Understanding and Resolving the “Objects Are Not Valid as a React Child” Error in React Development
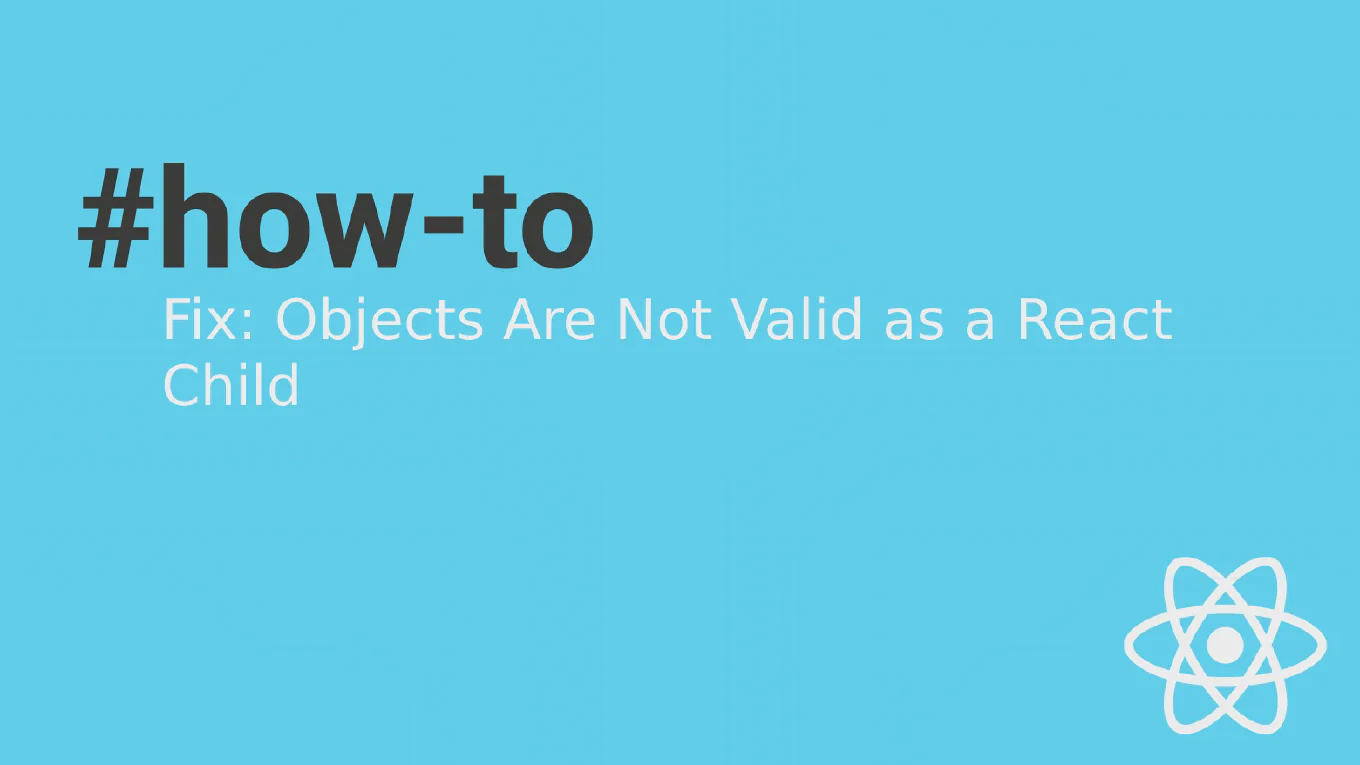
React has revolutionized the way developers build user interfaces, standing out for its modular approach through components and state management. As with any robust technology, developers, from beginners to veterans, encounter their share of error messages. One that often crops up is the Objects Are Not Valid as a React Child
error. In this comprehensive article, we’ll dissect the causes of this error and provide actionable solutions for overcoming it.
Understanding React Child and Valid Types
React is a popular framework for building web applications using a component-based architecture. In React, a child refers to any valid element that can be rendered within a parent component. These children can be strings, numbers, or other React elements. However, certain types of data are not considered valid React children.
One common error that developers encounter is the “Objects are not valid as a React child” error message. This error occurs when developers try to pass an array or an object as a child in their JSX code. React expects only valid React elements as children, such as strings, numbers, or React components.
To resolve this error, developers should ensure that they only pass valid React children to their parent components. If they need to render a collection of items, they can use array methods like map to transform the data into valid React elements before rendering them. They can also create a separate child component to render each item in the collection.
Understanding the concept of valid React children is important for both new and experienced developers working with React. By adhering to the valid types and following React’s guidelines, developers can avoid the common error of trying to render non-primitive types directly as children.
Introduction to the Error
The error message Objects Are Not Valid as a React Child signals that a React component expects a valid element—such as strings, numbers, or React elements—as its child but received an object or an array, which are non-serializable data types. Here’s a closer look at the error message you might encounter:
Warning: Objects are not valid as a React child (found: object with keys {key}). If you meant to render a collection of children, use an array instead.
This error typically occurs when a developer inadvertently passes an object where React expects a child element.
Root Causes of the Error
React treats children elements as content between the opening and closing tags of a component. These can be strings, React elements, or components themselves—but not objects or arrays as they are iterable data structures and not serializable for rendering purposes.
Example of an Error-Inducing Code Snippet
Consider the following example:
class App extends React.Component {
render() {
const user = {
name: 'John Doe',
age: 20,
};
// Trying to render an object directly.
return <div>{user}</div>;
}
}
In this snippet, attempting to render an object (user
) directly as a React child triggers the infamous error.
Addressing Arrays and Objects
While both arrays and objects can lead to this error, it’s important to note that JavaScript views arrays as a specific type of object. Thus, the error message remains the same whether the child is an array or an object.
Wrong Approach
class App extends React.Component {
render() {
const users = [
{ name: 'Fred', age: 25 },
{ name: 'Kate', age: 22 },
{ name: 'Alan', age: 28 },
];
// Incorrectly attempting to render an array directly.
return <div>{users}</div>;
}
}
Rendering the users
array directly, as shown above, will trigger the error because an array is also considered an object.
Solving the Error
React developers can follow several approaches to prevent and fix the Objects Are Not Valid as a React Child
error.
Converting the Object to a String or Number
One immediate solution is serialization—converting the non-serializable object to a string or number.
class App extends React.Component {
render() {
const message = { text: 'Hello World' };
// Converting object to a string
return <div>{JSON.stringify(message)}</div>;
}
}
Properly Using Arrays in React
Avoid directly rendering arrays by employing the map()
function to iterate over the array items, creating valid React child elements.
class App extends React.Component {
render() {
const users = [
{ name: 'Fred', age: 25 },
{ name: 'Kate', age: 22 },
{ name: 'Alan', age: 28 },
];
return (
<div>
{users.map((user, index) => (
<div key={index}>
Name: {user.name}, Age: {user.age}
</div>
))}
</div>
);
}
}
Remember to include a unique key prop for each child element to help React manage and optimize the rendering process.
Utilizing Conditional Rendering
Conditional rendering can safeguard against invalid children by ensuring that only serializable data types are rendered.
class App extends React.Component {
render() {
const message = 'Hello World';
return (
<div>
{typeof message === 'string' ? message : 'Not a valid React child'}
</div>
);
}
}
Following Best Practices
To sidestep the issue, implement best practices such as always validating data types before rendering and treating arrays and objects properly within your components.
Best Practices & Preventions
- Data Validation: Ensure components receive the correct data type for children.
- Serialization: Serialize objects with
JSON.stringify()
before attempting to render them as child elements. - Array Iteration: Use array methods like
map()
to create serializable React children. - Conditional Rendering: Guard against incorrect data types.
Conclusion
The Objects Are Not Valid as a React Child
error is a gentle reminder of the data types React accepts for rendering. Understanding and applying the discussed solutions and practices will significantly mitigate encountering this error.
React developers are encouraged to share their encounters with this error in the comments below, including any unique solutions or insights not covered in this article. If you’re looking to deploy a React application and are in need of hosting services, consider exploring options like Kinsta’s Application Hosting service, which offers a free starting point. Your experiences and solutions are invaluable learning opportunities for the wider developer community.