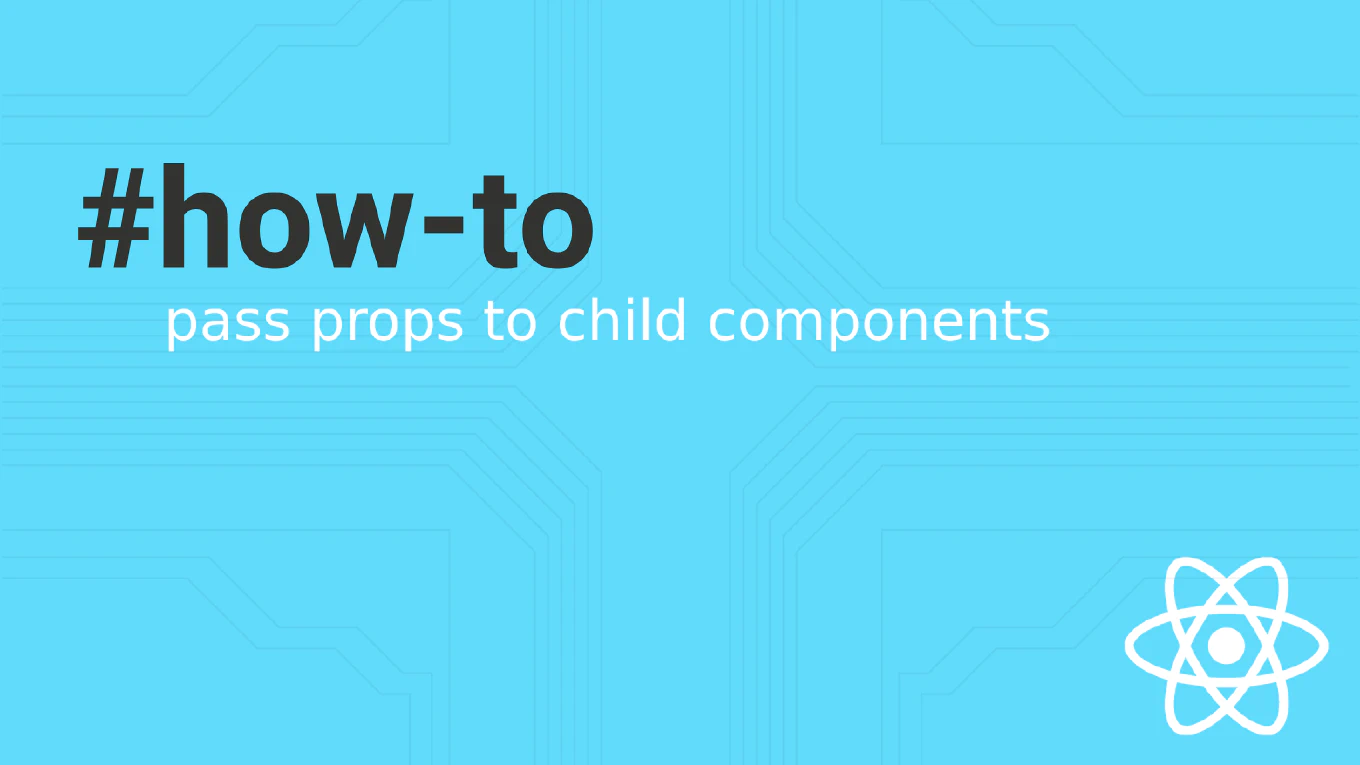
Passing props in React is a fundamental concept that allows data to flow between components. When building user interfaces with React, you often need to pass additional props from a parent component to its child components. This article explores how to pass props to child components, focusing on function components and best practices.
How to loop inside React JSX
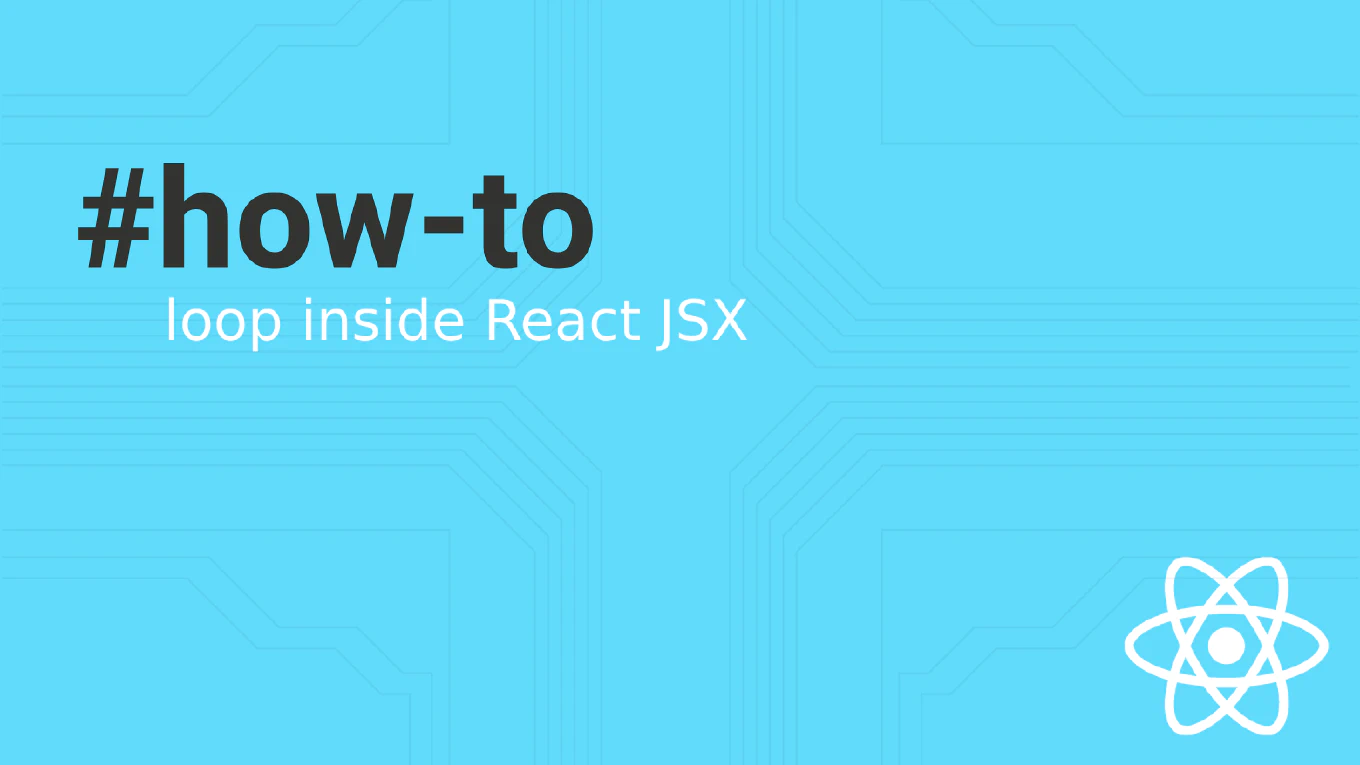
As a full stack developer working with React, mastering the art of looping inside React JSX is an essential skill. This guide will cover the different ways to render lists and elements using loops in React, focusing on the use of the map function, traditional loops, and best practices. Let’s explore how to create components dynamically and render lists efficiently using React JSX.
How to validate an email address in JavaScript
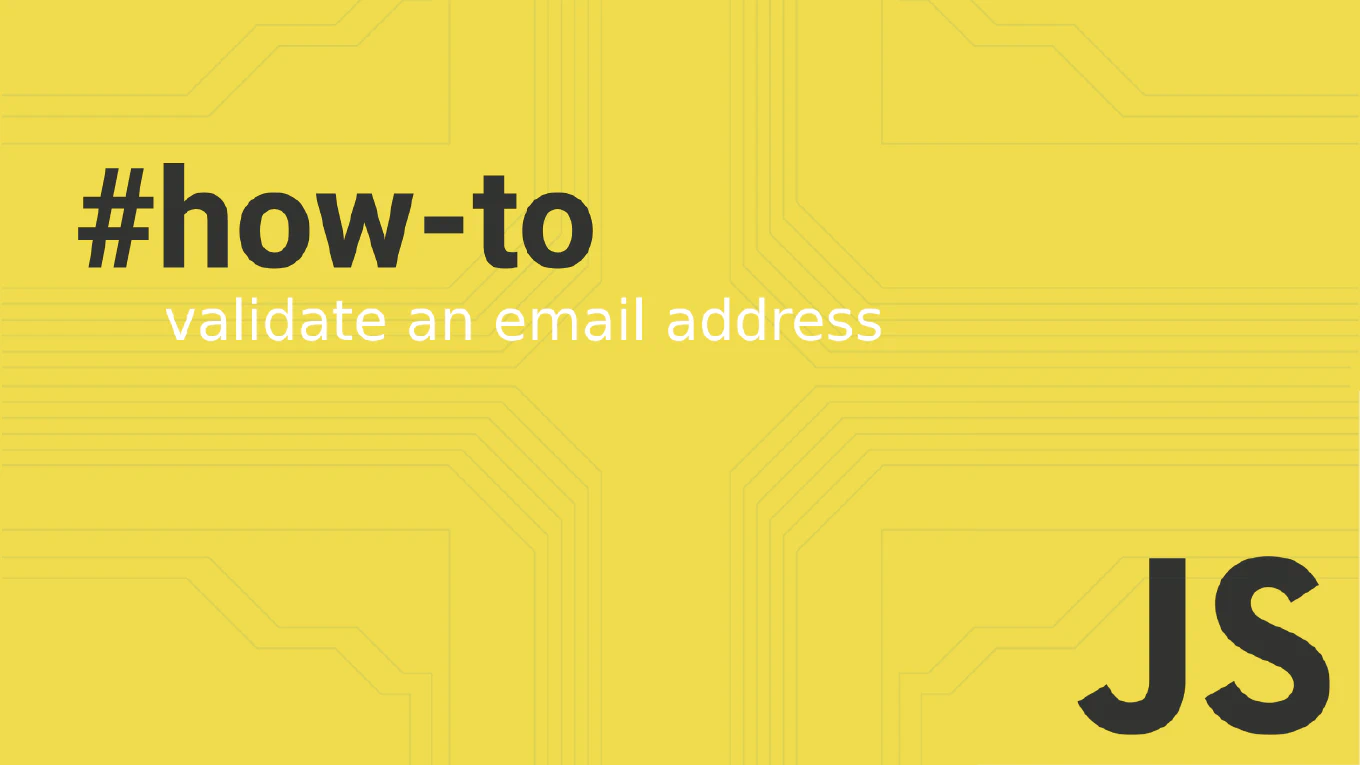
In web development, ensuring that user input is formatted correctly is crucial for maintaining data integrity and enhancing user experience. One common requirement is email validation. Implementing email validation in JavaScript allows developers to provide immediate feedback to users, ensuring that the email addresses entered are valid and correctly formatted. In this guide, we’ll explore how to implement email validation in JavaScript, using regular expressions and other techniques to validate email addresses effectively. We will also provide practical examples and methods using JavaScript code that you can directly copy and paste.
What is the difference between typeof and instanceof in JavaScript
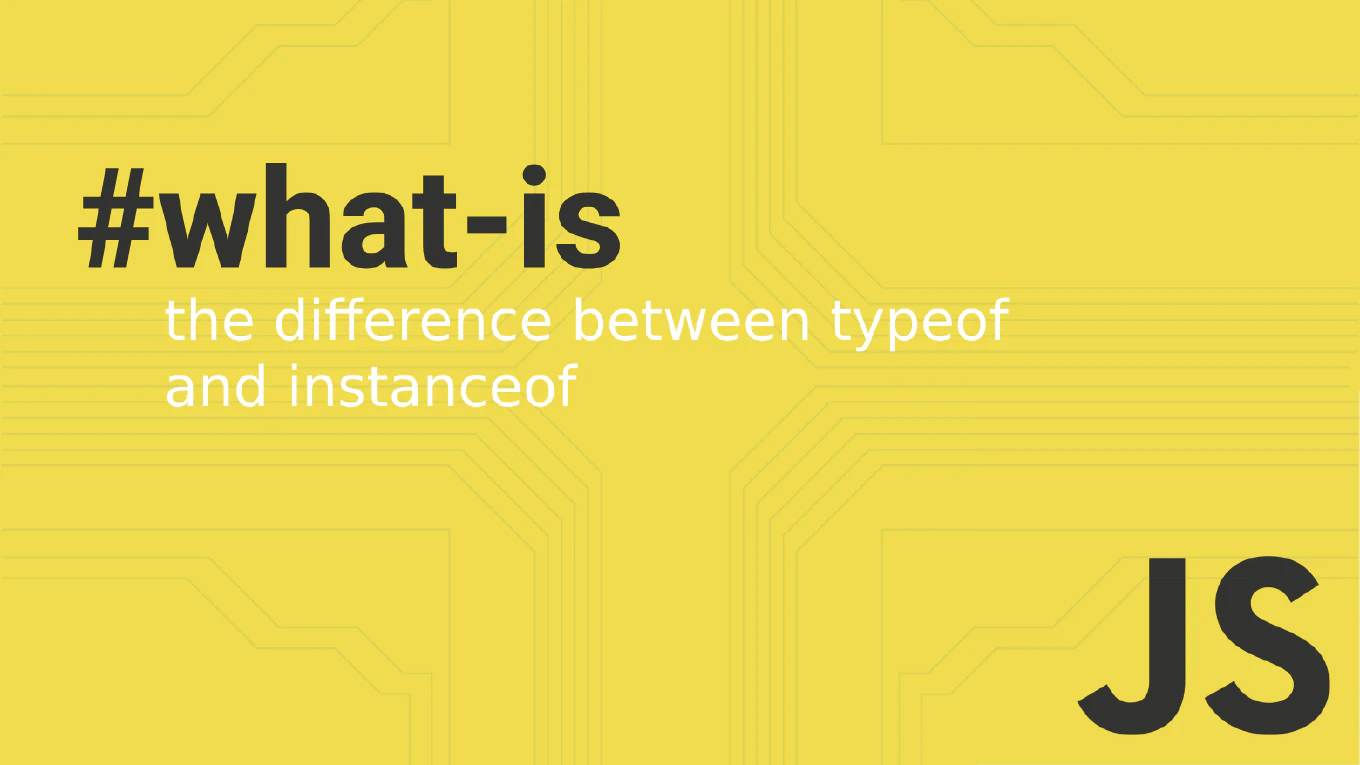
As a JavaScript developer, you might often wonder how to correctly identify the type of a variable or determine if an object belongs to a particular class. Two operators that help with this are typeof
and instanceof
. While they may seem similar, they serve different purposes and work in distinct ways. This article will break down these operators, explain their differences, and guide you on when to use each.
How to conditionally add attributes to React components
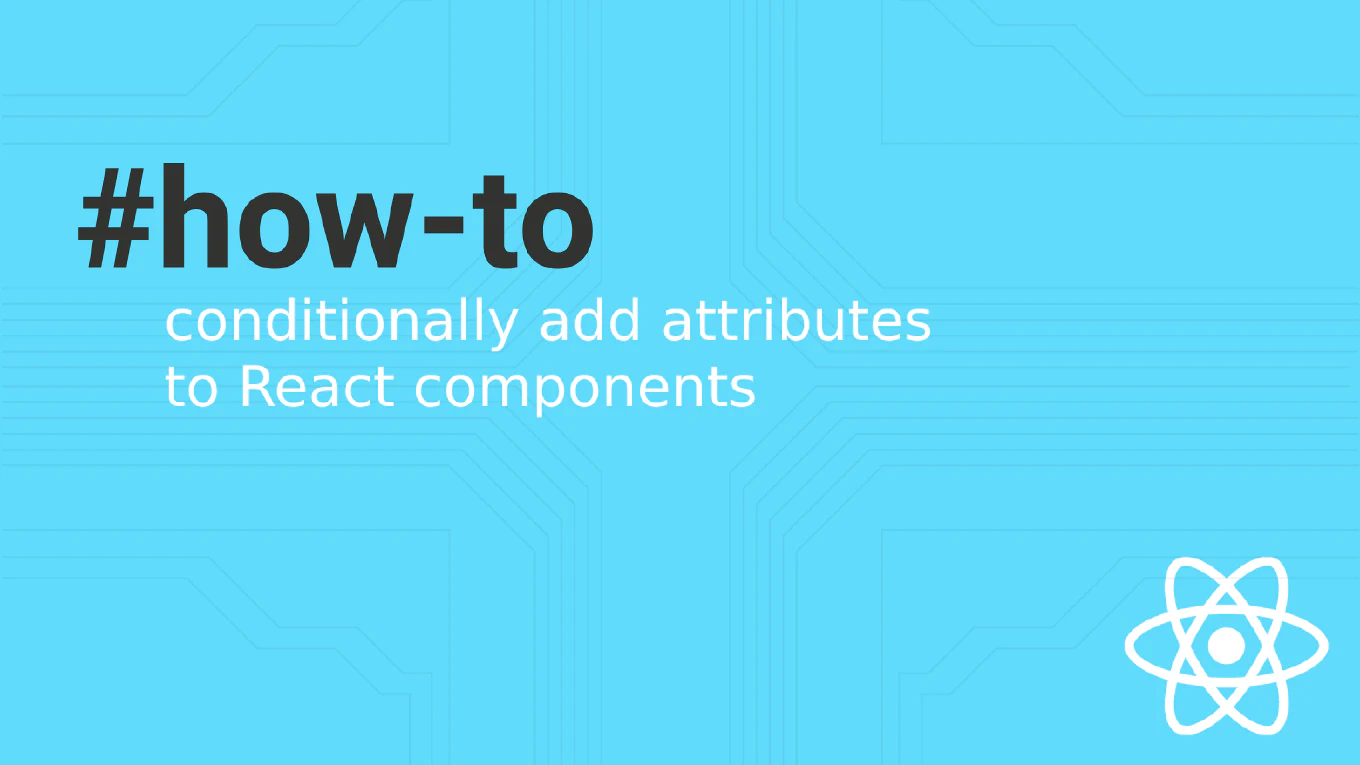
When building dynamic React applications, it’s common to encounter scenarios where you need to conditionally add attributes to React components based on user actions or state changes. This could involve enabling or disabling buttons, applying styles conditionally, or toggling event handlers based on different props.
How to force a React component to re-render
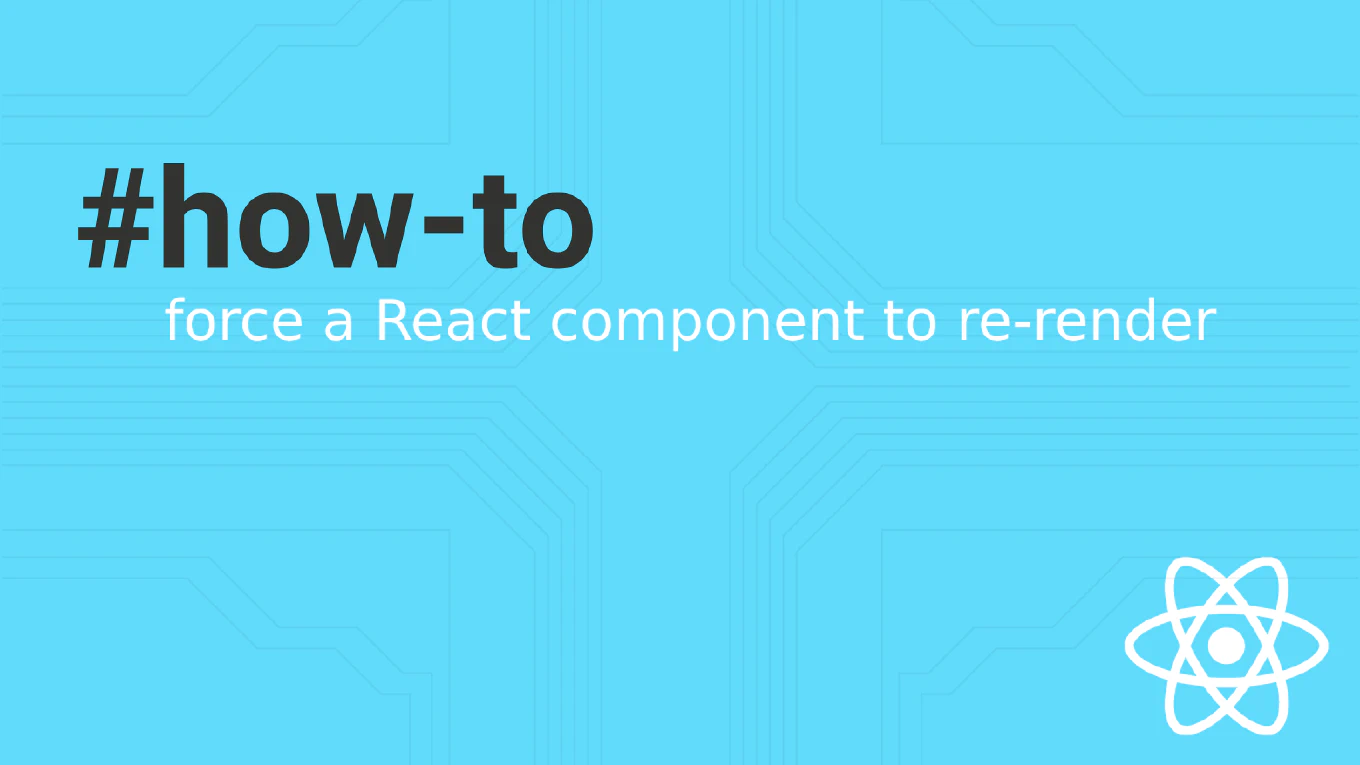
React triggers re-rendering when there’s a change in state or props. However, there are scenarios where you might need to force a component to re-render manually without explicitly modifying its state. This article will explore ways to force re-renders in both class and functional components to ensure the UI reflects the latest data.
What are the three dots `...` in JavaScript do?
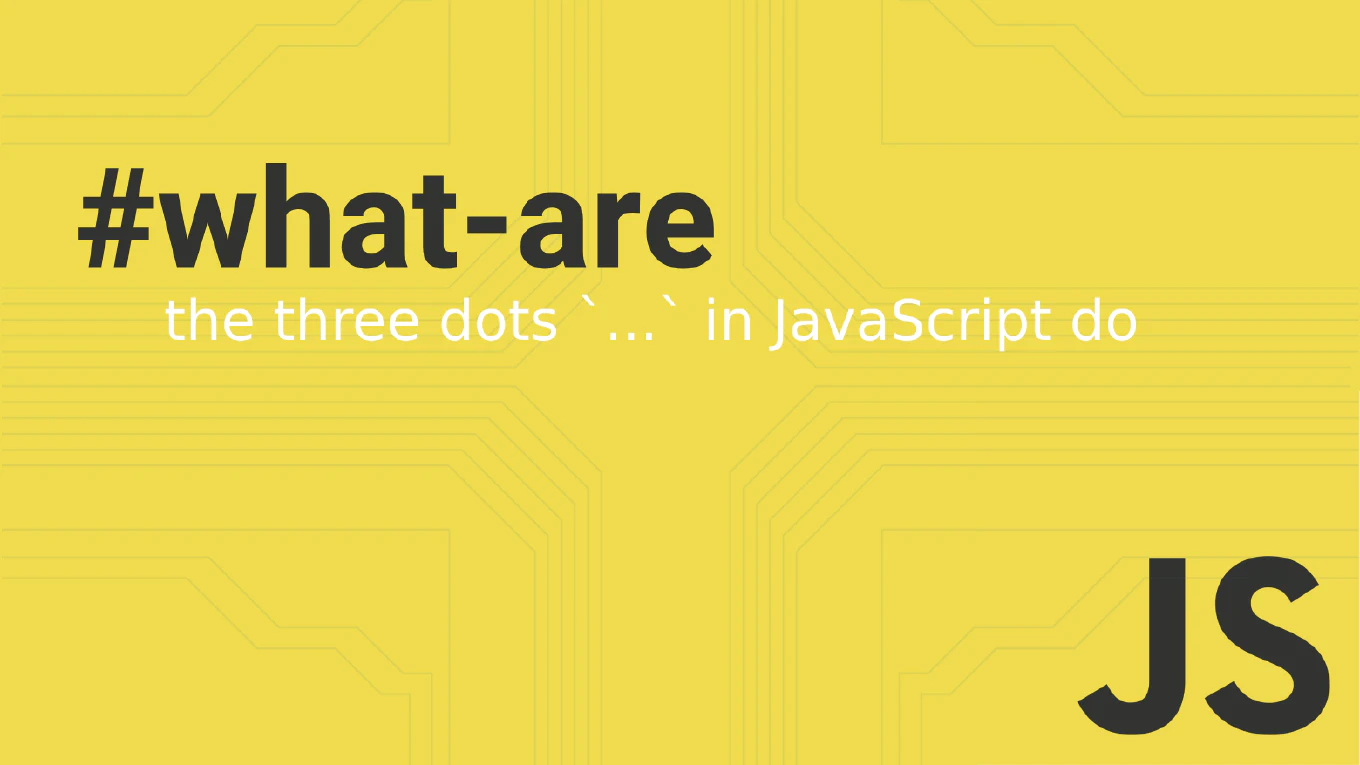
In JavaScript, the three dots operator (...
) is a versatile feature introduced in ES6. Known as the spread operator and rest parameter, these three dots have transformed how developers handle arrays, objects, and function parameters. Understanding this syntax is crucial for any software developer looking to write clean, efficient, and maintainable code. In this article, we’ll explore the various ways you can use the spread operator, spread syntax, and rest operator in your JavaScript code.