Understanding and Resolving the “React Must Be in Scope When Using JSX
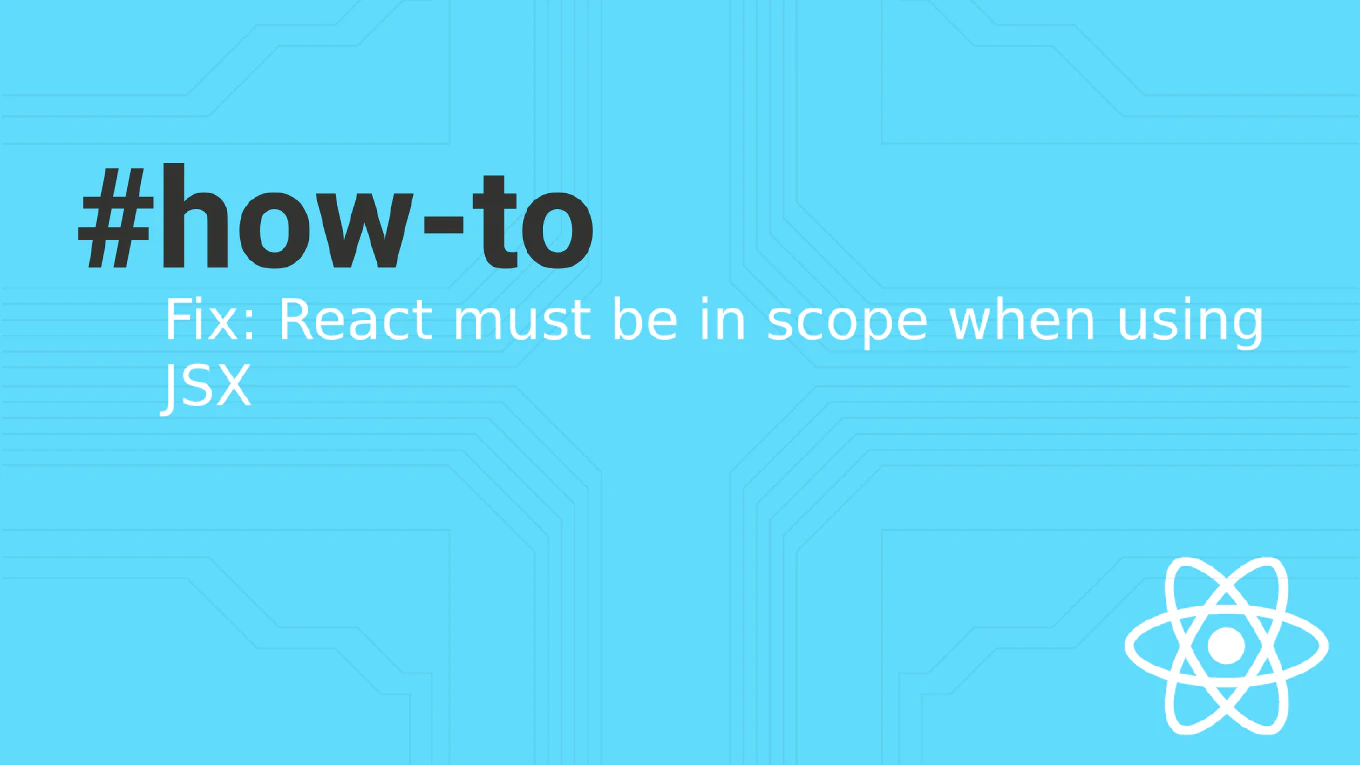
React developers often encounter the error message “React must be in scope when using JSX.” This common error can be confusing, especially for beginners. In this article, we will explore why this error occurs, how to fix it, and best practices to avoid it in the future.
What Causes the “React Must Be in Scope When Using JSX” Error?
JSX (JavaScript XML) is a syntax extension that enables developers to write code similar to HTML in JavaScript. Browsers do not understand JSX directly, so a toolchain is needed to transform JSX into standard JavaScript. In React versions prior to 17, this transformation involves converting JSX into React.createElement()
calls. This means that React must be in scope when using JSX.
// JSX Code
const App = () => {
return <h1>Hello, World!</h1>
}
// Transformed JavaScript Code
const App = () => {
return React.createElement('h1', null, 'Hello, World!')
}
If React is not properly imported, the above JavaScript code will throw errors. This is a common error developers face, especially when working with older versions of the React library.
Fixing the “React Must Be in Scope When Using JSX” Error
The fix for this error depends on the version of React you are using.
React Versions Prior to 17
In older versions of React, you need to ensure that React is imported correctly at the top of your JS file. Here’s how you can do it:
// Correct Import
import React from 'react'
A common mistake is using an incorrect import statement:
// Incorrect Import
import react from 'react'
React 17 and Higher
With React 17, a new JSX transform was introduced that automatically imports the necessary functions from the React package. This means you no longer need to explicitly import React in every file that uses JSX. However, you might still encounter this error if your ESLint configuration is outdated.
Updating ESLint Configuration
To fix this error in React 17 and above, you need to update your ESLint configuration. Add the following rules to your .eslintrc.js
or .eslintrc.json
file:
"rules": {
"react/react-in-jsx-scope": "off",
"react/jsx-uses-react": "off"
}
Alternatively, update the eslintConfig object in your package.json
:
{
"eslintConfig": {
"extends": ["react-app", "react-app/jest"],
"rules": {
"react/jsx-uses-react": "off",
"react/react-in-jsx-scope": "off"
}
}
}
Additional Solutions
If the error persists, here are additional steps you can take:
-
Update Create React App: Ensure that your project’s react-scripts are up to date.
npm install react-scripts@latest
-
Reinstall Dependencies: Delete the
node_modules
folder andpackage-lock.json
file, then reinstall the dependencies.rm -rf node_modules package-lock.json npm install
-
Update React and React-DOM: Make sure you are using the latest versions of React and React-DOM.
npm install react@latest react-dom@latest
Best Practices to Avoid Errors in React project
To avoid encountering the “React must be in scope when using JSX” error, follow these best practices:
- Keep Dependencies Updated: Regularly update your project dependencies to the latest versions.
- Check ESLint Configurations: Ensure your ESLint configurations are compatible with your React version.
- Review Import Statements: Always double-check your import statements for correctness.
Conclusion
The “React must be in scope when using JSX” error is a common issue that can be easily fixed by ensuring React is properly imported or by updating your ESLint configurations. By following the steps outlined in this article, you can prevent this error from occurring and streamline your React development process.