How to conditionally add attributes to React components
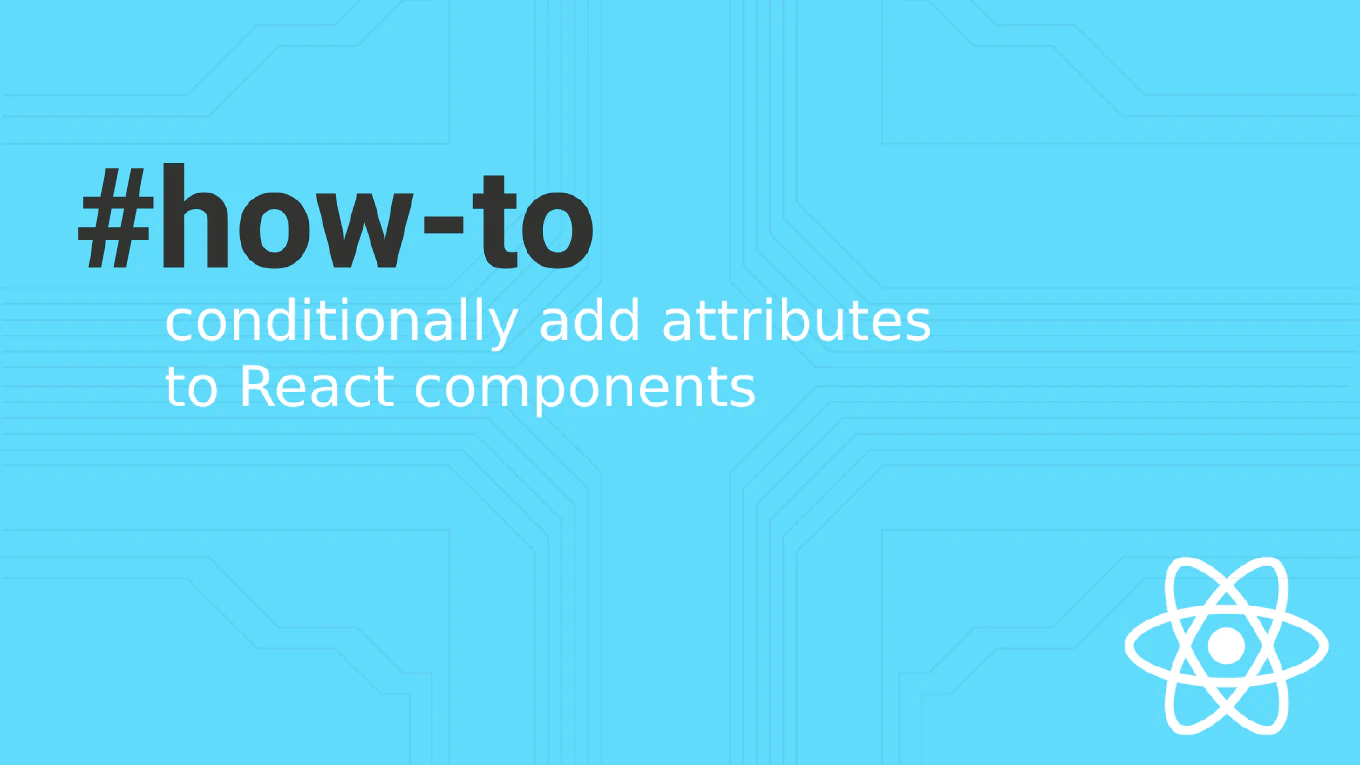
When building dynamic React applications, it’s common to encounter scenarios where you need to conditionally add attributes to React components based on user actions or state changes. This could involve enabling or disabling buttons, applying styles conditionally, or toggling event handlers based on different props.
This guide will explore several techniques to add attributes and conditional props to React components conditionally.
React Conditional Props
React components often require flexibility in managing props and attributes, particularly when different behaviors are triggered under certain conditions. For instance, a form button should only be enabled when all required fields are filled out. Managing conditional props allows your application to react dynamically to user interactions.
Inline Conditional Logic with Ternary Operator
One of the simplest and most commonly used methods for conditionally adding attributes is to use the ternary operator directly inside JSX. This approach is excellent for straightforward conditions like toggling the disabled attribute or className.
const SubmitButton = ({ isValid }) => {
return (
<button
disabled={!isValid}
className={isValid ? 'btn-primary' : 'btn-disabled'}
>
Submit
</button>
)
}
In this example, the ternary operator is used to conditionally set the disabled attribute and className based on the isValid prop. The disabled attribute is applied when the incomplete form allows the button to react to different states.
Using Logical Operators
You can also use logical operators like &&
or ||
for simpler cases. These operators allow you to add attributes only if a condition is met.
const ErrorMessage = ({ hasError }) => {
return (
<div>
{hasError && <p className='error'>Error occurred</p>}
</div>
)
}
This example uses the &&
operator to render the error message conditionally when hasError is true. This is a clean way to render JSX conditionally without a complex if statement.
The Spread Operator for Multiple Attributes
When managing multiple attributes in a React component, using the spread operator (...
) is a cleaner approach to dynamically applying props. This is especially useful when the component supports multiple configurations.
const Card = ({ isShadow }) => {
const cardProps = isShadow ? { className: 'card-shadow', onClick: handleClick } : {}
return <div {...cardProps}>Card Content</div>
}
In this case, the spread operator is used to apply multiple attributes to the div
conditionally. This method can help avoid cluttering JSX with too many ternary expressions.
Helper Functions for Complex Logic
You might want to separate the conditional logic into a helper function for more complex scenarios. This keeps your JSX cleaner and your logic reusable.
const getButtonProps = (isValid) => {
return isValid
? { className: 'btn-success', onClick: handleSubmit }
: { className: 'btn-disabled', disabled: true }
}
const SubmitButton = ({ isValid }) => {
return <button {...getButtonProps(isValid)}>Submit</button>
}
Here, the getButtonProps
function defines the conditional props logic. This approach is particularly useful when dealing with multiple props or attributes.
Conditional Rendering with a Generic Object
In cases where multiple conditions are at play, you can define a generic object and conditionally pass it into the component.
const avatarProps = isOnline
? { className: 'avatar-online', alt: 'User is online' }
: { className: 'avatar-offline', alt: 'User is offline' }
const Avatar = <img {...avatarProps} />
This generic object approach makes it easy to toggle all the properties of an avatar based on a single boolean value, keeping the JSX clean.
Handling Conditional Props in Forms
When working with forms, you may want to conditionally add attributes to an input element. For instance, a password input might be required based on the state of the form.
const InputField = ({ isRequired }) => {
return <input type='password' required={isRequired} />
}
Here, the required
attribute is conditionally applied using the props object, ensuring that the form element behaves differently based on a boolean value.
Conclusion
React’s flexibility allows you to easily conditionally add attributes and conditional props to components based on different states or interactions. Whether you use inline logic, if statements, the spread operator, or helper functions, there’s an appropriate option for every use case. These techniques help ensure that your React components remain dynamic, responsive, and easy to maintain while avoiding conflicting properties and enhancing performance.
By using these strategies, you can optimize your render method and improve the user experience in your React applications.