How to migrate CoreUI React Templates to Vite
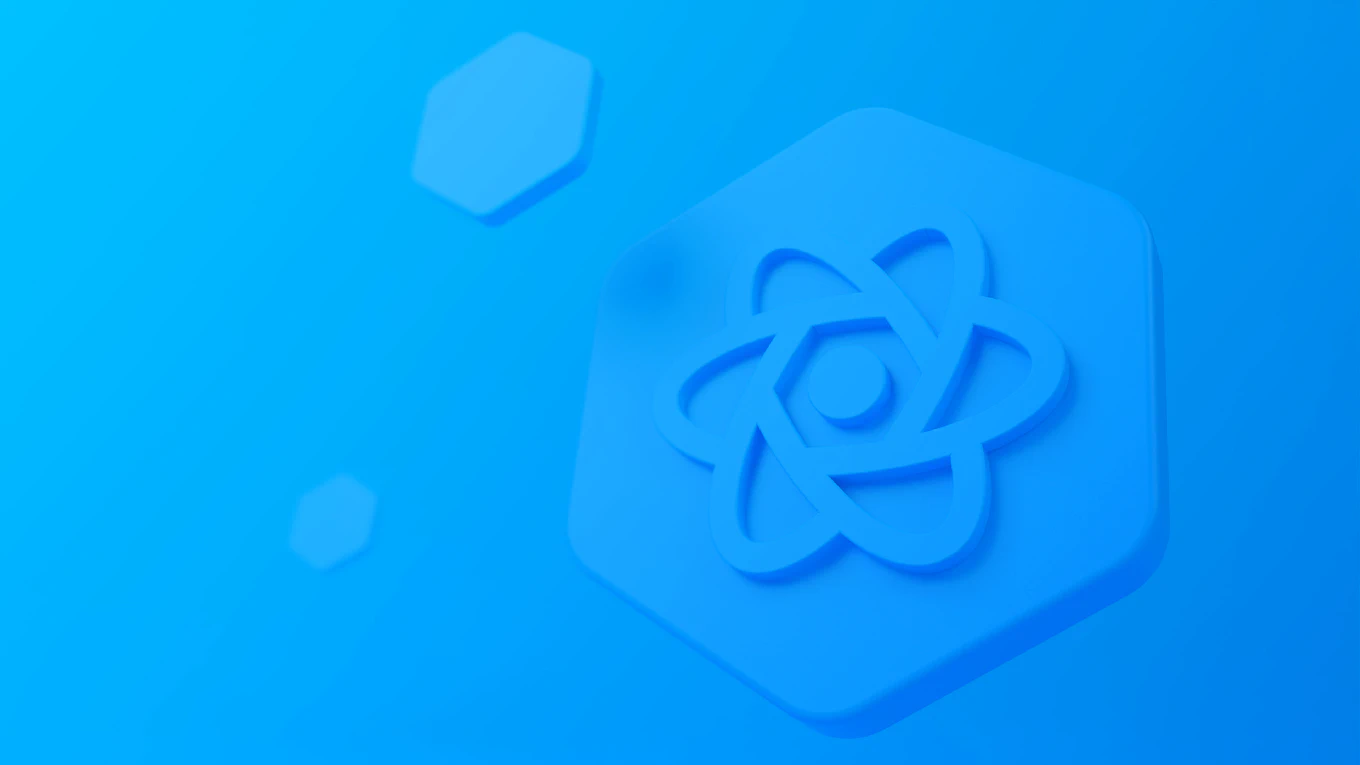
After carefully considering and evaluating our build and development tools in version 5 of our React.js admin templates, we have decided to migrate from Create React App (CRA) to Vite. This decision was driven by several key factors that align with our goals for a more efficient and modern development experience.
Vite offers faster start-up and update times during development due to its use of native ES modules over traditional webpack bundling. This leads to a significant improvement in the speed and responsiveness of our development process. Additionally, Vite’s out-of-the-box features, such as hot module replacement (HMR) and rich built-in features, meant we could streamline our toolchain and reduce configuration overhead.
Migrating to Vite can improve build times and a more responsive development experience. This guide will walk you through the process step by step. For a comprehensive view of the specific changes, you can also check this commit: - https://github.com/coreui/coreui-free-react-admin-template/commit/1d05bd4cd636da9a1ea884ea964085883323b93f
Prerequisites
- Ensure you have Node.js installed on your system.
- Familiarity with command line tools and npm/yarn package management.
- Existing CRA project that you wish to migrate.
Step 1: Update ESLint Configuration
Navigate to your project’s .eslintrc.js
file and remove the 'react-app'
and 'react-app/jest'
configurations from the extends
array. After the change, your ESLint configuration should resemble the following:
module.exports = {
parserOptions: {
ecmaVersion: 2020,
sourceType: 'module',
ecmaFeatures: {
jsx: true,
},
},
settings: {
react: {
version: 'detect',
},
},
extends: [
'plugin:react/recommended',
'plugin:prettier/recommended',
],
plugins: ['react', 'react-hooks'],
rules: {
// Custom ESLint rules can be added here
},
};
Step 2: Reorganize Public Assets
-
Move your
index.html
from thepublic
directory to the project root and update any%PUBLIC_URL%
references to./
.mv public/index.html .
-
Update the
<script>
tag inindex.html
to correctly point to your entry file, adjusting the path to match the new project structure:<script type="module" src="/src/index.jsx"></script>
Step 3: Install Vite and Update Dependencies
Install Vite and remove react-scripts:
npm install vite --save-dev
npm uninstall react-scripts
Add or update other dependencies:
npm install @vitejs/plugin-react autoprefixer eslint eslint-plugin-react eslint-plugin-react-hooks postcss --save-dev
npm uninstall @babel/plugin-proposal-private-property-in-object @testing-library/jest-dom @testing-library/react @testing-library/user-event web-vitals
Step 4: Setting Up Vite
Create a new file named vite.config.js
or vite.comfig.mjs
at the root of your project and fill it with the following basic Vite configuration:
import { defineConfig, loadEnv } from 'vite';
import react from '@vitejs/plugin-react';
import path from 'node:path';
import autoprefixer from 'autoprefixer';
export default defineConfig(({ mode }) => {
const env = loadEnv(mode, process.cwd(), '');
process.env = { ...process.env, ...env };
return {
base: './',
build: {
outDir: 'build',
},
css: {
postcss: {
plugins: [autoprefixer({})], // Add options as needed
},
},
define: {
'process.env': process.env,
},
esbuild: {
loader: 'jsx',
include: /src\/.*\.jsx?$/,
exclude: [],
},
optimizeDeps: {
force: true,
esbuildOptions: {
loader: {
'.js': 'jsx',
},
},
},
plugins: [react()],
resolve: {
alias: [{
find: 'src/',
replacement: `${path.resolve(__dirname, 'src')}/`,
}],
extensions: ['.mjs', '.js', '.ts', '.jsx', '.tsx', '.json', '.scss'],
},
server: {
port: 3000,
proxy: {
// Define proxies here (if needed)
},
},
};
});
Step 5: Updating package.json for Vite Commands
Adjust the scripts
section of your package.json
file to use Vite’s commands:
"scripts": {
"start": "vite",
"build": "vite build",
"serve": "vite preview"
},
Step 8: Update Main Application Entry Point
Modify the main entry point of your application to reflect the new imports and setup:
- Remove the import for
react-app-polyfill/stable
if you’re confident about browser compatibility or if Vite’s default polyfilling meets your needs. - Ensure that
core-js
is imported if you rely on its polyfills. - Remove
reportWebVitals
if you no longer need to track performance metrics, or if you will be using an alternative method in Vite. - Add the
Provider
component fromreact-redux
to wrap your<App />
, enabling Redux state management throughout your application.
Your src/index.js
should now look like this:
import React from 'react';
import { createRoot } from 'react-dom/client';
import 'core-js';
import App from './App';
import store from './store';
import { Provider } from 'react-redux';
createRoot(document.getElementById('root')).render(
<Provider store={store}>
<App />
</Provider>
);
Step 9: Clean Up Unused Files
Remove any CRA-specific files that are no longer needed:
jest.config.js
jsconfig.json
src/App.test.js
src/reportWebVitals.js
src/setupTests.js
This can be done using your file system or a command line interface.
rm jest.config.js jsconfig.json src/App.test.js src/reportWebVitals.js src/setupTests.js
Step 10: Finalize the Migration
Run your project with Vite to ensure everything works correctly:
npm start
If you encounter issues, consult the Vite documentation or rollback changes and migrate one step at a time.
Congratulations on migrating your project to Vite!