How to loop inside React JSX
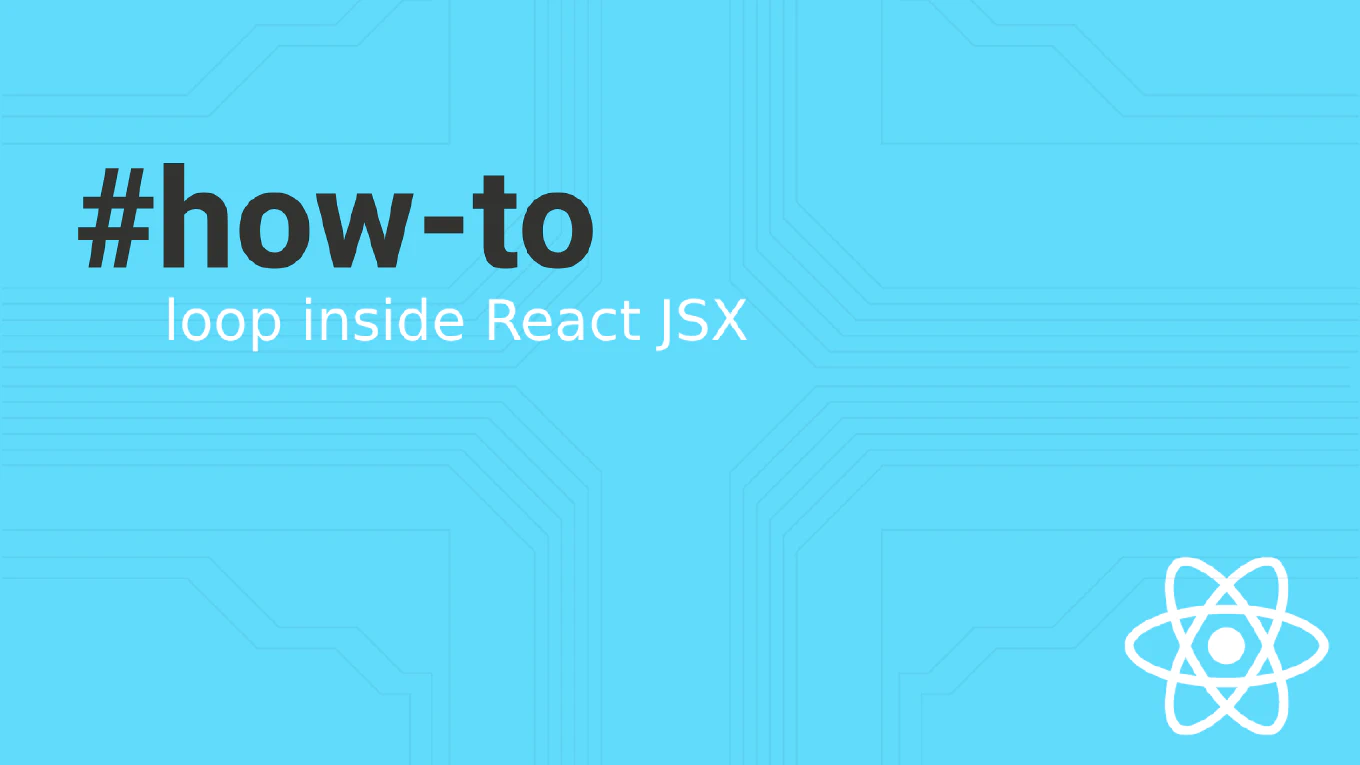
As a full stack developer working with React, mastering the art of looping inside React JSX is an essential skill. This guide will cover the different ways to render lists and elements using loops in React, focusing on the use of the map function, traditional loops, and best practices. Let’s explore how to create components dynamically and render lists efficiently using React JSX.
Understanding JSX and Its Limitations
JSX (JavaScript XML) is a JavaScript syntax extension that allows you to write HTML-like code within JavaScript files. However, normal JavaScript constructs, like for
loops, aren’t directly supported within JSX because they are statements, not expressions. Therefore, React developers need to rely on function calls and methods like map to achieve dynamic rendering.
Using the map
Function
The most common and recommended way to render lists in React is by using the map
method. The map
function creates a new array by calling a provided function on every element in the calling array.
Example: Rendering a List of Items
import React from 'react';
const items = ['Apple', 'Banana', 'Cherry'];
function FruitList() {
return (
<ul>
{items.map((item, index) => (
<li key={index}>{item}</li>
))}
</ul>
);
}
export default FruitList;
In this code example:
- We use the
map
function to iterate over theitems
array. - For each item, we return a
<li>
element containing the item. - We provide a
key
attribute, which is essential for helping React identify which items have changed, are added, or are removed.
Why Use map
?
- Declarative Syntax: map provides a clear, concise way to work with arrays and render elements.
- Dynamic Rendering: It simplifies how we iterate over data and render UI components.
- Functional Approach: React encourages the use of pure functions and immutable data structures.
Implementing for Loops Inside JSX
While the map
method is the go-to, there might be instances where a for
loop is necessary for more complex logic. However, for loops can’t be used directly in JSX.
Method 1: Using a Helper Function
function renderList(items) {
const listItems = []
for (let i = 0; i < items.length; i++) {
listItems.push(<li key={i}>{items[i]}</li>)
}
return listItems
}
function FruitList() {
return <ul>{renderList(items)}</ul>
}
Method 2: Using Immediately Invoked Function Expressions (IIFE)
function FruitList() {
return (
<ul>
{(() => {
const listItems = [];
for (let i = 0; i < items.length; i++) {
listItems.push(<li key={i}>{items[i]}</li>)
}
return listItems
})()}
</ul>
)
}
Limitations of Using for
Loops
- Verbosity: More code compared to using
map
. - Imperative: Less in line with React’s declarative nature.
- Readability: Can be harder to read and maintain.
Keys in Lists and the Importance of Unique Identifiers
When rendering lists, React requires a unique key
property to identify which elements have changed, been added, or removed. This is crucial for performance optimization.
Best Practices for Keys
- Use Unique IDs: If your data has unique IDs, use them as keys.
- Avoid Using Index as a Key: Using the array index can lead to issues, especially if the list can change.
items.map((item) => (
<li key={item.id}>{item.name}</li>
))
Common Mistakes and How to Avoid Them
Modifying the Original Array
Avoid mutating the original array when rendering lists.
// Bad Practice
const listItems = items.push(<li key={i}>{items[i]}</li>)
// Good Practice
const listItems = [...items, newItem]
Missing Return Statements
Ensure that your functions return the JSX elements.
items.map((item) => {
return <li key={item.id}>{item.name}</li>
})
Not Providing a Key Attribute
Always include the key
when rendering lists.
Performance Considerations
- Avoid Inline Functions: Define functions outside of the render method when possible.
- Memoization: Use
React.memo
oruseMemo
for performance optimization. - Efficient Keys: Use stable and unique keys to prevent unnecessary re-renders.
Best Practices for Looping in React
- Prefer
map
Overfor
Loops: Aligns with React’s declarative style. - Keep Components Pure: Avoid side effects in your render methods.
- Use Functional Components: Leverage hooks for state and lifecycle methods.
- Maintain Readability: Write code that is easy to understand and maintain.
Conclusion
Looping in React JSX doesn’t have to be complicated. By understanding the map
function and how to use loops efficiently, you can handle dynamic rendering in your React applications. Remember to always use unique keys and follow best practices for optimal performance.
Further Reading