How to dynamically add, remove, and toggle CSS classes in React.js
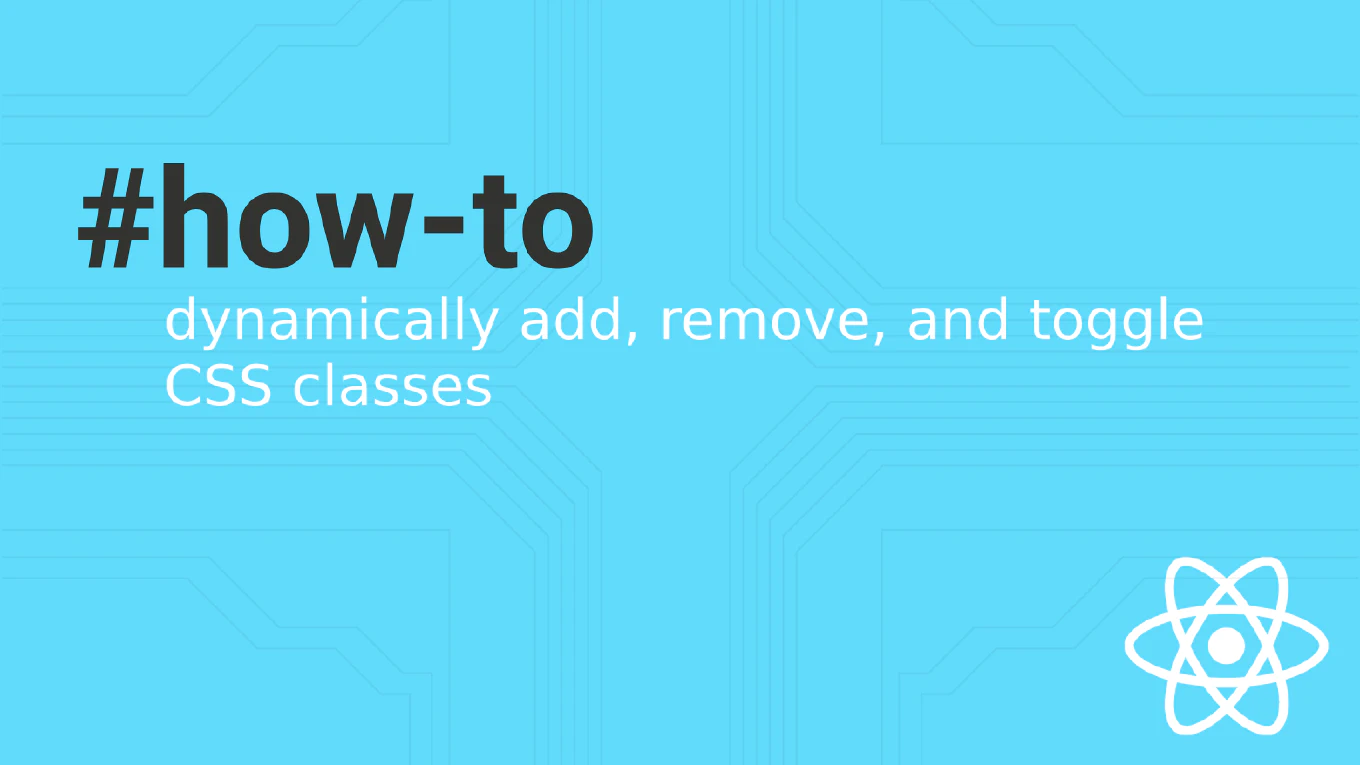
Managing CSS classes dynamically in a React application is a common requirement, especially when it comes to creating interactive components. This article will guide you through various techniques to add, remove, and toggle CSS classes in React.
Speed up your responsive apps and websites with fully-featured, ready-to-use open-source admin panel templates—free to use and built for efficiency.
Understanding the Basics of CSS Class Management in React
In React, CSS classes are applied to elements using the className
attribute. Unlike traditional HTML where you might directly manipulate class names via JavaScript, React provides a declarative way to manage CSS classes through state and props. This approach not only makes your code cleaner but also more predictable.
Adding and Removing a CSS Class Dynamically
To add or remove a CSS class dynamically, you typically rely on the component’s state. Here’s an example:
import React, { useState } from 'react'
import './App.css'
const ToggleButton = () => {
const [isActive, setIsActive] = useState(false)
const handleClick = () => {
setIsActive(!isActive)
}
return (
<div className="container">
<button onClick={handleClick} className={isActive ? 'active' : ''}>
Click me to toggle
</button>
</div>
)
}
export default ToggleButton
How It Works
In this example, the button toggles the active
CSS class based on the isActive
state. The className
attribute is dynamically set, allowing you to easily add or remove a CSS class as needed.
Toggling CSS Classes in React
Toggling a CSS class in React is a straightforward process that involves updating the component’s state. Let’s extend the previous example:
import React, { useState } from 'react'
import './App.css'
const ToggleButton = () => {
const [isActive, setIsActive] = useState(false)
const toggleClass = () => {
setIsActive(!isActive)
}
return (
<div className="container">
<button onClick={toggleClass} className={isActive ? 'active' : ''}>
Toggle Active Class
</button>
</div>
)
}
export default ToggleButton
What’s Happening Here?
In this code, the toggleClass
function flips the isActive
state between true
and false
, effectively allowing you to toggle a CSS class on the button.
Managing Multiple CSS Classes
Sometimes, you may need to manage multiple classes on an element. This can be achieved by concatenating class names or using a helper library like classnames
:
import React, { useState } from 'react'
import classNames from 'classnames'
import './App.css'
const MultiClassButton = () => {
const [isActive, setIsActive] = useState(false)
const [isHighlighted, setIsHighlighted] = useState(false)
const toggleClasses = () => {
setIsActive(!isActive)
setIsHighlighted(!isHighlighted)
}
return (
<div className="container">
<button
onClick={toggleClasses}
className={classNames({ 'active': isActive, 'highlight': isHighlighted })}
>
Toggle Multiple Classes
</button>
</div>
)
}
export default MultiClassButton
Using classnames
for Conditional Styling
Here, the classnames
library is used to dynamically toggle multiple classes based on state. The classNames
function helps in conditionally applying classes in a clean and readable way, allowing you to manage and add CSS classes more efficiently.
Advanced Example: Managing Complex CSS Logic with classnames
In more complex scenarios, you might need to apply different classes based on multiple conditions, which can be neatly handled using classnames
. Here’s an advanced example:
import React, { useState } from 'react'
import classNames from 'classnames'
import './App.css'
const AdvancedButton = () => {
const [isActive, setIsActive] = useState(false)
const [isHighlighted, setIsHighlighted] = useState(false)
const [isDisabled, setIsDisabled] = useState(false)
const toggleClasses = () => {
setIsActive(!isActive)
setIsHighlighted(!isHighlighted)
}
const buttonClasses = classNames({
'active': isActive,
'highlight': isHighlighted,
'disabled': isDisabled,
'rounded': true, // Always apply 'rounded' class
})
return (
<div className="container">
<button
onClick={toggleClasses}
className={buttonClasses}
disabled={isDisabled}
>
Advanced Toggle Button
</button>
<button onClick={setIsDisabled(true)} className="disable-button">
Disable Button
</button>
</div>
)
}
export default AdvancedButton
Understanding the Advanced Example
In this advanced example:
isActive
,isHighlighted
, andisDisabled
: These states control the application of the respective classes.classnames
: Theclassnames
function combines multiple classes into a single string based on the conditions, making it easier to toggle CSS classes based on complex logic.rounded
Class: This class is always applied, demonstrating that you can mix static and dynamic classes.
This setup allows for complex CSS styling scenarios where you might need to apply multiple classes based on different conditions, providing a flexible and powerful way to add CSS classes and manage your CSS in React components.
Inline Styles vs. CSS Classes
While dynamically adding/removing CSS classes is a powerful approach, sometimes you might need to apply styles directly using inline styles. Inline styles can be managed similarly by using the style
attribute and passing a style object:
import React, { useState } from 'react'
const InlineStyleButton = () => {
const [isActive, setIsActive] = useState(false)
const buttonStyle = {
backgroundColor: isActive ? 'blue' : 'gray',
padding: '10px',
textAlign: 'center'
}
return (
<button onClick={() => setIsActive(!isActive)} style={buttonStyle}>
Toggle Style
</button>
)
}
export default InlineStyleButton
When to Use Inline Styles
Here, the button’s backgroundColor
, padding
, and textAlign
properties are dynamically changed based on the isActive
state. This method is particularly useful for styles that are calculated dynamically or aren’t predefined in your CSS files.
Best Practices and Conclusion
When managing CSS in a React app, it’s important to choose the right approach depending on your use case:
- CSS Classes: Use CSS classes for styles that are reused across multiple components.
classnames
Library: Consider using libraries likeclassnames
for managing complex class logic and making it easier to add CSS classes based on conditions.- Inline Styles: Use inline styles for dynamic, one-off styles that don’t need to be reused.
By following these techniques, you can effectively control the appearance and behavior of your React components, creating a more interactive and polished user experience. The ability to toggle CSS class dynamically allows for a more responsive and engaging UI.
Remember, the key to mastering React is understanding how to leverage its declarative nature. Dynamically managing CSS classes is just one example of how you can harness this power to build robust and maintainable user interfaces.