How to force a React component to re-render
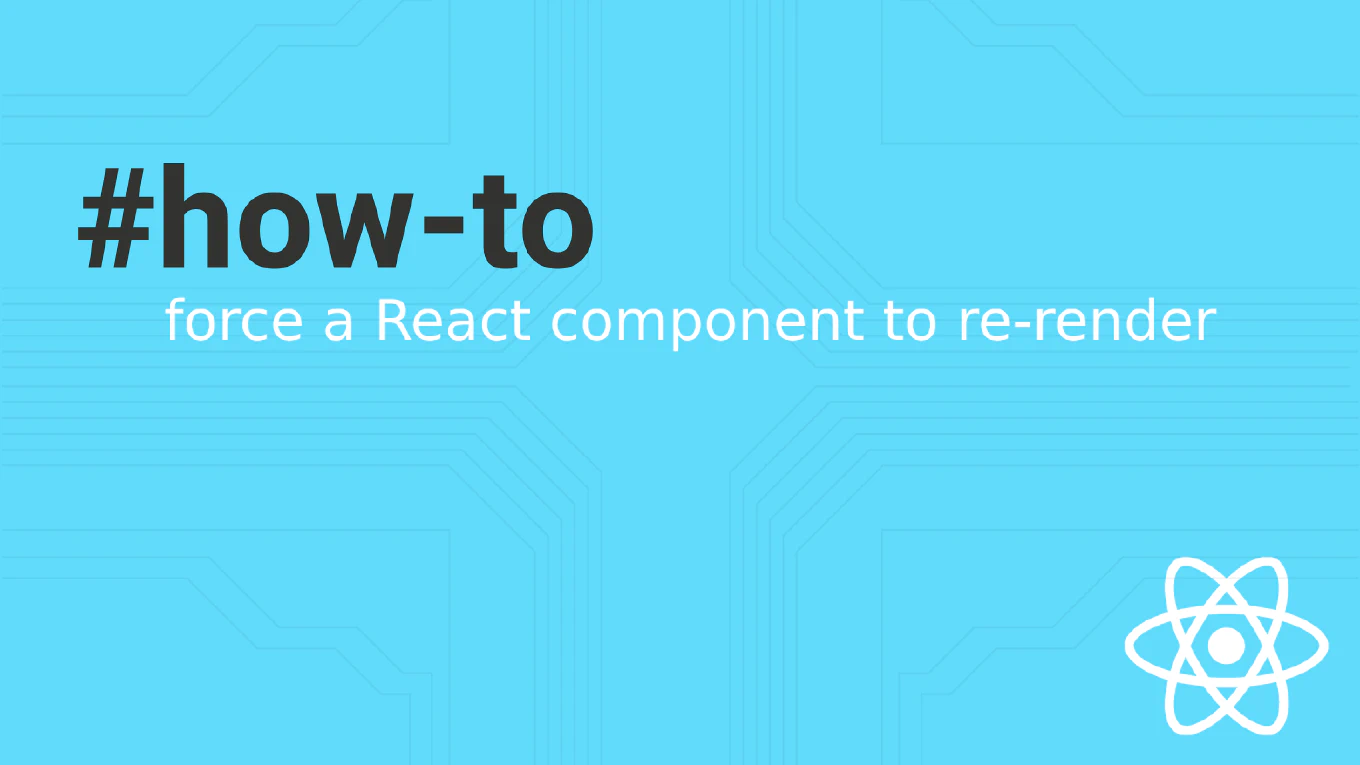
React triggers re-rendering when there’s a change in state or props. However, there are scenarios where you might need to force a component to re-render manually without explicitly modifying its state. This article will explore ways to force re-renders in both class and functional components to ensure the UI reflects the latest data.
Speed up your responsive apps and websites with fully-featured, ready-to-use open-source admin panel templates—free to use and built for efficiency.
Why Force a Re-render?
You might need to force a re-render in a React app for several reasons. For example, a component might depend on external data that doesn’t trigger the usual React re-rendering process. You might also need to refresh the UI due to third-party libraries that bypass React’s built-in state management. Another everyday use case is preventing unnecessary re-renders when optimizing performance.
While react developers often prefer to rely on state and props for re-rendering, there are situations where manually triggering the process becomes necessary to maintain a seamless user experience. However, forcing re-renders should be done sparingly to avoid performance degradation in your react application.
Techniques to Force a React Component to Re-render
1. Using forceUpdate
in Class Components
For class components, React provides the forceUpdate
method. This method can be called when you must re-render the component without updating the state directly. This simple but often unnecessary re-render technique can bypass React’s shouldComponentUpdate lifecycle method.
class MyComponent extends React.Component {
render() {
return (
<div>
<button onClick={() => this.forceUpdate()}>Force Re-render</button>
</div>
)
}
}
By calling this.forceUpdate()
, you trigger the component’s render method without affecting the component’s state. This is particularly useful for class components but should be used with caution, as it may lead to unnecessary re-renders that can affect react performance.
2. Changing the key
Prop
Updating the key
prop is another effective way to force re-rendering in both class and functional components. React uses the key
attribute to identify react components uniquely. When the key
changes, React treats the component as new, forcing a full re-render.
const MyComponent = () => {
const [key, setKey] = React.useState(0)
return (
<div key={key}>
<button onClick={() => setKey(key + 1)}>Force Re-render</button>
</div>
)
}
In this example, changing the key prop triggers re-rendering the entire component tree, including any child components. This method is helpful when you want to reset the component state or when dealing with dynamic lists of app components.
3. Using the useReducer
Hook
The useReducer
hook can be an efficient way to manage forced re-renders in functional components. It allows you to trigger the re-rendering process by updating a simple counter, which causes React to re-render the component.
const MyComponent = () => {
const [, forceUpdate] = React.useReducer(x => x + 1, 0)
return (
<div>
<button onClick={forceUpdate}>Force Re-render</button>
</div>
)
}
This approach mimics the behavior of setState without maintaining a state variable. Each time the forceUpdate
function is called, the component re-renders because the internal state changes. This is useful when dealing with external factors that require a refresh of the component tree.
4. Custom Hook for Force Re-renders
Creating a custom hook is a reusable way to force re-rendering in a functional component. This hook toggles a piece of state to force a re-render whenever necessary.
function useForceUpdate() {
const [, setToggle] = React.useState(false)
return () => setToggle(toggle => !toggle)
}
const MyComponent = () => {
const forceUpdate = useForceUpdate()
return (
<div>
<button onClick={forceUpdate}>Force Re-render</button>
</div>
)
}
The custom hook encapsulates the re-rendering logic, making it easy to apply across different components without duplicating code.
Downsides of Forcing Re-renders
While it’s possible to force react components to re-render, it’s essential to understand the trade-offs:
-
Performance: Forced re-renders can bypass React’s optimization, leading to performance issues, especially in large applications. This contradicts React’s principle of only updating the virtual DOM where necessary.
-
Unnecessary Re-renders: Overusing these methods may lead to unnecessary re-renders, affecting both parent and descendant components in the entire component tree.
-
Complexity: Forcing re-renders can make the app harder to debug, complicating React’s reconciliation process.
Best Practices to Prevent Unnecessary Re-renders
To avoid the pitfalls of forced re-renders, focus on optimizing performance by minimizing unnecessary updates. You can prevent unnecessary re-renders using React’s memoization techniques like React.memo
for functional components or shouldComponentUpdate
for class components. Additionally, efficient use of state and props ensures that React only updates components when there’s a genuine change.
Conclusion
While React’s state and props system handles most re-rendering needs, there are cases where forcing a re-render is necessary. Whether using forceUpdate
, manipulating the key
prop, or leveraging hooks like useReducer
or custom hooks, these techniques provide flexibility for dealing with complex re-rendering scenarios. However, the potential performance impact should always be considered, and the aim should be to optimize re-renders wherever possible.